Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartTransformerAttribute.cs / 1305376 / WebPartTransformerAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; [AttributeUsage(AttributeTargets.Class)] public sealed class WebPartTransformerAttribute : Attribute { // Cache provider and consumer types for each WebPartTransformer type. We store an array of // 2 Types (consumer, provider) indexed by transformer type. private static readonly Hashtable transformerCache = Hashtable.Synchronized(new Hashtable()); private Type _consumerType; private Type _providerType; public WebPartTransformerAttribute(Type consumerType, Type providerType) { if (consumerType == null) { throw new ArgumentNullException("consumerType"); } if (providerType == null) { throw new ArgumentNullException("providerType"); } _consumerType = consumerType; _providerType = providerType; } public Type ConsumerType { get { return _consumerType; } } public Type ProviderType { get { return _providerType; } } public static Type GetConsumerType(Type transformerType) { return GetTransformerTypes(transformerType)[0]; } public static Type GetProviderType(Type transformerType) { return GetTransformerTypes(transformerType)[1]; } ////// Returns the types a transformer can accept on its "connection points" /// private static Type[] GetTransformerTypes(Type transformerType) { if (transformerType == null) { throw new ArgumentNullException("transformerType"); } if (!transformerType.IsSubclassOf(typeof(WebPartTransformer))) { throw new InvalidOperationException( SR.GetString(SR.WebPartTransformerAttribute_NotTransformer, transformerType.FullName)); } Type[] types = (Type[])transformerCache[transformerType]; if (types == null) { types = GetTransformerTypesFromAttribute(transformerType); transformerCache[transformerType] = types; } return types; } private static Type[] GetTransformerTypesFromAttribute(Type transformerType) { Type[] types = new Type[2]; object[] attributes = transformerType.GetCustomAttributes(typeof(WebPartTransformerAttribute), true); // WebPartTransformerAttribute.AllowMultiple is false Debug.Assert(attributes.Length == 0 || attributes.Length == 1); if (attributes.Length == 1) { WebPartTransformerAttribute attribute = (WebPartTransformerAttribute)attributes[0]; if (attribute.ConsumerType == attribute.ProviderType) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerAttribute_SameTypes)); } types[0] = attribute.ConsumerType; types[1] = attribute.ProviderType; } else { throw new InvalidOperationException( SR.GetString(SR.WebPartTransformerAttribute_Missing, transformerType.FullName)); } return types; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; [AttributeUsage(AttributeTargets.Class)] public sealed class WebPartTransformerAttribute : Attribute { // Cache provider and consumer types for each WebPartTransformer type. We store an array of // 2 Types (consumer, provider) indexed by transformer type. private static readonly Hashtable transformerCache = Hashtable.Synchronized(new Hashtable()); private Type _consumerType; private Type _providerType; public WebPartTransformerAttribute(Type consumerType, Type providerType) { if (consumerType == null) { throw new ArgumentNullException("consumerType"); } if (providerType == null) { throw new ArgumentNullException("providerType"); } _consumerType = consumerType; _providerType = providerType; } public Type ConsumerType { get { return _consumerType; } } public Type ProviderType { get { return _providerType; } } public static Type GetConsumerType(Type transformerType) { return GetTransformerTypes(transformerType)[0]; } public static Type GetProviderType(Type transformerType) { return GetTransformerTypes(transformerType)[1]; } ////// Returns the types a transformer can accept on its "connection points" /// private static