Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaAttribute.cs / 1305376 / XmlSchemaAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml.XPath; using System.Collections; using System.ComponentModel; using System.Xml.Serialization; namespace System.Xml.Schema { ////// /// public class XmlSchemaAttribute : XmlSchemaAnnotated { string defaultValue; string fixedValue; string name; XmlSchemaForm form = XmlSchemaForm.None; XmlSchemaUse use = XmlSchemaUse.None; XmlQualifiedName refName = XmlQualifiedName.Empty; XmlQualifiedName typeName = XmlQualifiedName.Empty; XmlQualifiedName qualifiedName = XmlQualifiedName.Empty; XmlSchemaSimpleType type; XmlSchemaSimpleType attributeType; SchemaAttDef attDef; ///[To be supplied.] ////// /// [XmlAttribute("default")] [DefaultValue(null)] public string DefaultValue { get { return defaultValue; } set { defaultValue = value; } } ///[To be supplied.] ////// /// [XmlAttribute("fixed")] [DefaultValue(null)] public string FixedValue { get { return fixedValue; } set { fixedValue = value; } } ///[To be supplied.] ////// /// [XmlAttribute("form"), DefaultValue(XmlSchemaForm.None)] public XmlSchemaForm Form { get { return form; } set { form = value; } } ///[To be supplied.] ////// /// [XmlAttribute("name")] public string Name { get { return name; } set { name = value; } } ///[To be supplied.] ////// /// [XmlAttribute("ref")] public XmlQualifiedName RefName { get { return refName; } set { refName = (value == null ? XmlQualifiedName.Empty : value); } } ///[To be supplied.] ////// /// [XmlAttribute("type")] public XmlQualifiedName SchemaTypeName { get { return typeName; } set { typeName = (value == null ? XmlQualifiedName.Empty : value); } } ///[To be supplied.] ////// /// [XmlElement("simpleType")] public XmlSchemaSimpleType SchemaType { get { return type; } set { type = value; } } ///[To be supplied.] ////// /// [XmlAttribute("use"), DefaultValue(XmlSchemaUse.None)] public XmlSchemaUse Use { get { return use; } set { use = value; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlQualifiedName QualifiedName { get { return qualifiedName; } } ///[To be supplied.] ////// /// [XmlIgnore] [Obsolete("This property has been deprecated. Please use AttributeSchemaType property that returns a strongly typed attribute type. http://go.microsoft.com/fwlink/?linkid=14202")] public object AttributeType { get { if (attributeType == null) return null; if (attributeType.QualifiedName.Namespace == XmlReservedNs.NsXs) { return attributeType.Datatype; } return attributeType; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaSimpleType AttributeSchemaType { get { return attributeType; } } internal XmlReader Validate(XmlReader reader, XmlResolver resolver, XmlSchemaSet schemaSet, ValidationEventHandler valEventHandler) { if (schemaSet != null) { XmlReaderSettings readerSettings = new XmlReaderSettings(); readerSettings.ValidationType = ValidationType.Schema; readerSettings.Schemas = schemaSet; readerSettings.ValidationEventHandler += valEventHandler; return new XsdValidatingReader(reader, resolver, readerSettings, this); } return null; } [XmlIgnore] internal XmlSchemaDatatype Datatype { get { if (attributeType != null) { return attributeType.Datatype; } return null; } } internal void SetQualifiedName(XmlQualifiedName value) { qualifiedName = value; } internal void SetAttributeType(XmlSchemaSimpleType value) { attributeType = value; } internal SchemaAttDef AttDef { get { return attDef; } set { attDef = value; } } internal bool HasDefault { get { return defaultValue != null; } } [XmlIgnore] internal override string NameAttribute { get { return Name; } set { Name = value; } } internal override XmlSchemaObject Clone() { XmlSchemaAttribute newAtt = (XmlSchemaAttribute)MemberwiseClone(); //Deep clone the QNames as these will be updated on chameleon includes newAtt.refName = this.refName.Clone(); newAtt.typeName = this.typeName.Clone(); newAtt.qualifiedName = this.qualifiedName.Clone(); return newAtt; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml.XPath; using System.Collections; using System.ComponentModel; using System.Xml.Serialization; namespace System.Xml.Schema { ////// /// public class XmlSchemaAttribute : XmlSchemaAnnotated { string defaultValue; string fixedValue; string name; XmlSchemaForm form = XmlSchemaForm.None; XmlSchemaUse use = XmlSchemaUse.None; XmlQualifiedName refName = XmlQualifiedName.Empty; XmlQualifiedName typeName = XmlQualifiedName.Empty; XmlQualifiedName qualifiedName = XmlQualifiedName.Empty; XmlSchemaSimpleType type; XmlSchemaSimpleType attributeType; SchemaAttDef attDef; ///[To be supplied.] ////// /// [XmlAttribute("default")] [DefaultValue(null)] public string DefaultValue { get { return defaultValue; } set { defaultValue = value; } } ///[To be supplied.] ////// /// [XmlAttribute("fixed")] [DefaultValue(null)] public string FixedValue { get { return fixedValue; } set { fixedValue = value; } } ///[To be supplied.] ////// /// [XmlAttribute("form"), DefaultValue(XmlSchemaForm.None)] public XmlSchemaForm Form { get { return form; } set { form = value; } } ///[To be supplied.] ////// /// [XmlAttribute("name")] public string Name { get { return name; } set { name = value; } } ///[To be supplied.] ////// /// [XmlAttribute("ref")] public XmlQualifiedName RefName { get { return refName; } set { refName = (value == null ? XmlQualifiedName.Empty : value); } } ///[To be supplied.] ////// /// [XmlAttribute("type")] public XmlQualifiedName SchemaTypeName { get { return typeName; } set { typeName = (value == null ? XmlQualifiedName.Empty : value); } } ///[To be supplied.] ////// /// [XmlElement("simpleType")] public XmlSchemaSimpleType SchemaType { get { return type; } set { type = value; } } ///[To be supplied.] ////// /// [XmlAttribute("use"), DefaultValue(XmlSchemaUse.None)] public XmlSchemaUse Use { get { return use; } set { use = value; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlQualifiedName QualifiedName { get { return qualifiedName; } } ///[To be supplied.] ////// /// [XmlIgnore] [Obsolete("This property has been deprecated. Please use AttributeSchemaType property that returns a strongly typed attribute type. http://go.microsoft.com/fwlink/?linkid=14202")] public object AttributeType { get { if (attributeType == null) return null; if (attributeType.QualifiedName.Namespace == XmlReservedNs.NsXs) { return attributeType.Datatype; } return attributeType; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaSimpleType AttributeSchemaType { get { return attributeType; } } internal XmlReader Validate(XmlReader reader, XmlResolver resolver, XmlSchemaSet schemaSet, ValidationEventHandler valEventHandler) { if (schemaSet != null) { XmlReaderSettings readerSettings = new XmlReaderSettings(); readerSettings.ValidationType = ValidationType.Schema; readerSettings.Schemas = schemaSet; readerSettings.ValidationEventHandler += valEventHandler; return new XsdValidatingReader(reader, resolver, readerSettings, this); } return