Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / FontDialog.cs / 1 / FontDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.Runtime.InteropServices; using System.ComponentModel; using System.Windows.Forms; using System.Security; using System.Security.Permissions; using Microsoft.Win32; ////// /// [ DefaultEvent("Apply"), DefaultProperty("Font"), SRDescription(SR.DescriptionFontDialog) ] public class FontDialog : CommonDialog { ////// Represents /// a common dialog box that displays a list of fonts that are currently installed /// on /// the system. /// ////// /// protected static readonly object EventApply = new object(); private const int defaultMinSize = 0; private const int defaultMaxSize = 0; private int options; private Font font; private Color color; private int minSize = defaultMinSize; private int maxSize = defaultMaxSize; private bool showColor = false; ///[To be supplied.] ////// /// [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // If the constructor does not call Reset // it would be a breaking change. ] public FontDialog() { Reset(); } ////// Initializes a new instance of the ////// class. /// /// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.FnDallowSimulationsDescr) ] public bool AllowSimulations { get { return !GetOption(NativeMethods.CF_NOSIMULATIONS); } set { SetOption(NativeMethods.CF_NOSIMULATIONS, !value); } } ////// Gets or sets a value indicating whether the dialog box allows graphics device interface /// (GDI) font simulations. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.FnDallowVectorFontsDescr) ] public bool AllowVectorFonts { get { return !GetOption(NativeMethods.CF_NOVECTORFONTS); } set { SetOption(NativeMethods.CF_NOVECTORFONTS, !value); } } ////// Gets or sets a value indicating whether the dialog box allows vector font selections. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.FnDallowVerticalFontsDescr) ] public bool AllowVerticalFonts { get { return !GetOption(NativeMethods.CF_NOVERTFONTS); } set { SetOption(NativeMethods.CF_NOVERTFONTS, !value); } } ////// Gets or sets a value indicating whether /// the dialog box displays both vertical and horizontal fonts or only /// horizontal fonts. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.FnDallowScriptChangeDescr) ] public bool AllowScriptChange { get { return !GetOption(NativeMethods.CF_SELECTSCRIPT); } set { SetOption(NativeMethods.CF_SELECTSCRIPT, !value); } } ////// Gets /// or sets a value indicating whether the user can change the character set specified /// in the Script combo box to display a character set other than the one /// currently displayed. /// ////// /// [ SRCategory(SR.CatData), SRDescription(SR.FnDcolorDescr), DefaultValue(typeof(Color), "Black") ] public Color Color { get { return color; } set { if (!value.IsEmpty) { color = value; } else { color = Color.Black; } } } ////// Gets or sets a value indicating the selected font color. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDfixedPitchOnlyDescr) ] public bool FixedPitchOnly { get { return GetOption(NativeMethods.CF_FIXEDPITCHONLY); } set { SetOption(NativeMethods.CF_FIXEDPITCHONLY, value); } } ////// Gets or sets /// a value indicating whether the dialog box allows only the selection of fixed-pitch fonts. /// ////// /// [ SRCategory(SR.CatData), SRDescription(SR.FnDfontDescr) ] public Font Font { get { Font result = font; if (result == null) result = Control.DefaultFont; float actualSize = result.SizeInPoints; if (minSize != defaultMinSize && actualSize < MinSize) result = new Font(result.FontFamily, MinSize, result.Style, GraphicsUnit.Point); if (maxSize != defaultMaxSize && actualSize > MaxSize) result = new Font(result.FontFamily, MaxSize, result.Style, GraphicsUnit.Point); return result; } set { font = value; } } ////// Gets or sets a value indicating the selected font. