Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SafeNativeHandle.cs / 1 / SafeNativeHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using Microsoft.Win32.SafeHandles; // // Summary: // This is a wrapper for Native handles that ensures that they get closed and that the handle // held by this object isn't recycled. // internal sealed class SafeNativeHandle : SafeHandleZeroOrMinusOneIsInvalid { // Called by P/Invoke marshaler private SafeNativeHandle() : base( true ) { } // // Summary: // Creates a new SafeNativeHandle. // // Parameters: // existingHandle - A pointer to an existing native handle. // ownsHandle - Indicates whether this object owns the handle and should clean it up. // public SafeNativeHandle( IntPtr existingHandle, bool ownsHandle ) : base( ownsHandle ) { SetHandle( existingHandle ); } override protected bool ReleaseHandle() { // // Presharp: we want to return even in the case of failure. Useless to examine return error. // #pragma warning suppress 56523 return NativeMethods.SafeHandleOnlyMethods.CloseHandle( handle ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
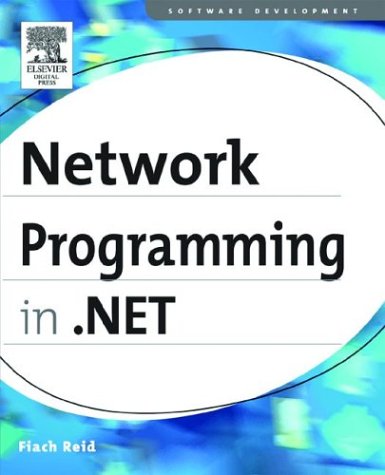
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceContextMode.cs
- ObjectListCommandEventArgs.cs
- EntityDataSourceColumn.cs
- OdbcError.cs
- SupportingTokenSecurityTokenResolver.cs
- XmlSchemaAnnotation.cs
- UnionQueryOperator.cs
- SmtpDigestAuthenticationModule.cs
- smtppermission.cs
- JsonReader.cs
- GC.cs
- TakeOrSkipQueryOperator.cs
- NativeRecognizer.cs
- ClientSettingsSection.cs
- InstanceLockedException.cs
- SqlDataSourceAdvancedOptionsForm.cs
- UrlAuthorizationModule.cs
- PageTheme.cs
- ParameterBuilder.cs
- Operator.cs
- ElementUtil.cs
- _HTTPDateParse.cs
- ClientConfigurationHost.cs
- GPRECT.cs
- HostedController.cs
- Events.cs
- OracleTransaction.cs
- StyleSelector.cs
- TypeListConverter.cs
- FontNamesConverter.cs
- JsonDeserializer.cs
- JsonDataContract.cs
- UnmanagedBitmapWrapper.cs
- SecurityElement.cs
- Visual3DCollection.cs
- SerializationAttributes.cs
- StateMachineHelpers.cs
- CompoundFileReference.cs
- nulltextcontainer.cs
- ConnectionManagementSection.cs
- TextEditorContextMenu.cs
- ObjectItemLoadingSessionData.cs
- DoubleLink.cs
- EnumerationRangeValidationUtil.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- FileVersionInfo.cs
- WithParamAction.cs
- StylusPlugin.cs
- TextEditorParagraphs.cs
- BitmapEffectOutputConnector.cs
- StringUtil.cs
- SessionStateItemCollection.cs
- ErrorRuntimeConfig.cs
- HostedElements.cs
- DependencyStoreSurrogate.cs
- Padding.cs
- SQLBytes.cs
- ScrollBar.cs
- CaseCqlBlock.cs
- DispatchWrapper.cs
- SecurityException.cs
- DefaultValueTypeConverter.cs
- ArglessEventHandlerProxy.cs
- ListChunk.cs
- TTSEvent.cs
- Enum.cs
- KeySpline.cs
- SqlDependencyListener.cs
- GeometryModel3D.cs
- DocumentPage.cs
- Native.cs
- StylusPointPropertyInfoDefaults.cs
- Dynamic.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- XmlEncoding.cs
- CreateUserErrorEventArgs.cs
- _ProxyRegBlob.cs
- DriveNotFoundException.cs
- ContainerSelectorActiveEvent.cs
- OperationPickerDialog.designer.cs
- XPathScanner.cs
- Registry.cs
- SecurityContext.cs
- SafeNativeMethods.cs
- TextServicesPropertyRanges.cs
- MetadataReference.cs
- QueryStringHandler.cs
- ProgressBarRenderer.cs
- AuthenticationServiceManager.cs
- ControlAdapter.cs
- ChildrenQuery.cs
- HttpRawResponse.cs
- WizardPanel.cs
- IPEndPoint.cs
- Version.cs
- FixedPageProcessor.cs
- ToolTip.cs
- BaseResourcesBuildProvider.cs
- TempEnvironment.cs
- TextRange.cs