Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Runtime / Remoting / DynamicPropertyHolder.cs / 1 / DynamicPropertyHolder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // DynamicPropertyHolder manages the dynamically registered properties // and the sinks contributed by them. Dynamic properties may be registered // to contribute sinks on a per-object basis (on the proxy or server side) // or on a per-Context basis (in both the client and server contexts). // // See also: RemotingServices.RegisterDynamicSink() API // namespace System.Runtime.Remoting.Contexts { using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System; using System.Collections; using System.Globalization; internal class DynamicPropertyHolder { private const int GROW_BY = 0x8; private IDynamicProperty[] _props; private int _numProps; private IDynamicMessageSink[] _sinks; internal virtual bool AddDynamicProperty(IDynamicProperty prop) { lock(this) { // We have to add a sink specific to the given context CheckPropertyNameClash(prop.Name, _props, _numProps); // check if we need to grow the array. bool bGrow=false; if (_props == null || _numProps == _props.Length) { _props = GrowPropertiesArray(_props); bGrow = true; } // now add the property _props[_numProps++] = prop; // we need to grow the sinks if we grew the props array or we had thrown // away the sinks array due to a recent removal! if (bGrow) { _sinks = GrowDynamicSinksArray(_sinks); } if (_sinks == null) { // Some property got unregistered -- we need to recreate // the list of sinks. _sinks = new IDynamicMessageSink[_props.Length]; for (int i=0; i<_numProps; i++) { _sinks[i] = ((IContributeDynamicSink)_props[i]).GetDynamicSink(); } } else { // append the Sink to the existing array of Sinks _sinks[_numProps-1] = ((IContributeDynamicSink)prop).GetDynamicSink(); } return true; } } internal virtual bool RemoveDynamicProperty(String name) { lock(this) { // We have to remove a property for a specific context for (int i=0; i<_numProps; i++) { if (_props[i].Name.Equals(name)) { _props[i] = _props[_numProps-1]; _numProps--; // throw away the dynamic sink list _sinks = null; return true; } } throw new RemotingException( String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString("Remoting_Contexts_NoProperty"), name)); } } internal virtual IDynamicProperty[] DynamicProperties { get { if (_props == null) { return null; } lock (this) { IDynamicProperty[] retProps = new IDynamicProperty[_numProps]; Array.Copy(_props, retProps, _numProps); return retProps; } } } // We have to do this ArrayWithSize thing instead of // separately providing the Array and a Count ... since they // may not be in synch with multiple threads changing things // We do not want to provide a copy of the array for each // call for perf reasons. Besides this is used internally anyways. internal virtual ArrayWithSize DynamicSinks { get { if (_numProps == 0) { return null; } lock (this) { if (_sinks == null) { // Some property got unregistered -- we need to recreate // the list of sinks. _sinks = new IDynamicMessageSink[_numProps+GROW_BY]; for (int