Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Sys / System / Configuration / DictionarySectionHandler.cs / 1 / DictionarySectionHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; ////// Simple dictionary config factory /// config is a dictionary mapping key->value /// /// <add key="name" value="text"> sets key=text /// <remove key="name"> removes the definition of key /// <clear> removes all definitions /// /// public class DictionarySectionHandler : IConfigurationSectionHandler { ////// Given a partially composed config object (possibly null) /// and some input from the config system, return a /// further partially composed config object /// public virtual object Create(Object parent, Object context, XmlNode section) { Hashtable res; // start res off as a shallow clone of the parent if (parent == null) res = new Hashtable(StringComparer.OrdinalIgnoreCase); else res = (Hashtable)((Hashtable)parent).Clone(); // process XML HandlerBase.CheckForUnrecognizedAttributes(section); foreach (XmlNode child in section.ChildNodes) { // skip whitespace and comments, throws if non-element if (HandlerBase.IsIgnorableAlsoCheckForNonElement(child)) continue; // handle, , tags if (child.Name == "add") { HandlerBase.CheckForChildNodes(child); String key = HandlerBase.RemoveRequiredAttribute(child, KeyAttributeName); String value; if (ValueRequired) value = HandlerBase.RemoveRequiredAttribute(child, ValueAttributeName); else value = HandlerBase.RemoveAttribute(child, ValueAttributeName); HandlerBase.CheckForUnrecognizedAttributes(child); if (value == null) value = ""; res[key] = value; } else if (child.Name == "remove") { HandlerBase.CheckForChildNodes(child); String key = HandlerBase.RemoveRequiredAttribute(child, KeyAttributeName); HandlerBase.CheckForUnrecognizedAttributes(child); res.Remove(key); } else if (child.Name.Equals("clear")) { HandlerBase.CheckForChildNodes(child); HandlerBase.CheckForUnrecognizedAttributes(child); res.Clear(); } else { HandlerBase.ThrowUnrecognizedElement(child); } } return res; } /// /// Make the name of the key attribute configurable by derived classes. /// protected virtual string KeyAttributeName { get { return "key";} } ////// Make the name of the value attribute configurable by derived classes. /// protected virtual string ValueAttributeName { get { return "value";} } // internal virtual bool ValueRequired { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; ////// Simple dictionary config factory /// config is a dictionary mapping key->value /// /// <add key="name" value="text"> sets key=text /// <remove key="name"> removes the definition of key /// <clear> removes all definitions /// /// public class DictionarySectionHandler : IConfigurationSectionHandler { ////// Given a partially composed config object (possibly null) /// and some input from the config system, return a /// further partially composed config object /// public virtual object Create(Object parent, Object context, XmlNode section) { Hashtable res; // start res off as a shallow clone of the parent if (parent == null) res = new Hashtable(StringComparer.OrdinalIgnoreCase); else res = (Hashtable)((Hashtable)parent).Clone(); // process XML HandlerBase.CheckForUnrecognizedAttributes(section); foreach (XmlNode child in section.ChildNodes) { // skip whitespace and comments, throws if non-element if (HandlerBase.IsIgnorableAlsoCheckForNonElement(child)) continue; // handle, , tags if (child.Name == "add") { HandlerBase.CheckForChildNodes(child); String key = HandlerBase.RemoveRequiredAttribute(child, KeyAttributeName); String value; if (ValueRequired) value = HandlerBase.RemoveRequiredAttribute(child, ValueAttributeName); else value = HandlerBase.RemoveAttribute(child, ValueAttributeName); HandlerBase.CheckForUnrecognizedAttributes(child); if (value == null) value = ""; res[key] = value; } else if (child.Name == "remove") { HandlerBase.CheckForChildNodes(child); String key = HandlerBase.RemoveRequiredAttribute(child, KeyAttributeName); HandlerBase.CheckForUnrecognizedAttributes(child); res.Remove(key); } else if (child.Name.Equals("clear")) { HandlerBase.CheckForChildNodes(child); HandlerBase.CheckForUnrecognizedAttributes(child); res.Clear(); } else { HandlerBase.ThrowUnrecognizedElement(child); } } return res; } /// /// Make the name of the key attribute configurable by derived classes. /// protected virtual string KeyAttributeName { get { return "key";} } ////// Make the name of the value attribute configurable by derived classes. /// protected virtual string ValueAttributeName { get { return "value";} } // internal virtual bool ValueRequired { get { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
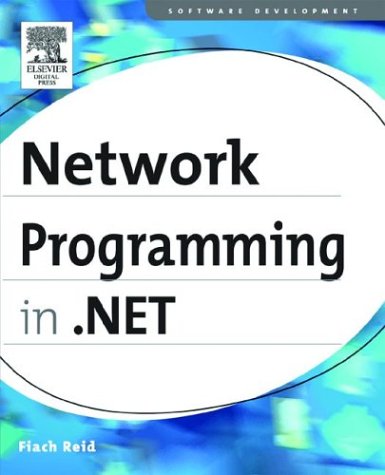
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PathFigure.cs
- HttpHostedTransportConfiguration.cs
- AssemblyResourceLoader.cs
- XmlNamespaceManager.cs
- InOutArgumentConverter.cs
- RegistrationServices.cs
- EdmConstants.cs
- WindowsScroll.cs
- ObjectDisposedException.cs
- InputReport.cs
- NullableDecimalSumAggregationOperator.cs
- TextTreeInsertUndoUnit.cs
- InputScope.cs
- ProfileSection.cs
- FilterException.cs
- DataControlFieldCollection.cs
- AspNetPartialTrustHelpers.cs
- GridItemCollection.cs
- EventTrigger.cs
- OletxTransactionManager.cs
- FontNameEditor.cs
- PolicyManager.cs
- RepeaterCommandEventArgs.cs
- CodeTypeOfExpression.cs
- WizardPanel.cs
- PropertyTabAttribute.cs
- WinCategoryAttribute.cs
- ListViewItemMouseHoverEvent.cs
- ListControl.cs
- DbException.cs
- OutKeywords.cs
- documentsequencetextcontainer.cs
- DLinqDataModelProvider.cs
- ResourceExpressionBuilder.cs
- SaveWorkflowAsyncResult.cs
- SqlNamer.cs
- sqlnorm.cs
- SafeWaitHandle.cs
- CompletionBookmark.cs
- JoinGraph.cs
- SqlDataSourceStatusEventArgs.cs
- ComboBox.cs
- CommandConverter.cs
- PartitionedDataSource.cs
- ResourceDescriptionAttribute.cs
- SiteMapProvider.cs
- StringFunctions.cs
- ReachDocumentSequenceSerializerAsync.cs
- TextDecorationCollection.cs
- mediaeventshelper.cs
- MenuScrollingVisibilityConverter.cs
- securitycriticaldata.cs
- FixedTextContainer.cs
- Documentation.cs
- EncoderReplacementFallback.cs
- BasePattern.cs
- Token.cs
- PromptBuilder.cs
- ExceptionUtility.cs
- CoTaskMemUnicodeSafeHandle.cs
- CachedRequestParams.cs
- AutoSizeComboBox.cs
- WindowVisualStateTracker.cs
- StatusCommandUI.cs
- HMACRIPEMD160.cs
- _Connection.cs
- ObjectDesignerDataSourceView.cs
- InfoCardTraceRecord.cs
- ComponentEvent.cs
- VerificationException.cs
- MimeTypeAttribute.cs
- HtmlGenericControl.cs
- AnnotationDocumentPaginator.cs
- QilValidationVisitor.cs
- ProgressPage.cs
- XmlQueryRuntime.cs
- XmlnsCache.cs
- FileInfo.cs
- DoubleAnimationBase.cs
- HwndKeyboardInputProvider.cs
- ClrPerspective.cs
- SqlServer2KCompatibilityAnnotation.cs
- BStrWrapper.cs
- TextSpanModifier.cs
- SimpleType.cs
- PnrpPermission.cs
- PerformanceCounterCategory.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- MSHTMLHost.cs
- DragEventArgs.cs
- SplitterCancelEvent.cs
- VirtualPathProvider.cs
- ServicePerformanceCounters.cs
- CodeGotoStatement.cs
- CatalogPartChrome.cs
- ScrollViewerAutomationPeer.cs
- KnownIds.cs
- XmlILAnnotation.cs
- DesignerInterfaces.cs
- UriWriter.cs