Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlContainerControl.cs / 1 / HtmlContainerControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.HtmlControls { using System.Runtime.Serialization.Formatters; using System; using System.Collections; using System.ComponentModel; using System.IO; using System.Web.UI; using System.Security.Permissions; /* * A control representing an intrinsic Html tag. */ ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] abstract public class HtmlContainerControl : HtmlControl { /* * Creates a new WebControl */ ///The ////// class defines the methods, /// properties and events /// available to all Html Server controls that must have a /// closing tag. /// protected HtmlContainerControl() : this("span") { } /* * Creates a new HtmlContainerControl */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlContainerControl(string tag) : base(tag) { } /* * The inner html content between the begin and end tag. * A set will replace any existing child controls with a single literal. * A get will return the text of a single literal child OR * will throw an exception if there are no children, more than one * child, or the single child is not a literal. */ ///Initializes a new instance of a ///class using the /// specified string. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), HtmlControlPersistable(false), ] public virtual string InnerHtml { get { if (IsLiteralContent()) return((LiteralControl) Controls[0]).Text; else if (HasControls() && (Controls.Count == 1) && Controls[0] is DataBoundLiteralControl) return ((DataBoundLiteralControl) Controls[0]).Text; else { if (Controls.Count == 0) return String.Empty; throw new HttpException(SR.GetString(SR.Inner_Content_not_literal, ID)); } } set { Controls.Clear(); Controls.Add(new LiteralControl(value)); ViewState["innerhtml"] = value; } } /* * The inner text content between the begin and end tag. * A set will replace any existing child controls with a single literal. * A get will return the text of a single literal child OR * will throw an exception if there are no children, more than one child, or * the single child is not a literal. */ ////// Gets or sets the /// content found between the opening and closing tags of the specified HTML server control. /// ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), HtmlControlPersistable(false), ] public virtual string InnerText { get { return HttpUtility.HtmlDecode(InnerHtml); } set { InnerHtml = HttpUtility.HtmlEncode(value); } } ////// Gets or sets all text between the opening and closing tags /// of the specified HTML server control. /// ////// protected override ControlCollection CreateControlCollection() { return new ControlCollection(this); } ///[To be supplied.] ////// /// protected override void LoadViewState(object savedState) { if (savedState != null) { base.LoadViewState(savedState); string s = (string)ViewState["innerhtml"]; if (s != null) InnerHtml = s; } } /* * Render the control into the given writer. */ ////// /// protected internal override void Render(HtmlTextWriter writer) { RenderBeginTag(writer); RenderChildren(writer); RenderEndTag(writer); } /* * Override to prevent InnerHtml from being rendered as an attribute. */ ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { ViewState.Remove("innerhtml"); base.RenderAttributes(writer); } /* * Render the end tag, </TAGNAME>. */ ////// /// protected virtual void RenderEndTag(HtmlTextWriter writer) { writer.WriteEndTag(TagName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.HtmlControls { using System.Runtime.Serialization.Formatters; using System; using System.Collections; using System.ComponentModel; using System.IO; using System.Web.UI; using System.Security.Permissions; /* * A control representing an intrinsic Html tag. */ ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] abstract public class HtmlContainerControl : HtmlControl { /* * Creates a new WebControl */ ///The ////// class defines the methods, /// properties and events /// available to all Html Server controls that must have a /// closing tag. /// protected HtmlContainerControl() : this("span") { } /* * Creates a new HtmlContainerControl */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlContainerControl(string tag) : base(tag) { } /* * The inner html content between the begin and end tag. * A set will replace any existing child controls with a single literal. * A get will return the text of a single literal child OR * will throw an exception if there are no children, more than one * child, or the single child is not a literal. */ ///Initializes a new instance of a ///class using the /// specified string. