Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / SqlClient / TdsRecordBufferSetter.cs / 1 / TdsRecordBufferSetter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using Microsoft.SqlServer.Server; using System; using System.Data; using System.Data.SqlClient; using System.Diagnostics; using System.Data.SqlTypes; // TdsRecordBufferSetter handles writing a structured value out to a TDS stream internal class TdsRecordBufferSetter : SmiRecordBuffer { #region Fields (private) private TdsValueSetter[] _fieldSetters; // setters for individual fields private TdsParserStateObject _stateObj; // target to write to private SmiMetaData _metaData; // metadata describing value #if DEBUG private const int ReadyForToken = -1; // must call new/end element next private const int EndElementsCalled = -2; // already called EndElements, can only call Close private const int Closed = -3; // closed (zombied) private int _currentField; // validate that caller sets columns in correct order. #endif #endregion #region Exposed Construct and control methods/properties internal TdsRecordBufferSetter(TdsParserStateObject stateObj, SmiMetaData md) { Debug.Assert(SqlDbType.Structured == md.SqlDbType, "Unsupported SqlDbType: " + md.SqlDbType); _fieldSetters = new TdsValueSetter[md.FieldMetaData.Count]; for(int i=0; i_currentField, "_currentField out of range for setting a column:" + _currentField); Debug.Assert(ordinal == _currentField, "Setter called out of order. Should be " + _currentField + ", but was " + ordinal); // Must not write to field with a DefaultFieldsProperty set to true Debug.Assert(!((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[ordinal], "Attempt to write to a default-valued field: " + ordinal); #endif } // Handle logic of skipping default columns [Conditional("DEBUG")] private void SkipPossibleDefaultedColumns(int targetColumn) { #if DEBUG Debug.Assert(targetColumn < _metaData.FieldMetaData.Count && targetColumn >= ReadyForToken, "TdsRecordBufferSetter.SkipPossibleDefaultedColumns: Invalid target column: " + targetColumn); // special setup for ReadyForToken as the target if (targetColumn == ReadyForToken) { if (ReadyForToken == _currentField) { return; } // Handle readyfortoken by using count of columns in the loop. targetColumn = _metaData.FieldMetaData.Count; } // Handle skipping default-valued fields while (targetColumn > _currentField) { // All intermediate fields must be default fields (i.e. have a "true" entry in SmiDefaultFieldsProperty Debug.Assert(((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[_currentField], "Skipping a field that was not default: " + _currentField); _currentField++; } if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } [Conditional("DEBUG")] internal void CheckSettingColumn(int ordinal) { #if DEBUG // Make sure target column can be written to. CheckWritingToColumn(ordinal); _currentField++; if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using Microsoft.SqlServer.Server; using System; using System.Data; using System.Data.SqlClient; using System.Diagnostics; using System.Data.SqlTypes; // TdsRecordBufferSetter handles writing a structured value out to a TDS stream internal class TdsRecordBufferSetter : SmiRecordBuffer { #region Fields (private) private TdsValueSetter[] _fieldSetters; // setters for individual fields private TdsParserStateObject _stateObj; // target to write to private SmiMetaData _metaData; // metadata describing value #if DEBUG private const int ReadyForToken = -1; // must call new/end element next private const int EndElementsCalled = -2; // already called EndElements, can only call Close private const int Closed = -3; // closed (zombied) private int _currentField; // validate that caller sets columns in correct order. #endif #endregion #region Exposed Construct and control methods/properties internal TdsRecordBufferSetter(TdsParserStateObject stateObj, SmiMetaData md) { Debug.Assert(SqlDbType.Structured == md.SqlDbType, "Unsupported SqlDbType: " + md.SqlDbType); _fieldSetters = new TdsValueSetter[md.FieldMetaData.Count]; for(int i=0; i_currentField, "_currentField out of range for setting a column:" + _currentField); Debug.Assert(ordinal == _currentField, "Setter called out of order. Should be " + _currentField + ", but was " + ordinal); // Must not write to field with a DefaultFieldsProperty set to true Debug.Assert(!