Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / ComponentModel / Design / ServiceObjectContainer.cs / 1 / ServiceObjectContainer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using Microsoft.Win32; using System; using System.Collections; using System.Diagnostics; using System.Security.Permissions; ////// This is a simple implementation of IServiceContainer. /// [HostProtection(SharedState = true)] public class ServiceContainer : IServiceContainer, IDisposable { private Hashtable services; private IServiceProvider parentProvider; private static Type[] _defaultServices = new Type[] { typeof(IServiceContainer), typeof(ServiceContainer) }; private static TraceSwitch TRACESERVICE = new TraceSwitch("TRACESERVICE", "ServiceProvider: Trace service provider requests."); ////// Creates a new service object container. /// public ServiceContainer() { } ////// Creates a new service object container. /// public ServiceContainer(IServiceProvider parentProvider) { this.parentProvider = parentProvider; } ////// Retrieves the parent service container, or null /// if there is no parent container. /// private IServiceContainer Container { get { IServiceContainer container = null; if (parentProvider != null) { container = (IServiceContainer)parentProvider.GetService(typeof(IServiceContainer)); } return container; } } ////// This property returns the default services that are implemented directly on this IServiceContainer. /// the default implementation of this property is to return the IServiceContainer and ServiceContainer /// types. You may override this proeprty and return your own types, modifying the default behavior /// of GetService. /// protected virtual Type[] DefaultServices { get { return _defaultServices; } } ////// Our hashtable of services. The hashtable is demand /// created here. /// private Hashtable Services { get { if (services == null) { services = new Hashtable(); } return services; } } ////// Adds the given service to the service container. /// public void AddService(Type serviceType, object serviceInstance) { AddService(serviceType, serviceInstance, false); } ////// Adds the given service to the service container. /// public virtual void AddService(Type serviceType, object serviceInstance, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Adding service (instance) " + serviceType.Name + ". Promoting: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Promoting to container"); Debug.Unindent(); container.AddService(serviceType, serviceInstance, promote); return; } } // We're going to add this locally. Ensure that the service instance // is correct. // if (serviceType == null) throw new ArgumentNullException("serviceType"); if (serviceInstance == null) throw new ArgumentNullException("serviceInstance"); if (!(serviceInstance is ServiceCreatorCallback) && !serviceInstance.GetType().IsCOMObject && !serviceType.IsAssignableFrom(serviceInstance.GetType())) { throw new ArgumentException(SR.GetString(SR.ErrorInvalidServiceInstance, serviceType.FullName)); } if (Services.ContainsKey(serviceType)) { throw new ArgumentException(SR.GetString(SR.ErrorServiceExists, serviceType.FullName), "serviceType"); } Services[serviceType] = serviceInstance; } ////// Adds the given service to the service container. /// public void AddService(Type serviceType, ServiceCreatorCallback callback) { AddService(serviceType, callback, false); } ////// Adds the given service to the service container. /// public virtual void AddService(Type serviceType, ServiceCreatorCallback callback, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Adding service (callback) " + serviceType.Name + ". Promoting: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Promoting to container"); Debug.Unindent(); container.AddService(serviceType, callback, promote); return; } } // We're going to add this locally. Ensure that the service instance // is correct. // if (serviceType == null) throw new ArgumentNullException("serviceType"); if (callback == null) throw new ArgumentNullException("callback"); if (Services.ContainsKey(serviceType)) { throw new ArgumentException(SR.GetString(SR.ErrorServiceExists, serviceType.FullName), "serviceType"); } Services[serviceType] = callback; } ////// Disposes this service container. This also walks all instantiated services within the container /// and disposes any that implement IDisposable, and clears the service list. /// public void