Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / CustomLineCap.cs / 1305376 / CustomLineCap.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Versioning; ////// /// Encapsulates a custom user-defined line /// cap. /// public class CustomLineCap : MarshalByRefObject, ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif /* * Handle to native line cap object */ internal IntPtr nativeCap; // For subclass creation internal CustomLineCap() {} ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath) : this(fillPath, strokePath, LineCap.Flat) {} ////// Initializes a new instance of the ///class with the specified outline /// and fill. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath, LineCap baseCap) : this(fillPath, strokePath, baseCap, 0) {} ////// Initializes a new instance of the ///class from the /// specified existing with the specified outline and /// fill. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath, LineCap baseCap, float baseInset) { IntPtr nativeCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateCustomLineCap( new HandleRef(fillPath, (fillPath == null) ? IntPtr.Zero : fillPath.nativePath), new HandleRef(strokePath, (strokePath == null) ? IntPtr.Zero : strokePath.nativePath), baseCap, baseInset, out nativeCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeLineCap(nativeCap); } internal CustomLineCap(IntPtr nativeLineCap) { SetNativeLineCap(nativeLineCap); } internal void SetNativeLineCap(IntPtr handle) { if (handle == IntPtr.Zero) throw new ArgumentNullException("handle"); nativeCap = handle; } ////// Initializes a new instance of the ///class from the /// specified existing with the specified outline, fill, and /// inset. /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///. /// protected virtual void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeCap != IntPtr.Zero) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (nativeCap != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(this, nativeCap)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeCap = IntPtr.Zero; } } } /// /// /// Cleans up Windows resources for this /// ~CustomLineCap() { Dispose(false); } ///. /// /// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public object Clone() { IntPtr cloneCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneCustomLineCap(new HandleRef(this, nativeCap), out cloneCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return CustomLineCap.CreateCustomLineCapObject(cloneCap); } internal static CustomLineCap CreateCustomLineCapObject(IntPtr cap) { CustomLineCapType capType = 0; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapType(new HandleRef(null, cap), out capType); if (status != SafeNativeMethods.Gdip.Ok) { SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(null, cap)); throw SafeNativeMethods.Gdip.StatusException(status); } switch (capType) { case CustomLineCapType.Default: return new CustomLineCap(cap); case CustomLineCapType.AdjustableArrowCap: return new AdjustableArrowCap(cap); } SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(null, cap)); throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.NotImplemented); } ///. /// /// /// Sets the caps used to start and end lines. /// public void SetStrokeCaps(LineCap startCap, LineCap endCap) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapStrokeCaps(new HandleRef(this, nativeCap), startCap, endCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// Gets the caps used to start and end lines. /// public void GetStrokeCaps(out LineCap startCap, out LineCap endCap) { int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapStrokeCaps(new HandleRef(this, nativeCap), out startCap, out endCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private void _SetStrokeJoin(LineJoin lineJoin) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapStrokeJoin(new HandleRef(this, nativeCap), lineJoin); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private LineJoin _GetStrokeJoin() { LineJoin lineJoin; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapStrokeJoin(new HandleRef(this, nativeCap), out lineJoin); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return lineJoin; } ////// /// Gets or sets the public LineJoin StrokeJoin { get { return _GetStrokeJoin(); } set { _SetStrokeJoin(value); } } private void _SetBaseCap(LineCap baseCap) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapBaseCap(new HandleRef(this, nativeCap), baseCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private LineCap _GetBaseCap() { LineCap baseCap; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapBaseCap(new HandleRef(this, nativeCap), out baseCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return baseCap; } ///used by this custom cap. /// /// /// Gets or sets the public LineCap BaseCap { get { return _GetBaseCap(); } set { _SetBaseCap(value); } } private void _SetBaseInset(float