((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[ordinal], "Attempt to write to a default-valued field: " + ordinal); #endif } // Handle logic of skipping default columns [Conditional("DEBUG")] private void SkipPossibleDefaultedColumns(int targetColumn) { #if DEBUG Debug.Assert(targetColumn < _metaData.FieldMetaData.Count && targetColumn >= ReadyForToken, "TdsRecordBufferSetter.SkipPossibleDefaultedColumns: Invalid target column: " + targetColumn); // special setup for ReadyForToken as the target if (targetColumn == ReadyForToken) { if (ReadyForToken == _currentField) { return; } // Handle readyfortoken by using count of columns in the loop. targetColumn = _metaData.FieldMetaData.Count; } // Handle skipping default-valued fields while (targetColumn > _currentField) { // All intermediate fields must be default fields (i.e. have a "true" entry in SmiDefaultFieldsProperty Debug.Assert(((SmiDefaultFieldsProperty)_metaData.ExtendedProperties[SmiPropertySelector.DefaultFields])[_currentField], "Skipping a field that was not default: " + _currentField); _currentField++; } if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } [Conditional("DEBUG")] internal void CheckSettingColumn(int ordinal) { #if DEBUG // Make sure target column can be written to. CheckWritingToColumn(ordinal); _currentField++; if (_metaData.FieldMetaData.Count == _currentField) { _currentField = ReadyForToken; } #endif } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
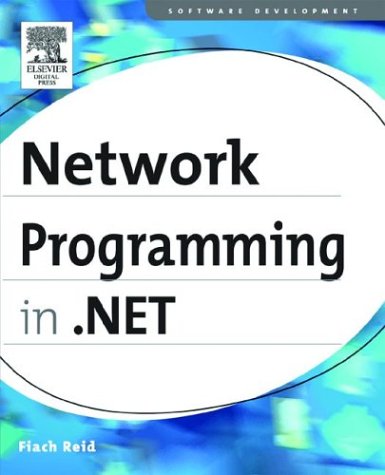
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContactManager.cs
- TraceSection.cs
- PropertyInformation.cs
- MobileUITypeEditor.cs
- MulticastNotSupportedException.cs
- APCustomTypeDescriptor.cs
- TypeNameConverter.cs
- XmlSchemaDatatype.cs
- HttpRequestTraceRecord.cs
- StrongNamePublicKeyBlob.cs
- HttpCachePolicy.cs
- ThemeDirectoryCompiler.cs
- FloatUtil.cs
- ListView.cs
- XmlDocumentType.cs
- X509ImageLogo.cs
- OdbcErrorCollection.cs
- SetterTriggerConditionValueConverter.cs
- ViewCellRelation.cs
- SqlTransaction.cs
- QilGenerator.cs
- PipelineComponent.cs
- SafeNativeMethodsOther.cs
- DataGridViewRowCollection.cs
- QilScopedVisitor.cs
- ReflectionServiceProvider.cs
- OleDbConnectionInternal.cs
- UntypedNullExpression.cs
- ValidatorUtils.cs
- LineGeometry.cs
- CrossAppDomainChannel.cs
- HostDesigntimeLicenseContext.cs
- X509CertificateRecipientClientCredential.cs
- DbProviderConfigurationHandler.cs
- Tracer.cs
- IndicFontClient.cs
- XsdBuilder.cs
- RegistrySecurity.cs
- GridViewRowCollection.cs
- CombinedGeometry.cs
- ProviderIncompatibleException.cs
- oledbmetadatacolumnnames.cs
- AndCondition.cs
- DataGridPagerStyle.cs
- StateFinalizationActivity.cs
- Win32Exception.cs
- _LazyAsyncResult.cs
- BatchStream.cs
- Condition.cs
- VisualCollection.cs
- XhtmlBasicLiteralTextAdapter.cs
- UnaryNode.cs
- XmlSerializerFactory.cs
- ClientBuildManagerCallback.cs
- BinaryFormatterWriter.cs
- TreeNode.cs
- ReversePositionQuery.cs
- CopyCodeAction.cs
- InputLanguage.cs
- Win32PrintDialog.cs
- ZipIOModeEnforcingStream.cs
- XmlCharacterData.cs
- DbConnectionClosed.cs
- AuthenticateEventArgs.cs
- SettingsSection.cs
- VisualTreeUtils.cs
- COM2FontConverter.cs
- InputLanguageCollection.cs
- PixelFormats.cs
- XmlIlTypeHelper.cs
- ApplicationTrust.cs
- QilReplaceVisitor.cs
- RequestCachePolicyConverter.cs
- AVElementHelper.cs
- Debug.cs
- FolderLevelBuildProviderCollection.cs
- RemotingServices.cs
- Convert.cs
- BindingExpressionBase.cs
- EdmValidator.cs
- SoundPlayer.cs
- SafeWaitHandle.cs
- AuthenticationModuleElementCollection.cs
- PrimitiveDataContract.cs
- ProxyHelper.cs
- ToolStripDropDownClosedEventArgs.cs
- XmlNotation.cs
- StylusPointPropertyInfoDefaults.cs
- ExtensionSimplifierMarkupObject.cs
- ColorConvertedBitmap.cs
- CacheSection.cs
- UserPersonalizationStateInfo.cs
- Propagator.cs
- SystemIPInterfaceProperties.cs
- Exceptions.cs
- WebPartsPersonalization.cs
- RecordConverter.cs
- PropertyToken.cs
- X509ClientCertificateAuthentication.cs
- MsmqOutputMessage.cs