i=0; i<_numProps; i++) { _sinks[i] = ((IContributeDynamicSink)_props[i]).GetDynamicSink(); } } } return new ArrayWithSize(_sinks, _numProps); } } private static IDynamicMessageSink[] GrowDynamicSinksArray(IDynamicMessageSink[] sinks) { // grow the array int newSize = (sinks != null ? sinks.Length : 0) + GROW_BY; IDynamicMessageSink[] newSinks = new IDynamicMessageSink[newSize]; if (sinks != null) { // Copy existing properties over // Initial size should be chosen so that this rarely happens Array.Copy(sinks, newSinks, sinks.Length); } return newSinks; } internal static void NotifyDynamicSinks(IMessage msg, ArrayWithSize dynSinks, bool bCliSide, bool bStart, bool bAsync) { for (int i=0; i
Link Menu
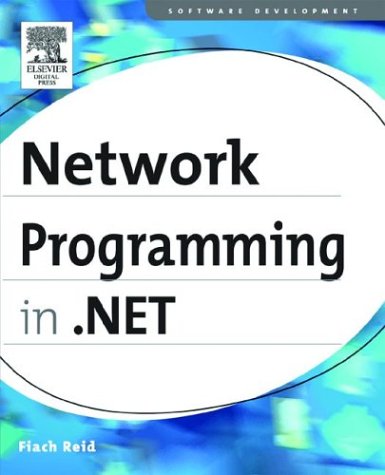
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageBuildProvider.cs
- XmlSchemaComplexContent.cs
- GifBitmapEncoder.cs
- DataGridTablesFactory.cs
- ISAPIApplicationHost.cs
- SizeAnimationUsingKeyFrames.cs
- LayoutEngine.cs
- HitTestWithPointDrawingContextWalker.cs
- SqlHelper.cs
- ColorConverter.cs
- QueryCacheEntry.cs
- SemanticResultValue.cs
- KnownAssemblyEntry.cs
- Animatable.cs
- IssuedTokenClientElement.cs
- RandomDelayQueuedSendsAsyncResult.cs
- EditorZoneDesigner.cs
- ProtocolsConfigurationEntry.cs
- ButtonFlatAdapter.cs
- TableCell.cs
- SqlRetyper.cs
- BinaryCommonClasses.cs
- XmlAttributes.cs
- ModelUIElement3D.cs
- ExistsInCollection.cs
- XsltQilFactory.cs
- CriticalHandle.cs
- LineBreak.cs
- BitmapImage.cs
- PerfCounters.cs
- ReaderWriterLockWrapper.cs
- WindowsFont.cs
- DrawingImage.cs
- SHA256.cs
- TextElementCollectionHelper.cs
- WebSysDefaultValueAttribute.cs
- Endpoint.cs
- WorkBatch.cs
- DecoratedNameAttribute.cs
- LookupBindingPropertiesAttribute.cs
- Operand.cs
- AuthenticatingEventArgs.cs
- ReadOnlyDictionary.cs
- ReachPageContentSerializer.cs
- SingleObjectCollection.cs
- ColumnBinding.cs
- BindingBase.cs
- SmiGettersStream.cs
- HtmlTernaryTree.cs
- PieceNameHelper.cs
- EnumUnknown.cs
- RectConverter.cs
- StylusDownEventArgs.cs
- SqlDataReaderSmi.cs
- EnumMember.cs
- MetadataPropertyCollection.cs
- SQLSingleStorage.cs
- TableHeaderCell.cs
- DocumentApplicationJournalEntry.cs
- documentsequencetextview.cs
- WindowsToolbarItemAsMenuItem.cs
- EncodingTable.cs
- HScrollProperties.cs
- BaseParser.cs
- IntSecurity.cs
- RetriableClipboard.cs
- CacheForPrimitiveTypes.cs
- SendOperation.cs
- CodeIdentifiers.cs
- ListDictionaryInternal.cs
- ServiceDocument.cs
- LocalizationComments.cs
- SecurityUtils.cs
- behaviorssection.cs
- SynchronizationFilter.cs
- VisemeEventArgs.cs
- MetabaseReader.cs
- QuadraticBezierSegment.cs
- EntityProviderFactory.cs
- CodeCatchClauseCollection.cs
- MimeMultiPart.cs
- CombinedGeometry.cs
- RepeaterItemEventArgs.cs
- SelectionHighlightInfo.cs
- GrammarBuilderBase.cs
- SchemaElementDecl.cs
- AsyncPostBackTrigger.cs
- ThrowHelper.cs
- Application.cs
- HeaderPanel.cs
- shaper.cs
- MaxValueConverter.cs
- Processor.cs
- unsafenativemethodsother.cs
- ListViewTableRow.cs
- Rfc2898DeriveBytes.cs
- ReliableOutputConnection.cs
- XpsException.cs
- PingReply.cs
- EncoderFallback.cs