Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / SqlNodeAnnotations.cs / 1 / SqlNodeAnnotations.cs
using System; using System.Collections.Generic; using System.Text; namespace System.Data.Linq.SqlClient { ////// Associate annotations with SqlNodes. /// internal class SqlNodeAnnotations { Dictionary> annotationMap = new Dictionary >(); Dictionary uniqueTypes = new Dictionary (); /// /// Add an annotation to the given node. /// internal void Add(SqlNode node, SqlNodeAnnotation annotation) { Listlist = null; if (!this.annotationMap.TryGetValue(node, out list)) { list = new List (); this.annotationMap[node]=list; } uniqueTypes[annotation.GetType()] = String.Empty; list.Add(annotation); } /// /// Gets the annotations for the given node. Null if none. /// internal ListGet(SqlNode node) { List list = null; this.annotationMap.TryGetValue(node, out list); return list; } /// /// Whether the given node has annotations. /// internal bool NodeIsAnnotated(SqlNode node) { if (node == null) return false; return this.annotationMap.ContainsKey(node); } ////// Checks whether there's at least one annotation of the given type. /// internal bool HasAnnotationType(Type type) { return this.uniqueTypes.ContainsKey(type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; namespace System.Data.Linq.SqlClient { ////// Associate annotations with SqlNodes. /// internal class SqlNodeAnnotations { Dictionary> annotationMap = new Dictionary >(); Dictionary uniqueTypes = new Dictionary (); /// /// Add an annotation to the given node. /// internal void Add(SqlNode node, SqlNodeAnnotation annotation) { Listlist = null; if (!this.annotationMap.TryGetValue(node, out list)) { list = new List (); this.annotationMap[node]=list; } uniqueTypes[annotation.GetType()] = String.Empty; list.Add(annotation); } /// /// Gets the annotations for the given node. Null if none. /// internal ListGet(SqlNode node) { List list = null; this.annotationMap.TryGetValue(node, out list); return list; } /// /// Whether the given node has annotations. /// internal bool NodeIsAnnotated(SqlNode node) { if (node == null) return false; return this.annotationMap.ContainsKey(node); } ////// Checks whether there's at least one annotation of the given type. /// internal bool HasAnnotationType(Type type) { return this.uniqueTypes.ContainsKey(type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
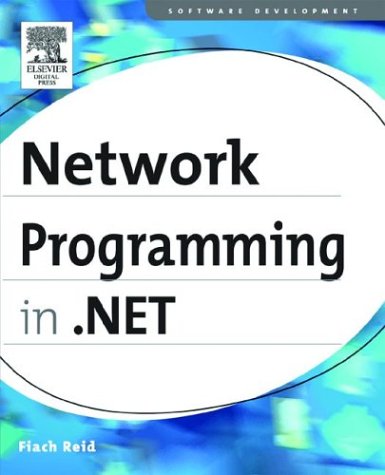
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DependencyPropertyHelper.cs
- DynamicScriptObject.cs
- Button.cs
- Parallel.cs
- ILGen.cs
- ToolStripProgressBar.cs
- ExtractCollection.cs
- DataSource.cs
- XmlAutoDetectWriter.cs
- PerformanceCounterPermission.cs
- SQLInt16.cs
- MessageDecoder.cs
- ControlPropertyNameConverter.cs
- ProgressChangedEventArgs.cs
- SamlSubject.cs
- ConnectivityStatus.cs
- _FtpControlStream.cs
- Char.cs
- ContextMenuStrip.cs
- NominalTypeEliminator.cs
- Helpers.cs
- CrossAppDomainChannel.cs
- ColumnMapProcessor.cs
- HttpCachePolicy.cs
- NeedSkipTokenVisitor.cs
- SqlUnionizer.cs
- DataTrigger.cs
- SqlXmlStorage.cs
- SchemaEntity.cs
- AutoGeneratedField.cs
- ConstructorNeedsTagAttribute.cs
- Control.cs
- SQLBinary.cs
- WebPartEditorOkVerb.cs
- NegatedConstant.cs
- MSG.cs
- OdbcConnectionOpen.cs
- DragDeltaEventArgs.cs
- PartialTrustHelpers.cs
- GridViewRow.cs
- ClickablePoint.cs
- MemberNameValidator.cs
- FormViewPageEventArgs.cs
- BaseResourcesBuildProvider.cs
- TraceSection.cs
- TextRangeSerialization.cs
- PreservationFileReader.cs
- PersonalizableAttribute.cs
- SchemaHelper.cs
- ZipPackagePart.cs
- JavaScriptObjectDeserializer.cs
- LifetimeServices.cs
- ParameterCollection.cs
- ProcessingInstructionAction.cs
- UriParserTemplates.cs
- XmlLinkedNode.cs
- ContainerParagraph.cs
- TextParagraphProperties.cs
- PrivateFontCollection.cs
- SaveFileDialog.cs
- Interop.cs
- AutomationEvent.cs
- SByte.cs
- TableRow.cs
- WebBrowserNavigatingEventHandler.cs
- Int32KeyFrameCollection.cs
- XmlRootAttribute.cs
- SimpleType.cs
- CellParaClient.cs
- Drawing.cs
- DataMisalignedException.cs
- DurationConverter.cs
- SessionKeyExpiredException.cs
- EnterpriseServicesHelper.cs
- CompilerTypeWithParams.cs
- Facet.cs
- Color.cs
- EncryptedPackageFilter.cs
- FileReservationCollection.cs
- XmlSchemas.cs
- OutputWindow.cs
- TextEditorMouse.cs
- HostExecutionContextManager.cs
- LineProperties.cs
- TableLayoutPanel.cs
- ExcludePathInfo.cs
- XmlSchemaProviderAttribute.cs
- DesignOnlyAttribute.cs
- HostedTcpTransportManager.cs
- WebRequestModulesSection.cs
- BooleanFunctions.cs
- SQLGuid.cs
- IApplicationTrustManager.cs
- SqlWorkflowPersistenceService.cs
- SecurityDocument.cs
- XmlSerializableWriter.cs
- TargetException.cs
- DiffuseMaterial.cs
- DrawingContext.cs
- FrameworkName.cs