Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / commandenforcer.cs / 1 / commandenforcer.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Manager for Enforcing RM Permissions on DocumentApplication-specific RoutedUICommands. // // History: // 06/24/2005 - [....] created //--------------------------------------------------------------------------- using MS.Internal.Documents; using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows.Input; using System.Windows.TrustUI; namespace MS.Internal.Documents.Application { ////// A PolicyBinding represents a binding between a Command and the RMPolicy required to /// Invoke said command. /// internal sealed class PolicyBinding { ////// Constructor for a PolicyBinding. /// /// The Command to bind the permission to. /// The RMPolicy to be bound to the above Command. public PolicyBinding(RoutedUICommand command, RightsManagementPolicy policy) { if (command == null) { throw new ArgumentNullException("command"); } _command = command; _policy = policy; } ////// The Command associated with this binding. /// public RoutedUICommand Command { get { return _command; } } ////// The RMPolicy associated with this binding. /// public RightsManagementPolicy Policy { get { return _policy; } } private RoutedUICommand _command; private RightsManagementPolicy _policy; } ////// The CommandEnforcer class provides a very simple central repository for RM enforced /// Commands used by Mongoose. /// internal sealed class CommandEnforcer { ////// Constructor /// /// The DocumentApplicationDocumentViewer parent. ////// Critical /// 1) Modifies the list of bindings. /// TreatAsSafe /// 1) The list is set to a safe value, an empty list. /// [SecurityCritical, SecurityTreatAsSafe] internal CommandEnforcer(DocumentApplicationDocumentViewer docViewer) { //Create our bindings List. _bindings = new List(_initialBindCount); _docViewer = docViewer; } /// /// Finalizer /// ////// Critical - Calls DisablePrintScreen to modify system PrintScreen access. /// TreatAsSafe - Calls to re-enable the system PrintScreen functionality /// and does not require any external information. /// [SecurityCritical, SecurityTreatAsSafe] ~CommandEnforcer() { // Ensure that PrintScreen is re-enabled in native code DisablePrintScreen(false); } ////// Adds a new binding to the Enforcer. /// NOTE: CommandEnforcer does not check for duplicate entries, so be warned /// that if you have duplicate entries, the newest duplicate will be the one whose /// enforcement is honored. /// /// The binding to add ////// Critical /// 1) Modifies the list of bindings. /// NotSafe /// 1) This can be used to "shadow" existing bindings and thus elevate privilege. /// [SecurityCritical] internal void AddBinding(PolicyBinding bind) { if (bind == null) { throw new ArgumentNullException("bind"); } _bindings.Add(bind); } ////// Enforces the RMPolicy by walking through the PolicyBinding and setting the /// IsBlockedByRM property on the associated Commands as appropriate. /// /// ////// Critical /// 1) Modifies access to all commands (to set the IsBlockedByRM property). /// 2) Accesses the list of bindings. /// 3) Calls DisablePrintScreen to modify system PrintScreen access /// TreatAsSafe /// 1) The policyToEnforce is acquired from the parent DocumentApplicationDocumentViewer /// through safe means. /// 2) The list of bindings is not modified; reading the list is defined as a safe /// operation. /// [SecurityCritical, SecurityTreatAsSafe] internal void Enforce() { Invariant.Assert( _docViewer != null, "CommandEnforcer has no reference to the parent DocumentApplicationDocumentViewer."); // Get the current policy from the parent DocumentApplicationDocumentViewer RightsManagementPolicy policyToEnforce = _docViewer.RightsManagementPolicy; // Walk through the list of bindings foreach (PolicyBinding binding in _bindings) { // If the incoming policy allows this action, and it completely masks our binding policy, // then we set the IsBlockedByRM property to false, as this Command is permitted. binding.Command.IsBlockedByRM = !((policyToEnforce & binding.Policy) == binding.Policy); } // Enforce PrintScreen // This will disable PrintScreen if neither AllowCopy or AllowPrint // permission is granted. bool disablePrintScreen = !(((policyToEnforce & RightsManagementPolicy.AllowCopy) == RightsManagementPolicy.AllowCopy) || ((policyToEnforce & RightsManagementPolicy.AllowPrint) == RightsManagementPolicy.AllowPrint)); DisablePrintScreen(disablePrintScreen); } ////// Disable all PrintScreen related functions in the system. /// /// True if PrintScreen should be disabled, false otherwise. ////// Critical - Calls DRMRegisterContent which is an unsafe native method using /// the value of the parameter. /// - Sets and uses _isRMMissing and _printScreenDisabled /// [SecurityCritical] private void DisablePrintScreen(bool disable) { // To ensure that everytime PrintScreen is disabled it is also re-enabled we // only issue the system call if the value has changed. if (disable != _printScreenDisabled) { // Check if currently running in 32bit and that RM is available. if ((!