Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ImageIndexEditor.cs / 1 / ImageIndexEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.ImageIndexEditor..ctor()")] namespace System.Windows.Forms.Design { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using System.ComponentModel.Design; using Microsoft.Win32; using System.Windows.Forms.Design; using System.Windows.Forms.ComponentModel; ////// /// internal class ImageIndexEditor : UITypeEditor { protected ImageList currentImageList; protected PropertyDescriptor currentImageListProp; protected object currentInstance; protected UITypeEditor imageEditor; protected string parentImageListProperty = "Parent"; protected string imageListPropertyName = null; ///Provides an editor for visually picking an image index. ////// /// public ImageIndexEditor() { // Get the type editor for images. We use the properties on // this to determine if we support value painting, etc. // imageEditor = (UITypeEditor)TypeDescriptor.GetEditor(typeof(Image), typeof(UITypeEditor)); } internal UITypeEditor ImageEditor { get { return imageEditor; } } internal string ParentImageListProperty { get { return parentImageListProperty; } } ///Initializes a new instance of the ///class. /// /// Retrieves an image for the current context at current index. /// protected virtual Image GetImage(ITypeDescriptorContext context, int index, string key, bool useIntIndex) { Image image = null; object instance = context.Instance; if(instance is object[]) { // we would not know what to do in this case anyway (i.e. multiple selection of objects) return null; } // If the instances are different, then we need to re-aquire our image list. // if ((index >= 0) || (key != null)) { if (currentImageList == null || instance != currentInstance || (currentImageListProp != null && (ImageList)currentImageListProp.GetValue(currentInstance) != currentImageList)) { currentInstance = instance; // first look for an attribute PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); // not found as an attribute, do the old behavior while(instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { currentImageList = (ImageList)imageListProp.GetValue(instance); currentImageListProp = imageListProp; currentInstance = instance; } } if (currentImageList != null) { if (useIntIndex) { if (currentImageList != null && index < currentImageList.Images.Count) { index = (index > 0) ? index : 0; image = currentImageList.Images[index]; } } else { image = currentImageList.Images[key]; } } else { // no image list, no image image = null; } } return image; } ////// /// public override bool GetPaintValueSupported(ITypeDescriptorContext context) { if (imageEditor != null) { return imageEditor.GetPaintValueSupported(context); } return false; } ///Gets a value indicating whether this editor supports the painting of a representation /// of an object's value. ////// /// public override void PaintValue(PaintValueEventArgs e) { if (ImageEditor != null){ Image image = null; if (e.Value is int) { image = GetImage(e.Context, (int)e.Value, null, true); } else if (e.Value is string) { image = GetImage(e.Context, -1, (string)e.Value, false); } if (image != null) { ImageEditor.PaintValue(new PaintValueEventArgs(e.Context, image, e.Graphics, e.Bounds)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Paints a representative value of the given object to the provided /// canvas. Painting should be done within the boundaries of the /// provided rectangle. /// ///
Link Menu
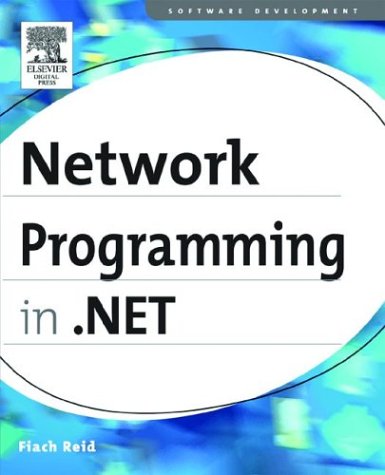
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionPoint.cs
- querybuilder.cs
- NonParentingControl.cs
- QueryFunctions.cs
- AssemblyUtil.cs
- TextEffect.cs
- HtmlInputReset.cs
- StickyNote.cs
- MenuItemStyleCollection.cs
- Crypto.cs
- indexingfiltermarshaler.cs
- SqlConnectionFactory.cs
- ThicknessAnimationBase.cs
- DataRowCollection.cs
- CompleteWizardStep.cs
- ReferenceTypeElement.cs
- ICspAsymmetricAlgorithm.cs
- MouseEventArgs.cs
- BitArray.cs
- DownloadProgressEventArgs.cs
- ExceptionCollection.cs
- BaseHashHelper.cs
- StringKeyFrameCollection.cs
- SoapSchemaExporter.cs
- WizardStepCollectionEditor.cs
- DataObjectAttribute.cs
- ModifierKeysConverter.cs
- DataGridViewLinkColumn.cs
- CornerRadius.cs
- GroupBox.cs
- BamlMapTable.cs
- serverconfig.cs
- cookie.cs
- TextModifierScope.cs
- SQLBytesStorage.cs
- Material.cs
- VisualProxy.cs
- DSACryptoServiceProvider.cs
- SQLUtility.cs
- cookiecollection.cs
- ImageField.cs
- PathFigure.cs
- CompensationTokenData.cs
- TypeExtensionSerializer.cs
- RectValueSerializer.cs
- BuilderElements.cs
- XmlSchemaNotation.cs
- TemplateControlCodeDomTreeGenerator.cs
- KeyValuePairs.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- AssemblyAttributesGoHere.cs
- XmlSchemaObjectCollection.cs
- UriTemplateTable.cs
- AQNBuilder.cs
- PropertyDescriptors.cs
- GridViewSortEventArgs.cs
- DisplayNameAttribute.cs
- CompilerScopeManager.cs
- StreamWriter.cs
- XmlSchemaAppInfo.cs
- XPathNodeInfoAtom.cs
- RelationshipType.cs
- XmlUnspecifiedAttribute.cs
- PropertyInfoSet.cs
- NativeObjectSecurity.cs
- SchemaAttDef.cs
- TagNameToTypeMapper.cs
- TextSchema.cs
- XmlHierarchicalDataSourceView.cs
- Application.cs
- FixedPageProcessor.cs
- WindowsFormsSectionHandler.cs
- ComplexTypeEmitter.cs
- DependencyProperty.cs
- XmlSchemaAppInfo.cs
- DbgUtil.cs
- ConfigurationValues.cs
- UInt16.cs
- ExpressionWriter.cs
- SqlRetyper.cs
- FileClassifier.cs
- TextEndOfParagraph.cs
- SafeRightsManagementSessionHandle.cs
- DesignerProperties.cs
- DataTableClearEvent.cs
- keycontainerpermission.cs
- UserControlBuildProvider.cs
- WmfPlaceableFileHeader.cs
- ToolBarButton.cs
- SocketCache.cs
- DataBoundControlHelper.cs
- MarkupWriter.cs
- RangeValueProviderWrapper.cs
- WriteFileContext.cs
- ClientEventManager.cs
- CompositeFontFamily.cs
- NavigationExpr.cs
- DrawTreeNodeEventArgs.cs
- MbpInfo.cs
- ListCollectionView.cs