Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Packaging / PartEditor.cs / 1 / PartEditor.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: PartEditor.cs Abstract: This file contains the definition and implementation for PartEditor and XmlPartEditor classes. These classes are used as wrappers around the Metro package API part classes. Author: [....] ([....]) 1-November-2004 Revision History: --*/ using System; using System.Diagnostics; using System.Security.Permissions; using System.IO; using System.Collections; using System.Collections.Generic; using System.IO.Packaging; using System.Windows; using System.Windows.Markup; using System.Xml; namespace System.Windows.Xps.Packaging { ////// Provides access to the stream of a Metro part /// internal class PartEditor : IDisposable { #region Constructors ////// Attach an editor to a part /// /// internal PartEditor( PackagePart metroPart ) { if (null == metroPart) { throw new ArgumentNullException("metroPart"); } _metroPart = metroPart; } #endregion Constructors #region Protected properties protected PackagePart MetroPart { get { return _metroPart; } } #endregion Protected properties #region Internal properties ////// Return a data stream for the part /// ///internal Stream DataStream { get { if (null == _partDataStream) { if (_metroPart.Package.FileOpenAccess == FileAccess.Write) { _partDataStream = _metroPart.GetStream(FileMode.Create); } else { _partDataStream = _metroPart.GetStream(FileMode.OpenOrCreate); } } return _partDataStream; } } #endregion Internal properties #region Internal methods /// /// Close the part data stream /// internal virtual void Close( ) { if (null != _partDataStream) { if (_partDataStream.CanWrite) { _partDataStream.Close(); } _partDataStream = null; } } ////// Forcibly flush the part data stream /// internal virtual void Flush( ) { if (null != _partDataStream) { _partDataStream.Flush(); } } ////// Forcibly flush the part data stream /// internal virtual void FlushRelationships( ) { if (null != _metroPart) { _metroPart.FlushRelationships(); } } #endregion Internal methods #region Private data private PackagePart _metroPart; private Stream _partDataStream; #endregion Private data #region IDisposable implementation void IDisposable.Dispose( ) { Close(); } #endregion IDisposable implementation } ////// Part editor with XML content /// internal class XmlPartEditor : PartEditor { #region Constructors ////// Attach an editor to an existing part /// /// internal XmlPartEditor( PackagePart metroPart ) : base(metroPart) { _doesWriteStartEndTags = true; _isStartElementWritten = false; } #endregion Constructors #region Internal properties internal bool DoesWriteStartEndTags { get { return _doesWriteStartEndTags; } set { _doesWriteStartEndTags = value; } } internal bool IsStartElementWritten { get { return _isStartElementWritten; } } internal XmlTextWriter XmlWriter { get { if (null == _xmlWriter) { OpenDocumentForWrite(); } return _xmlWriter; } } internal XmlTextReader XmlReader { get { if (null == _xmlReader) { OpenDocumentForRead(); } return _xmlReader; } } #endregion Internal properties #region Internal methods ////// Setup for writing /// internal void OpenDocumentForRead( ) { if (_xmlReader != null) { throw new XpsPackagingException(ReachSR.Get(ReachSRID.ReachPackaging_OpenDocOrElementAlreadyCalled)); } Stream stream = MetroPart.GetStream(FileMode.Open); _xmlReader = new XmlTextReader(stream); } ////// Setup for writing /// internal void OpenDocumentForWrite( ) { if (_xmlWriter != null) { throw new