inset) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapBaseInset(new HandleRef(this, nativeCap), inset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetBaseInset() { float inset; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapBaseInset(new HandleRef(this, nativeCap), out inset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return inset; } ///on which this is based. /// /// /// Gets or sets the distance between the cap /// and the line. /// public float BaseInset { get { return _GetBaseInset(); } set { _SetBaseInset(value); } } private void _SetWidthScale(float widthScale) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapWidthScale(new HandleRef(this, nativeCap), widthScale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetWidthScale() { float widthScale; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapWidthScale(new HandleRef(this, nativeCap), out widthScale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return widthScale; } ////// /// Gets or sets the amount by which to scale /// the width of the cap. /// public float WidthScale { get { return _GetWidthScale(); } set { _SetWidthScale(value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Versioning; ////// /// Encapsulates a custom user-defined line /// cap. /// public class CustomLineCap : MarshalByRefObject, ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif /* * Handle to native line cap object */ internal IntPtr nativeCap; // For subclass creation internal CustomLineCap() {} ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath) : this(fillPath, strokePath, LineCap.Flat) {} ////// Initializes a new instance of the ///class with the specified outline /// and fill. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath, LineCap baseCap) : this(fillPath, strokePath, baseCap, 0) {} ////// Initializes a new instance of the ///class from the /// specified existing with the specified outline and /// fill. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public CustomLineCap(GraphicsPath fillPath, GraphicsPath strokePath, LineCap baseCap, float baseInset) { IntPtr nativeCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateCustomLineCap( new HandleRef(fillPath, (fillPath == null) ? IntPtr.Zero : fillPath.nativePath), new HandleRef(strokePath, (strokePath == null) ? IntPtr.Zero : strokePath.nativePath), baseCap, baseInset, out nativeCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeLineCap(nativeCap); } internal CustomLineCap(IntPtr nativeLineCap) { SetNativeLineCap(nativeLineCap); } internal void SetNativeLineCap(IntPtr handle) { if (handle == IntPtr.Zero) throw new ArgumentNullException("handle"); nativeCap = handle; } ////// Initializes a new instance of the ///class from the /// specified existing with the specified outline, fill, and /// inset. /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///. /// protected virtual void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeCap != IntPtr.Zero) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (nativeCap != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(this, nativeCap)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeCap = IntPtr.Zero; } } } /// /// /// Cleans up Windows resources for this /// ~CustomLineCap() { Dispose(false); } ///. /// /// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public object Clone() { IntPtr cloneCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneCustomLineCap(new HandleRef(this, nativeCap), out cloneCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return CustomLineCap.CreateCustomLineCapObject(cloneCap); } internal static CustomLineCap CreateCustomLineCapObject(IntPtr cap) { CustomLineCapType capType = 0; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapType(new HandleRef(null, cap), out capType); if (status != SafeNativeMethods.Gdip.Ok) { SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(null, cap)); throw SafeNativeMethods.Gdip.StatusException(status); } switch (capType) { case CustomLineCapType.Default: return new CustomLineCap(cap); case CustomLineCapType.AdjustableArrowCap: return new AdjustableArrowCap(cap); } SafeNativeMethods.Gdip.GdipDeleteCustomLineCap(new HandleRef(null, cap)); throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.NotImplemented); } ///. /// /// /// Sets the caps used to start and end lines. /// public void SetStrokeCaps(LineCap startCap, LineCap endCap) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapStrokeCaps(new HandleRef(this, nativeCap), startCap, endCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// /// Gets the caps used to start and end lines. /// public void GetStrokeCaps(out LineCap startCap, out LineCap endCap) { int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapStrokeCaps(new HandleRef(this, nativeCap), out startCap, out endCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private void _SetStrokeJoin(LineJoin