Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / Validation / ValidationError.cs / 1305376 / ValidationError.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections; using System.Globalization; #region Class ValidationError [Serializable()] public sealed class ValidationError { private string errorText = string.Empty; private int errorNumber = 0; private Hashtable userData = null; private bool isWarning = false; string propertyName = null; public ValidationError(string errorText, int errorNumber) : this(errorText, errorNumber, false, null) { } public ValidationError(string errorText, int errorNumber, bool isWarning) : this(errorText, errorNumber, isWarning, null) { } public ValidationError(string errorText, int errorNumber, bool isWarning, string propertyName) { this.errorText = errorText; this.errorNumber = errorNumber; this.isWarning = isWarning; this.propertyName = propertyName; } public string PropertyName { get { return this.propertyName; } set { this.propertyName = value; } } public string ErrorText { get { return this.errorText; } } public bool IsWarning { get { return this.isWarning; } } public int ErrorNumber { get { return this.errorNumber; } } public IDictionary UserData { get { if (this.userData == null) this.userData = new Hashtable(); return this.userData; } } public static ValidationError GetNotSetValidationError(string propertyName) { ValidationError error = new ValidationError(SR.GetString(SR.Error_PropertyNotSet, propertyName), ErrorNumbers.Error_PropertyNotSet); error.PropertyName = propertyName; return error; } public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0} {1}: {2}", this.isWarning ? "warning" : "error", this.errorNumber, this.errorText); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
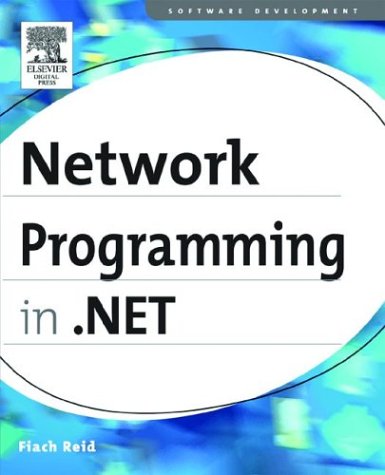
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SiteMapNodeCollection.cs
- TriggerBase.cs
- MenuRendererClassic.cs
- OLEDB_Util.cs
- XmlEncApr2001.cs
- VirtualizedContainerService.cs
- ReturnType.cs
- Int64AnimationBase.cs
- CellQuery.cs
- TextHidden.cs
- RegistryConfigurationProvider.cs
- TextServicesDisplayAttribute.cs
- Quaternion.cs
- DataGridViewRowCancelEventArgs.cs
- ProxyFragment.cs
- AnnouncementInnerClient11.cs
- MonitorWrapper.cs
- Wizard.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- XmlAttributeOverrides.cs
- DependencyPropertyKind.cs
- SmiGettersStream.cs
- CompressStream.cs
- SchemaMerger.cs
- SqlMetaData.cs
- FixedSOMPage.cs
- SingleConverter.cs
- SafeNativeHandle.cs
- StateMachineWorkflow.cs
- ToolStripDropDownItem.cs
- controlskin.cs
- Freezable.cs
- LinkDescriptor.cs
- TableRowGroup.cs
- SecureStringHasher.cs
- SessionEndingEventArgs.cs
- SplineKeyFrames.cs
- StringStorage.cs
- Boolean.cs
- FirstMatchCodeGroup.cs
- TreeWalker.cs
- ObjectDataSourceEventArgs.cs
- compensatingcollection.cs
- DeploymentExceptionMapper.cs
- LogLogRecordEnumerator.cs
- _LazyAsyncResult.cs
- PublisherMembershipCondition.cs
- Operator.cs
- URLAttribute.cs
- RemoteWebConfigurationHostStream.cs
- OleDbException.cs
- IERequestCache.cs
- ListBindableAttribute.cs
- Figure.cs
- ActivityStateQuery.cs
- RelatedPropertyManager.cs
- mediaeventshelper.cs
- StreamUpgradeInitiator.cs
- TextTreeText.cs
- MatrixCamera.cs
- TaskFormBase.cs
- ConstraintCollection.cs
- XmlNavigatorStack.cs
- _ProxyChain.cs
- Matrix3DConverter.cs
- SQLSingleStorage.cs
- ExceptionHelpers.cs
- SqlLiftIndependentRowExpressions.cs
- processwaithandle.cs
- DocumentSchemaValidator.cs
- DataGridPagerStyle.cs
- BitmapEffectDrawingContextState.cs
- JsonGlobals.cs
- HttpPostProtocolReflector.cs
- SymbolType.cs
- DataGridViewCellStyle.cs
- Util.cs
- XmlReflectionImporter.cs
- MetadataItemSerializer.cs
- FlowLayoutSettings.cs
- MonitoringDescriptionAttribute.cs
- PermissionSetEnumerator.cs
- CodeArrayIndexerExpression.cs
- QueryContinueDragEvent.cs
- ByteKeyFrameCollection.cs
- BehaviorEditorPart.cs
- Assign.cs
- TransformedBitmap.cs
- FontDifferentiator.cs
- SelectedGridItemChangedEvent.cs
- CompressStream.cs
- FileDialogCustomPlaces.cs
- ExpressionBinding.cs
- AsymmetricSignatureFormatter.cs
- PEFileReader.cs
- ProcessModule.cs
- XmlTextReader.cs
- InputLangChangeEvent.cs
- CLSCompliantAttribute.cs
- WindowsEditBox.cs