Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / Protocols / SoapMessage.cs / 1305376 / SoapMessage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Protocols { using System.Web.Services; using System.Xml.Serialization; using System; using System.Reflection; using System.Collections; using System.IO; using System.ComponentModel; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Diagnostics; using System.Web.Services.Diagnostics; ////// /// [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] public abstract class SoapMessage { SoapMessageStage stage; SoapHeaderCollection headers = new SoapHeaderCollection(); Stream stream; SoapExtensionStream extensionStream; string contentType; string contentEncoding; object[] parameterValues; SoapException exception; internal SoapMessage() { } internal void SetParameterValues(object[] parameterValues) { this.parameterValues = parameterValues; } internal object[] GetParameterValues() { return parameterValues; } ///[To be supplied.] ////// /// public abstract bool OneWay { get; } ///[To be supplied.] ////// /// public object GetInParameterValue(int index) { EnsureInStage(); EnsureNoException(); if (index < 0 || index >= parameterValues.Length) throw new IndexOutOfRangeException(Res.GetString(Res.indexMustBeBetweenAnd0Inclusive, parameterValues.Length)); return parameterValues[index]; } ///[To be supplied.] ////// /// public object GetOutParameterValue(int index) { EnsureOutStage(); EnsureNoException(); if (!MethodInfo.IsVoid) { if (index == int.MaxValue) throw new IndexOutOfRangeException(Res.GetString(Res.indexMustBeBetweenAnd0Inclusive, parameterValues.Length)); index++; } if (index < 0 || index >= parameterValues.Length) throw new IndexOutOfRangeException(Res.GetString(Res.indexMustBeBetweenAnd0Inclusive, parameterValues.Length)); return parameterValues[index]; } ///[To be supplied.] ////// /// public object GetReturnValue() { EnsureOutStage(); EnsureNoException(); if (MethodInfo.IsVoid) throw new InvalidOperationException(Res.GetString(Res.WebNoReturnValue)); return parameterValues[0]; } ///[To be supplied.] ////// /// protected abstract void EnsureOutStage(); ///[To be supplied.] ////// /// protected abstract void EnsureInStage(); void EnsureNoException() { if (exception != null) throw new InvalidOperationException(Res.GetString(Res.WebCannotAccessValue), exception); } ///[To be supplied.] ////// /// public SoapException Exception { get { return exception; } set { exception = value; } } ///[To be supplied.] ////// /// public abstract LogicalMethodInfo MethodInfo { get; } /* internal abstract SoapReflectedExtension[] Extensions { get; } internal abstract object[] ExtensionInitializers { get; } */ ///[To be supplied.] ////// /// protected void EnsureStage(SoapMessageStage stage) { if ((this.stage & stage) == 0) throw new InvalidOperationException(Res.GetString(Res.WebCannotAccessValueStage, this.stage.ToString())); } ///[To be supplied.] ////// /// public SoapHeaderCollection Headers { get { return headers; } } internal void SetStream(Stream stream) { if (extensionStream != null) { extensionStream.SetInnerStream(stream); extensionStream.SetStreamReady(); // The extension stream should now be referenced by either this.stream // or an extension that has chained it to another stream. extensionStream = null; } else this.stream = stream; } internal void SetExtensionStream(SoapExtensionStream extensionStream) { this.extensionStream = extensionStream; this.stream = extensionStream; } ///[To be supplied.] ////// /// public Stream Stream { get { return stream; } } ///[To be supplied.] ////// /// public string ContentType { get { EnsureStage(SoapMessageStage.BeforeSerialize | SoapMessageStage.BeforeDeserialize); return contentType; } set { EnsureStage(SoapMessageStage.BeforeSerialize | SoapMessageStage.BeforeDeserialize); contentType = value; } } ///[To be supplied.] ///public string ContentEncoding { get { EnsureStage(SoapMessageStage.BeforeSerialize | SoapMessageStage.BeforeDeserialize); return contentEncoding; } set { EnsureStage(SoapMessageStage.BeforeSerialize | SoapMessageStage.BeforeDeserialize); contentEncoding = value; } } /// /// /// public SoapMessageStage Stage { get { return stage; } } internal void SetStage(SoapMessageStage stage) { this.stage = stage; } ///[To be supplied.] ////// /// public abstract string Url { get; } ///[To be supplied.] ////// /// public abstract string Action { get; } ///[To be supplied.] ///[ComVisible(false)] [DefaultValue(SoapProtocolVersion.Default)] public