Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / RawStylusSystemGestureInputReport.cs / 1305600 / RawStylusSystemGestureInputReport.cs
using System; using System.ComponentModel; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// [TBS] /// internal class RawStylusSystemGestureInputReport : RawStylusInputReport { // We don't want to publically expose the double tap system gesture so we define it here. internal const SystemGesture InternalSystemGestureDoubleTap = (SystemGesture)0x11; ////// Validates whether a given SystemGesture has an allowable value. /// /// The SysemGesture to test. /// Whether Flick is allowed. /// Whether DoubleTab is allowed. ///True if the SystemGesture matches an allowed gesture. False otherwise. internal static bool IsValidSystemGesture(SystemGesture systemGesture, bool allowFlick, bool allowDoubleTap) { switch (systemGesture) { case SystemGesture.None: case SystemGesture.Tap: case SystemGesture.RightTap: case SystemGesture.Drag: case SystemGesture.RightDrag: case SystemGesture.HoldEnter: case SystemGesture.HoldLeave: case SystemGesture.HoverEnter: case SystemGesture.HoverLeave: case SystemGesture.TwoFingerTap: #if ROLLOVER_IMPLEMENTED case SystemGesture.Rollover: #endif return true; case SystemGesture.Flick: return allowFlick; case InternalSystemGestureDoubleTap: return allowDoubleTap; default: return false; } } ///////////////////////////////////////////////////////////////////// ////// Constructs an instance of the RawStylusSystemGestureInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// PenContext that generated this event. /// /// /// tablet id. /// /// /// Stylus device id. /// /// /// System Gesture. /// /// /// X location of the system gesture (in tablet device coordindates). /// /// /// Y location of the system gesture (in tablet device coordindates). /// /// /// Button state info data. /// ////// Critical:This handles critical data in the form of PresentationSource /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusSystemGestureInputReport( InputMode mode, int timestamp, PresentationSource inputSource, PenContext penContext, int tabletId, int stylusDeviceId, SystemGesture systemGesture, int gestureX, int gestureY, int buttonState) : base( mode, timestamp, inputSource, penContext, RawStylusActions.SystemGesture, tabletId, stylusDeviceId, new int[] {}) { if (!RawStylusSystemGestureInputReport.IsValidSystemGesture(systemGesture, true, true)) { throw new InvalidEnumArgumentException(SR.Get( SRID.Enum_Invalid, "systemGesture")); } _id = systemGesture; _gestureX = gestureX; _gestureY = gestureY; _buttonState = buttonState; } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the stylus gesture id. /// internal SystemGesture SystemGesture { get { return _id; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the X location of the system gesture /// in tablet device coordinates. /// internal int GestureX { get { return _gestureX; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the Y location of the system gesture /// in tablet device coordinates. /// internal int GestureY { get { return _gestureY; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the button state info (useful for flicks). /// internal int ButtonState { get { return _buttonState; } } ///////////////////////////////////////////////////////////////////// SystemGesture _id; int _gestureX;// gesture location in tablet device coordinates int _gestureY; int _buttonState; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
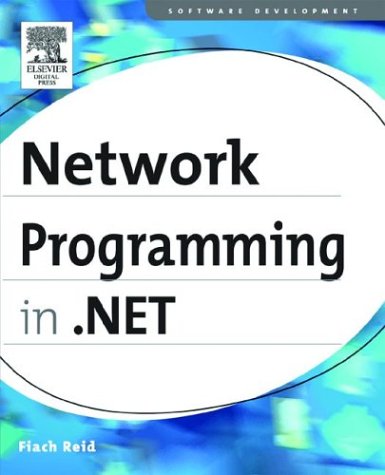
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- login.cs
- TemplateInstanceAttribute.cs
- DetectRunnableInstancesTask.cs
- ControlCollection.cs
- RawUIStateInputReport.cs
- HtmlGenericControl.cs
- BodyGlyph.cs
- DispatcherTimer.cs
- SettingsBindableAttribute.cs
- COAUTHINFO.cs
- AssociationEndMember.cs
- ConversionContext.cs
- MdiWindowListItemConverter.cs
- ClientSettingsProvider.cs
- DecimalKeyFrameCollection.cs
- UIElementParaClient.cs
- ItemTypeToolStripMenuItem.cs
- diagnosticsswitches.cs
- SqlNotificationRequest.cs
- Random.cs
- PersonalizableAttribute.cs
- FreezableCollection.cs
- ReliableChannelBinder.cs
- SelectionProviderWrapper.cs
- UnderstoodHeaders.cs
- ExpressionList.cs
- Enlistment.cs
- InputReport.cs
- ProcessHostServerConfig.cs
- UnsafeNativeMethods.cs
- SqlCacheDependency.cs
- PinnedBufferMemoryStream.cs
- ScaleTransform.cs
- Rule.cs
- DataGridViewCellPaintingEventArgs.cs
- ProfileInfo.cs
- SmiRequestExecutor.cs
- ObjectItemConventionAssemblyLoader.cs
- OleDbRowUpdatingEvent.cs
- MethodBody.cs
- ExpressionTextBoxAutomationPeer.cs
- VirtualizingPanel.cs
- HtmlInputImage.cs
- LifetimeServices.cs
- EntityCollection.cs
- IdentityModelStringsVersion1.cs
- RoutingBehavior.cs
- ScriptReference.cs
- Membership.cs
- ConvertEvent.cs
- TreeWalkHelper.cs
- Fx.cs
- HtmlHead.cs
- HtmlElementErrorEventArgs.cs
- DebugControllerThread.cs
- ModelItemCollection.cs
- TrackBar.cs
- ArrayTypeMismatchException.cs
- ForwardPositionQuery.cs
- Maps.cs
- ObjectDataSourceChooseMethodsPanel.cs
- XPathDocument.cs
- SecurityManager.cs
- DefaultValueAttribute.cs
- IPPacketInformation.cs
- RequestResponse.cs
- RuleSettingsCollection.cs
- DataRecord.cs
- ScrollViewer.cs
- XmlAttributeHolder.cs
- NetworkAddressChange.cs
- CommonObjectSecurity.cs
- SafeNativeMethods.cs
- CriticalFinalizerObject.cs
- GenericTextProperties.cs
- DateTimeStorage.cs
- SpeakCompletedEventArgs.cs
- SmiConnection.cs
- JavaScriptObjectDeserializer.cs
- XmlWriter.cs
- Guid.cs
- IndentedTextWriter.cs
- XmlEventCache.cs
- SpecialFolderEnumConverter.cs
- PageThemeBuildProvider.cs
- XmlQueryType.cs
- ImageCodecInfo.cs
- StorageEntityContainerMapping.cs
- SQLRoleProvider.cs
- SourceInterpreter.cs
- Matrix3D.cs
- RecordManager.cs
- DesignerActionUIService.cs
- EntityDataSourceStatementEditor.cs
- TableStyle.cs
- DictionarySectionHandler.cs
- Part.cs
- SafeLibraryHandle.cs
- TypefaceMetricsCache.cs
- RSAOAEPKeyExchangeDeformatter.cs