Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Util / SingleObjectCollection.cs / 1 / SingleObjectCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * SingleObjectCollection class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Util { using System.Collections; /* * Fast implementation of a collection with a single object */ internal class SingleObjectCollection: ICollection { private class SingleObjectEnumerator: IEnumerator { private object _object; private bool done; public SingleObjectEnumerator(object o) { _object = o; } public object Current { get { return _object; } } public bool MoveNext() { if (!done) { done = true; return true; } return false; } public void Reset() { done = false; } } private object _object; public SingleObjectCollection(object o) { _object = o; } IEnumerator IEnumerable.GetEnumerator() { return new SingleObjectEnumerator(_object); } public int Count { get { return 1; } } bool ICollection.IsSynchronized { get { return true; } } object ICollection.SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { array.SetValue(_object, index); } } }
Link Menu
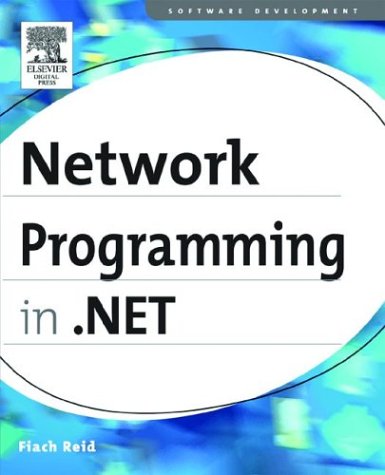
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceBuildProvider.cs
- SqlConnection.cs
- RequestCachePolicy.cs
- Grant.cs
- TableColumnCollection.cs
- WpfXamlLoader.cs
- TreeViewCancelEvent.cs
- ByteStack.cs
- ItemContainerPattern.cs
- CommaDelimitedStringAttributeCollectionConverter.cs
- EntityExpressionVisitor.cs
- PixelFormats.cs
- QueryOperationResponseOfT.cs
- IdentityReference.cs
- DuplicateWaitObjectException.cs
- CodeMemberMethod.cs
- Events.cs
- TemplateBindingExtension.cs
- AutomationProperties.cs
- DataGridViewAccessibleObject.cs
- MetadataItem_Static.cs
- Nullable.cs
- FileVersion.cs
- DesignOnlyAttribute.cs
- ChooseAction.cs
- ErrorFormatterPage.cs
- SizeChangedEventArgs.cs
- SerializerProvider.cs
- DoubleAnimation.cs
- CustomExpressionEventArgs.cs
- ConfigUtil.cs
- CodeGenHelper.cs
- wgx_exports.cs
- DetailsViewRowCollection.cs
- ObjectViewQueryResultData.cs
- RequestResizeEvent.cs
- TableAdapterManagerHelper.cs
- ObjectListGeneralPage.cs
- OutputScope.cs
- SQLBinaryStorage.cs
- TimelineCollection.cs
- MemberExpression.cs
- StatusCommandUI.cs
- ColumnMapVisitor.cs
- GeometryCombineModeValidation.cs
- ReliableReplySessionChannel.cs
- FrameDimension.cs
- WebHttpSecurityModeHelper.cs
- DrawingAttributesDefaultValueFactory.cs
- BulletChrome.cs
- ZipIOCentralDirectoryFileHeader.cs
- BrowserDefinitionCollection.cs
- hebrewshape.cs
- PageParser.cs
- DataServiceProviderMethods.cs
- CacheModeConverter.cs
- MetadataWorkspace.cs
- HtmlTitle.cs
- WebPartConnection.cs
- Int64Animation.cs
- ProviderIncompatibleException.cs
- DynamicDiscoveryDocument.cs
- CodeTypeParameter.cs
- MissingManifestResourceException.cs
- HttpConfigurationSystem.cs
- TableItemPatternIdentifiers.cs
- XmlQueryCardinality.cs
- SchemaCollectionCompiler.cs
- SymLanguageVendor.cs
- ImportCatalogPart.cs
- ComponentResourceKeyConverter.cs
- ApplicationServiceManager.cs
- XmlDataProvider.cs
- IResourceProvider.cs
- Internal.cs
- MessageUtil.cs
- DurationConverter.cs
- ComponentManagerBroker.cs
- VBCodeProvider.cs
- ColumnWidthChangedEvent.cs
- HexParser.cs
- cookiecollection.cs
- ManagementOptions.cs
- TrustManagerPromptUI.cs
- DataGridViewSortCompareEventArgs.cs
- OleDbDataReader.cs
- ReliabilityContractAttribute.cs
- Math.cs
- PropertyHelper.cs
- XamlSerializationHelper.cs
- Memoizer.cs
- StateRuntime.cs
- ComponentSerializationService.cs
- DataSourceHelper.cs
- WinFormsSpinner.cs
- CodePageUtils.cs
- RequestSecurityTokenForGetBrowserToken.cs
- XmlSchemaElement.cs
- GorillaCodec.cs
- WebColorConverter.cs