Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / RoutedEventArgs.cs / 1 / RoutedEventArgs.cs
using System; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; using System.Collections.Specialized ; using System.Windows.Input; using System.Diagnostics; using MS.Internal; namespace System.Windows { ////// The container for all state associated /// with a RoutedEvent /// ////// public class RoutedEventArgs : EventArgs { #region Construction ////// constitutes the /// , /// , /// and /// /// /// /// Different /// can be used with a single /// /// /// The is responsible /// for packaging the , /// providing extra event state info, and invoking the /// handler associated with the RoutedEvent /// /// Constructor for ////// /// All members take default values public RoutedEventArgs() { } ////// /// /// /// defaults to null /// defaults to /// false /// defaults to null /// also defaults to null /// /// /// Constructor for /// The new value that the RoutedEvent Property is being set to public RoutedEventArgs(RoutedEvent routedEvent) : this( routedEvent, null) { } ////// /// Constructor for /// The new value that the SourceProperty is being set to /// The new value that the RoutedEvent Property is being set to public RoutedEventArgs(RoutedEvent routedEvent, object source) { _routedEvent = routedEvent; _source = _originalSource = source; } #endregion Construction #region External API ////// /// Returns the ///associated /// with this /// /// The public RoutedEvent RoutedEvent { get {return _routedEvent;} set { if (UserInitiated && InvokingHandler) throw new InvalidOperationException(SR.Get(SRID.RoutedEventCannotChangeWhileRouting)); _routedEvent = value; } } ///cannot be null /// at any time /// /// Changes the RoutedEvent assocatied with these RoutedEventArgs /// ////// Only used internally. Added to support cracking generic MouseButtonDown/Up events /// into MouseLeft/RightButtonDown/Up events. /// /// /// The new RoutedEvent to associate with these RoutedEventArgs /// internal void OverrideRoutedEvent( RoutedEvent newRoutedEvent ) { _routedEvent = newRoutedEvent; } ////// Returns a boolean flag indicating if or not this /// RoutedEvent has been handled this far in the route /// ////// Initially starts with a false value before routing /// has begun /// ////// Critical - _flags is critical due to UserInitiated value. /// PublicOK - in this function we're not setting UserInitiated - we're setting Handled. /// public bool Handled { [SecurityCritical ] get { return _flags[ HandledIndex ] ; } [SecurityCritical ] set { if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } if( TraceRoutedEvent.IsEnabled ) { TraceRoutedEvent.TraceActivityItem( TraceRoutedEvent.HandleEvent, value, RoutedEvent.OwnerType.Name, RoutedEvent.Name, this ); } // Note: We need to allow the caller to change the handled value // from true to false. // // We are concerned about scenarios where a child element // listens to a high-level event (such as TextInput) while a // parent element listens tp a low-level event such as KeyDown. // In these scenarios, we want the parent to not respond to the // KeyDown event, in deference to the child. // // Internally we implement this by asking the parent to only // respond to KeyDown events if they have focus. This works // around the problem and is an example of an unofficial // protocol coordinating the two elements. // // But we imagine that there will be some cases we miss or // that third parties introduce. For these cases, we expect // that the application author may need to mark the KeyDown // as handled in the child, and then reset the event to // being unhandled after the parent, so that default processing // and promotion still occur. // // For more information see the following task: // 20284: Input promotion breaks down when lower level input is intercepted _flags[ HandledIndex ] = value; } } ////// Returns Source object that raised the RoutedEvent /// public object Source { get {return _source;} set { if (UserInitiated && InvokingHandler) throw new InvalidOperationException(SR.Get(SRID.RoutedEventCannotChangeWhileRouting)); if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } object source = value ; if (_source == null && _originalSource == null) { // Gets here when it is the first time that the source is set. // This implies that this is also the original source of the event _source = _originalSource = source; OnSetSource(source); } else if (_source != source) { // This is the actiaon taken at all other times when the // source is being set to a different value from what it was _source = source; OnSetSource(source); } } } ////// Changes the Source assocatied with these RoutedEventArgs /// ////// Only used internally. Added to support cracking generic MouseButtonDown/Up events /// into MouseLeft/RightButtonDown/Up events. /// /// /// The new object to associate as the source of these