Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / IntSecurity.cs / 1 / IntSecurity.cs
namespace System.Web { using System.Web; using System.Web.Util; using System.Security; using System.Security.Permissions; internal static class InternalSecurityPermissions { private static IStackWalk _unrestricted; private static IStackWalk _unmanagedCode; #if UNUSED_CODE private static IStackWalk _sensitiveInformation; #endif private static IStackWalk _controlPrincipal; #if UNUSED_CODE private static IStackWalk _controlEvidence; #endif private static IStackWalk _reflection; private static IStackWalk _appPathDiscovery; private static IStackWalk _controlThread; #if UNUSED_CODE private static IStackWalk _levelMinimal; #endif private static IStackWalk _levelLow; private static IStackWalk _levelMedium; private static IStackWalk _levelHigh; #if UNUSED_CODE private static IStackWalk _levelUnrestricted; #endif // // Static permissions as properties, created on demand // internal static IStackWalk Unrestricted { get { if (_unrestricted == null) _unrestricted = new PermissionSet(PermissionState.Unrestricted); Debug.Trace("Permissions", "Unrestricted Set"); return _unrestricted; } } internal static IStackWalk UnmanagedCode { get { if (_unmanagedCode == null) _unmanagedCode = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); Debug.Trace("Permissions", "UnmanagedCode"); return _unmanagedCode; } } #if UNUSED_CODE internal static IStackWalk SensitiveInformation { get { if (_sensitiveInformation == null) _sensitiveInformation = new EnvironmentPermission(PermissionState.Unrestricted); Debug.Trace("Permissions", "SensitiveInformation"); return _sensitiveInformation; } } #endif internal static IStackWalk ControlPrincipal { get { if (_controlPrincipal == null) _controlPrincipal = new SecurityPermission(SecurityPermissionFlag.ControlPrincipal); Debug.Trace("Permissions", "ControlPrincipal"); return _controlPrincipal; } } #if UNUSED_CODE internal static IStackWalk ControlEvidence { get { if (_controlEvidence == null) _controlEvidence = new SecurityPermission(SecurityPermissionFlag.ControlEvidence); Debug.Trace("Permissions", "ControlEvidence"); return _controlEvidence; } } #endif internal static IStackWalk Reflection { get { if (_reflection == null) _reflection = new ReflectionPermission(ReflectionPermissionFlag.MemberAccess); Debug.Trace("Permissions", "Reflection"); return _reflection; } } internal static IStackWalk AppPathDiscovery { get { if (_appPathDiscovery == null) _appPathDiscovery = new FileIOPermission(FileIOPermissionAccess.PathDiscovery, HttpRuntime.AppDomainAppPathInternal); Debug.Trace("Permissions", "AppPathDiscovery"); return _appPathDiscovery; } } internal static IStackWalk ControlThread { get { if (_controlThread == null) _controlThread = new SecurityPermission(SecurityPermissionFlag.ControlThread); Debug.Trace("Permissions", "ControlThread"); return _controlThread; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelMinimal { get { if (_levelMinimal == null) _levelMinimal = new AspNetHostingPermission(AspNetHostingPermissionLevel.Minimal); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMinimal"); return _levelMinimal; } } #endif internal static IStackWalk AspNetHostingPermissionLevelLow { get { if (_levelLow == null) _levelLow = new AspNetHostingPermission(AspNetHostingPermissionLevel.Low); Debug.Trace("Permissions", "AspNetHostingPermissionLevelLow"); return _levelLow; } } internal static IStackWalk AspNetHostingPermissionLevelMedium { get { if (_levelMedium == null) _levelMedium = new AspNetHostingPermission(AspNetHostingPermissionLevel.Medium); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMedium"); return _levelMedium; } } internal static IStackWalk AspNetHostingPermissionLevelHigh { get { if (_levelHigh == null) _levelHigh = new AspNetHostingPermission(AspNetHostingPermissionLevel.High); Debug.Trace("Permissions", "AspNetHostingPermissionLevelHigh"); return _levelHigh; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelUnrestricted { get { if (_levelUnrestricted == null) _levelUnrestricted = new AspNetHostingPermission(AspNetHostingPermissionLevel.Unrestricted); Debug.Trace("Permissions", "AspNetHostingPermissionLevelUnrestricted"); return _levelUnrestricted; } } #endif // Parameterized permissions internal static IStackWalk FileReadAccess(String filename) { Debug.Trace("Permissions", "FileReadAccess(" + filename + ")"); return new FileIOPermission(FileIOPermissionAccess.Read, filename); } internal static IStackWalk PathDiscovery(String path) { Debug.Trace("Permissions", "PathDiscovery(" + path + ")"); return new FileIOPermission(FileIOPermissionAccess.PathDiscovery, path); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web { using System.Web; using System.Web.Util; using System.Security; using System.Security.Permissions; internal static class InternalSecurityPermissions { private static IStackWalk _unrestricted; private static IStackWalk _unmanagedCode; #if UNUSED_CODE private static IStackWalk _sensitiveInformation; #endif private static IStackWalk _controlPrincipal; #if UNUSED_CODE private static IStackWalk _controlEvidence; #endif private static IStackWalk _reflection; private static IStackWalk _appPathDiscovery; private static IStackWalk _controlThread; #if UNUSED_CODE private static IStackWalk _levelMinimal; #endif private static IStackWalk _levelLow; private static IStackWalk _levelMedium; private static IStackWalk _levelHigh; #if UNUSED_CODE private static IStackWalk _levelUnrestricted; #endif // // Static permissions as properties, created on demand // internal static IStackWalk Unrestricted { get { if (_unrestricted == null) _unrestricted = new PermissionSet(PermissionState.Unrestricted); Debug.Trace("Permissions", "Unrestricted Set"); return _unrestricted; } } internal static IStackWalk UnmanagedCode { get { if (_unmanagedCode == null) _unmanagedCode = new SecurityPermission(SecurityPermissionFlag.UnmanagedCode); Debug.Trace("Permissions", "UnmanagedCode"); return _unmanagedCode; } } #if UNUSED_CODE internal static IStackWalk SensitiveInformation { get { if (_sensitiveInformation == null) _sensitiveInformation = new EnvironmentPermission(PermissionState.Unrestricted); Debug.Trace("Permissions", "SensitiveInformation"); return _sensitiveInformation; } } #endif internal static IStackWalk ControlPrincipal { get { if (_controlPrincipal == null) _controlPrincipal = new SecurityPermission(SecurityPermissionFlag.ControlPrincipal); Debug.Trace("Permissions", "ControlPrincipal"); return _controlPrincipal; } } #if UNUSED_CODE internal static IStackWalk ControlEvidence { get { if (_controlEvidence == null) _controlEvidence = new SecurityPermission(SecurityPermissionFlag.ControlEvidence); Debug.Trace("Permissions", "ControlEvidence"); return _controlEvidence; } } #endif internal static IStackWalk Reflection { get { if (_reflection == null) _reflection = new ReflectionPermission(ReflectionPermissionFlag.MemberAccess); Debug.Trace("Permissions", "Reflection"); return _reflection; } } internal static IStackWalk AppPathDiscovery { get { if (_appPathDiscovery == null) _appPathDiscovery = new FileIOPermission(FileIOPermissionAccess.PathDiscovery, HttpRuntime.AppDomainAppPathInternal); Debug.Trace("Permissions", "AppPathDiscovery"); return _appPathDiscovery; } } internal static IStackWalk ControlThread { get { if (_controlThread == null) _controlThread = new SecurityPermission(SecurityPermissionFlag.ControlThread); Debug.Trace("Permissions", "ControlThread"); return _controlThread; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelMinimal { get { if (_levelMinimal == null) _levelMinimal = new AspNetHostingPermission(AspNetHostingPermissionLevel.Minimal); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMinimal"); return _levelMinimal; } } #endif internal static IStackWalk AspNetHostingPermissionLevelLow { get { if (_levelLow == null) _levelLow = new AspNetHostingPermission(AspNetHostingPermissionLevel.Low); Debug.Trace("Permissions", "AspNetHostingPermissionLevelLow"); return _levelLow; } } internal static IStackWalk AspNetHostingPermissionLevelMedium { get { if (_levelMedium == null) _levelMedium = new AspNetHostingPermission(AspNetHostingPermissionLevel.Medium); Debug.Trace("Permissions", "AspNetHostingPermissionLevelMedium"); return _levelMedium; } } internal static IStackWalk AspNetHostingPermissionLevelHigh { get { if (_levelHigh == null) _levelHigh = new AspNetHostingPermission(AspNetHostingPermissionLevel.High); Debug.Trace("Permissions", "AspNetHostingPermissionLevelHigh"); return _levelHigh; } } #if UNUSED_CODE internal static IStackWalk AspNetHostingPermissionLevelUnrestricted { get { if (_levelUnrestricted == null) _levelUnrestricted = new AspNetHostingPermission(AspNetHostingPermissionLevel.Unrestricted); Debug.Trace("Permissions", "AspNetHostingPermissionLevelUnrestricted"); return _levelUnrestricted; } } #endif // Parameterized permissions internal static IStackWalk FileReadAccess(String filename) { Debug.Trace("Permissions", "FileReadAccess(" + filename + ")"); return new FileIOPermission(FileIOPermissionAccess.Read, filename); } internal static IStackWalk PathDiscovery(String path) { Debug.Trace("Permissions", "PathDiscovery(" + path + ")"); return new FileIOPermission(FileIOPermissionAccess.PathDiscovery, path); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
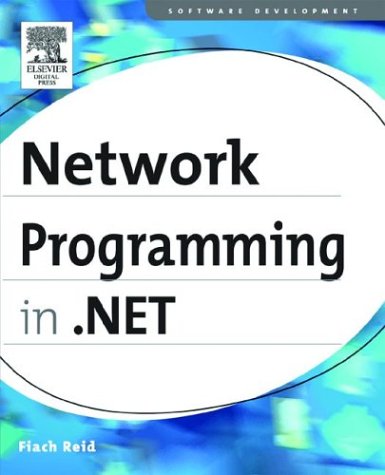
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CrossAppDomainChannel.cs
- XmlDocumentSerializer.cs
- HtmlInputControl.cs
- DataGridViewDataConnection.cs
- RegexInterpreter.cs
- ButtonBase.cs
- OletxTransactionManager.cs
- SerializableAttribute.cs
- Margins.cs
- FirstMatchCodeGroup.cs
- arabicshape.cs
- RangeContentEnumerator.cs
- QueryLifecycle.cs
- CharAnimationUsingKeyFrames.cs
- DataSourceCache.cs
- AuthorizationRule.cs
- ChannelCacheDefaults.cs
- CodeAttributeArgument.cs
- InvokeProviderWrapper.cs
- HtmlInputHidden.cs
- SqlProcedureAttribute.cs
- DefaultProfileManager.cs
- InputReport.cs
- GPRECTF.cs
- FreezableOperations.cs
- PointF.cs
- DataServiceRequest.cs
- SelectionProviderWrapper.cs
- GenericAuthenticationEventArgs.cs
- SerialErrors.cs
- UITypeEditor.cs
- ImpersonationContext.cs
- CompilerErrorCollection.cs
- FlowDocument.cs
- XmlNodeReader.cs
- Span.cs
- ComPlusTypeValidator.cs
- BitVector32.cs
- SetStateDesigner.cs
- HtmlTitle.cs
- HttpModuleAction.cs
- StringStorage.cs
- Rotation3DAnimation.cs
- BrowserCapabilitiesCompiler.cs
- initElementDictionary.cs
- VersionPair.cs
- ToolStripScrollButton.cs
- printdlgexmarshaler.cs
- XsltOutput.cs
- TextEffectResolver.cs
- DesignerAdapterAttribute.cs
- wpf-etw.cs
- MILUtilities.cs
- MultipartIdentifier.cs
- HorizontalAlignConverter.cs
- Message.cs
- TextComposition.cs
- FileDialogPermission.cs
- AnyReturnReader.cs
- TcpAppDomainProtocolHandler.cs
- DataServiceQueryContinuation.cs
- XslNumber.cs
- ValidationRuleCollection.cs
- TextServicesCompartment.cs
- FixedSOMTableRow.cs
- TreeNodeStyleCollection.cs
- basecomparevalidator.cs
- ConfigPathUtility.cs
- ValueProviderWrapper.cs
- BehaviorEditorPart.cs
- ToolStripItemClickedEventArgs.cs
- MethodCallTranslator.cs
- CLSCompliantAttribute.cs
- UpdateExpressionVisitor.cs
- OdbcHandle.cs
- ProvideValueServiceProvider.cs
- RegexWorker.cs
- ComplexLine.cs
- BaseCollection.cs
- WebPartTransformer.cs
- Validator.cs
- QueryStringParameter.cs
- DefaultParameterValueAttribute.cs
- PersonalizationProvider.cs
- ErrorLog.cs
- AdornerLayer.cs
- HtmlWindow.cs
- MD5CryptoServiceProvider.cs
- CatalogZoneBase.cs
- OrderingExpression.cs
- CacheMode.cs
- CollectionBuilder.cs
- OdbcCommand.cs
- WebContentFormatHelper.cs
- FrameworkElement.cs
- HtmlElementEventArgs.cs
- HttpCapabilitiesEvaluator.cs
- ThreadAttributes.cs
- XamlDesignerSerializationManager.cs
- GroupItem.cs