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), HtmlControlPersistable(false), ] public virtual string InnerHtml { get { if (IsLiteralContent()) return((LiteralControl) Controls[0]).Text; else if (HasControls() && (Controls.Count == 1) && Controls[0] is DataBoundLiteralControl) return ((DataBoundLiteralControl) Controls[0]).Text; else { if (Controls.Count == 0) return String.Empty; throw new HttpException(SR.GetString(SR.Inner_Content_not_literal, ID)); } } set { Controls.Clear(); Controls.Add(new LiteralControl(value)); ViewState["innerhtml"] = value; } } /* * The inner text content between the begin and end tag. * A set will replace any existing child controls with a single literal. * A get will return the text of a single literal child OR * will throw an exception if there are no children, more than one child, or * the single child is not a literal. */ ////// Gets or sets the /// content found between the opening and closing tags of the specified HTML server control. /// ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), HtmlControlPersistable(false), ] public virtual string InnerText { get { return HttpUtility.HtmlDecode(InnerHtml); } set { InnerHtml = HttpUtility.HtmlEncode(value); } } ////// Gets or sets all text between the opening and closing tags /// of the specified HTML server control. /// ////// protected override ControlCollection CreateControlCollection() { return new ControlCollection(this); } ///[To be supplied.] ////// /// protected override void LoadViewState(object savedState) { if (savedState != null) { base.LoadViewState(savedState); string s = (string)ViewState["innerhtml"]; if (s != null) InnerHtml = s; } } /* * Render the control into the given writer. */ ////// /// protected internal override void Render(HtmlTextWriter writer) { RenderBeginTag(writer); RenderChildren(writer); RenderEndTag(writer); } /* * Override to prevent InnerHtml from being rendered as an attribute. */ ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { ViewState.Remove("innerhtml"); base.RenderAttributes(writer); } /* * Render the end tag, </TAGNAME>. */ ////// /// protected virtual void RenderEndTag(HtmlTextWriter writer) { writer.WriteEndTag(TagName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
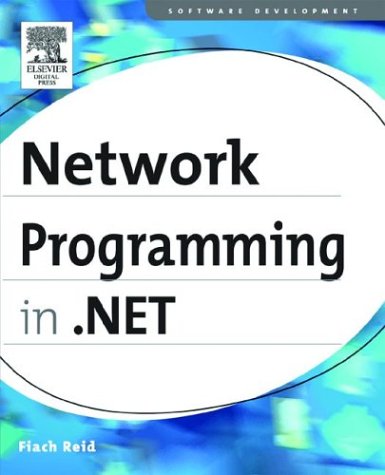
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyCollection.cs
- QilName.cs
- SoapElementAttribute.cs
- URLString.cs
- WorkItem.cs
- ResourceContainer.cs
- SafeSystemMetrics.cs
- RecognizedAudio.cs
- MethodBody.cs
- IsolatedStorage.cs
- XmlBoundElement.cs
- ButtonBase.cs
- WebResponse.cs
- RSAPKCS1SignatureFormatter.cs
- ExpressionBuilder.cs
- PropertyGeneratedEventArgs.cs
- UidManager.cs
- DesignObjectWrapper.cs
- DataGridViewBindingCompleteEventArgs.cs
- NavigationProgressEventArgs.cs
- HTMLTagNameToTypeMapper.cs
- HandleCollector.cs
- PageThemeBuildProvider.cs
- Tile.cs
- ImageDrawing.cs
- CodeEventReferenceExpression.cs
- RSACryptoServiceProvider.cs
- TypeToken.cs
- ScrollPattern.cs
- DataBindingValueUIHandler.cs
- CustomTypeDescriptor.cs
- Track.cs
- StateElement.cs
- DataRelationPropertyDescriptor.cs
- Font.cs
- RuntimeCompatibilityAttribute.cs
- SafeRightsManagementQueryHandle.cs
- IgnoreDeviceFilterElementCollection.cs
- PersonalizableTypeEntry.cs
- BaseConfigurationRecord.cs
- XmlChoiceIdentifierAttribute.cs
- BasePattern.cs
- ControlBuilder.cs
- CharacterString.cs
- datacache.cs
- BackStopAuthenticationModule.cs
- SamlAuthorityBinding.cs
- TransformValueSerializer.cs
- EngineSiteSapi.cs
- DispatcherProcessingDisabled.cs
- SQLRoleProvider.cs
- FileCodeGroup.cs
- NativeCppClassAttribute.cs
- StringConverter.cs
- SQLString.cs
- DataGridRelationshipRow.cs
- RSAProtectedConfigurationProvider.cs
- ComplexTypeEmitter.cs
- RightsManagementEncryptionTransform.cs
- FlowNode.cs
- BuildProviderAppliesToAttribute.cs
- RtfControls.cs
- Journal.cs
- EnumMember.cs
- StrongNameUtility.cs
- CompilerInfo.cs
- Parser.cs
- Substitution.cs
- GuidelineSet.cs
- Path.cs
- EventLogPermission.cs
- Util.cs
- Mapping.cs
- PathFigureCollectionValueSerializer.cs
- FirstMatchCodeGroup.cs
- WebPartUserCapability.cs
- SmiEventStream.cs
- PauseStoryboard.cs
- LinqDataSourceView.cs
- TextDpi.cs
- UpnEndpointIdentity.cs
- PrintPageEvent.cs
- ContentValidator.cs
- BitmapMetadataBlob.cs
- FixedLineResult.cs
- SubqueryTrackingVisitor.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- FacetDescription.cs
- TextDocumentView.cs
- TableProviderWrapper.cs
- WebReferencesBuildProvider.cs
- TypeUtils.cs
- XsdBuildProvider.cs
- lengthconverter.cs
- Resources.Designer.cs
- ContractValidationHelper.cs
- ParallelQuery.cs
- File.cs
- ControlParameter.cs
- AssemblySettingAttributes.cs