Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / PixelFormats.cs / 1305600 / PixelFormats.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: PixelFormats.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media { #region PixelFormats ////// PixelFormats - The collection of supported Pixel Formats /// public static class PixelFormats { ////// Default: for situations when the pixel format may not be important /// public static PixelFormat Default { get { return new PixelFormat(PixelFormatEnum.Default); } } ////// Indexed1: Paletted image with 2 colors. /// public static PixelFormat Indexed1 { get { return new PixelFormat(PixelFormatEnum.Indexed1); } } ////// Indexed2: Paletted image with 4 colors. /// public static PixelFormat Indexed2 { get { return new PixelFormat(PixelFormatEnum.Indexed2); } } ////// Indexed4: Paletted image with 16 colors. /// public static PixelFormat Indexed4 { get { return new PixelFormat(PixelFormatEnum.Indexed4); } } ////// Indexed8: Paletted image with 256 colors. /// public static PixelFormat Indexed8 { get { return new PixelFormat(PixelFormatEnum.Indexed8); } } ////// BlackWhite: Monochrome, 2-color image, black and white only. /// public static PixelFormat BlackWhite { get { return new PixelFormat(PixelFormatEnum.BlackWhite); } } ////// Gray2: Image with 4 shades of gray /// public static PixelFormat Gray2 { get { return new PixelFormat(PixelFormatEnum.Gray2); } } ////// Gray4: Image with 16 shades of gray /// public static PixelFormat Gray4 { get { return new PixelFormat(PixelFormatEnum.Gray4); } } ////// Gray8: Image with 256 shades of gray /// public static PixelFormat Gray8 { get { return new PixelFormat(PixelFormatEnum.Gray8); } } ////// Bgr555: 16 bpp SRGB format /// public static PixelFormat Bgr555 { get { return new PixelFormat(PixelFormatEnum.Bgr555); } } ////// Bgr565: 16 bpp SRGB format /// public static PixelFormat Bgr565 { get { return new PixelFormat(PixelFormatEnum.Bgr565); } } ////// Rgb128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb128Float { get { return new PixelFormat(PixelFormatEnum.Rgb128Float); } } ////// Bgr24: 24 bpp SRGB format /// public static PixelFormat Bgr24 { get { return new PixelFormat(PixelFormatEnum.Bgr24); } } ////// Rgb24: 24 bpp SRGB format /// public static PixelFormat Rgb24 { get { return new PixelFormat(PixelFormatEnum.Rgb24); } } ////// Bgr101010: 32 bpp SRGB format /// public static PixelFormat Bgr101010 { get { return new PixelFormat(PixelFormatEnum.Bgr101010); } } ////// Bgr32: 32 bpp SRGB format /// public static PixelFormat Bgr32 { get { return new PixelFormat(PixelFormatEnum.Bgr32); } } ////// Bgra32: 32 bpp SRGB format /// public static PixelFormat Bgra32 { get { return new PixelFormat(PixelFormatEnum.Bgra32); } } ////// Pbgra32: 32 bpp SRGB format /// public static PixelFormat Pbgra32 { get { return new PixelFormat(PixelFormatEnum.Pbgra32); } } ////// Rgb48: 48 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb48 { get { return new PixelFormat(PixelFormatEnum.Rgb48); } } ////// Rgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba64 { get { return new PixelFormat(PixelFormatEnum.Rgba64); } } ////// Prgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba64 { get { return new PixelFormat(PixelFormatEnum.Prgba64); } } ////// Gray16: 16 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray16 { get { return new PixelFormat(PixelFormatEnum.Gray16); } } ////// Gray32Float: 32 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray32Float { get { return new PixelFormat(PixelFormatEnum.Gray32Float); } } ////// Rgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba128Float { get { return new PixelFormat(PixelFormatEnum.Rgba128Float); } } ////// Prgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba128Float { get { return new PixelFormat(PixelFormatEnum.Prgba128Float); } } ////// Cmyk32: 32 bpp format /// public static PixelFormat Cmyk32 { get { return new PixelFormat(PixelFormatEnum.Cmyk32); } } } #endregion // PixelFormats } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: PixelFormats.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media { #region PixelFormats ////// PixelFormats - The collection of supported Pixel Formats /// public static class PixelFormats { ////// Default: for situations when the pixel format may not be important /// public static PixelFormat Default { get { return new PixelFormat(PixelFormatEnum.Default); } } ////// Indexed1: Paletted image with 2 colors. /// public static PixelFormat Indexed1 { get { return new PixelFormat(PixelFormatEnum.Indexed1); } } ////// Indexed2: Paletted image with 4 colors. /// public static PixelFormat Indexed2 { get { return new PixelFormat(PixelFormatEnum.Indexed2); } } ////// Indexed4: Paletted image with 16 colors. /// public static PixelFormat Indexed4 { get { return new PixelFormat(PixelFormatEnum.Indexed4); } } ////// Indexed8: Paletted image with 256 colors. /// public static PixelFormat Indexed8 { get { return new PixelFormat(PixelFormatEnum.Indexed8); } } ////// BlackWhite: Monochrome, 2-color image, black and white only. /// public static PixelFormat BlackWhite { get { return new PixelFormat(PixelFormatEnum.BlackWhite); } } ////// Gray2: Image with 4 shades of gray /// public static PixelFormat Gray2 { get { return new PixelFormat(PixelFormatEnum.Gray2); } } ////// Gray4: Image with 16 shades of gray /// public static PixelFormat Gray4 { get { return new PixelFormat(PixelFormatEnum.Gray4); } } ////// Gray8: Image with 256 shades of gray /// public static PixelFormat Gray8 { get { return new PixelFormat(PixelFormatEnum.Gray8); } } ////// Bgr555: 16 bpp SRGB format /// public static PixelFormat Bgr555 { get { return new PixelFormat(PixelFormatEnum.Bgr555); } } ////// Bgr565: 16 bpp SRGB format /// public static PixelFormat Bgr565 { get { return new PixelFormat(PixelFormatEnum.Bgr565); } } ////// Rgb128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb128Float { get { return new PixelFormat(PixelFormatEnum.Rgb128Float); } } ////// Bgr24: 24 bpp SRGB format /// public static PixelFormat Bgr24 { get { return new PixelFormat(PixelFormatEnum.Bgr24); } } ////// Rgb24: 24 bpp SRGB format /// public static PixelFormat Rgb24 { get { return new PixelFormat(PixelFormatEnum.Rgb24); } } ////// Bgr101010: 32 bpp SRGB format /// public static PixelFormat Bgr101010 { get { return new PixelFormat(PixelFormatEnum.Bgr101010); } } ////// Bgr32: 32 bpp SRGB format /// public static PixelFormat Bgr32 { get { return new PixelFormat(PixelFormatEnum.Bgr32); } } ////// Bgra32: 32 bpp SRGB format /// public static PixelFormat Bgra32 { get { return new PixelFormat(PixelFormatEnum.Bgra32); } } ////// Pbgra32: 32 bpp SRGB format /// public static PixelFormat Pbgra32 { get { return new PixelFormat(PixelFormatEnum.Pbgra32); } } ////// Rgb48: 48 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb48 { get { return new