Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / WebResponse.cs / 1305376 / WebResponse.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Runtime.Serialization; using System.Security.Permissions; /*++ WebResponse - The abstract base class for all WebResponse objects. --*/ ////// [Serializable] public abstract class WebResponse : MarshalByRefObject, ISerializable, IDisposable { private bool m_IsCacheFresh; private bool m_IsFromCache; ////// A /// response from a Uniform Resource Indentifier (Uri). This is an abstract class. /// ////// protected WebResponse() { } // // ISerializable constructor // ///Initializes a new /// instance of the ////// class. /// protected WebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext) { } // // ISerializable method // ///[To be supplied.] ///[SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Justification = "System.dll is still using pre-v4 security model and needs this demand")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide a way for derived classes to access this method even if they reimplement ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected virtual void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { } /*++ Close - Closes the Response after the use. This causes the read stream to be closed. --*/ public virtual void Close() { throw ExceptionHelper.MethodNotImplementedException; } /// void IDisposable.Dispose() { try { Close(); OnDispose(); } catch { } } internal virtual void OnDispose(){ } public virtual bool IsFromCache { get {return m_IsFromCache;} } internal bool InternalSetFromCache { set { m_IsFromCache = value; } } internal virtual bool IsCacheFresh { get {return m_IsCacheFresh;} } internal bool InternalSetIsCacheFresh { set { m_IsCacheFresh = value; } } public virtual bool IsMutuallyAuthenticated { get {return false;} } /*++ ContentLength - Content length of response. This property returns the content length of the response. --*/ /// /// public virtual long ContentLength { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ContentType - Content type of response. This property returns the content type of the response. --*/ ///When overridden in a derived class, gets or /// sets /// the content length of data being received. ////// public virtual string ContentType { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ResponseStream - Get the response stream for this response. This property returns the response stream for this WebResponse. Input: Nothing. Returns: Response stream for response. read-only --*/ ///When overridden in a derived class, /// gets /// or sets the content type of the data being received. ////// public virtual Stream GetResponseStream() { throw ExceptionHelper.MethodNotImplementedException; } /*++ ResponseUri - Gets the final Response Uri, that includes any changes that may have transpired from the orginal request This property returns Uri for this WebResponse. Input: Nothing. Returns: Response Uri for response. read-only --*/ ///When overridden in a derived class, returns the ///object used /// for reading data from the resource referenced in the /// object. /// public virtual Uri ResponseUri { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ Headers - Gets any request specific headers associated with this request, this is simply a name/value pair collection Input: Nothing. Returns: This property returns WebHeaderCollection. read-only --*/ ///When overridden in a derived class, gets the Uri that /// actually responded to the request. ////// public virtual WebHeaderCollection Headers { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } }; // class WebResponse } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //When overridden in a derived class, gets /// a collection of header name-value pairs associated with this /// request. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Runtime.Serialization; using System.Security.Permissions; /*++ WebResponse - The abstract base class for all WebResponse objects. --*/ ////// [Serializable] public abstract class WebResponse : MarshalByRefObject, ISerializable, IDisposable { private bool m_IsCacheFresh; private bool m_IsFromCache; ////// A /// response from a Uniform Resource Indentifier (Uri). This is an abstract class. /// ////// protected WebResponse() { } // // ISerializable constructor // ///Initializes a new /// instance of the ////// class. /// protected WebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext) { } // // ISerializable method // ///[To be supplied.] ///[SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Justification = "System.dll is still using pre-v4 security model and needs this demand")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide a way for derived classes to access this method even if they reimplement ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected virtual void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { } /*++ Close - Closes the Response after the use. This causes the read stream to be closed. --*/ public virtual void Close() { throw