Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / CqlWriter.cs / 1305376 / CqlWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text.RegularExpressions; using System.Text; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // This class contains helper methods needed for generating Cql internal static class CqlWriter { #region Fields private static readonly Regex s_wordIdentifierRegex = new Regex(@"^[_A-Za-z]\w*$", RegexOptions.ECMAScript | RegexOptions.Compiled); #endregion #region Helper Methods // effects: Given a block name and a field in it -- returns a string // of form "blockName.field". Does not perform any escaping internal static string GetQualifiedName(string blockName, string field) { string result = StringUtil.FormatInvariant("{0}.{1}", blockName, field); return result; } // effects: Modifies builder to contain an escaped version of type's name as "[namespace.typename]" internal static void AppendEscapedTypeName(StringBuilder builder, EdmType type) { AppendEscapedName(builder, GetQualifiedName(type.NamespaceName, type.Name)); } // effects: Modifies builder to contain an escaped version of "name1.name2" as "[name1].[name2]" internal static void AppendEscapedQualifiedName(StringBuilder builder, string name1, string name2) { AppendEscapedName(builder, name1); builder.Append('.'); AppendEscapedName(builder, name2); } // effects: Modifies builder to contain an escaped version of "name" internal static void AppendEscapedName(StringBuilder builder, string name) { if (s_wordIdentifierRegex.IsMatch(name) && false == ExternalCalls.IsReservedKeyword(name)) { // We do not need to escape the name if it is a simple name and it is not a keyword builder.Append(name); } else { string newName = name.Replace("]", "]]"); builder.Append('[') .Append(newName) .Append(']'); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text.RegularExpressions; using System.Text; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // This class contains helper methods needed for generating Cql internal static class CqlWriter { #region Fields private static readonly Regex s_wordIdentifierRegex = new Regex(@"^[_A-Za-z]\w*$", RegexOptions.ECMAScript | RegexOptions.Compiled); #endregion #region Helper Methods // effects: Given a block name and a field in it -- returns a string // of form "blockName.field". Does not perform any escaping internal static string GetQualifiedName(string blockName, string field) { string result = StringUtil.FormatInvariant("{0}.{1}", blockName, field); return result; } // effects: Modifies builder to contain an escaped version of type's name as "[namespace.typename]" internal static void AppendEscapedTypeName(StringBuilder builder, EdmType type) { AppendEscapedName(builder, GetQualifiedName(type.NamespaceName, type.Name)); } // effects: Modifies builder to contain an escaped version of "name1.name2" as "[name1].[name2]" internal static void AppendEscapedQualifiedName(StringBuilder builder, string name1, string name2) { AppendEscapedName(builder, name1); builder.Append('.'); AppendEscapedName(builder, name2); } // effects: Modifies builder to contain an escaped version of "name" internal static void AppendEscapedName(StringBuilder builder, string name) { if (s_wordIdentifierRegex.IsMatch(name) && false == ExternalCalls.IsReservedKeyword(name)) { // We do not need to escape the name if it is a simple name and it is not a keyword builder.Append(name); } else { string newName = name.Replace("]", "]]"); builder.Append('[') .Append(newName) .Append(']'); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
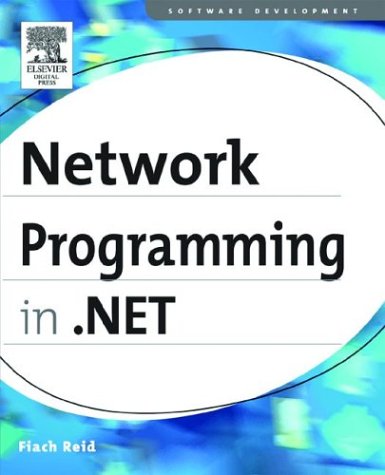
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServerValidateEventArgs.cs
- SchemaTableOptionalColumn.cs
- GroupItemAutomationPeer.cs
- HtmlLink.cs
- ProcessProtocolHandler.cs
- TextCharacters.cs
- GridViewActionList.cs
- VBIdentifierNameEditor.cs
- StylusButton.cs
- EqualityComparer.cs
- PixelFormats.cs
- UriSection.cs
- PermissionSet.cs
- AssemblyNameProxy.cs
- SoapAttributeOverrides.cs
- SettingsContext.cs
- AsyncResult.cs
- TextRangeEdit.cs
- ClientScriptManager.cs
- CryptoApi.cs
- Parser.cs
- ObjectCloneHelper.cs
- ByteStack.cs
- RenamedEventArgs.cs
- AuthorizationContext.cs
- StyleReferenceConverter.cs
- ServiceNameElement.cs
- EventLogInternal.cs
- WebBrowsableAttribute.cs
- CopyCodeAction.cs
- OperationAbortedException.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ToolStripItem.cs
- DefaultHttpHandler.cs
- CheckBoxPopupAdapter.cs
- StreamGeometry.cs
- MonitorWrapper.cs
- PartDesigner.cs
- RtfControlWordInfo.cs
- SingleObjectCollection.cs
- TextTreeNode.cs
- ProxyAttribute.cs
- CodeNamespaceImport.cs
- MessageDecoder.cs
- DataColumnPropertyDescriptor.cs
- ServicePointManager.cs
- RealizationDrawingContextWalker.cs
- BitmapFrameEncode.cs
- EventLogQuery.cs
- AppSettingsExpressionEditor.cs
- PasswordRecoveryAutoFormat.cs
- XmlNamespaceManager.cs
- ChangeConflicts.cs
- XmlReflectionImporter.cs
- SystemColors.cs
- IntPtr.cs
- Visual.cs
- DataGridColumnCollection.cs
- IisTraceListener.cs
- Attribute.cs
- VirtualizingPanel.cs
- ComponentResourceKeyConverter.cs
- FileClassifier.cs
- ToolbarAUtomationPeer.cs
- PropertyTabChangedEvent.cs
- FlowDocumentScrollViewer.cs
- _SslStream.cs
- TypeSystemHelpers.cs
- GenericWebPart.cs
- UInt32Storage.cs
- safelinkcollection.cs
- DataGridTableCollection.cs
- FixedSOMTableCell.cs
- CacheDependency.cs
- mda.cs
- LayoutTableCell.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- KerberosTicketHashIdentifierClause.cs
- UdpSocketReceiveManager.cs
- LocalizationCodeDomSerializer.cs
- RuleSettings.cs
- InvokeMethod.cs
- DesignerView.xaml.cs
- AutomationElementIdentifiers.cs
- GeometryCombineModeValidation.cs
- ExtentCqlBlock.cs
- PropertyValueChangedEvent.cs
- QuadraticBezierSegment.cs
- AdRotator.cs
- MailWebEventProvider.cs
- EntityDataSourceWizardForm.cs
- TextDecorations.cs
- OracleColumn.cs
- AuthenticateEventArgs.cs
- Funcletizer.cs
- PeerEndPoint.cs
- Executor.cs
- ComplexTypeEmitter.cs
- LocalizationParserHooks.cs
- Transform.cs