RoutedEventArgs /// internal void OverrideSource( object source ) { _source = source; } ////// Returns OriginalSource object that raised the RoutedEvent /// ////// Always returns the OriginalSource object that raised the /// RoutedEvent unlike public object OriginalSource { get {return _originalSource;} } ////// that may vary under specific scenarios /// This property acquires its value once before the event /// handlers are invoked and never changes then on /// /// Invoked when the source of the event is set /// ////// Changing the source of an event can often /// require updating the data within the event. /// For this reason, the OnSource= method is /// protected virtual and is meant to be /// overridden by sub-classes of /// /// /// The new value that the SourceProperty is being set to /// protected virtual void OnSetSource(object source) { } ////// Also see /// /// Invokes the generic handler with the /// appropriate arguments /// ////// Is meant to be overridden by sub-classes of /// /// /// Generic Handler to be invoked /// /// /// Target on whom the Handler will be invoked /// protected virtual void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } InvokingHandler = true; try { if (genericHandler is RoutedEventHandler) { ((RoutedEventHandler)genericHandler)(genericTarget, this); } else { // Restricted Action - reflection permission required genericHandler.DynamicInvoke(new object[] {genericTarget, this}); } } finally { InvokingHandler = false; } } #endregion External API #region Operations // Calls the InvokeEventHandler protected // virtual method // // This method is needed because // delegates are invoked from // RoutedEventHandler which is not a // sub-class of RoutedEventArgs // and hence cannot invoke protected // method RoutedEventArgs.FireEventHandler internal void InvokeHandler(Delegate handler, object target) { InvokingHandler = true; try { InvokeEventHandler(handler, target); } finally { InvokingHandler = false; } } ///to provide /// more efficient invocation of their delegate /// /// Critical - access critical information, if this is a user initiated command /// TreatAsSafe - checking user initiated bit considered safe. /// internal bool UserInitiated { [SecurityCritical, SecurityTreatAsSafe ] [FriendAccessAllowed] // Also used by Framework. get { return _flags [ UserInitiatedIndex ] ; } } ////// Critical - access critical information, if this is a user initiated command /// [SecurityCritical] internal void MarkAsUserInitiated() { _flags [ UserInitiatedIndex ] = true; } ////// Critical - access critical information, if this is a user initiated command /// TreatAsSafe - clearing user initiated bit considered safe. /// [SecurityCritical, SecurityTreatAsSafe ] internal void ClearUserInitiated() { _flags [ UserInitiatedIndex ] = false ; } ////// Critical - _flags is critical due to UserInitiated value. /// TreatAsSafe - in this function we're not setting UserInitiated - we're setting InvokingHandler. /// private bool InvokingHandler { [SecurityCritical, SecurityTreatAsSafe ] get { return _flags[InvokingHandlerIndex]; } [SecurityCritical, SecurityTreatAsSafe ] set { _flags[InvokingHandlerIndex] = value; } } #endregion Operations #region Data private RoutedEvent _routedEvent; private object _source; private object _originalSource; ////// Critical - the UserInitiated flag value is critical. /// [SecurityCritical] private BitVector32 _flags; private const int HandledIndex = 1; private const int UserInitiatedIndex = 2; private const int InvokingHandlerIndex = 4; #endregion Data } ////// RoutedEventHandler Definition /// ///public delegate void RoutedEventHandler(object sender, RoutedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal.PresentationCore; using System.Collections.Specialized ; using System.Windows.Input; using System.Diagnostics; using MS.Internal; namespace System.Windows { /// /// The container for all state associated /// with a RoutedEvent /// ////// public class RoutedEventArgs : EventArgs { #region Construction ////// constitutes the /// , /// , /// and /// /// /// /// Different /// can be used with a single /// /// /// The is responsible /// for packaging the , /// providing extra event state info, and invoking the /// handler associated with the RoutedEvent /// /// Constructor for ////// /// All members take default values public RoutedEventArgs() { } ////// /// /// /// defaults to null /// defaults to /// false /// defaults to null /// also defaults to null /// /// /// Constructor for /// The new value that the RoutedEvent Property is being set to public RoutedEventArgs(RoutedEvent routedEvent) : this( routedEvent, null) { } ////// /// Constructor for /// The new value that the SourceProperty is being set to /// The new value that the RoutedEvent Property is being set to public RoutedEventArgs(RoutedEvent routedEvent, object source) { _routedEvent = routedEvent; _source = _originalSource = source; } #endregion Construction #region External API ////// /// Returns the ///associated /// with this /// /// The public RoutedEvent RoutedEvent { get {return _routedEvent;} set { if (UserInitiated && InvokingHandler) throw new InvalidOperationException(SR.Get(SRID.RoutedEventCannotChangeWhileRouting)); _routedEvent = value; } } ///cannot be null /// at any time /// /// Changes the RoutedEvent assocatied with these RoutedEventArgs /// ////// Only used internally. Added to support cracking generic MouseButtonDown/Up events /// into MouseLeft/RightButtonDown/Up events. /// /// /// The new RoutedEvent to associate with these RoutedEventArgs /// internal void OverrideRoutedEvent( RoutedEvent newRoutedEvent ) { _routedEvent = newRoutedEvent; } ////// Returns a boolean flag indicating if or not this /// RoutedEvent has been handled this far in the route /// ////// Initially starts with a false value before routing /// has begun /// ////// Critical - _flags is critical due to UserInitiated value. /// PublicOK - in this function we're not setting UserInitiated - we're setting Handled. /// public bool Handled { [SecurityCritical ] get { return _flags[ HandledIndex ] ; } [SecurityCritical ] set { if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } if( TraceRoutedEvent.IsEnabled ) { TraceRoutedEvent.TraceActivityItem( TraceRoutedEvent.HandleEvent, value, RoutedEvent.OwnerType.Name, RoutedEvent.Name, this ); } // Note: We need to allow the caller to change the handled value // from true to false. // // We are concerned about scenarios where a child element // listens to a high-level event (such as TextInput) while a // parent element listens tp a low-level event such as KeyDown. // In these scenarios, we want the parent to not respond to the // KeyDown event, in deference to the child. // // Internally we implement this by asking the parent to only // respond to KeyDown events if they have focus. This works // around the problem and is an example of an unofficial // protocol coordinating the two elements. // // But we imagine that there will be some cases we miss or // that third parties introduce. For these cases, we expect // that the application author may need to mark the KeyDown // as handled in the child, and then reset the event to // being unhandled after the parent, so that default processing // and promotion still occur. // // For more information see the following task: // 20284: Input promotion breaks down when lower level input is intercepted _flags[ HandledIndex ] = value; } } ////// Returns Source object that raised the RoutedEvent /// public object Source { get {return _source;} set { if (UserInitiated && InvokingHandler) throw new InvalidOperationException(SR.Get(SRID.RoutedEventCannotChangeWhileRouting)); if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } object source = value ; if (_source == null && _originalSource == null) { // Gets here when it is the first time that the source is set. // This implies that this is also the original source of the event _source = _originalSource = source; OnSetSource(source); } else if (_source != source) { // This is the actiaon taken at all other times when the // source is being set to a different value from what it was _source = source; OnSetSource(source); } } } ////// Changes the Source assocatied with these RoutedEventArgs /// ////// Only used internally. Added to support cracking generic MouseButtonDown/Up events /// into MouseLeft/RightButtonDown/Up events. /// /// /// The new object to associate as the source of these RoutedEventArgs /// internal void OverrideSource( object source ) { _source = source; } ////// Returns OriginalSource object that raised the RoutedEvent /// ////// Always returns the OriginalSource object that raised the /// RoutedEvent unlike public object OriginalSource { get {return _originalSource;} } ////// that may vary under specific scenarios /// This property acquires its value once before the event /// handlers are invoked and never changes then on /// /// Invoked when the source of the event is set /// ////// Changing the source of an event can often /// require updating the data within the event. /// For this reason, the OnSource= method is /// protected virtual and is meant to be /// overridden by sub-classes of /// /// /// The new value that the SourceProperty is being set to /// protected virtual void OnSetSource(object source) { } ////// Also see /// /// Invokes the generic handler with the /// appropriate arguments /// ////// Is meant to be overridden by sub-classes of /// /// /// Generic Handler to be invoked /// /// /// Target on whom the Handler will be invoked /// protected virtual void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (_routedEvent == null) { throw new InvalidOperationException(SR.Get(SRID.RoutedEventArgsMustHaveRoutedEvent)); } InvokingHandler = true; try { if (genericHandler