null; } [XmlIgnore] internal XmlSchemaDatatype Datatype { get { if (attributeType != null) { return attributeType.Datatype; } return null; } } internal void SetQualifiedName(XmlQualifiedName value) { qualifiedName = value; } internal void SetAttributeType(XmlSchemaSimpleType value) { attributeType = value; } internal SchemaAttDef AttDef { get { return attDef; } set { attDef = value; } } internal bool HasDefault { get { return defaultValue != null; } } [XmlIgnore] internal override string NameAttribute { get { return Name; } set { Name = value; } } internal override XmlSchemaObject Clone() { XmlSchemaAttribute newAtt = (XmlSchemaAttribute)MemberwiseClone(); //Deep clone the QNames as these will be updated on chameleon includes newAtt.refName = this.refName.Clone(); newAtt.typeName = this.typeName.Clone(); newAtt.qualifiedName = this.qualifiedName.Clone(); return newAtt; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
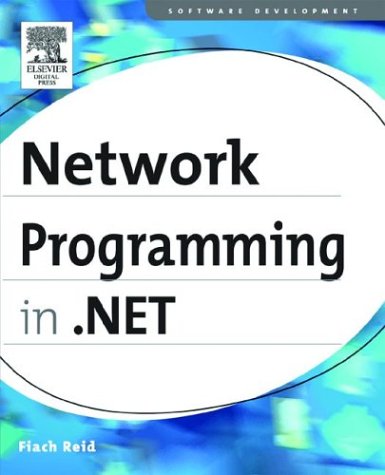
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpotLight.cs
- AlphabetConverter.cs
- RemoteCryptoRsaServiceProvider.cs
- CompilationUtil.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- XmlCollation.cs
- AsymmetricAlgorithm.cs
- ShapingWorkspace.cs
- ConnectionPoolManager.cs
- SettingsSection.cs
- XmlQueryContext.cs
- DataControlFieldCell.cs
- WCFBuildProvider.cs
- AccessKeyManager.cs
- KoreanCalendar.cs
- RowVisual.cs
- HtmlInputFile.cs
- ValidationSummary.cs
- SqlCacheDependencySection.cs
- keycontainerpermission.cs
- BitmapScalingModeValidation.cs
- WpfGeneratedKnownTypes.cs
- InternalResources.cs
- PackagePartCollection.cs
- DataSvcMapFileSerializer.cs
- Int32Storage.cs
- ToolbarAUtomationPeer.cs
- SessionPageStateSection.cs
- ApplicationFileParser.cs
- SerializationObjectManager.cs
- XmlSchemaIdentityConstraint.cs
- WebResourceUtil.cs
- MD5.cs
- UserMapPath.cs
- _Events.cs
- Matrix3DStack.cs
- LookupTables.cs
- IntegerCollectionEditor.cs
- OAVariantLib.cs
- ComponentCollection.cs
- DataGridViewColumnStateChangedEventArgs.cs
- DataGridLengthConverter.cs
- CompiledRegexRunner.cs
- EmptyImpersonationContext.cs
- DescendantQuery.cs
- RadioButtonStandardAdapter.cs
- InkCanvasAutomationPeer.cs
- Int64AnimationBase.cs
- TriggerCollection.cs
- EntityClientCacheEntry.cs
- ImagingCache.cs
- CodePageEncoding.cs
- AgileSafeNativeMemoryHandle.cs
- NamedObject.cs
- ModifierKeysConverter.cs
- DesignerValidatorAdapter.cs
- StyleTypedPropertyAttribute.cs
- SkewTransform.cs
- TemplateKeyConverter.cs
- MethodToken.cs
- Classification.cs
- SecurityPolicySection.cs
- InvocationExpression.cs
- TextRangeProviderWrapper.cs
- InvalidCastException.cs
- IPPacketInformation.cs
- AffineTransform3D.cs
- DynamicRendererThreadManager.cs
- DataGridRowClipboardEventArgs.cs
- DocumentPageHost.cs
- ExplicitDiscriminatorMap.cs
- Blend.cs
- ParallelTimeline.cs
- Html32TextWriter.cs
- ListControl.cs
- FrameworkContextData.cs
- NullableDecimalSumAggregationOperator.cs
- XmlLanguage.cs
- ActivityScheduledRecord.cs
- Adorner.cs
- DiscoveryMessageSequence11.cs
- DataGridHeaderBorder.cs
- ItemCheckEvent.cs
- dataprotectionpermission.cs
- PageParserFilter.cs
- MarkedHighlightComponent.cs
- MethodExpr.cs
- WindowsGraphics.cs
- CqlWriter.cs
- CallContext.cs
- DateTimeConverter2.cs
- MenuBase.cs
- SqlDataSourceCache.cs
- Parameter.cs
- DllNotFoundException.cs
- XmlILIndex.cs
- ProxyElement.cs
- ComponentRenameEvent.cs
- ObjectConverter.cs
- GrabHandleGlyph.cs