Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Utility / Maps.cs / 1305600 / Maps.cs
/****************************************************************************\ * * File: Maps.cs * * Description: * Contains specialized data structures for mapping a key to data. * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Windows; namespace MS.Utility { /***************************************************************************\ ***************************************************************************** * * DTypeMap (DType --> Object) * * Maps the first N used DependencyObject-derived types via an array * (low constant time lookup) for mapping. After which falls back on a * hash table. * * - Fastest gets and sets (normally single array access). * - Large memory footprint. * * Starting mapping is all map to null * ***************************************************************************** \***************************************************************************/ using MS.Internal.PresentationCore; [FriendAccessAllowed] // Built into Core, also used by Framework. internal class DTypeMap { public DTypeMap(int entryCount) { // Constant Time Lookup entries (array size) _entryCount = entryCount; _entries = new object[_entryCount]; _activeDTypes = new ItemStructList(128); } public object this[DependencyObjectType dType] { get { if (dType.Id < _entryCount) { return _entries[dType.Id]; } else { if (_overFlow != null) { return _overFlow[dType]; } return null; } } set { if (dType.Id < _entryCount) { _entries[dType.Id] = value; } else { if (_overFlow == null) { _overFlow = new Hashtable(); } _overFlow[dType] = value; } _activeDTypes.Add(dType); } } // Return list of non-null DType mappings public ItemStructList ActiveDTypes { get { return _activeDTypes; } } // Clear the data-structures to be able to start over public void Clear() { for (int i=0; i<_entryCount; i++) { _entries[i] = null; } for (int i=0; i<_activeDTypes.Count; i++) { _activeDTypes.List[i] = null; } if (_overFlow != null) { _overFlow.Clear(); } } private int _entryCount; private object[] _entries; private Hashtable _overFlow; private ItemStructList _activeDTypes; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: Maps.cs * * Description: * Contains specialized data structures for mapping a key to data. * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Windows; namespace MS.Utility { /***************************************************************************\ ***************************************************************************** * * DTypeMap (DType --> Object) * * Maps the first N used DependencyObject-derived types via an array * (low constant time lookup) for mapping. After which falls back on a * hash table. * * - Fastest gets and sets (normally single array access). * - Large memory footprint. * * Starting mapping is all map to null * ***************************************************************************** \***************************************************************************/ using MS.Internal.PresentationCore; [FriendAccessAllowed] // Built into Core, also used by Framework. internal class DTypeMap { public DTypeMap(int entryCount) { // Constant Time Lookup entries (array size) _entryCount = entryCount; _entries = new object[_entryCount]; _activeDTypes = new ItemStructList (128); } public object this[DependencyObjectType dType] { get { if (dType.Id < _entryCount) { return _entries[dType.Id]; } else { if (_overFlow != null) { return _overFlow[dType]; } return null; } } set { if (dType.Id < _entryCount) { _entries[dType.Id] = value; } else { if (_overFlow == null) { _overFlow = new Hashtable(); } _overFlow[dType] = value; } _activeDTypes.Add(dType); } } // Return list of non-null DType mappings public ItemStructList ActiveDTypes { get { return _activeDTypes; } } // Clear the data-structures to be able to start over public void Clear() { for (int i=0; i<_entryCount; i++) { _entries[i] = null; } for (int i=0; i<_activeDTypes.Count; i++) { _activeDTypes.List[i] = null; } if (_overFlow != null) { _overFlow.Clear(); } } private int _entryCount; private object[] _entries; private Hashtable _overFlow; private ItemStructList _activeDTypes; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
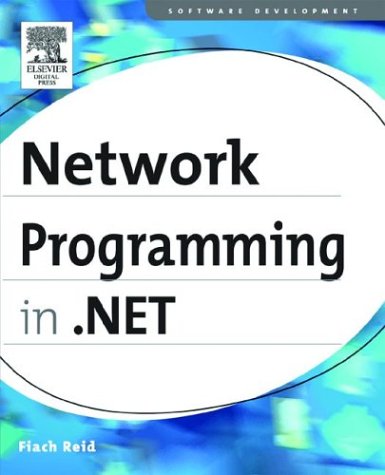
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileDialogCustomPlaces.cs
- KeyedByTypeCollection.cs
- XPathNodeHelper.cs
- TextStore.cs
- EnumBuilder.cs
- LeaseManager.cs
- SiteMembershipCondition.cs
- HttpConfigurationContext.cs
- DataColumnMapping.cs
- LiteralSubsegment.cs
- MemoryFailPoint.cs
- PropertyMetadata.cs
- ArrangedElement.cs
- CodeDirectoryCompiler.cs
- ColumnBinding.cs
- InputReport.cs
- TileModeValidation.cs
- GradientBrush.cs
- PasswordBox.cs
- ContextProperty.cs
- TaskDesigner.cs
- SqlDataSourceStatusEventArgs.cs
- DefaultProxySection.cs
- ProfileBuildProvider.cs
- MultilineStringConverter.cs
- XmlTextAttribute.cs
- ServiceAuthorizationElement.cs
- Pipe.cs
- RayMeshGeometry3DHitTestResult.cs
- DeflateStream.cs
- FontDialog.cs
- TextBoxBase.cs
- MediaElement.cs
- TextBox.cs
- SizeValueSerializer.cs
- PostBackTrigger.cs
- VirtualPath.cs
- HtmlWindow.cs
- RbTree.cs
- JournalNavigationScope.cs
- Gdiplus.cs
- ISFClipboardData.cs
- KeyInterop.cs
- FormViewInsertedEventArgs.cs
- DispatcherHooks.cs
- Int64Converter.cs
- UnauthorizedWebPart.cs
- TrackingMemoryStreamFactory.cs
- RelatedPropertyManager.cs
- SqlDataSource.cs
- DisplayToken.cs
- newitemfactory.cs
- CommandField.cs
- StorageConditionPropertyMapping.cs
- DynamicDocumentPaginator.cs
- BitmapMetadataBlob.cs
- MultiPageTextView.cs
- FilteredReadOnlyMetadataCollection.cs
- DesignerOptionService.cs
- SiteMapNodeCollection.cs
- GridSplitter.cs
- TaskFileService.cs
- PartialArray.cs
- MembershipSection.cs
- URIFormatException.cs
- OrderedDictionary.cs
- TrackingMemoryStream.cs
- MiniConstructorInfo.cs
- PropertyFilterAttribute.cs
- listitem.cs
- MetadataArtifactLoader.cs
- SplitterCancelEvent.cs
- ViewUtilities.cs
- __Filters.cs
- DoubleAnimationBase.cs
- AccessViolationException.cs
- RangeValidator.cs
- MachinePropertyVariants.cs
- KnownBoxes.cs
- SystemException.cs
- CodeDOMProvider.cs
- PartialList.cs
- FactoryRecord.cs
- indexingfiltermarshaler.cs
- filewebresponse.cs
- TypeCollectionDesigner.xaml.cs
- IncrementalReadDecoders.cs
- UdpRetransmissionSettings.cs
- MimeTypeMapper.cs
- WindowCollection.cs
- OleDbMetaDataFactory.cs
- Identity.cs
- ContentType.cs
- LayoutEngine.cs
- ChannelServices.cs
- MetafileEditor.cs
- WindowsListViewItemStartMenu.cs
- indexingfiltermarshaler.cs
- LoadMessageLogger.cs
- SqlConnectionStringBuilder.cs