Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RequestSecurityTokenForGetBrowserToken.cs / 1 / RequestSecurityTokenForGetBrowserToken.cs
namespace Microsoft.InfoCards { using System; using System.Xml; using System.Collections; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class is used to write an RST to the STS for the get browser token case. // internal class RequestSecurityTokenForGetBrowserToken : RequestSecurityToken { public RequestSecurityTokenForGetBrowserToken( RequestSecurityTokenParameters rstParams ) : base( rstParams ) { } protected override void WriteKeyTypeElement() { // // Write the KeyType element. In the browser case, this value must be No Proof Key. // IDT.Assert( ProtocolVersionProfile.WSTrust.KeyTypeBearer.ToString() == Policy.GetKeyTypeString(), "Only no proof key allowed in browser case" ); IDT.TraceDebug( "IPSTSCLIENT: Writing key type {0} to RST", Policy.GetKeyTypeString() ); Serializer.WriteKeyTypeElement( Policy.GetKeyTypeString() ); } protected override void WriteRequestTypeElement() { // // For browser case, we only support the Issue action. // IDT.TraceDebug( "IPSTSCLIENT: Writing RequestType {0} to RST", ProtocolVersionProfile.WSTrust.IssueRequestType ); Serializer.WriteRequestTypeElement( ProtocolVersionProfile.WSTrust.IssueRequestType ); } protected override void WriteAppliesToElement() { // // Always send AppliesTo in browser case - same behavior as Indigo federated token provider // GetBrowserTokenRequest.WriteSourceUrlAppliesTo( Writer, m_rstParams.BrowserTokenParams, ProtocolVersionProfile ); } protected override void WriteSecondaryParametersElement() { // // No need to check appliesTo in the browser case, since it will always be sent. // // // Write out a copy of the policy XML to the SecondaryParameters element if we are using the // oasis 2007 version of WS-Trust. // if( XmlNames.WSSpecificationVersion.WSTrustOasis2007 == ProtocolVersionProfile.WSTrust.Version ) { // // If the policy contains optional claims but the user has elected to not sent optional claims, then we // cannot sent secondaryParameters (as we do not want accidental disclosure of information to a // non-auditing STS). // bool writeSecondaryParameters = true; if( Policy.OptionalClaims.Length > 0 ) { // // If the policy contains one or more optional claims, check to see if the user // is willing to send optional claims. // writeSecondaryParameters = m_rstParams.DiscloseOptionalClaims; } if( writeSecondaryParameters ) { if( null != Policy.RelyingPartyPolicy ) { Serializer.WriteSecondaryParametersElement( Policy.RelyingPartyPolicy.PolicyXml ); } else { // // If the incoming RST Template did not contain SecondaryParameters, we simply write the // original request. // Serializer.WriteSecondaryParametersElement( Policy.ClientPolicy.PolicyXml ); } } } } protected override void CustomWriteBodyContents( XmlDictionaryWriter writer ) { InitializeWriters( writer ); WriteRSTOpeningElement(); WriteKeyTypeElement(); WriteRequestTypeElement(); WriteAppliesToElement(); WriteClaimsElement(); WriteTokenTypeElement(); WriteSecondaryParametersElement(); WriteEndElement(); // // The following elements are explicitly excluded from the browser case: // // InfoCardReferenceElement // KeySupportingElements // PPIDElement // EncryptionAlgorithmElement // DisplayTokenElement // PassOnElements // UnprocessedPolicyElements // } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
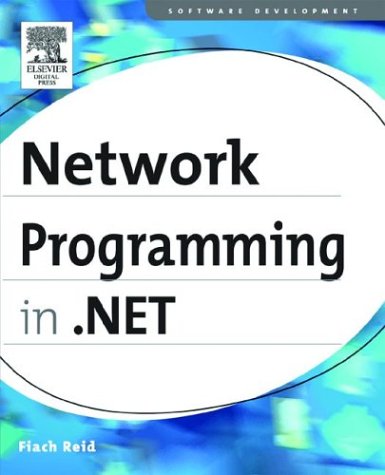
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerInputChannel.cs
- Calendar.cs
- NotifyIcon.cs
- CompilationUtil.cs
- ConfigurationSectionGroupCollection.cs
- MergeEnumerator.cs
- ExceptQueryOperator.cs
- DesignConnection.cs
- EmptyCollection.cs
- MenuAutomationPeer.cs
- Matrix.cs
- DataSourceCache.cs
- ResourceSetExpression.cs
- ActiveXSite.cs
- TextEffect.cs
- WebConfigManager.cs
- PackageDigitalSignature.cs
- StorageModelBuildProvider.cs
- ExeConfigurationFileMap.cs
- UDPClient.cs
- TagNameToTypeMapper.cs
- CompilerInfo.cs
- HttpResponseHeader.cs
- ParallelEnumerableWrapper.cs
- TimeSpanValidatorAttribute.cs
- Registry.cs
- ZipIOExtraFieldElement.cs
- DoubleLink.cs
- StartFileNameEditor.cs
- CommonDialog.cs
- WeakReadOnlyCollection.cs
- HttpAsyncResult.cs
- LogicalTreeHelper.cs
- ObfuscateAssemblyAttribute.cs
- EntityException.cs
- MiniAssembly.cs
- SchemeSettingElement.cs
- RelationshipManager.cs
- FlowLayoutPanel.cs
- SpinLock.cs
- ArrayTypeMismatchException.cs
- XmlnsCompatibleWithAttribute.cs
- TrustLevel.cs
- Control.cs
- WorkflowRuntimeElement.cs
- TagElement.cs
- SrgsNameValueTag.cs
- SecurityCapabilities.cs
- IListConverters.cs
- AssemblyInfo.cs
- PageVisual.cs
- FixedTextPointer.cs
- CounterSample.cs
- User.cs
- entitydatasourceentitysetnameconverter.cs
- BitSet.cs
- FixedPageAutomationPeer.cs
- CodeBlockBuilder.cs
- AncestorChangedEventArgs.cs
- HttpClientCertificate.cs
- AccessText.cs
- ValidationError.cs
- AlternationConverter.cs
- UTF7Encoding.cs
- CodeNamespace.cs
- Assembly.cs
- DataObjectMethodAttribute.cs
- Error.cs
- WindowsIdentity.cs
- NativeMethodsOther.cs
- WebPartCollection.cs
- DBDataPermissionAttribute.cs
- DefaultAsyncDataDispatcher.cs
- GridItemPatternIdentifiers.cs
- RichTextBoxConstants.cs
- ApplicationInterop.cs
- OracleTimeSpan.cs
- diagnosticsswitches.cs
- SignatureToken.cs
- MemberPathMap.cs
- SqlErrorCollection.cs
- PartialTrustVisibleAssembliesSection.cs
- DataKey.cs
- XmlAttributeProperties.cs
- SoapException.cs
- DatagridviewDisplayedBandsData.cs
- SevenBitStream.cs
- EndpointDiscoveryBehavior.cs
- DBDataPermissionAttribute.cs
- XslTransform.cs
- XmlWriterTraceListener.cs
- XMLSyntaxException.cs
- MouseEventArgs.cs
- QilInvokeLateBound.cs
- HttpHandlersSection.cs
- ISFTagAndGuidCache.cs
- TableDetailsRow.cs
- UriSectionReader.cs
- KeyEvent.cs
- oledbmetadatacolumnnames.cs