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDfontMustExistDescr) ] public bool FontMustExist { get { return GetOption(NativeMethods.CF_FORCEFONTEXIST); } set { SetOption(NativeMethods.CF_FORCEFONTEXIST, value); } } ////// Gets or sets a value indicating whether the dialog box specifies an error condition if the /// user attempts to select a font or style that does not exist. /// ////// /// [ SRCategory(SR.CatData), DefaultValue(defaultMaxSize), SRDescription(SR.FnDmaxSizeDescr) ] public int MaxSize { get { return maxSize; } set { if (value < 0) { value = 0; } maxSize = value; if (maxSize > 0 && maxSize < minSize) { minSize = maxSize; } } } ////// Gets or sets the maximum /// point size a user can select. /// ////// /// [ SRCategory(SR.CatData), DefaultValue(defaultMinSize), SRDescription(SR.FnDminSizeDescr) ] public int MinSize { get { return minSize; } set { if (value < 0) { value = 0; } minSize = value; if (maxSize > 0 && maxSize < minSize) { maxSize = minSize; } } } ////// Gets or sets a value indicating the minimum point size a user can select. /// ////// /// /// protected int Options { get { return options; } } ////// Gets the value passed to CHOOSEFONT.Flags. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDscriptsOnlyDescr) ] public bool ScriptsOnly { get { return GetOption(NativeMethods.CF_SCRIPTSONLY); } set { SetOption(NativeMethods.CF_SCRIPTSONLY, value); } } ////// Gets or sets a /// value indicating whether the dialog box allows selection of fonts for all non-OEM and Symbol character /// sets, as well as the American National Standards Institute (ANSI) character set. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDshowApplyDescr) ] public bool ShowApply { get { return GetOption(NativeMethods.CF_APPLY); } set { SetOption(NativeMethods.CF_APPLY, value); } } ////// Gets or sets a value indicating whether the dialog box contains an Apply button. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDshowColorDescr) ] public bool ShowColor { get { return showColor; } set { this.showColor = value; } } ////// Gets or sets a value indicating whether the dialog box displays the color choice. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.FnDshowEffectsDescr) ] public bool ShowEffects { get { return GetOption(NativeMethods.CF_EFFECTS); } set { SetOption(NativeMethods.CF_EFFECTS, value); } } ////// Gets or sets a value indicating whether the dialog box contains controls that allow the /// user to specify strikethrough, underline, and text color options. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.FnDshowHelpDescr) ] public bool ShowHelp { get { return GetOption(NativeMethods.CF_SHOWHELP); } set { SetOption(NativeMethods.CF_SHOWHELP, value); } } ////// Gets or sets a value indicating whether the dialog box displays a Help button. /// ////// /// [SRDescription(SR.FnDapplyDescr)] public event EventHandler Apply { add { Events.AddHandler(EventApply, value); } remove { Events.RemoveHandler(EventApply, value); } } ////// Occurs when the user clicks the Apply button in the font /// dialog box. /// ////// /// Returns the state of the given option flag. /// ///internal bool GetOption(int option) { return(options & option) != 0; } /// /// /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] protected override IntPtr HookProc(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { switch (msg) { case NativeMethods.WM_COMMAND: if ((int)wparam == 0x402) { NativeMethods.LOGFONT lf = new NativeMethods.LOGFONT(); UnsafeNativeMethods.SendMessage(new HandleRef(null, hWnd), NativeMethods.WM_CHOOSEFONT_GETLOGFONT, 0, lf); UpdateFont(lf); int index = (int)UnsafeNativeMethods.SendDlgItemMessage(new HandleRef(null, hWnd), 0x473, NativeMethods.CB_GETCURSEL, IntPtr.Zero, IntPtr.Zero); if (index != NativeMethods.CB_ERR) { UpdateColor((int)UnsafeNativeMethods.SendDlgItemMessage(new HandleRef(null, hWnd), 0x473, NativeMethods.CB_GETITEMDATA, (IntPtr) index, IntPtr.Zero)); } if (NativeWindow.WndProcShouldBeDebuggable) { OnApply(EventArgs.Empty); } else { try { OnApply(EventArgs.Empty); } catch (Exception e) { Application.OnThreadException(e); } } } break; case NativeMethods.WM_INITDIALOG: if (!showColor) { IntPtr hWndCtl = UnsafeNativeMethods.GetDlgItem(new HandleRef(null, hWnd), NativeMethods.cmb4); SafeNativeMethods.ShowWindow(new HandleRef(null, hWndCtl), NativeMethods.SW_HIDE); hWndCtl = UnsafeNativeMethods.GetDlgItem(new HandleRef(null, hWnd), NativeMethods.stc4); SafeNativeMethods.ShowWindow(new HandleRef(null, hWndCtl), NativeMethods.SW_HIDE); } break; } return base.HookProc(hWnd, msg, wparam, lparam); } ////// Specifies the common dialog box hook procedure that is overridden to add /// specific functionality to a common dialog box. /// ////// /// protected virtual void OnApply(EventArgs e) { EventHandler handler = (EventHandler)Events[EventApply]; if (handler != null) handler(this, e); } ////// Raises the ///event. /// /// /// public override void Reset() { options = NativeMethods.CF_SCREENFONTS | NativeMethods.CF_EFFECTS; font = null; color = Color.Black; showColor = false; minSize = defaultMinSize; maxSize = defaultMaxSize; SetOption(NativeMethods.CF_TTONLY, true); } private void ResetFont() { font = null; } ////// Resets all dialog box options to their default values. /// ////// /// /// protected override bool RunDialog(IntPtr hWndOwner) { NativeMethods.WndProc hookProcPtr = new NativeMethods.WndProc(this.HookProc); NativeMethods.CHOOSEFONT cf = new NativeMethods.CHOOSEFONT(); IntPtr screenDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); NativeMethods.LOGFONT lf = new NativeMethods.LOGFONT(); Graphics graphics = Graphics.FromHdcInternal(screenDC); // SECREVIEW : The Font.ToLogFont method is marked with the 'unsafe' modifier cause it needs to write bytes into the logFont struct, // here we are passing that struct so the assert is safe. // IntSecurity.ObjectFromWin32Handle.Assert(); try { Font.ToLogFont(lf, graphics); } finally { CodeAccessPermission.RevertAssert(); graphics.Dispose(); } UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, screenDC)); IntPtr logFontPtr = IntPtr.Zero; try { logFontPtr = Marshal.AllocCoTaskMem(Marshal.SizeOf(typeof(NativeMethods.LOGFONT))); Marshal.StructureToPtr(lf, logFontPtr, false); cf.lStructSize = Marshal.SizeOf(typeof(NativeMethods.CHOOSEFONT)); cf.hwndOwner = hWndOwner; cf.hDC = IntPtr.Zero; cf.lpLogFont = logFontPtr; cf.Flags = Options | NativeMethods.CF_INITTOLOGFONTSTRUCT | NativeMethods.CF_ENABLEHOOK; if (minSize > 0 || maxSize > 0) { cf.Flags |= NativeMethods.CF_LIMITSIZE; } //if ShowColor=true then try to draw the sample text in color, //if ShowEffects=false then we will draw the sample text in black regardless. //(limitation of windows control) // if (ShowColor || ShowEffects) { cf.rgbColors = ColorTranslator.ToWin32(color); } else { cf.rgbColors = ColorTranslator.ToWin32(Color.Black); } cf.lpfnHook = hookProcPtr; cf.hInstance = UnsafeNativeMethods.GetModuleHandle(null); cf.nSizeMin = minSize; if (maxSize == 0) { cf.nSizeMax = Int32.MaxValue; } else { cf.nSizeMax = maxSize; } Debug.Assert(cf.nSizeMin <= cf.nSizeMax, "min and max font sizes are the wrong way around"); if (!SafeNativeMethods.ChooseFont(cf)) return false; NativeMethods.LOGFONT lfReturned = null; lfReturned = (NativeMethods.LOGFONT)UnsafeNativeMethods.PtrToStructure(logFontPtr, typeof(NativeMethods.LOGFONT)); if (lfReturned.lfFaceName != null && lfReturned.lfFaceName.Length > 0) { lf = lfReturned; UpdateFont(lf); UpdateColor(cf.rgbColors); } return true; } finally { if (logFontPtr != IntPtr.Zero) Marshal.FreeCoTaskMem(logFontPtr); } } ////// The actual implementation of running the dialog. Inheriting classes /// should override this if they want to add more functionality, and call /// base.runDialog() if necessary /// /// ////// /// Sets the given option to the given boolean value. /// ///internal