virtual SoapProtocolVersion SoapVersion { get { return SoapProtocolVersion.Default; } } internal static SoapExtension[] InitializeExtensions(SoapReflectedExtension[] reflectedExtensions, object[] extensionInitializers) { if (reflectedExtensions == null) return null; SoapExtension[] extensions = new SoapExtension[reflectedExtensions.Length]; for (int i = 0; i < extensions.Length; i++) { extensions[i] = reflectedExtensions[i].CreateInstance(extensionInitializers[i]); } return extensions; } internal void InitExtensionStreamChain(SoapExtension[] extensions) { if (extensions == null) return; for (int i = 0; i < extensions.Length; i++) { stream = extensions[i].ChainStream(stream); } } internal void RunExtensions(SoapExtension[] extensions, bool throwOnException) { if (extensions == null) return; TraceMethod caller = Tracing.On ? new TraceMethod(this, "RunExtensions", extensions, throwOnException) : null; // Higher priority extensions (earlier in the list) run earlier for deserialization stages, // and later for serialization stages if ((stage & (SoapMessageStage.BeforeDeserialize | SoapMessageStage.AfterDeserialize)) != 0) { for (int i = 0; i < extensions.Length; i++) { if (Tracing.On) Tracing.Enter("SoapExtension", caller, new TraceMethod(extensions[i], "ProcessMessage", stage)); extensions[i].ProcessMessage(this); if (Tracing.On) Tracing.Exit("SoapExtension", caller); if (Exception != null) { if (throwOnException) throw Exception; if (Tracing.On) Tracing.ExceptionIgnore(TraceEventType.Warning, caller, Exception); } } } else { for (int i = extensions.Length - 1; i >= 0; i--) { if (Tracing.On) Tracing.Enter("SoapExtension", caller, new TraceMethod(extensions[i], "ProcessMessage", stage)); extensions[i].ProcessMessage(this); if (Tracing.On) Tracing.Exit("SoapExtension", caller); if (Exception != null) { if (throwOnException) throw Exception; if (Tracing.On) Tracing.ExceptionIgnore(TraceEventType.Warning, caller, Exception); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
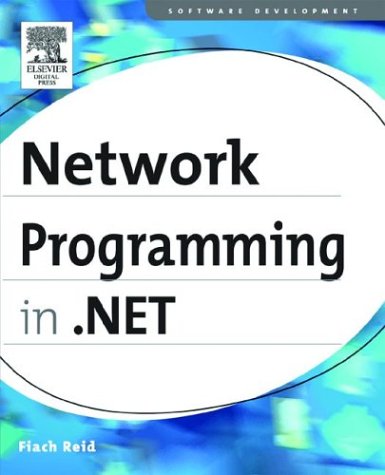
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuleInfoComparer.cs
- ControlBuilder.cs
- DetailsViewInsertedEventArgs.cs
- CqlQuery.cs
- WindowsListViewItemCheckBox.cs
- MsmqIntegrationOutputChannel.cs
- HtmlElementCollection.cs
- AppSettingsExpressionBuilder.cs
- ReadWriteSpinLock.cs
- Registration.cs
- TextTreePropertyUndoUnit.cs
- CellParagraph.cs
- XPathEmptyIterator.cs
- BaseParaClient.cs
- CodeNamespace.cs
- SoapWriter.cs
- XmlCustomFormatter.cs
- XmlSchemaObjectCollection.cs
- AsymmetricSignatureDeformatter.cs
- XMLDiffLoader.cs
- DesignRelationCollection.cs
- FlowDocumentFormatter.cs
- RoleBoolean.cs
- SHA512.cs
- TypedDatasetGenerator.cs
- SmtpSection.cs
- UrlMappingsSection.cs
- UrlPath.cs
- ZoneIdentityPermission.cs
- TableItemProviderWrapper.cs
- tooltip.cs
- XsltConvert.cs
- JulianCalendar.cs
- DrawingContextDrawingContextWalker.cs
- UpdatableGenericsFeature.cs
- FillRuleValidation.cs
- DataGridDesigner.cs
- XamlStyleSerializer.cs
- Logging.cs
- TerminatorSinks.cs
- MultipleViewProviderWrapper.cs
- DesignTimeTemplateParser.cs
- ScriptControlDescriptor.cs
- SafeNativeMethods.cs
- InputMethodStateChangeEventArgs.cs
- ObfuscationAttribute.cs
- ExtensionFile.cs
- CorrelationKey.cs
- InstanceContextManager.cs
- contentDescriptor.cs
- RelationshipConverter.cs
- AuthenticatedStream.cs
- Int32Collection.cs
- LocatorGroup.cs
- UpdateProgress.cs
- Int32Animation.cs
- SchemeSettingElementCollection.cs
- DateTimeValueSerializer.cs
- BasicKeyConstraint.cs
- WeakEventTable.cs
- UndirectedGraph.cs
- Shape.cs
- JsonStringDataContract.cs
- OracleColumn.cs
- TextEndOfLine.cs
- IApplicationTrustManager.cs
- TreeBuilder.cs
- QueryExtender.cs
- WithStatement.cs
- LinqDataSourceStatusEventArgs.cs
- TransformDescriptor.cs
- RegexCode.cs
- ControlBindingsCollection.cs
- XamlInt32CollectionSerializer.cs
- xdrvalidator.cs
- DefaultMergeHelper.cs
- HuffModule.cs
- _ConnectOverlappedAsyncResult.cs
- SocketException.cs
- File.cs
- RoutedCommand.cs
- AssemblySettingAttributes.cs
- XmlWellformedWriter.cs
- SizeF.cs
- Assert.cs
- DbConnectionFactory.cs
- ConfigXmlText.cs
- CommonObjectSecurity.cs
- IPipelineRuntime.cs
- XmlSchemaSequence.cs
- HtmlTable.cs
- DispatcherProcessingDisabled.cs
- WindowsListViewItemCheckBox.cs
- StringArrayConverter.cs
- Path.cs
- GeneralTransform2DTo3D.cs
- ObjectListFieldsPage.cs
- ConstructorBuilder.cs
- Rotation3DAnimationBase.cs
- DbMetaDataFactory.cs