Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / ObservableCollectionDefaultValueFactory.cs / 1305600 / ObservableCollectionDefaultValueFactory.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultvalueFactory for ObservableCollection // // History: // 2009/24/07 : [....] - Created. // //--------------------------------------------------------------------------- using MS.Internal.WindowsBase; using System; using System.Diagnostics; using System.Windows; using System.Collections.ObjectModel; // ObservableCollection using System.Collections.Specialized; // NotifyCollectionChangedEventHandler namespace MS.Internal { //// ObservableCollectionDefaultValueFactory is a DefaultValueFactory implementation which will // promote the default value to a local value if the collection is modified. // [FriendAccessAllowed] // built into Base, used by Framework internal class ObservableCollectionDefaultValueFactory: DefaultValueFactory { internal ObservableCollectionDefaultValueFactory() { _default = new ObservableCollection (); } /// /// This is used for Sealed objects. ObservableCollections are inherently mutable, so they shouldn't /// be used with sealed objects. The PropertyDescriptor calls this, so we'll just return the same empty collection. /// internal override object DefaultValue { get { return _default; } } ////// internal override object CreateDefaultValue(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "It is the caller responsibility to ensure that owner and property are non-null."); var result = new ObservableCollection(); // Wire up a ObservableCollectionDefaultPromoter to observe the default value we // just created and automatically promote it to local if it is modified. // NOTE: We do not holding a reference to this because it should have the same lifetime as // the collection. It will not be immediately GC'ed because it hooks the collections change event. new ObservableCollectionDefaultPromoter(owner, property, result); return result; } /// /// The ObservableCollectionDefaultPromoter observes the mutable defaults we hand out /// for changed events. If the default is ever modified this class will /// promote it to a local value by writing it to the local store and /// clear the cached default value so we will generate a new default /// the next time the property system is asked for one. /// private class ObservableCollectionDefaultPromoter { internal ObservableCollectionDefaultPromoter(DependencyObject owner, DependencyProperty property, ObservableCollectioncollection) { Debug.Assert(owner != null && property != null, "Caller is responsible for ensuring that owner and property are non-null."); Debug.Assert(property.GetMetadata(owner.DependencyObjectType).UsingDefaultValueFactory, "How did we end up observing a mutable if we were not registered for the factory pattern?"); // We hang on to the property and owner so we can write the default // value back to the local store if it changes. See also // OnDefaultValueChanged. _owner = owner; _property = property; _collection = collection; _collection.CollectionChanged += OnDefaultValueChanged; } internal void OnDefaultValueChanged(object sender, NotifyCollectionChangedEventArgs e) { PropertyMetadata metadata = _property.GetMetadata(_owner.DependencyObjectType); // Remove this value from the DefaultValue cache so we stop // handing it out as the default value now that it has changed. metadata.ClearCachedDefaultValue(_owner, _property); // If someone else hasn't already written a local value, // promote the default value to local. if (_owner.ReadLocalValue(_property) == DependencyProperty.UnsetValue) { // Read-only properties must be set using the Key if (_property.ReadOnly) { _owner.SetValue(_property.DependencyPropertyKey, _collection); } else { _owner.SetValue(_property, _collection); } } // Unhook the change handler because we're finsihed promoting _collection.CollectionChanged -= OnDefaultValueChanged; } private readonly DependencyObject _owner; private readonly DependencyProperty _property; private readonly ObservableCollection _collection; } private ObservableCollection _default; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultvalueFactory for ObservableCollection // // History: // 2009/24/07 : [....] - Created. // //--------------------------------------------------------------------------- using MS.Internal.WindowsBase; using System; using System.Diagnostics; using System.Windows; using System.Collections.ObjectModel; // ObservableCollection using System.Collections.Specialized; // NotifyCollectionChangedEventHandler namespace MS.Internal { //// ObservableCollectionDefaultValueFactory is a DefaultValueFactory implementation which will // promote the default value to a local value if the collection is modified. // [FriendAccessAllowed] // built into Base, used by Framework internal class ObservableCollectionDefaultValueFactory: DefaultValueFactory { internal ObservableCollectionDefaultValueFactory() { _default = new ObservableCollection (); } /// /// This is used for Sealed objects. ObservableCollections are inherently mutable, so they shouldn't /// be used with sealed objects. The PropertyDescriptor calls this, so we'll just return the same empty collection. /// internal override object DefaultValue { get { return _default; } } ////// internal override object CreateDefaultValue(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "It is the caller responsibility to ensure that owner and property are non-null."); var result = new ObservableCollection(); // Wire up a ObservableCollectionDefaultPromoter to observe the default value we // just created and automatically promote it to local if it is modified. // NOTE: We do not holding a reference to this because it should have the same lifetime as // the collection. It will not be immediately GC'ed because it hooks the collections change event. new ObservableCollectionDefaultPromoter(owner, property, result); return result; } /// /// The ObservableCollectionDefaultPromoter observes the mutable defaults we hand out /// for changed events. If the default is ever modified this class will /// promote it to a local value by writing it to the local store and /// clear the cached default value so we will generate a new default /// the next time the property system is asked for one. /// private class ObservableCollectionDefaultPromoter { internal ObservableCollectionDefaultPromoter(DependencyObject owner, DependencyProperty property, ObservableCollectioncollection) { Debug.Assert(owner != null && property != null, "Caller is responsible for ensuring that owner and property are non-null."); Debug.Assert(property.GetMetadata(owner.DependencyObjectType).UsingDefaultValueFactory, "How did we end up observing a mutable if we were not registered for the factory pattern?"); // We hang on to the property and owner so we can write the default // value back to the local store if it changes. See also // OnDefaultValueChanged. _owner = owner; _property = property; _collection = collection; _collection.CollectionChanged += OnDefaultValueChanged; } internal void OnDefaultValueChanged(object sender, NotifyCollectionChangedEventArgs e) { PropertyMetadata metadata = _property.GetMetadata(_owner.DependencyObjectType); // Remove this value from the DefaultValue cache so we stop // handing it out as the default value now that it has changed. metadata.ClearCachedDefaultValue(_owner, _property); // If someone else hasn't already written a local value, // promote the default value to local. if (_owner.ReadLocalValue(_property) == DependencyProperty.UnsetValue) { // Read-only properties must be set using the Key if (_property.ReadOnly) { _owner.SetValue(_property.DependencyPropertyKey, _collection); } else { _owner.SetValue(_property, _collection); } } // Unhook the change handler because we're finsihed promoting _collection.CollectionChanged -= OnDefaultValueChanged; } private readonly DependencyObject _owner; private readonly DependencyProperty _property; private readonly ObservableCollection _collection; } private ObservableCollection _default; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
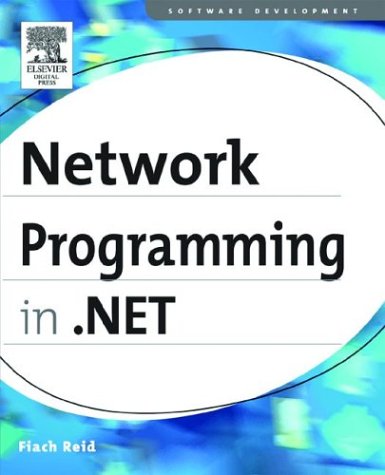
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MaterialCollection.cs
- SiteMapDataSourceView.cs
- FixedSOMPageElement.cs
- TransactionException.cs
- TypeValidationEventArgs.cs
- XmlRootAttribute.cs
- _NegotiateClient.cs
- HwndSourceKeyboardInputSite.cs
- SpinWait.cs
- GridViewCommandEventArgs.cs
- DateTimeConverter.cs
- CodeSubDirectoriesCollection.cs
- ColumnHeader.cs
- PropertyNames.cs
- WebBrowserDesigner.cs
- SiteMapPath.cs
- ServiceModelActivationSectionGroup.cs
- LockCookie.cs
- PathGeometry.cs
- OleDbParameterCollection.cs
- UnknownWrapper.cs
- StrongNameSignatureInformation.cs
- DiagnosticsConfigurationHandler.cs
- IgnoreFlushAndCloseStream.cs
- ActiveXSerializer.cs
- DesignerSerializerAttribute.cs
- Activity.cs
- DeviceContexts.cs
- XmlSubtreeReader.cs
- ToolStripPanel.cs
- NavigationEventArgs.cs
- CssTextWriter.cs
- FieldTemplateFactory.cs
- XmlDictionary.cs
- HtmlContainerControl.cs
- ViewLoader.cs
- SystemUdpStatistics.cs
- HandleCollector.cs
- SoapFault.cs
- DecimalAverageAggregationOperator.cs
- SkipStoryboardToFill.cs
- ProgramNode.cs
- ListDictionary.cs
- PixelShader.cs
- MultiPartWriter.cs
- ParserOptions.cs
- BitmapFrameEncode.cs
- SoapConverter.cs
- CharEnumerator.cs
- InstanceDataCollection.cs
- NumberFunctions.cs
- TreeViewCancelEvent.cs
- TypeExtensionSerializer.cs
- ParallelTimeline.cs
- EdmTypeAttribute.cs
- LightweightEntityWrapper.cs
- ProtocolsConfigurationEntry.cs
- Int16.cs
- EdmComplexPropertyAttribute.cs
- OdbcRowUpdatingEvent.cs
- CqlLexer.cs
- ItemsChangedEventArgs.cs
- GridViewColumn.cs
- CachedPathData.cs
- ShapingWorkspace.cs
- OneOf.cs
- MaxSessionCountExceededException.cs
- SqlGenericUtil.cs
- TreeWalker.cs
- UrlMappingsSection.cs
- InstalledFontCollection.cs
- UdpDiscoveryEndpoint.cs
- EntityParameter.cs
- ObjectParameterCollection.cs
- KeyValueInternalCollection.cs
- BuildDependencySet.cs
- ClientSideQueueItem.cs
- ExtensibleClassFactory.cs
- RouteItem.cs
- WeakEventTable.cs
- _HTTPDateParse.cs
- MouseActionConverter.cs
- PlaceHolder.cs
- EventBuilder.cs
- ProvidersHelper.cs
- cache.cs
- PropertyFilter.cs
- ToolStripContextMenu.cs
- CacheOutputQuery.cs
- TextAction.cs
- DesignerSerializationManager.cs
- PositiveTimeSpanValidator.cs
- ListViewItem.cs
- VScrollProperties.cs
- controlskin.cs
- RsaKeyGen.cs
- Decimal.cs
- HistoryEventArgs.cs
- TypeHelpers.cs
- XmlSecureResolver.cs