XpsPackagingException(ReachSR.Get(ReachSRID.ReachPackaging_OpenDocOrElementAlreadyCalled)); } Stream stream = MetroPart.GetStream(FileMode.Create); _xmlWriter = new XmlTextWriter(stream, System.Text.Encoding.UTF8); } internal void PrepareXmlWriter( string startTag, string namespaceUri ) { if (null == _xmlWriter) { // // Stream not open yet, create it and write open tag // OpenDocumentForWrite(); if (_doesWriteStartEndTags) { _xmlWriter.WriteStartDocument(); } _xmlWriter.WriteStartElement(startTag, namespaceUri); _isStartElementWritten = true; } } ////// Flush any XML written so far for sequence (does not end Sequence tag) /// internal override void Flush( ) { if (null != _xmlWriter) { _xmlWriter.Flush(); } } ////// Close XML written for sequence (adds end Sequence tag) /// internal override void Close( ) { if (null != _xmlWriter) { _xmlWriter.Close(); _xmlWriter = null; } if (null != _xmlReader) { _xmlReader.Close(); _xmlReader = null; } base.Close(); } #endregion Internal methods #region Private data private bool _doesWriteStartEndTags; private XmlTextWriter _xmlWriter; private XmlTextReader _xmlReader; private bool _isStartElementWritten; #endregion Private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
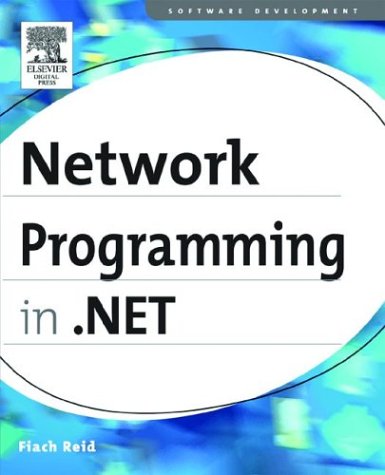
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SplitContainer.cs
- HttpMethodConstraint.cs
- SecurityUniqueId.cs
- DBPropSet.cs
- DbProviderSpecificTypePropertyAttribute.cs
- WebPartTransformer.cs
- InfoCardBinaryReader.cs
- ThaiBuddhistCalendar.cs
- RichTextBox.cs
- NamespaceCollection.cs
- HtmlTitle.cs
- CuspData.cs
- Scene3D.cs
- TypefaceCollection.cs
- ExpandCollapsePattern.cs
- BaseCodeDomTreeGenerator.cs
- HttpDictionary.cs
- WebHttpBehavior.cs
- TextElementEnumerator.cs
- COM2IDispatchConverter.cs
- ConstraintStruct.cs
- DataObject.cs
- ColorContextHelper.cs
- AdjustableArrowCap.cs
- AssociationSetEnd.cs
- CreateUserErrorEventArgs.cs
- Comparer.cs
- TextStore.cs
- DetailsViewInsertEventArgs.cs
- StreamingContext.cs
- ConsumerConnectionPoint.cs
- ImageClickEventArgs.cs
- LOSFormatter.cs
- ScalarType.cs
- ValueHandle.cs
- _HeaderInfoTable.cs
- WindowsListBox.cs
- HttpHeaderCollection.cs
- SrgsNameValueTag.cs
- GenericPrincipal.cs
- DataGridColumn.cs
- NodeFunctions.cs
- ModulesEntry.cs
- HtmlInputFile.cs
- AppDomainUnloadedException.cs
- MarshalDirectiveException.cs
- GACMembershipCondition.cs
- AuthorizationPolicyTypeElementCollection.cs
- ByteAnimation.cs
- UnsafeNativeMethods.cs
- DesignerVerbCollection.cs
- FileVersion.cs
- Mapping.cs
- Ray3DHitTestResult.cs
- CaretElement.cs
- DSASignatureFormatter.cs
- RegexBoyerMoore.cs
- safelink.cs
- ExpressionBinding.cs
- WindowInteractionStateTracker.cs
- ObjectDataSourceMethodEventArgs.cs
- MaterialGroup.cs
- UserControlDocumentDesigner.cs
- RegexStringValidatorAttribute.cs
- WebColorConverter.cs
- EntityDataSource.cs
- DatasetMethodGenerator.cs
- IntSecurity.cs
- BindingSource.cs
- EndpointReference.cs
- templategroup.cs
- FieldNameLookup.cs
- RuleElement.cs
- Literal.cs
- SafeBitVector32.cs
- TextBoxAutomationPeer.cs
- GroupQuery.cs
- GraphicsState.cs
- ChainedAsyncResult.cs
- BoundPropertyEntry.cs
- Line.cs
- CodeSubDirectory.cs
- BitmapEffect.cs
- SafeProcessHandle.cs
- CallContext.cs
- RMEnrollmentPage1.cs
- While.cs
- TypeBuilder.cs
- SqlDataSourceParameterParser.cs
- DynamicResourceExtensionConverter.cs
- Debug.cs
- BamlReader.cs
- ReverseInheritProperty.cs
- SecurityResources.cs
- RectangleConverter.cs
- TypeConverter.cs
- WeakHashtable.cs
- assemblycache.cs
- PolyLineSegment.cs
- KeyInterop.cs