Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Actions / CallSiteOps.cs / 1305376 / CallSiteOps.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.ComponentModel; using System.Diagnostics; using System.Dynamic; using System.Linq.Expressions; using System.Collections.Generic; namespace System.Runtime.CompilerServices { // Conceptually these are instance methods on CallSitebut // we don't want users to see them /// /// This API supports the .NET Framework infrastructure and is not intended to be used directly from your code. /// [EditorBrowsable(EditorBrowsableState.Never), DebuggerStepThrough] public static class CallSiteOps { ////// Creates an instance of a dynamic call site used for cache lookup. /// ///The type of the delegate of the ///. The new call site. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static CallSiteCreateMatchmaker (CallSite site) where T : class { var mm = site.CreateMatchMaker(); CallSiteOps.ClearMatch(mm); return mm; } /// /// Checks if a dynamic site requires an update. /// /// An instance of the dynamic call site. ///true if rule does not need updating, false otherwise. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static bool SetNotMatched(CallSite site) { var res = site._match; site._match = false; //avoid branch here to make sure the method is inlined return res; } ////// Checks whether the executed rule matched /// /// An instance of the dynamic call site. ///true if rule matched, false otherwise. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static bool GetMatch(CallSite site) { return site._match; } ////// Clears the match flag on the matchmaker call site. /// /// An instance of the dynamic call site. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static void ClearMatch(CallSite site) { site._match = true; } ////// Adds a rule to the cache maintained on the dynamic call site. /// ///The type of the delegate of the /// An instance of the dynamic call site. /// An instance of the call site rule. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static void AddRule. (CallSite site, T rule) where T : class { site.AddRule(rule); } /// /// Updates rules in the cache. /// ///The type of the delegate of the /// An instance of the dynamic call site. /// The matched rule index. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static void UpdateRules. (CallSite @this, int matched) where T : class { if (matched > 1) { @this.MoveRule(matched); } } /// /// Gets the dynamic binding rules from the call site. /// ///The type of the delegate of the /// An instance of the dynamic call site. ///. An array of dynamic binding rules. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static T[] GetRules(CallSite site) where T : class { return site.Rules; } /// /// Retrieves binding rule cache. /// ///The type of the delegate of the /// An instance of the dynamic call site. ///. The cache. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static RuleCacheGetRuleCache (CallSite site) where T : class { return site.Binder.GetRuleCache (); } /// /// Moves the binding rule within the cache. /// ///The type of the delegate of the /// The call site rule cache. /// An instance of the call site rule. /// An index of the call site rule. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static void MoveRule. (RuleCache cache, T rule, int i) where T : class { if (i > 1) { cache.MoveRule(rule, i); } } /// /// Searches the dynamic rule cache for rules applicable to the dynamic operation. /// ///The type of the delegate of the /// The cache. ///. The collection of applicable rules. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static T[] GetCachedRules(RuleCache cache) where T : class { return cache.GetRules(); } /// /// Updates the call site target with a new rule based on the arguments. /// ///The type of the delegate of the /// The call site binder. /// An instance of the dynamic call site. /// Arguments to the call site. ///. The new call site target. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static T Bind(CallSiteBinder binder, CallSite site, object[] args) where T : class { return binder.BindCore(site, args); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
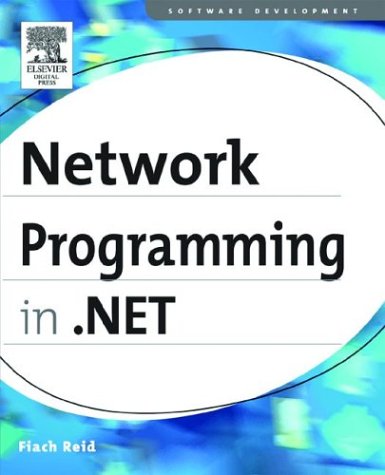
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataObjectEventArgs.cs
- TableMethodGenerator.cs
- ServiceDebugBehavior.cs
- Nullable.cs
- sitestring.cs
- EventLogPermissionAttribute.cs
- WindowsListViewGroupSubsetLink.cs
- RuleSetBrowserDialog.cs
- RSAPKCS1SignatureFormatter.cs
- Visitor.cs
- BitmapDecoder.cs
- MapPathBasedVirtualPathProvider.cs
- DbQueryCommandTree.cs
- DeriveBytes.cs
- MexTcpBindingCollectionElement.cs
- ElementHostAutomationPeer.cs
- Drawing.cs
- _SecureChannel.cs
- _HeaderInfo.cs
- DecimalStorage.cs
- NativeMethods.cs
- MarkupObject.cs
- GeometryConverter.cs
- LayoutEngine.cs
- MethodCallTranslator.cs
- TextTreeRootTextBlock.cs
- CorrelationManager.cs
- FontUnit.cs
- Figure.cs
- ResizeBehavior.cs
- ApplicationSecurityInfo.cs
- HttpServerVarsCollection.cs
- WebBrowsableAttribute.cs
- BrowserTree.cs
- TdsRecordBufferSetter.cs
- CAGDesigner.cs
- XslCompiledTransform.cs
- TogglePattern.cs
- PrinterResolution.cs
- XmlSchemaSimpleContentExtension.cs
- SpinWait.cs
- SiteMapSection.cs
- DragEventArgs.cs
- HashCodeCombiner.cs
- SystemUdpStatistics.cs
- ColorConvertedBitmapExtension.cs
- Bookmark.cs
- TransactionsSectionGroup.cs
- WebPartEventArgs.cs
- MimeBasePart.cs
- FixedHighlight.cs
- DefaultValueTypeConverter.cs
- LocalizationComments.cs
- FormViewPagerRow.cs
- EmbeddedMailObjectsCollection.cs
- LoginView.cs
- IpcManager.cs
- MultiAsyncResult.cs
- TriggerBase.cs
- SapiAttributeParser.cs
- Compilation.cs
- TypeUsage.cs
- InputLanguageCollection.cs
- LocalizedNameDescriptionPair.cs
- SecuritySessionSecurityTokenProvider.cs
- SecurityHeaderElementInferenceEngine.cs
- AspCompat.cs
- CodeBinaryOperatorExpression.cs
- VectorCollection.cs
- cookiecollection.cs
- PropertyEmitterBase.cs
- PaintEvent.cs
- ColorContextHelper.cs
- FontUnitConverter.cs
- AssemblyUtil.cs
- FacetValueContainer.cs
- ACE.cs
- DataSetViewSchema.cs
- DataGridTablesFactory.cs
- SocketInformation.cs
- DeviceContexts.cs
- ResourceContainerWrapper.cs
- BindingElement.cs
- ErrorFormatterPage.cs
- FormViewModeEventArgs.cs
- VectorAnimation.cs
- RegexRunner.cs
- ColumnCollection.cs
- QueryPrefixOp.cs
- XmlSchemaAttribute.cs
- ValidateNames.cs
- TextBoxBase.cs
- TextElement.cs
- ReplyAdapterChannelListener.cs
- WebConfigManager.cs
- CssStyleCollection.cs
- EffectiveValueEntry.cs
- XmlTextReaderImpl.cs
- ImageKeyConverter.cs
- DataViewListener.cs