Dispose() { Dispose(true); } ////// Disposes this service container. This also walks all instantiated services within the container /// and disposes any that implement IDisposable, and clears the service list. /// protected virtual void Dispose(bool disposing) { if (disposing) { Hashtable serviceHash = services; services = null; if (serviceHash != null) { foreach(object o in serviceHash.Values) { if (o is IDisposable) { ((IDisposable)o).Dispose(); } } } } } ////// Retrieves the requested service. /// public virtual object GetService(Type serviceType) { object service = null; Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Searching for service " + serviceType.Name); Debug.Indent(); // Try locally. We first test for services we // implement and then look in our hashtable. // Type[] defaults = DefaultServices; for (int idx = 0; idx < defaults.Length; idx++) { if (serviceType == defaults[idx]) { service = this; break; } } if (service == null) { service = Services[serviceType]; } // Is the service a creator delegate? // if (service is ServiceCreatorCallback) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Encountered a callback. Invoking it"); service = ((ServiceCreatorCallback)service)(this, serviceType); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Callback return object: " + (service == null ? "(null)" : service.ToString())); if (service != null && !service.GetType().IsCOMObject && !serviceType.IsAssignableFrom(service.GetType())) { // Callback passed us a bad service. NULL it, rather than throwing an exception. // Callers here do not need to be prepared to handle bad callback implemetations. Debug.Fail("Object " + service.GetType().Name + " was returned from a service creator callback but it does not implement the registered type of " + serviceType.Name); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "**** Object does not implement service interface"); service = null; } // And replace the callback with our new service. // Services[serviceType] = service; } if (service == null && parentProvider != null) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Service unresolved. Trying parent"); service = parentProvider.GetService(serviceType); } #if DEBUG if (TRACESERVICE.TraceVerbose && service == null) { Debug.WriteLine("******************************************"); Debug.WriteLine("FAILED to resolve service " + serviceType.Name); Debug.WriteLine("AT: " + Environment.StackTrace); Debug.WriteLine("******************************************"); } #endif Debug.Unindent(); return service; } ////// Removes the given service type from the service container. /// public void RemoveService(Type serviceType) { RemoveService(serviceType, false); } ////// Removes the given service type from the service container. /// public virtual void RemoveService(Type serviceType, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Removing service: " + serviceType.Name + ", Promote: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Invoking parent container"); Debug.Unindent(); container.RemoveService(serviceType, promote); return; } } // We're going to remove this from our local list. // if (serviceType == null) throw new ArgumentNullException("serviceType"); Services.Remove(serviceType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using Microsoft.Win32; using System; using System.Collections; using System.Diagnostics; using System.Security.Permissions; ////// This is a simple implementation of IServiceContainer. /// [HostProtection(SharedState = true)] public class ServiceContainer : IServiceContainer, IDisposable { private Hashtable services; private IServiceProvider parentProvider; private static Type[] _defaultServices = new Type[] { typeof(IServiceContainer), typeof(ServiceContainer) }; private static TraceSwitch TRACESERVICE = new TraceSwitch("TRACESERVICE", "ServiceProvider: Trace service provider requests."); ////// Creates a new service object container. /// public ServiceContainer() { } ////// Creates a new service object container. /// public ServiceContainer(IServiceProvider parentProvider) { this.parentProvider = parentProvider; } ////// Retrieves the parent service container, or null /// if there is no parent container. /// private IServiceContainer Container { get { IServiceContainer container = null; if (parentProvider != null) { container = (IServiceContainer)parentProvider.GetService(typeof(IServiceContainer)); } return container; } } ////// This