is RoutedEventHandler) { ((RoutedEventHandler)genericHandler)(genericTarget, this); } else { // Restricted Action - reflection permission required genericHandler.DynamicInvoke(new object[] {genericTarget, this}); } } finally { InvokingHandler = false; } } #endregion External API #region Operations // Calls the InvokeEventHandler protected // virtual method // // This method is needed because // delegates are invoked from // RoutedEventHandler which is not a // sub-class of RoutedEventArgs // and hence cannot invoke protected // method RoutedEventArgs.FireEventHandler internal void InvokeHandler(Delegate handler, object target) { InvokingHandler = true; try { InvokeEventHandler(handler, target); } finally { InvokingHandler = false; } } ///to provide /// more efficient invocation of their delegate /// /// Critical - access critical information, if this is a user initiated command /// TreatAsSafe - checking user initiated bit considered safe. /// internal bool UserInitiated { [SecurityCritical, SecurityTreatAsSafe ] [FriendAccessAllowed] // Also used by Framework. get { return _flags [ UserInitiatedIndex ] ; } } ////// Critical - access critical information, if this is a user initiated command /// [SecurityCritical] internal void MarkAsUserInitiated() { _flags [ UserInitiatedIndex ] = true; } ////// Critical - access critical information, if this is a user initiated command /// TreatAsSafe - clearing user initiated bit considered safe. /// [SecurityCritical, SecurityTreatAsSafe ] internal void ClearUserInitiated() { _flags [ UserInitiatedIndex ] = false ; } ////// Critical - _flags is critical due to UserInitiated value. /// TreatAsSafe - in this function we're not setting UserInitiated - we're setting InvokingHandler. /// private bool InvokingHandler { [SecurityCritical, SecurityTreatAsSafe ] get { return _flags[InvokingHandlerIndex]; } [SecurityCritical, SecurityTreatAsSafe ] set { _flags[InvokingHandlerIndex] = value; } } #endregion Operations #region Data private RoutedEvent _routedEvent; private object _source; private object _originalSource; ////// Critical - the UserInitiated flag value is critical. /// [SecurityCritical] private BitVector32 _flags; private const int HandledIndex = 1; private const int UserInitiatedIndex = 2; private const int InvokingHandlerIndex = 4; #endregion Data } ////// RoutedEventHandler Definition /// ///public delegate void RoutedEventHandler(object sender, RoutedEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
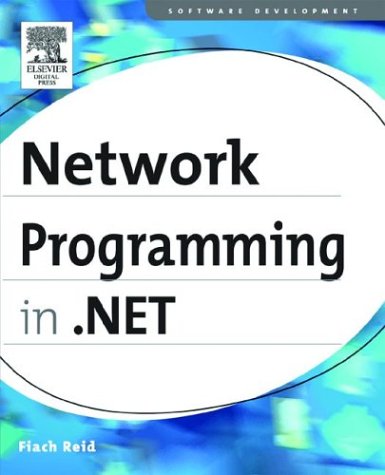
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SingleObjectCollection.cs
- XpsFilter.cs
- WpfWebRequestHelper.cs
- SqlAggregateChecker.cs
- Automation.cs
- CompareValidator.cs
- TypeDescriptor.cs
- ConsoleEntryPoint.cs
- Converter.cs
- ListenerElementsCollection.cs
- DataBindingsDialog.cs
- SoapParser.cs
- MenuAutoFormat.cs
- NameValuePermission.cs
- Helper.cs
- _Events.cs
- SurrogateChar.cs
- StrokeCollection.cs
- ExpandCollapseIsCheckedConverter.cs
- AutoResetEvent.cs
- ClientConfigurationHost.cs
- CompressedStack.cs
- SafeNativeMethods.cs
- ConfigurationStrings.cs
- MobileControlsSectionHandler.cs
- ComPlusServiceLoader.cs
- BooleanConverter.cs
- InternalConfigHost.cs
- InkCollectionBehavior.cs
- GridViewColumnCollection.cs
- CssStyleCollection.cs
- Debugger.cs
- BamlReader.cs
- ConfigurationElementCollection.cs
- Subtree.cs
- PackageRelationshipCollection.cs
- ProcessingInstructionAction.cs
- SqlTriggerContext.cs
- ReferenceEqualityComparer.cs
- AddingNewEventArgs.cs
- SequentialUshortCollection.cs
- ResXFileRef.cs
- CallContext.cs
- SqlConnectionPoolProviderInfo.cs
- DesignerActionPanel.cs
- TextDocumentView.cs
- XpsDocument.cs
- WindowsBrush.cs
- VariableQuery.cs
- ListenerConstants.cs
- HighlightComponent.cs
- HMACSHA256.cs
- WorkflowInstanceTerminatedRecord.cs
- CustomCredentialPolicy.cs
- QueryContinueDragEventArgs.cs
- BinaryFormatter.cs
- _TLSstream.cs
- OptimalTextSource.cs
- ResolvedKeyFrameEntry.cs
- indexingfiltermarshaler.cs
- ConfigXmlText.cs
- TransformerTypeCollection.cs
- SizeChangedEventArgs.cs
- CultureInfo.cs
- TransformCollection.cs
- MappingMetadataHelper.cs
- RunInstallerAttribute.cs
- SqlStream.cs
- ItemPager.cs
- TogglePattern.cs
- ReadOnlyDataSource.cs
- DataControlFieldCollection.cs
- TokenCreationException.cs
- EdmMember.cs
- ObjRef.cs
- BrushValueSerializer.cs
- FixedElement.cs
- ListControlConvertEventArgs.cs
- Propagator.cs
- OneOfElement.cs
- GenericsInstances.cs
- ApplicationInfo.cs
- GcHandle.cs
- RepeatBehavior.cs
- DockingAttribute.cs
- WsiProfilesElement.cs
- PixelFormats.cs
- CodeTypeDeclaration.cs
- MultipleViewProviderWrapper.cs
- DataComponentMethodGenerator.cs
- OpenFileDialog.cs
- TypeConverterHelper.cs
- RedirectionProxy.cs
- GenericWebPart.cs
- WeakReference.cs
- AudioStateChangedEventArgs.cs
- SspiHelper.cs
- TransformPatternIdentifiers.cs
- Light.cs
- XmlException.cs