Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / FontUnitConverter.cs / 1 / FontUnitConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class FontUnitConverter : TypeConverter { private StandardValuesCollection values; ///Converts a ///to and from a specified data type. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } ///Determines if the specified data type can be converted to a ///. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; string stringValue = value as string; if (stringValue != null) { string textValue = stringValue.Trim(); if (textValue.Length == 0) { return FontUnit.Empty; } return FontUnit.Parse(textValue, culture); } else { return base.ConvertFrom(context, culture, value); } } ///Converts the specified ///into a . /// /// Returns a value indicating whether the converter can /// convert to the specified destination type. /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ((destinationType == typeof(string)) || (destinationType == typeof(InstanceDescriptor))) { return true; } else { return base.CanConvertTo(context, destinationType); } } ////// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string)) { if ((value == null) || (((FontUnit)value).Type == FontSize.NotSet)) return String.Empty; else return ((FontUnit)value).ToString(culture); } else if ((destinationType == typeof(InstanceDescriptor)) && (value != null)) { FontUnit u = (FontUnit)value; MemberInfo member = null; object[] args = null; if (u.IsEmpty) { member = typeof(FontUnit).GetField("Empty"); } else if (u.Type != FontSize.AsUnit) { string fieldName = null; switch (u.Type) { case FontSize.Smaller: fieldName = "Smaller"; break; case FontSize.Larger: fieldName = "Larger"; break; case FontSize.XXSmall: fieldName = "XXSmall"; break; case FontSize.XSmall: fieldName = "XSmall"; break; case FontSize.Small: fieldName = "Small"; break; case FontSize.Medium: fieldName = "Medium"; break; case FontSize.Large: fieldName = "Large"; break; case FontSize.XLarge: fieldName = "XLarge"; break; case FontSize.XXLarge: fieldName = "XXLarge"; break; } Debug.Assert(fieldName != null, "Invalid FontSize type"); if (fieldName != null) { member = typeof(FontUnit).GetField(fieldName); } } else { member = typeof(FontUnit).GetConstructor(new Type[] { typeof(Unit) }); args = new object[] { u.Unit }; } Debug.Assert(member != null, "Looks like we're missing FontUnit static fields or FontUnit::ctor(Unit)"); if (member != null) { return new InstanceDescriptor(member, args); } else { return null; } } else { return base.ConvertTo(context, culture, value, destinationType); } } ///Converts the specified ///into the specified . /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { object[] namedUnits = new object[] { FontUnit.Smaller, FontUnit.Larger, FontUnit.XXSmall, FontUnit.XSmall, FontUnit.Small, FontUnit.Medium, FontUnit.Large, FontUnit.XLarge, FontUnit.XXLarge }; values = new StandardValuesCollection(namedUnits); } return values; } ///Returns a ////// containing standard values. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ///Indicates whether the specified context contains exclusive standard /// values. ////// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } }Indicates whether the specified context contains suppurted standard /// values. ///
Link Menu
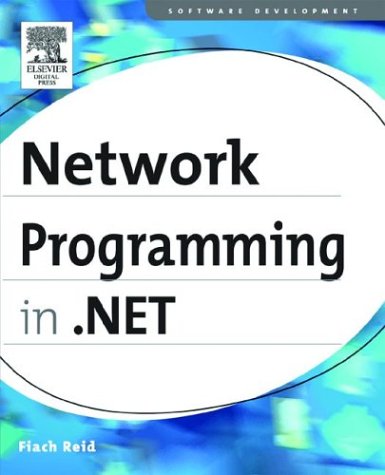
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpModuleAction.cs
- SoapConverter.cs
- PageCodeDomTreeGenerator.cs
- StrokeCollection.cs
- PropertyAccessVisitor.cs
- DeviceContexts.cs
- LinkUtilities.cs
- EdmScalarPropertyAttribute.cs
- FrameworkContentElement.cs
- DataGridViewTopLeftHeaderCell.cs
- DataSourceView.cs
- Delegate.cs
- DataGridViewRowHeaderCell.cs
- OperationAbortedException.cs
- PermissionSetEnumerator.cs
- RotateTransform3D.cs
- ProviderIncompatibleException.cs
- DebugManager.cs
- CursorInteropHelper.cs
- Mappings.cs
- XmlEnumAttribute.cs
- unitconverter.cs
- SqlMethods.cs
- SinglePhaseEnlistment.cs
- QueryContinueDragEventArgs.cs
- WindowPatternIdentifiers.cs
- SystemSounds.cs
- DataGridCell.cs
- SqlDataSourceQueryEditorForm.cs
- GridSplitterAutomationPeer.cs
- LazyTextWriterCreator.cs
- ExternalCalls.cs
- Win32Exception.cs
- ProjectedSlot.cs
- UIElementIsland.cs
- FlowDocumentScrollViewer.cs
- Graph.cs
- _LazyAsyncResult.cs
- InputLanguageEventArgs.cs
- AspNetSynchronizationContext.cs
- InternalBase.cs
- DeviceContexts.cs
- SafeTimerHandle.cs
- NamespaceList.cs
- DragDeltaEventArgs.cs
- PropertySourceInfo.cs
- TimeStampChecker.cs
- Stylesheet.cs
- RelationHandler.cs
- SystemWebSectionGroup.cs
- OleDbParameter.cs
- GlyphsSerializer.cs
- CodeDOMProvider.cs
- FormatConvertedBitmap.cs
- TraceListener.cs
- PartitionResolver.cs
- XmlSerializerNamespaces.cs
- AuthStoreRoleProvider.cs
- Light.cs
- Animatable.cs
- PropertyItemInternal.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- XamlFigureLengthSerializer.cs
- AndCondition.cs
- CodeNamespaceImportCollection.cs
- BypassElement.cs
- ExpressionBuilder.cs
- DocumentPageView.cs
- NoResizeSelectionBorderGlyph.cs
- HttpSessionStateWrapper.cs
- basevalidator.cs
- DiscriminatorMap.cs
- VBIdentifierNameEditor.cs
- ConfigXmlCDataSection.cs
- datacache.cs
- ToolStripContainer.cs
- MailHeaderInfo.cs
- GeneralTransform3DTo2D.cs
- ProxyHwnd.cs
- AttachedPropertyBrowsableAttribute.cs
- CodeTypeReferenceExpression.cs
- DataSysAttribute.cs
- OdbcTransaction.cs
- SQLInt32.cs
- TempEnvironment.cs
- BlockUIContainer.cs
- WindowsToolbarAsMenu.cs
- StrokeNodeEnumerator.cs
- PenLineJoinValidation.cs
- ScriptBehaviorDescriptor.cs
- Ipv6Element.cs
- ToolboxItemCollection.cs
- ValidationManager.cs
- TitleStyle.cs
- VirtualPathProvider.cs
- FtpWebResponse.cs
- SiteMap.cs
- RenamedEventArgs.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- EventLogWatcher.cs