Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Automation / Peers / TextBoxAutomationPeer.cs / 1 / TextBoxAutomationPeer.cs
using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Documents; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class TextBoxAutomationPeer : TextAutomationPeer, IValueProvider { /// public TextBoxAutomationPeer(TextBox owner): base(owner) {} /// override protected string GetClassNameCore() { return "TextBox"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Edit; } /// override public object GetPattern(PatternInterface patternInterface) { object returnValue = null; if(patternInterface == PatternInterface.Value) returnValue = this; if (patternInterface == PatternInterface.Text) { returnValue = new MS.Internal.Automation.TextAdaptor(this, ((TextBoxBase)Owner).TextContainer); } if (patternInterface == PatternInterface.Scroll) { TextBox owner = (TextBox)Owner; if (owner.ScrollViewer != null) { returnValue = owner.ScrollViewer.CreateAutomationPeer(); ((AutomationPeer)returnValue).EventsSource = this; } } return returnValue; } bool IValueProvider.IsReadOnly { get { TextBox owner = (TextBox)Owner; return owner.IsReadOnly; } } string IValueProvider.Value { get { TextBox owner = (TextBox)Owner; return owner.Text; } } void IValueProvider.SetValue(string value) { if(!IsEnabled()) throw new ElementNotEnabledException(); TextBox owner = (TextBox)Owner; if (owner.IsReadOnly) { throw new ElementNotEnabledException(); } if (value == null) { throw new ArgumentNullException("value"); } owner.Text = value; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(string oldValue, string newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.ValueProperty, oldValue, newValue); } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseIsReadOnlyPropertyChangedEvent(bool oldValue, bool newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.IsReadOnlyProperty, oldValue, newValue); } } ////// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { return new List (); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Documents; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class TextBoxAutomationPeer : TextAutomationPeer, IValueProvider { /// public TextBoxAutomationPeer(TextBox owner): base(owner) {} /// override protected string GetClassNameCore() { return "TextBox"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Edit; } /// override public object GetPattern(PatternInterface patternInterface) { object returnValue = null; if(patternInterface == PatternInterface.Value) returnValue = this; if (patternInterface == PatternInterface.Text) { returnValue = new MS.Internal.Automation.TextAdaptor(this, ((TextBoxBase)Owner).TextContainer); } if (patternInterface == PatternInterface.Scroll) { TextBox owner = (TextBox)Owner; if (owner.ScrollViewer != null) { returnValue = owner.ScrollViewer.CreateAutomationPeer(); ((AutomationPeer)returnValue).EventsSource = this; } } return returnValue; } bool IValueProvider.IsReadOnly { get { TextBox owner = (TextBox)Owner; return owner.IsReadOnly; } } string IValueProvider.Value { get { TextBox owner = (TextBox)Owner; return owner.Text; } } void IValueProvider.SetValue(string value) { if(!IsEnabled()) throw new ElementNotEnabledException(); TextBox owner = (TextBox)Owner; if (owner.IsReadOnly) { throw new ElementNotEnabledException(); } if (value == null) { throw new ArgumentNullException("value"); } owner.Text = value; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(string oldValue, string newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.ValueProperty, oldValue, newValue); } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseIsReadOnlyPropertyChangedEvent(bool oldValue, bool newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.IsReadOnlyProperty, oldValue, newValue); } } /// /// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { return new List (); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
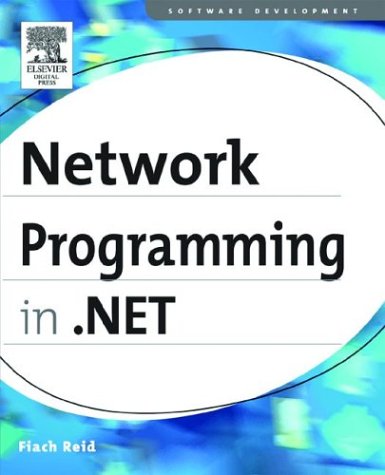
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridLength.cs
- Baml2006KeyRecord.cs
- InternalConfirm.cs
- SchemaEntity.cs
- Misc.cs
- Help.cs
- EndpointNotFoundException.cs
- SqlDataSourceAdvancedOptionsForm.cs
- XPathScanner.cs
- Cursor.cs
- ColumnWidthChangedEvent.cs
- CatalogPartChrome.cs
- SafeNativeMethods.cs
- OrderedEnumerableRowCollection.cs
- DefaultHttpHandler.cs
- StringReader.cs
- TimeSpanValidator.cs
- TextCompositionManager.cs
- PreservationFileWriter.cs
- ObjectDataSourceView.cs
- HTTPAPI_VERSION.cs
- ConfigXmlSignificantWhitespace.cs
- ObjectReaderCompiler.cs
- PropertyIDSet.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- OdbcException.cs
- Rect.cs
- ColorConvertedBitmap.cs
- EntityStoreSchemaFilterEntry.cs
- GlyphingCache.cs
- AbstractExpressions.cs
- DataGridViewDesigner.cs
- RMEnrollmentPage1.cs
- SafeViewOfFileHandle.cs
- LOSFormatter.cs
- BuildManagerHost.cs
- SiblingIterators.cs
- DecoratedNameAttribute.cs
- RectAnimationBase.cs
- MaskDesignerDialog.cs
- ContentElementAutomationPeer.cs
- clipboard.cs
- MenuAdapter.cs
- HttpConfigurationContext.cs
- ObjectDisposedException.cs
- EntityDataSourceState.cs
- CompressEmulationStream.cs
- BaseTransportHeaders.cs
- CompensationHandlingFilter.cs
- UnauthorizedWebPart.cs
- ListViewItemSelectionChangedEvent.cs
- XsltArgumentList.cs
- OleDbMetaDataFactory.cs
- QueryTreeBuilder.cs
- RuntimeConfigLKG.cs
- ToolStripRenderer.cs
- COAUTHIDENTITY.cs
- FileDialogPermission.cs
- PartBasedPackageProperties.cs
- XamlFilter.cs
- SchemaConstraints.cs
- NavigationHelper.cs
- TreeIterators.cs
- SQLMembershipProvider.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- Console.cs
- HiddenField.cs
- TextEffectCollection.cs
- AnnotationService.cs
- TextParagraphProperties.cs
- ListView.cs
- UserPreferenceChangedEventArgs.cs
- GeometryModel3D.cs
- SqlDataSourceCommandEventArgs.cs
- TypeValidationEventArgs.cs
- wgx_commands.cs
- DateTimeValueSerializerContext.cs
- RelatedView.cs
- WSTrustFeb2005.cs
- SessionPageStatePersister.cs
- TrustManagerMoreInformation.cs
- EditingScope.cs
- ComNativeDescriptor.cs
- ProviderConnectionPoint.cs
- StrokeCollectionDefaultValueFactory.cs
- ObjectStateEntryDbDataRecord.cs
- ProcessHostFactoryHelper.cs
- Size3DValueSerializer.cs
- QilReplaceVisitor.cs
- NavigatorInvalidBodyAccessException.cs
- ProviderConnectionPoint.cs
- BamlLocalizableResourceKey.cs
- TreeNodeBindingDepthConverter.cs
- MethodExpr.cs
- BindingGraph.cs
- XmlWrappingReader.cs
- SqlUnionizer.cs
- TypeSemantics.cs
- xmlsaver.cs
- DataGridViewCheckBoxColumn.cs