void SetOption(int option, bool value) { if (value) { options |= option; } else { options &= ~option; } } /// /// /// private bool ShouldSerializeFont() { return !Font.Equals(Control.DefaultFont); } ////// Indicates whether the ///property should be /// persisted. /// /// /// /// public override string ToString() { string s = base.ToString(); return s + ", Font: " + Font.ToString(); } ////// Retrieves a string that includes the name of the current font selected in /// the dialog box. /// /// ////// /// ///private void UpdateColor(int rgb) { if (ColorTranslator.ToWin32(color) != rgb) { color = ColorTranslator.FromOle(rgb); } } /// /// /// ///private void UpdateFont(NativeMethods.LOGFONT lf) { IntPtr screenDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); try { Font fontInWorldUnits = null; try { IntSecurity.UnmanagedCode.Assert(); try { fontInWorldUnits = Font.FromLogFont(lf, screenDC); } finally { CodeAccessPermission.RevertAssert(); } // The dialog claims its working in points (a device-independent unit), // but actually gives us something in world units (device-dependent). font = ControlPaint.FontInPoints(fontInWorldUnits); } finally { if (fontInWorldUnits != null) { fontInWorldUnits.Dispose(); } } } finally { UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, screenDC)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
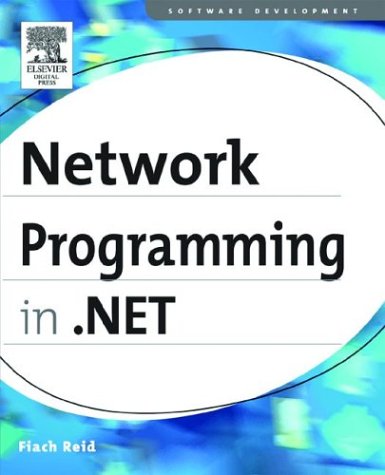
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResolvedKeyFrameEntry.cs
- Bitmap.cs
- StructuredType.cs
- CollectionBase.cs
- Dynamic.cs
- ClickablePoint.cs
- HtmlTableCell.cs
- CommandManager.cs
- TextUtf8RawTextWriter.cs
- SmiGettersStream.cs
- BindingCollection.cs
- DataServiceRequestException.cs
- MemberPathMap.cs
- InitializerFacet.cs
- SafeNativeMethods.cs
- TextRunProperties.cs
- TreeNodeBindingDepthConverter.cs
- CryptoStream.cs
- DataRowComparer.cs
- XmlAttributes.cs
- HostingPreferredMapPath.cs
- ConsumerConnectionPoint.cs
- RoleBoolean.cs
- XmlChildNodes.cs
- _KerberosClient.cs
- _NTAuthentication.cs
- WizardDesigner.cs
- RootContext.cs
- GPPOINTF.cs
- RoleService.cs
- PartialArray.cs
- EntityClassGenerator.cs
- FixedPosition.cs
- FloaterBaseParagraph.cs
- PassportAuthenticationModule.cs
- MenuBase.cs
- ScheduleChanges.cs
- HtmlControlPersistable.cs
- DataBinding.cs
- ServiceModelExtensionElement.cs
- CardSpacePolicyElement.cs
- VoiceChangeEventArgs.cs
- ImageSourceConverter.cs
- CodeParameterDeclarationExpression.cs
- MulticastOption.cs
- AsyncCompletedEventArgs.cs
- TextTreeExtractElementUndoUnit.cs
- SiteMapNodeItemEventArgs.cs
- DataGridSortCommandEventArgs.cs
- ThumbAutomationPeer.cs
- CurrentChangingEventManager.cs
- ProgressBarRenderer.cs
- DbResourceAllocator.cs
- HighContrastHelper.cs
- ContextStaticAttribute.cs
- NullableDecimalSumAggregationOperator.cs
- EventTrigger.cs
- SafeLibraryHandle.cs
- IPEndPointCollection.cs
- GenericRootAutomationPeer.cs
- ContentDesigner.cs
- DiagnosticEventProvider.cs
- ComboBoxItem.cs
- RegexCompilationInfo.cs
- XmlAttributeOverrides.cs
- FileInfo.cs
- AlternateView.cs
- ChannelDispatcher.cs
- SchemaImporterExtension.cs
- Html32TextWriter.cs
- ConfigXmlCDataSection.cs
- FormViewPageEventArgs.cs
- VisualCollection.cs
- FormsAuthenticationTicket.cs
- CompilationRelaxations.cs
- SectionVisual.cs
- CustomAttributeSerializer.cs
- JavascriptCallbackBehaviorAttribute.cs
- PropertyNames.cs
- TypeDescriptorFilterService.cs
- RichTextBoxConstants.cs
- GroupItem.cs
- Profiler.cs
- SHA1CryptoServiceProvider.cs
- SchemaImporterExtensionsSection.cs
- BamlReader.cs
- SqlInternalConnectionTds.cs
- RequestSecurityTokenResponse.cs
- TextEditorMouse.cs
- DefaultPrintController.cs
- LoginUtil.cs
- RawStylusInputReport.cs
- SafeArrayTypeMismatchException.cs
- QueryContinueDragEvent.cs
- Convert.cs
- X509CertificateChain.cs
- DataViewSetting.cs
- RC2CryptoServiceProvider.cs
- NullableLongSumAggregationOperator.cs
- CheckBoxField.cs