Type[] GetTransformerTypes(Type transformerType) { if (transformerType == null) { throw new ArgumentNullException("transformerType"); } if (!transformerType.IsSubclassOf(typeof(WebPartTransformer))) { throw new InvalidOperationException( SR.GetString(SR.WebPartTransformerAttribute_NotTransformer, transformerType.FullName)); } Type[] types = (Type[])transformerCache[transformerType]; if (types == null) { types = GetTransformerTypesFromAttribute(transformerType); transformerCache[transformerType] = types; } return types; } private static Type[] GetTransformerTypesFromAttribute(Type transformerType) { Type[] types = new Type[2]; object[] attributes = transformerType.GetCustomAttributes(typeof(WebPartTransformerAttribute), true); // WebPartTransformerAttribute.AllowMultiple is false Debug.Assert(attributes.Length == 0 || attributes.Length == 1); if (attributes.Length == 1) { WebPartTransformerAttribute attribute = (WebPartTransformerAttribute)attributes[0]; if (attribute.ConsumerType == attribute.ProviderType) { throw new InvalidOperationException(SR.GetString(SR.WebPartTransformerAttribute_SameTypes)); } types[0] = attribute.ConsumerType; types[1] = attribute.ProviderType; } else { throw new InvalidOperationException( SR.GetString(SR.WebPartTransformerAttribute_Missing, transformerType.FullName)); } return types; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
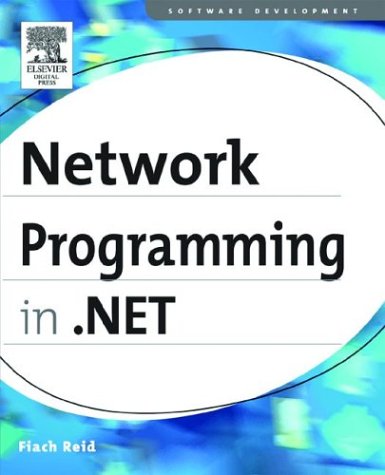
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Baml2006SchemaContext.cs
- IPGlobalProperties.cs
- RemotingConfigParser.cs
- XPathAxisIterator.cs
- SemanticAnalyzer.cs
- BindableTemplateBuilder.cs
- ConfigXmlComment.cs
- Atom10FormatterFactory.cs
- TypeForwardedToAttribute.cs
- IisTraceWebEventProvider.cs
- MessageQueue.cs
- ReadOnlyDictionary.cs
- RoutingConfiguration.cs
- sitestring.cs
- ControlHelper.cs
- Propagator.JoinPropagator.cs
- CommandValueSerializer.cs
- FileUtil.cs
- FigureHelper.cs
- OleDbRowUpdatedEvent.cs
- AuthStoreRoleProvider.cs
- XPathConvert.cs
- Helper.cs
- NavigationProgressEventArgs.cs
- RIPEMD160Managed.cs
- NativeMethods.cs
- AlternationConverter.cs
- XmlDownloadManager.cs
- MultiByteCodec.cs
- VirtualizingStackPanel.cs
- RowUpdatingEventArgs.cs
- DragSelectionMessageFilter.cs
- SqlRowUpdatedEvent.cs
- ScrollViewer.cs
- DoubleAnimationUsingKeyFrames.cs
- OleDbCommand.cs
- RegisteredDisposeScript.cs
- ACE.cs
- RangeValueProviderWrapper.cs
- FrugalMap.cs
- ServiceObjectContainer.cs
- XmlElementAttributes.cs
- ContentElement.cs
- ContainerVisual.cs
- IPPacketInformation.cs
- TextParaClient.cs
- FixedSOMTableCell.cs
- controlskin.cs
- DataGridItemCollection.cs
- ModelItem.cs
- BackStopAuthenticationModule.cs
- DefaultValueMapping.cs
- LinqDataSourceUpdateEventArgs.cs
- ResolvedKeyFrameEntry.cs
- Identifier.cs
- XmlToDatasetMap.cs
- ImageKeyConverter.cs
- EncodingDataItem.cs
- DeclarativeCatalogPart.cs
- AdjustableArrowCap.cs
- StatusBarPanel.cs
- DriveInfo.cs
- Scene3D.cs
- HtmlFormWrapper.cs
- AtomEntry.cs
- DomainUpDown.cs
- GrammarBuilderBase.cs
- AsyncDataRequest.cs
- ExtenderProvidedPropertyAttribute.cs
- HttpProxyTransportBindingElement.cs
- CharStorage.cs
- ControlType.cs
- HwndProxyElementProvider.cs
- ProcessHostServerConfig.cs
- AutomationAttributeInfo.cs
- Utils.cs
- SQLStringStorage.cs
- XmlDictionaryReaderQuotas.cs
- _SslStream.cs
- FunctionParameter.cs
- TopClause.cs
- Version.cs
- UTF7Encoding.cs
- XmlCharCheckingReader.cs
- CompilerLocalReference.cs
- PublisherIdentityPermission.cs
- DataSetMappper.cs
- SchemaElementLookUpTable.cs
- ClientEventManager.cs
- SqlConnection.cs
- UICuesEvent.cs
- GPPOINT.cs
- Image.cs
- ConfigXmlSignificantWhitespace.cs
- errorpatternmatcher.cs
- TreeViewDataItemAutomationPeer.cs
- Speller.cs
- sqlser.cs
- PropertyGridEditorPart.cs
- HttpCachePolicyElement.cs