Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / RepeatBehavior.cs / 1305600 / RepeatBehavior.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehavior.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System.ComponentModel; using System.Diagnostics; using System.Text; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// A RepeatBehavior describes how a Timeline object may repeat its simple duration. /// There are three types of RepeatBehavior behaviors: IterationCount, RepeatDuration, and Forever. /// [TypeConverter(typeof(RepeatBehaviorConverter))] public struct RepeatBehavior : IFormattable { private double _iterationCount; private TimeSpan _repeatDuration; private RepeatBehaviorType _type; #region Constructors ///An IterationCount RepeatBehavior specifies the number of times the simple duration of a Timeline will /// be repeated. An iteration count of 0.5 means the Timeline will only be active for half /// of its simple duration and will only reach 50% progress. An iteration count of 1.0 is the /// default and means a Timeline will be active for exactly one of its simple durations. An /// iteration count of 2.0 means a Timeline will run twice, or repeat its simple duration /// once after its initial simple duration. ///A RepeatDuration RepeatBehavior specifies the amount of time that a Timeline will repeat. /// For instance if a Timeline has a simple Duration value of 1 second and a RepeatBehavior with a /// RepeatDuration value of 2.5 seconds, then it will run for 2.5 iterations. ///A Forever RepeatBehavior specifies that a Timeline will repeat forever. ////// Creates a new RepeatBehavior that represents and iteration count. /// /// The number of iterations specified by this RepeatBehavior. public RepeatBehavior(double count) { if ( Double.IsInfinity(count) || DoubleUtil.IsNaN(count) || count < 0.0) { throw new ArgumentOutOfRangeException("count", SR.Get(SRID.Timing_RepeatBehaviorInvalidIterationCount, count)); } _repeatDuration = new TimeSpan(0); _iterationCount = count; _type = RepeatBehaviorType.IterationCount; } ////// Creates a new RepeatBehavior that represents a repeat duration for which a Timeline will repeat /// its simple duration. /// /// A TimeSpan representing the repeat duration specified by this RepeatBehavior. public RepeatBehavior(TimeSpan duration) { if (duration < new TimeSpan(0)) { throw new ArgumentOutOfRangeException("duration", SR.Get(SRID.Timing_RepeatBehaviorInvalidRepeatDuration, duration)); } _iterationCount = 0.0; _repeatDuration = duration; _type = RepeatBehaviorType.RepeatDuration; } ////// Creates and returns a RepeatBehavior that indicates that a Timeline should repeat its /// simple duration forever. /// ///A RepeatBehavior that indicates that a Timeline should repeat its simple duration /// forever. public static RepeatBehavior Forever { get { RepeatBehavior forever = new RepeatBehavior(); forever._type = RepeatBehaviorType.Forever; return forever; } } #endregion // Constructors #region Properties ////// Indicates whether this RepeatBehavior represents an iteration count. /// ///True if this RepeatBehavior represents an iteration count; otherwise false. public bool HasCount { get { return _type == RepeatBehaviorType.IterationCount; } } ////// Indicates whether this RepeatBehavior represents a repeat duration. /// ///True if this RepeatBehavior represents a repeat duration; otherwise false. public bool HasDuration { get { return _type == RepeatBehaviorType.RepeatDuration; } } ////// Returns the iteration count specified by this RepeatBehavior. /// ///The iteration count specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent an iteration count. public double Count { get { if (_type != RepeatBehaviorType.IterationCount) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotIterationCount, this)); } return _iterationCount; } } ////// Returns the repeat duration specified by this RepeatBehavior. /// ///A TimeSpan representing the repeat duration specified by this RepeatBehavior. ///Thrown if this RepeatBehavior does not represent a repeat duration. public TimeSpan Duration { get { if (_type != RepeatBehaviorType.RepeatDuration) { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_RepeatBehaviorNotRepeatDuration, this)); } return _repeatDuration; } } #endregion // Properties #region Methods ////// Indicates whether the specified Object is equal to this RepeatBehavior. /// /// ///true if value is a RepeatBehavior and is equal to this instance; otherwise false. public override bool Equals(Object value) { if (value is RepeatBehavior) { return this.Equals((RepeatBehavior)value); } else { return false; } } ////// Indicates whether the specified RepeatBehavior is equal to this RepeatBehavior. /// /// A RepeatBehavior to compare with this RepeatBehavior. ///true if repeatBehavior is equal to this instance; otherwise false. public bool Equals(RepeatBehavior repeatBehavior) { if (_type == repeatBehavior._type) { switch (_type) { case RepeatBehaviorType.Forever: return true; case RepeatBehaviorType.IterationCount: return _iterationCount == repeatBehavior._iterationCount; case RepeatBehaviorType.RepeatDuration: return _repeatDuration == repeatBehavior._repeatDuration; default: Debug.Fail("Unhandled RepeatBehaviorType"); return false; } } else { return false; } } ////// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool Equals(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Generates a hash code for this RepeatBehavior. /// ///A hash code for this RepeatBehavior. public override int GetHashCode() { switch (_type) { case RepeatBehaviorType.IterationCount: return _iterationCount.GetHashCode(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.GetHashCode(); case RepeatBehaviorType.Forever: // We try to choose an unlikely hash code value for Forever. // All Forevers need to return the same hash code value. return int.MaxValue - 42; default: Debug.Fail("Unhandled RepeatBehaviorType"); return base.GetHashCode(); } } ////// Creates a string representation of this RepeatBehavior based on the current culture. /// ///A string representation of this RepeatBehavior based on the current culture. public override string ToString() { return InternalToString(null, null); } ////// /// /// ///public string ToString(IFormatProvider formatProvider) { return InternalToString(null, formatProvider); } /// /// /// /// /// ///string IFormattable.ToString(string format, IFormatProvider formatProvider) { return InternalToString(format, formatProvider); } /// /// /// /// /// ///internal string InternalToString(string format, IFormatProvider formatProvider) { switch (_type) { case RepeatBehaviorType.Forever: return "Forever"; case RepeatBehaviorType.IterationCount: StringBuilder sb = new StringBuilder(); sb.AppendFormat( formatProvider, "{0:" + format + "}x", _iterationCount); return sb.ToString(); case RepeatBehaviorType.RepeatDuration: return _repeatDuration.ToString(); default: Debug.Fail("Unhandled RepeatBehaviorType."); return null; } } #endregion // Methods #region Operators /// /// Indicates whether the specified RepeatBehaviors are equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are equal; otherwise false. public static bool operator ==(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return repeatBehavior1.Equals(repeatBehavior2); } ////// Indicates whether the specified RepeatBehaviors are not equal to each other. /// /// /// ///true if repeatBehavior1 and repeatBehavior2 are not equal; otherwise false. public static bool operator !=(RepeatBehavior repeatBehavior1, RepeatBehavior repeatBehavior2) { return !repeatBehavior1.Equals(repeatBehavior2); } #endregion // Operators ////// An enumeration of the different types of RepeatBehavior behaviors. /// private enum RepeatBehaviorType { IterationCount, RepeatDuration, Forever } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
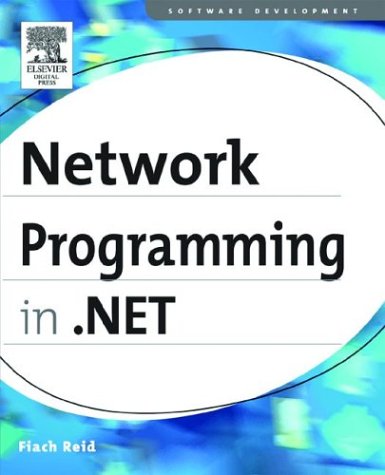
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SequenceDesignerAccessibleObject.cs
- DataPointer.cs
- ConstrainedGroup.cs
- ObfuscationAttribute.cs
- SqlXmlStorage.cs
- ZipIOCentralDirectoryFileHeader.cs
- EditorZoneBase.cs
- ComMethodElementCollection.cs
- HtmlFormWrapper.cs
- ContentControl.cs
- TableParaClient.cs
- Currency.cs
- SemaphoreFullException.cs
- FileClassifier.cs
- WebEventTraceProvider.cs
- SerialStream.cs
- SchemaCollectionPreprocessor.cs
- WebPartManager.cs
- SafeMILHandle.cs
- Point4D.cs
- Aes.cs
- InstanceDescriptor.cs
- UIAgentInitializationException.cs
- DbConnectionPoolOptions.cs
- OperandQuery.cs
- BamlTreeUpdater.cs
- BaseTreeIterator.cs
- JpegBitmapDecoder.cs
- RegexMatch.cs
- KeyValueConfigurationElement.cs
- GeometryCombineModeValidation.cs
- MenuAutomationPeer.cs
- PlainXmlWriter.cs
- ProxyGenerator.cs
- SettingsAttributeDictionary.cs
- SystemIcmpV6Statistics.cs
- ColumnMapCopier.cs
- DependencyObject.cs
- Expressions.cs
- Psha1DerivedKeyGenerator.cs
- IPCCacheManager.cs
- DecoderNLS.cs
- SerializerWriterEventHandlers.cs
- RegistrationServices.cs
- ParallelTimeline.cs
- RenderContext.cs
- VisualStyleElement.cs
- DictionarySectionHandler.cs
- CompositeDispatchFormatter.cs
- Point3DIndependentAnimationStorage.cs
- Underline.cs
- TraceSwitch.cs
- DropShadowEffect.cs
- GrammarBuilderRuleRef.cs
- FormatConvertedBitmap.cs
- UnsafeNativeMethods.cs
- NullableDecimalAverageAggregationOperator.cs
- SqlRowUpdatingEvent.cs
- OdbcConnectionHandle.cs
- ManipulationLogic.cs
- EntityKeyElement.cs
- ZipIOExtraFieldPaddingElement.cs
- _Semaphore.cs
- VariantWrapper.cs
- XmlResolver.cs
- TransformCollection.cs
- XmlDictionaryReaderQuotas.cs
- TextRangeBase.cs
- LayoutManager.cs
- DoubleCollectionConverter.cs
- DataGridViewColumnDesigner.cs
- DispatchWrapper.cs
- ControlUtil.cs
- PrefixQName.cs
- DocumentCollection.cs
- RuntimeTrackingProfile.cs
- LightweightCodeGenerator.cs
- CodeDOMUtility.cs
- EdmProviderManifest.cs
- ObjectDataSourceFilteringEventArgs.cs
- SerializationInfoEnumerator.cs
- ApplicationSettingsBase.cs
- GlyphCache.cs
- IntegerCollectionEditor.cs
- InternalException.cs
- Matrix.cs
- FramingFormat.cs
- GridSplitterAutomationPeer.cs
- _FtpControlStream.cs
- BitmapEffect.cs
- ObservableCollectionDefaultValueFactory.cs
- DataQuery.cs
- EntitySet.cs
- UidManager.cs
- ServicePoint.cs
- FontCollection.cs
- Oid.cs
- BuildProviderCollection.cs
- DataGridViewRowsAddedEventArgs.cs
- SafeNativeMethods.cs