Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / ImagingCache.cs / 1 / ImagingCache.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: ImagingCache.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region ImagingCache /// /// ImagingCache provides caching for different Imaging objects /// Caches are thread-safe. /// internal static class ImagingCache { #region Methods /// Adds an object to the image cache internal static void AddToImageCache(Uri uri, object obj) { AddToCache(uri, obj, _imageCache); } /// Removes an object from the image cache internal static void RemoveFromImageCache(Uri uri) { RemoveFromCache(uri, _imageCache); } /// Get object from the image cache internal static object CheckImageCache(Uri uri) { return CheckCache(uri, _imageCache); } /// Adds an object to the decoder cache internal static void AddToDecoderCache(Uri uri, object obj) { AddToCache(uri, obj, _decoderCache); } /// Removes an object from the decoder cache internal static void RemoveFromDecoderCache(Uri uri) { RemoveFromCache(uri, _decoderCache); } /// Get object from the image cache internal static object CheckDecoderCache(Uri uri) { return CheckCache(uri, _decoderCache); } /// Adds an object to a given table private static void AddToCache(Uri uri, object obj, Hashtable table) { lock(table) { // if entry is already there, exit if (table.Contains(uri)) { return; } // if the table has reached the max size, try to see if we can reduce its size if (table.Count == MAX_CACHE_SIZE) { ArrayList al = new ArrayList(); foreach (DictionaryEntry de in table) { // if the value is a WeakReference that has been GC'd, remove it WeakReference weakRef = de.Value as WeakReference; if ((weakRef != null) && (weakRef.Target == null)) { al.Add(de.Key); } } foreach (object o in al) { table.Remove(o); } } // if table is still maxed out, exit if (table.Count == MAX_CACHE_SIZE) { return; } // add it table[uri] = obj; } } /// Removes an object from a given table private static void RemoveFromCache(Uri uri, Hashtable table) { lock(table) { // if entry is there, remove it if (table.Contains(uri)) { table.Remove(uri); } } } /// Return an object from a given table private static object CheckCache(Uri uri, Hashtable table) { lock(table) { return table[uri]; } } #endregion #region Data Members /// image cache private static Hashtable _imageCache = new Hashtable(); /// decoder cache private static Hashtable _decoderCache = new Hashtable(); /// max size to limit the cache private static int MAX_CACHE_SIZE = 300; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: ImagingCache.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { #region ImagingCache /// /// ImagingCache provides caching for different Imaging objects /// Caches are thread-safe. /// internal static class ImagingCache { #region Methods /// Adds an object to the image cache internal static void AddToImageCache(Uri uri, object obj) { AddToCache(uri, obj, _imageCache); } /// Removes an object from the image cache internal static void RemoveFromImageCache(Uri uri) { RemoveFromCache(uri, _imageCache); } /// Get object from the image cache internal static object CheckImageCache(Uri uri) { return CheckCache(uri, _imageCache); } /// Adds an object to the decoder cache internal static void AddToDecoderCache(Uri uri, object obj) { AddToCache(uri, obj, _decoderCache); } /// Removes an object from the decoder cache internal static void RemoveFromDecoderCache(Uri uri) { RemoveFromCache(uri, _decoderCache); } /// Get object from the image cache internal static object CheckDecoderCache(Uri uri) { return CheckCache(uri, _decoderCache); } /// Adds an object to a given table private static void AddToCache(Uri uri, object obj, Hashtable table) { lock(table) { // if entry is already there, exit if (table.Contains(uri)) { return; } // if the table has reached the max size, try to see if we can reduce its size if (table.Count == MAX_CACHE_SIZE) { ArrayList al = new ArrayList(); foreach (DictionaryEntry de in table) { // if the value is a WeakReference that has been GC'd, remove it WeakReference weakRef = de.Value as WeakReference; if ((weakRef != null) && (weakRef.Target == null)) { al.Add(de.Key); } } foreach (object o in al) { table.Remove(o); } } // if table is still maxed out, exit if (table.Count == MAX_CACHE_SIZE) { return; } // add it table[uri] = obj; } } /// Removes an object from a given table private static void RemoveFromCache(Uri uri, Hashtable table) { lock(table) { // if entry is there, remove it if (table.Contains(uri)) { table.Remove(uri); } } } /// Return an object from a given table private static object CheckCache(Uri uri, Hashtable table) { lock(table) { return table[uri]; } } #endregion #region Data Members /// image cache private static Hashtable _imageCache = new Hashtable(); /// decoder cache private static Hashtable _decoderCache = new Hashtable(); /// max size to limit the cache private static int MAX_CACHE_SIZE = 300; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
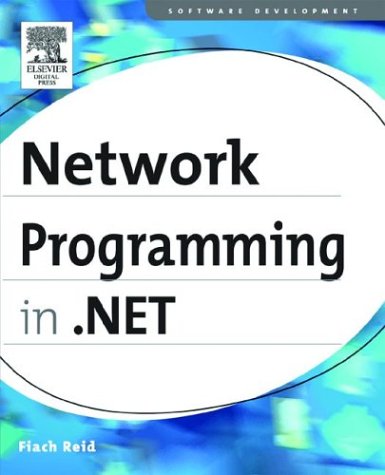
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InvalidStoreProtectionKeyException.cs
- XmlAttributeOverrides.cs
- ProtocolsConfiguration.cs
- ItemCheckEvent.cs
- XmlNamespaceManager.cs
- CommandBindingCollection.cs
- XmlILTrace.cs
- RecognizedAudio.cs
- WebBrowserHelper.cs
- ToolStripControlHost.cs
- CodeValidator.cs
- Profiler.cs
- DataGridItem.cs
- EventListenerClientSide.cs
- TrackBarRenderer.cs
- DPCustomTypeDescriptor.cs
- WebPartDescription.cs
- Schema.cs
- RequestCachePolicy.cs
- DbConnectionFactory.cs
- TreeChangeInfo.cs
- SoapHeaderException.cs
- EpmSourcePathSegment.cs
- Rect3D.cs
- MobileControlDesigner.cs
- SharedUtils.cs
- CLRBindingWorker.cs
- SqlDataReaderSmi.cs
- Content.cs
- HtmlInputText.cs
- BooleanAnimationBase.cs
- Pair.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- GridViewUpdatedEventArgs.cs
- AlternateView.cs
- DataKey.cs
- ColorTransformHelper.cs
- DataSourceCacheDurationConverter.cs
- ViewStateModeByIdAttribute.cs
- RegexCompiler.cs
- BoolExpressionVisitors.cs
- DataContractAttribute.cs
- XmlObjectSerializerReadContext.cs
- DataGridColumnEventArgs.cs
- KnownTypeDataContractResolver.cs
- ObjectDataSource.cs
- Keyboard.cs
- InputLanguageManager.cs
- FixUp.cs
- UserControlDocumentDesigner.cs
- ToolStripDropDownMenu.cs
- ToolStripDesignerAvailabilityAttribute.cs
- UntrustedRecipientException.cs
- DataRelationPropertyDescriptor.cs
- EnumValAlphaComparer.cs
- SimpleExpression.cs
- TimeZone.cs
- TypeConverterAttribute.cs
- WebResourceUtil.cs
- OneToOneMappingSerializer.cs
- ActivityTrace.cs
- MessageLoggingFilterTraceRecord.cs
- ReaderWriterLockWrapper.cs
- UserPreferenceChangedEventArgs.cs
- SizeIndependentAnimationStorage.cs
- XsdDateTime.cs
- UnicodeEncoding.cs
- SystemResources.cs
- DataMemberFieldEditor.cs
- ConstraintStruct.cs
- RMEnrollmentPage1.cs
- IUnknownConstantAttribute.cs
- XmlWriterDelegator.cs
- ParameterCollection.cs
- DashStyle.cs
- ConversionContext.cs
- NativeRightsManagementAPIsStructures.cs
- MetadataItem.cs
- PasswordRecovery.cs
- SliderAutomationPeer.cs
- HttpResponseInternalWrapper.cs
- IdlingCommunicationPool.cs
- UnsafeNativeMethods.cs
- WsatRegistrationHeader.cs
- DispatcherSynchronizationContext.cs
- CachedTypeface.cs
- KeyInterop.cs
- ColumnHeader.cs
- FontDialog.cs
- Win32Exception.cs
- DragDropManager.cs
- OleDbConnection.cs
- MimeObjectFactory.cs
- GeneralTransformGroup.cs
- DockPanel.cs
- codemethodreferenceexpression.cs
- BamlTreeUpdater.cs
- WebPartRestoreVerb.cs
- Int64Animation.cs
- XmlSubtreeReader.cs