property returns the default services that are implemented directly on this IServiceContainer. /// the default implementation of this property is to return the IServiceContainer and ServiceContainer /// types. You may override this proeprty and return your own types, modifying the default behavior /// of GetService. /// protected virtual Type[] DefaultServices { get { return _defaultServices; } } ////// Our hashtable of services. The hashtable is demand /// created here. /// private Hashtable Services { get { if (services == null) { services = new Hashtable(); } return services; } } ////// Adds the given service to the service container. /// public void AddService(Type serviceType, object serviceInstance) { AddService(serviceType, serviceInstance, false); } ////// Adds the given service to the service container. /// public virtual void AddService(Type serviceType, object serviceInstance, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Adding service (instance) " + serviceType.Name + ". Promoting: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Promoting to container"); Debug.Unindent(); container.AddService(serviceType, serviceInstance, promote); return; } } // We're going to add this locally. Ensure that the service instance // is correct. // if (serviceType == null) throw new ArgumentNullException("serviceType"); if (serviceInstance == null) throw new ArgumentNullException("serviceInstance"); if (!(serviceInstance is ServiceCreatorCallback) && !serviceInstance.GetType().IsCOMObject && !serviceType.IsAssignableFrom(serviceInstance.GetType())) { throw new ArgumentException(SR.GetString(SR.ErrorInvalidServiceInstance, serviceType.FullName)); } if (Services.ContainsKey(serviceType)) { throw new ArgumentException(SR.GetString(SR.ErrorServiceExists, serviceType.FullName), "serviceType"); } Services[serviceType] = serviceInstance; } ////// Adds the given service to the service container. /// public void AddService(Type serviceType, ServiceCreatorCallback callback) { AddService(serviceType, callback, false); } ////// Adds the given service to the service container. /// public virtual void AddService(Type serviceType, ServiceCreatorCallback callback, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Adding service (callback) " + serviceType.Name + ". Promoting: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Promoting to container"); Debug.Unindent(); container.AddService(serviceType, callback, promote); return; } } // We're going to add this locally. Ensure that the service instance // is correct. // if (serviceType == null) throw new ArgumentNullException("serviceType"); if (callback == null) throw new ArgumentNullException("callback"); if (Services.ContainsKey(serviceType)) { throw new ArgumentException(SR.GetString(SR.ErrorServiceExists, serviceType.FullName), "serviceType"); } Services[serviceType] = callback; } ////// Disposes this service container. This also walks all instantiated services within the container /// and disposes any that implement IDisposable, and clears the service list. /// public void Dispose() { Dispose(true); } ////// Disposes this service container. This also walks all instantiated services within the container /// and disposes any that implement IDisposable, and clears the service list. /// protected virtual void Dispose(bool disposing) { if (disposing) { Hashtable serviceHash = services; services = null; if (serviceHash != null) { foreach(object o in serviceHash.Values) { if (o is IDisposable) { ((IDisposable)o).Dispose(); } } } } } ////// Retrieves the requested service. /// public virtual object GetService(Type serviceType) { object service = null; Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Searching for service " + serviceType.Name); Debug.Indent(); // Try locally. We first test for services we // implement and then look in our hashtable. // Type[] defaults = DefaultServices; for (int idx = 0; idx < defaults.Length; idx++) { if (serviceType == defaults[idx]) { service = this; break; } } if (service == null) { service = Services[serviceType]; } // Is the service a creator delegate? // if (service is ServiceCreatorCallback) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Encountered a callback. Invoking it"); service = ((ServiceCreatorCallback)service)(this, serviceType); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Callback return object: " + (service == null ? "(null)" : service.ToString())); if (service != null && !service.GetType().IsCOMObject && !serviceType.IsAssignableFrom(service.GetType())) { // Callback passed us a bad service. NULL it, rather than throwing an exception. // Callers here do not need to be prepared to handle bad callback implemetations. Debug.Fail("Object " + service.GetType().Name + " was returned from a service creator callback but it does not implement the registered type of " + serviceType.Name); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "**** Object does not implement service interface"); service = null; } // And replace the callback with our new service. // Services[serviceType] = service; } if (service == null && parentProvider != null) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Service unresolved. Trying parent"); service = parentProvider.GetService(serviceType); } #if DEBUG if (TRACESERVICE.TraceVerbose && service == null) { Debug.WriteLine("******************************************"); Debug.WriteLine("FAILED to resolve service " + serviceType.Name); Debug.WriteLine("AT: " + Environment.StackTrace); Debug.WriteLine("******************************************"); } #endif Debug.Unindent(); return service; } ////// Removes the given service type from the service container. /// public void RemoveService(Type serviceType) { RemoveService(serviceType, false); } ////// Removes the given service type from the service container. /// public virtual void RemoveService(Type serviceType, bool promote) { Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Removing service: " + serviceType.Name + ", Promote: " + promote.ToString()); if (promote) { IServiceContainer container = Container; if (container != null) { Debug.Indent(); Debug.WriteLineIf(TRACESERVICE.TraceVerbose, "Invoking parent container"); Debug.Unindent(); container.RemoveService(serviceType, promote); return; } } // We're going to remove this from our local list. // if (serviceType == null) throw new ArgumentNullException("serviceType"); Services.Remove(serviceType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
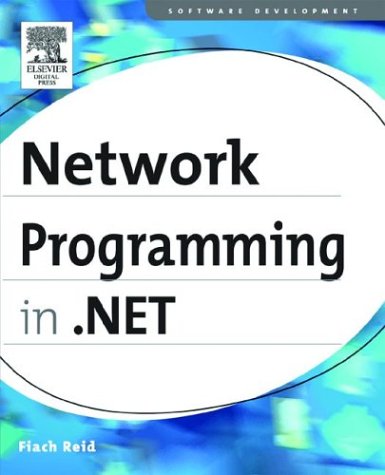
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Lease.cs
- EntityDataSourceDataSelection.cs
- Trace.cs
- XmlSchemaIdentityConstraint.cs
- RsaSecurityToken.cs
- Identifier.cs
- Walker.cs
- DataTransferEventArgs.cs
- NetTcpBindingCollectionElement.cs
- OLEDB_Util.cs
- SecureEnvironment.cs
- SqlDependencyUtils.cs
- XPathNavigatorKeyComparer.cs
- SplitterDesigner.cs
- ListItemsPage.cs
- DataGridViewDataErrorEventArgs.cs
- XmlTextReader.cs
- Typography.cs
- TableDetailsCollection.cs
- OutputCacheSettingsSection.cs
- StateWorkerRequest.cs
- CmsUtils.cs
- ChildDocumentBlock.cs
- BasicKeyConstraint.cs
- SafePEFileHandle.cs
- ScrollBar.cs
- Base64Stream.cs
- IgnoreFlushAndCloseStream.cs
- FixedHighlight.cs
- _OverlappedAsyncResult.cs
- BufferCache.cs
- TypeInformation.cs
- DesignerObjectListAdapter.cs
- WebRequest.cs
- PaintValueEventArgs.cs
- HtmlEncodedRawTextWriter.cs
- Root.cs
- DispatcherHooks.cs
- HttpBindingExtension.cs
- ListViewItem.cs
- RootBuilder.cs
- Schema.cs
- DocumentPageHost.cs
- DataGridCellItemAutomationPeer.cs
- DbUpdateCommandTree.cs
- DrawingAttributeSerializer.cs
- EmptyImpersonationContext.cs
- UnionExpr.cs
- ResourceAssociationTypeEnd.cs
- X500Name.cs
- InlineCollection.cs
- xmlsaver.cs
- TextTreeInsertUndoUnit.cs
- CreateUserWizard.cs
- RequestUriProcessor.cs
- ChtmlPhoneCallAdapter.cs
- ApplicationContext.cs
- HealthMonitoringSectionHelper.cs
- BamlVersionHeader.cs
- CertificateManager.cs
- ButtonFlatAdapter.cs
- _SslState.cs
- CompilerParameters.cs
- SymLanguageVendor.cs
- File.cs
- SettingsPropertyValueCollection.cs
- MessagePropertyFilter.cs
- TreeBuilderBamlTranslator.cs
- Events.cs
- CurrentTimeZone.cs
- ISAPIWorkerRequest.cs
- FolderNameEditor.cs
- DictionaryEntry.cs
- BridgeDataRecord.cs
- SafeIUnknown.cs
- XmlLoader.cs
- ZipIOLocalFileBlock.cs
- TabItem.cs
- CompareInfo.cs
- OracleParameter.cs
- PageBreakRecord.cs
- Activator.cs
- PathGeometry.cs
- DecoderFallback.cs
- XhtmlBasicPageAdapter.cs
- SHA384Managed.cs
- TextServicesHost.cs
- Stroke.cs
- ListenerConstants.cs
- Matrix3D.cs
- SoapServerMessage.cs
- ProviderConnectionPointCollection.cs
- ImageList.cs
- DesignerDeviceConfig.cs
- VirtualDirectoryMappingCollection.cs
- SignatureTargetIdManager.cs
- AlphabetConverter.cs
- HttpRuntimeSection.cs
- ArraySubsetEnumerator.cs
- DataGridViewCellStyle.cs