lineJoin) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapStrokeJoin(new HandleRef(this, nativeCap), lineJoin); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private LineJoin _GetStrokeJoin() { LineJoin lineJoin; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapStrokeJoin(new HandleRef(this, nativeCap), out lineJoin); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return lineJoin; } ////// /// Gets or sets the public LineJoin StrokeJoin { get { return _GetStrokeJoin(); } set { _SetStrokeJoin(value); } } private void _SetBaseCap(LineCap baseCap) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapBaseCap(new HandleRef(this, nativeCap), baseCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private LineCap _GetBaseCap() { LineCap baseCap; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapBaseCap(new HandleRef(this, nativeCap), out baseCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return baseCap; } ///used by this custom cap. /// /// /// Gets or sets the public LineCap BaseCap { get { return _GetBaseCap(); } set { _SetBaseCap(value); } } private void _SetBaseInset(float inset) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapBaseInset(new HandleRef(this, nativeCap), inset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetBaseInset() { float inset; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapBaseInset(new HandleRef(this, nativeCap), out inset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return inset; } ///on which this is based. /// /// /// Gets or sets the distance between the cap /// and the line. /// public float BaseInset { get { return _GetBaseInset(); } set { _SetBaseInset(value); } } private void _SetWidthScale(float widthScale) { int status = SafeNativeMethods.Gdip.GdipSetCustomLineCapWidthScale(new HandleRef(this, nativeCap), widthScale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetWidthScale() { float widthScale; int status = SafeNativeMethods.Gdip.GdipGetCustomLineCapWidthScale(new HandleRef(this, nativeCap), out widthScale); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return widthScale; } ////// /// Gets or sets the amount by which to scale /// the width of the cap. /// public float WidthScale { get { return _GetWidthScale(); } set { _SetWidthScale(value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
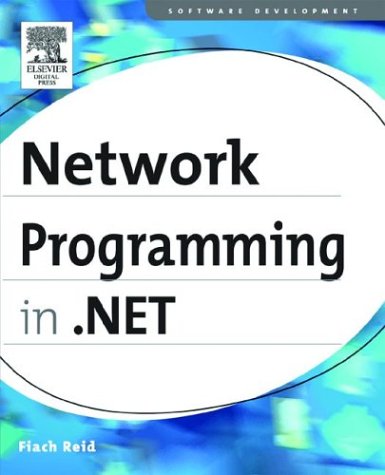
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Win32.cs
- NoneExcludedImageIndexConverter.cs
- PaintValueEventArgs.cs
- ControlLocalizer.cs
- DefaultPropertyAttribute.cs
- ColorMap.cs
- WaitHandleCannotBeOpenedException.cs
- HierarchicalDataSourceControl.cs
- XmlSchemaCollection.cs
- CircleEase.cs
- DataGridViewRowPostPaintEventArgs.cs
- XmlElementCollection.cs
- Transform.cs
- DefaultWorkflowSchedulerService.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- OutputCacheProfileCollection.cs
- DataMemberConverter.cs
- IndexingContentUnit.cs
- SafeNativeMethods.cs
- ListItemCollection.cs
- PassportPrincipal.cs
- ListBoxChrome.cs
- ServiceHostFactory.cs
- Codec.cs
- DesignOnlyAttribute.cs
- ZipIOBlockManager.cs
- LocalizedNameDescriptionPair.cs
- WebMessageBodyStyleHelper.cs
- MessageTraceRecord.cs
- Condition.cs
- TrueReadOnlyCollection.cs
- DataGridClipboardHelper.cs
- SoapCodeExporter.cs
- MasterPageCodeDomTreeGenerator.cs
- VisualTreeHelper.cs
- AvTrace.cs
- GridViewUpdateEventArgs.cs
- DataTableTypeConverter.cs
- VirtualizedContainerService.cs
- DataGridCellsPanel.cs
- ReadOnlyDictionary.cs
- _LazyAsyncResult.cs
- ExtendedPropertyDescriptor.cs
- BindingElementCollection.cs
- ItemsControlAutomationPeer.cs
- LiteralTextParser.cs
- SqlRowUpdatingEvent.cs
- DelegatingConfigHost.cs
- TrackingParameters.cs
- XPSSignatureDefinition.cs
- NamespaceEmitter.cs
- CodeNamespaceCollection.cs
- ADMembershipProvider.cs
- DesignSurface.cs
- OracleCommandSet.cs
- XmlSerializerFactory.cs
- MultiDataTrigger.cs
- DoubleKeyFrameCollection.cs
- WebPartRestoreVerb.cs
- Model3DGroup.cs
- SplitterEvent.cs
- NetCodeGroup.cs
- UpDownBase.cs
- HandledMouseEvent.cs
- Utils.cs
- DataBindingValueUIHandler.cs
- KoreanCalendar.cs
- DataTableCollection.cs
- HistoryEventArgs.cs
- AttributeUsageAttribute.cs
- ElementFactory.cs
- ToolboxItemCollection.cs
- CqlBlock.cs
- MemberListBinding.cs
- FilterEventArgs.cs
- SelectionEditingBehavior.cs
- PropertyGroupDescription.cs
- MessageDispatch.cs
- SoapAttributeAttribute.cs
- TypeGenericEnumerableViewSchema.cs
- XPathNodeInfoAtom.cs
- EntityDesignerBuildProvider.cs
- ToolboxBitmapAttribute.cs
- StrokeNodeData.cs
- Faults.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- InstanceHandleConflictException.cs
- NavigatorOutput.cs
- AutomationPeer.cs
- CheckedListBox.cs
- Property.cs
- MessageDecoder.cs
- SeparatorAutomationPeer.cs
- XmlNullResolver.cs
- ObjectIDGenerator.cs
- ellipse.cs
- CanExecuteRoutedEventArgs.cs
- StringCollection.cs
- CompilerCollection.cs
- SourceFilter.cs