_isRMMissing) && (IntPtr.Size == 4)) { // Initialize hresult to a valid return value. int hresult = UnsafeNativeMethods.FAIL; try { // Make call to RM API here to change the count. hresult = UnsafeNativeMethods.DRMRegisterContent(disable); } catch (System.DllNotFoundException) { // We could not find MSDRM.DLL to disable the print screen. // Just silently catch this exception and fail. // This is safe to catch, because it means that RM is not // installed on the machine so we don't need to worry about // disabling the print screen. _isRMMissing = true; } // Result should be S_OK for successful value assignment. Trace.SafeWrite( Trace.Presentation, "CommandEnforcer: DisablePrintScreen change to: {0} hresult: {1}", disable, hresult); } // Update property with new value _printScreenDisabled = disable; } } ////// The list of bindings to enforce. /// ////// Critical /// 1) Modifying the bindings to enforce is a critical operation. Note that reading from /// the list is considered safe; it is only adding or removing bindings that is unsafe. /// [SecurityCritical] private List_bindings; private DocumentApplicationDocumentViewer _docViewer; private const int _initialBindCount = 10; //We allocate space for 10 bindings initially. /// /// _printScreenDisabled is used to track the current PrintScreen state. It begins with /// false since PrintScreen is initially enabled, and tracks changes from there. /// ////// Critical - Maintains a record of the current state of native PrintScreen. /// [SecurityCritical] private bool _printScreenDisabled; // Trusted to be initialized to false ////// _isRMMissing is used to track if a successful call has been made to native RM. /// This is used for performance, to limit the number of Exceptions being caught. /// ////// Critical - Maintains a record of whether RM is available and working on the system. /// [SecurityCritical] private bool _isRMMissing; // Initially assumed that RM is installed and working. #region UnsafeNativeMethods (Private Class) ////// This class is used to provide access to unmanaged code in order to disable /// the native PrintScreen support. /// private static class UnsafeNativeMethods { ////// Called to toggle native PrintScreen support. This will increment/decrement /// a reference counter, so it must be reset after use (for stability). /// /// A bool value to determine if PrintScreen should be /// disabled. True means disabled, false re-enables. ///S_OK on success, an error code otherwise. ////// Critical - The call to DRMRegisterContent has SuppressUnmanagedCodeSecurity set. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("msdrm.dll", SetLastError = false, CharSet = CharSet.Unicode, CallingConvention = CallingConvention.StdCall)] public static extern int DRMRegisterContent(bool register); ////// Non-success value. /// // This value can be anything other than S_OK internal const int FAIL = -1; } #endregion UnsafeNativeMethods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
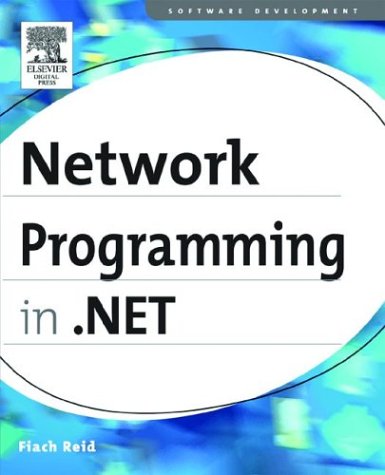
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridCommandEventArgs.cs
- EntityDataSourceChangingEventArgs.cs
- ListBoxChrome.cs
- OptionalColumn.cs
- EmptyStringExpandableObjectConverter.cs
- TextEditorCharacters.cs
- WinFormsSpinner.cs
- RelatedView.cs
- AbstractDataSvcMapFileLoader.cs
- PreviewKeyDownEventArgs.cs
- EventProviderWriter.cs
- FormViewPageEventArgs.cs
- ProcessInfo.cs
- MimePart.cs
- RecommendedAsConfigurableAttribute.cs
- coordinatorscratchpad.cs
- LeafCellTreeNode.cs
- ContextMenuStripActionList.cs
- BufferAllocator.cs
- MobileFormsAuthentication.cs
- ImageButton.cs
- DataGridItemCollection.cs
- ValidatorCompatibilityHelper.cs
- ToolStripLabel.cs
- Rules.cs
- SplitterEvent.cs
- DataGridViewAccessibleObject.cs
- CodeRegionDirective.cs
- TransformPattern.cs
- BinaryMessageEncodingElement.cs
- AccessibilityHelperForVista.cs
- StructuralCache.cs
- BuilderInfo.cs
- SolidBrush.cs
- ContentElementAutomationPeer.cs
- ProxyGenerationError.cs
- NativeMethods.cs
- AutomationElementIdentifiers.cs
- DefaultPropertyAttribute.cs
- TiffBitmapDecoder.cs
- EventSetterHandlerConverter.cs
- MessageProperties.cs
- TextMessageEncoder.cs
- Panel.cs
- UrlMapping.cs
- SecUtil.cs
- SEHException.cs
- AssignDesigner.xaml.cs
- ISessionStateStore.cs
- WpfPayload.cs
- AlignmentXValidation.cs
- SocketPermission.cs
- CalendarDay.cs
- WorkItem.cs
- PropertyMetadata.cs
- mansign.cs
- SecurityBindingElementImporter.cs
- ConstantSlot.cs
- ObjectContextServiceProvider.cs
- Crypto.cs
- BamlLocalizabilityResolver.cs
- RequestDescription.cs
- CompletionCallbackWrapper.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- ColumnWidthChangedEvent.cs
- DateTimeFormatInfo.cs
- DefaultPrintController.cs
- AliasedSlot.cs
- WindowPattern.cs
- MenuStrip.cs
- ExternalFile.cs
- Brush.cs
- RepeatInfo.cs
- ConfigXmlCDataSection.cs
- ConstraintStruct.cs
- QilSortKey.cs
- TreeViewImageIndexConverter.cs
- Geometry.cs
- PassportAuthenticationModule.cs
- AssemblyBuilderData.cs
- DesignerForm.cs
- FlowSwitchDesigner.xaml.cs
- SqlClientWrapperSmiStream.cs
- ProcessExitedException.cs
- LinqDataSourceDisposeEventArgs.cs
- Calendar.cs
- ConcatQueryOperator.cs
- SettingsProviderCollection.cs
- CannotUnloadAppDomainException.cs
- UIElement3DAutomationPeer.cs
- FilePrompt.cs
- ComponentManagerBroker.cs
- Region.cs
- TextWriterTraceListener.cs
- BaseAutoFormat.cs
- DocumentSequenceHighlightLayer.cs
- ReadContentAsBinaryHelper.cs
- SqlInfoMessageEvent.cs
- MaxValueConverter.cs
- MemoryResponseElement.cs