PixelFormat(PixelFormatEnum.Rgb48); } } ////// Rgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba64 { get { return new PixelFormat(PixelFormatEnum.Rgba64); } } ////// Prgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba64 { get { return new PixelFormat(PixelFormatEnum.Prgba64); } } ////// Gray16: 16 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray16 { get { return new PixelFormat(PixelFormatEnum.Gray16); } } ////// Gray32Float: 32 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray32Float { get { return new PixelFormat(PixelFormatEnum.Gray32Float); } } ////// Rgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba128Float { get { return new PixelFormat(PixelFormatEnum.Rgba128Float); } } ////// Prgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba128Float { get { return new PixelFormat(PixelFormatEnum.Prgba128Float); } } ////// Cmyk32: 32 bpp format /// public static PixelFormat Cmyk32 { get { return new PixelFormat(PixelFormatEnum.Cmyk32); } } } #endregion // PixelFormats } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
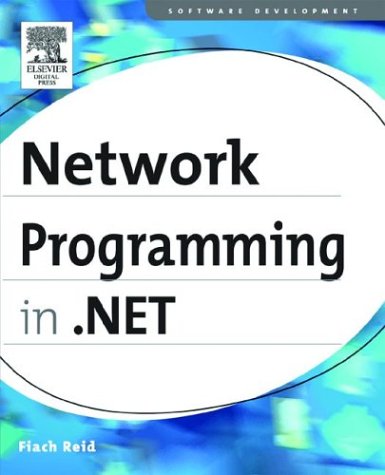
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileFormatException.cs
- Hyperlink.cs
- HtmlEncodedRawTextWriter.cs
- CalloutQueueItem.cs
- DataGridViewColumnHeaderCell.cs
- MenuItemBinding.cs
- DataControlFieldCollection.cs
- __Error.cs
- DesignerCategoryAttribute.cs
- SystemParameters.cs
- ListViewItemEventArgs.cs
- BezierSegment.cs
- UnsafeNativeMethods.cs
- localization.cs
- ManagementBaseObject.cs
- ObjectMaterializedEventArgs.cs
- DataTrigger.cs
- WSHttpBindingBase.cs
- DataGridViewCellEventArgs.cs
- DataViewManagerListItemTypeDescriptor.cs
- DatatypeImplementation.cs
- BooleanToVisibilityConverter.cs
- BitVector32.cs
- ProcessHostMapPath.cs
- Font.cs
- InkCollectionBehavior.cs
- ConstructorExpr.cs
- WebScriptEndpoint.cs
- SqlBinder.cs
- WeakKeyDictionary.cs
- PassportPrincipal.cs
- SecurityAccessDeniedException.cs
- CheckBoxPopupAdapter.cs
- TraceUtility.cs
- ClearCollection.cs
- DbQueryCommandTree.cs
- NotifyIcon.cs
- PointKeyFrameCollection.cs
- ControlValuePropertyAttribute.cs
- XMLSyntaxException.cs
- SecurityIdentifierElement.cs
- ConfigurationLockCollection.cs
- SharedPerformanceCounter.cs
- ProtocolViolationException.cs
- FontStretch.cs
- FunctionQuery.cs
- XmlLoader.cs
- XmlDataSourceView.cs
- Thumb.cs
- ArgIterator.cs
- ProfileEventArgs.cs
- BadImageFormatException.cs
- FlagsAttribute.cs
- StackSpiller.Temps.cs
- EventHandlersStore.cs
- XmlMapping.cs
- NamedObject.cs
- QueryAccessibilityHelpEvent.cs
- HttpListenerResponse.cs
- MethodBuilder.cs
- UnsafeMethods.cs
- ReverseInheritProperty.cs
- InputMethod.cs
- UxThemeWrapper.cs
- ConditionBrowserDialog.cs
- TextSelection.cs
- TTSEvent.cs
- ConstantProjectedSlot.cs
- LowerCaseStringConverter.cs
- PropertyChangedEventArgs.cs
- DataGridViewRowsAddedEventArgs.cs
- RsaElement.cs
- ValueProviderWrapper.cs
- SimpleWebHandlerParser.cs
- EncoderFallback.cs
- LightweightCodeGenerator.cs
- PrintingPermissionAttribute.cs
- RegistryKey.cs
- FastEncoderWindow.cs
- TreeNodeStyleCollection.cs
- TextTreeObjectNode.cs
- LabelDesigner.cs
- ServicePointManagerElement.cs
- WindowsTooltip.cs
- RotateTransform.cs
- _NegoState.cs
- DelegateBodyWriter.cs
- APCustomTypeDescriptor.cs
- OleStrCAMarshaler.cs
- StylusPoint.cs
- QuaternionAnimation.cs
- StylusPointProperty.cs
- Win32.cs
- PhonemeConverter.cs
- DataBoundLiteralControl.cs
- VectorConverter.cs
- Policy.cs
- TrackingRecord.cs
- GridViewRowPresenter.cs
- OdbcTransaction.cs