ExceptionHelper.MethodNotImplementedException; } /// void IDisposable.Dispose() { try { Close(); OnDispose(); } catch { } } internal virtual void OnDispose(){ } public virtual bool IsFromCache { get {return m_IsFromCache;} } internal bool InternalSetFromCache { set { m_IsFromCache = value; } } internal virtual bool IsCacheFresh { get {return m_IsCacheFresh;} } internal bool InternalSetIsCacheFresh { set { m_IsCacheFresh = value; } } public virtual bool IsMutuallyAuthenticated { get {return false;} } /*++ ContentLength - Content length of response. This property returns the content length of the response. --*/ /// /// public virtual long ContentLength { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ContentType - Content type of response. This property returns the content type of the response. --*/ ///When overridden in a derived class, gets or /// sets /// the content length of data being received. ////// public virtual string ContentType { get { throw ExceptionHelper.PropertyNotImplementedException; } set { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ ResponseStream - Get the response stream for this response. This property returns the response stream for this WebResponse. Input: Nothing. Returns: Response stream for response. read-only --*/ ///When overridden in a derived class, /// gets /// or sets the content type of the data being received. ////// public virtual Stream GetResponseStream() { throw ExceptionHelper.MethodNotImplementedException; } /*++ ResponseUri - Gets the final Response Uri, that includes any changes that may have transpired from the orginal request This property returns Uri for this WebResponse. Input: Nothing. Returns: Response Uri for response. read-only --*/ ///When overridden in a derived class, returns the ///object used /// for reading data from the resource referenced in the /// object. /// public virtual Uri ResponseUri { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } /*++ Headers - Gets any request specific headers associated with this request, this is simply a name/value pair collection Input: Nothing. Returns: This property returns WebHeaderCollection. read-only --*/ ///When overridden in a derived class, gets the Uri that /// actually responded to the request. ////// public virtual WebHeaderCollection Headers { // read-only get { throw ExceptionHelper.PropertyNotImplementedException; } } }; // class WebResponse } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007.When overridden in a derived class, gets /// a collection of header name-value pairs associated with this /// request. ///
Link Menu
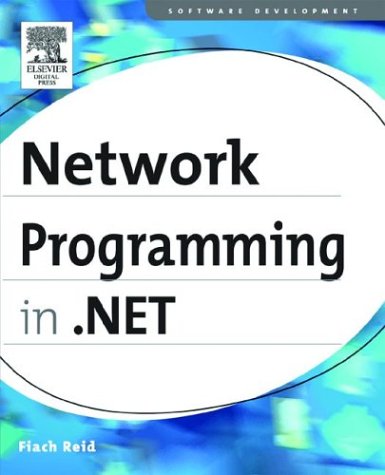
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RSAOAEPKeyExchangeDeformatter.cs
- TransportConfigurationTypeElementCollection.cs
- HtmlInputReset.cs
- GridViewColumnCollection.cs
- DictionaryChange.cs
- FontUnitConverter.cs
- TrackingMemoryStream.cs
- SystemIPv6InterfaceProperties.cs
- UnlockCardRequest.cs
- ImageListUtils.cs
- ApplicationException.cs
- ConstNode.cs
- SingleStorage.cs
- TextEffectResolver.cs
- InternalPolicyElement.cs
- ProxyAssemblyNotLoadedException.cs
- EditorZone.cs
- BaseCAMarshaler.cs
- BaseCodeDomTreeGenerator.cs
- RsaSecurityTokenAuthenticator.cs
- BindingManagerDataErrorEventArgs.cs
- AbstractDataSvcMapFileLoader.cs
- EntityDataSourceDataSelection.cs
- EdmTypeAttribute.cs
- RTLAwareMessageBox.cs
- RecipientInfo.cs
- ProviderUtil.cs
- XmlChildEnumerator.cs
- ServiceDeploymentInfo.cs
- DataFormats.cs
- MobileErrorInfo.cs
- XComponentModel.cs
- NGCPageContentCollectionSerializerAsync.cs
- CalendarDay.cs
- EncoderExceptionFallback.cs
- XsltQilFactory.cs
- PropertyBuilder.cs
- UIElement3D.cs
- ContextMarshalException.cs
- TypeListConverter.cs
- Span.cs
- RawMouseInputReport.cs
- SiteMapPath.cs
- Exceptions.cs
- OLEDB_Enum.cs
- OleDbParameterCollection.cs
- StylusCollection.cs
- OleDragDropHandler.cs
- ModuleBuilder.cs
- WebPartMinimizeVerb.cs
- SettingsPropertyWrongTypeException.cs
- Pair.cs
- RemoveStoryboard.cs
- FormatterServices.cs
- TemplateXamlParser.cs
- NotifyParentPropertyAttribute.cs
- XPathScanner.cs
- SqlHelper.cs
- DefaultBindingPropertyAttribute.cs
- Membership.cs
- Rule.cs
- Pts.cs
- ContravarianceAdapter.cs
- WorkingDirectoryEditor.cs
- UserControl.cs
- JsonQNameDataContract.cs
- AnnotationElement.cs
- coordinatorfactory.cs
- PrintDocument.cs
- URLIdentityPermission.cs
- DynamicPropertyHolder.cs
- FamilyMapCollection.cs
- DataBindingHandlerAttribute.cs
- ConvertEvent.cs
- CompressedStack.cs
- XmlNodeList.cs
- CannotUnloadAppDomainException.cs
- RadioButtonBaseAdapter.cs
- Query.cs
- RoleService.cs
- SmtpClient.cs
- WorkflowElementDialog.cs
- XmlRawWriter.cs
- QueryInterceptorAttribute.cs
- DbInsertCommandTree.cs
- SecurityBindingElement.cs
- GatewayIPAddressInformationCollection.cs
- TransformPatternIdentifiers.cs
- MaskedTextProvider.cs
- ImageList.cs
- Permission.cs
- SplineKeyFrames.cs
- MetadataWorkspace.cs
- MediaTimeline.cs
- SectionInput.cs
- XslCompiledTransform.cs
- CompModSwitches.cs
- LogLogRecordEnumerator.cs
- ViewBox.cs
- XPathDocument.cs