Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / ColorTypeConverter.cs / 1 / ColorTypeConverter.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: ColorTypeConverter.cs Abstract: This file implements the ColorTypeConverter used by the Xps Serialization APIs for serializing colors to a Xps package. Author: [....] ([....]) Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.Collections.Generic; using System.ComponentModel; using System.IO; using System.Text; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Xps.Packaging; namespace System.Windows.Xps.Serialization { ////// This class implements a type converter for converting /// Color to Uris. It handles the writing of the Color Context /// to a Xps package and returns a package URI to the /// caller. It also handles reading an Color Context from a /// Xps package given a Uri. /// public class ColorTypeConverter : ExpandableObjectConverter { #region Public overrides for ExpandableObjectConverted ////// Returns whether this converter can convert an object /// of the given type to the type of this converter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A Type that represents the type you want to convert from. /// ////// true if this converter can perform the conversion; /// otherwise, false. /// public override bool CanConvertFrom( ITypeDescriptorContext context, Type sourceType ) { return IsSupportedType(sourceType); } ////// Returns whether this converter can convert the object /// to the specified type. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A Type that represents the type you want to convert to. /// ////// true if this converter can perform the conversion; /// otherwise, false. /// public override bool CanConvertTo( ITypeDescriptorContext context, Type destinationType ) { return IsSupportedType(destinationType); } ////// Converts the given value to the type of this converter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// The CultureInfo to use as the current culture. /// /// /// The Object to convert. /// ////// An Object that represents the converted value. /// public override object ConvertFrom( ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value ) { if( value == null ) { throw new ArgumentNullException("value"); } if (!IsSupportedType(value.GetType())) { throw new NotSupportedException(ReachSR.Get(ReachSRID.Converter_ConvertFromNotSupported)); } throw new NotImplementedException(); } ////// Converts the given value object to the specified type, /// using the arguments. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A CultureInfo object. If null is passed, the current /// culture is assumed. /// /// /// The Object to convert. /// /// /// The Type to convert the value parameter to. /// ////// The Type to convert the value parameter to. /// public override object ConvertTo( ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType ) { if (!IsSupportedType(destinationType)) { throw new NotSupportedException(ReachSR.Get(ReachSRID.Converter_ConvertToNotSupported)); } Color color = (Color)value; string colorString; if (color.ColorContext == null) { colorString = color.ToString(culture); if (colorString.StartsWith("sc#", StringComparison.Ordinal) && colorString.Contains("E")) { // // Fix bug 1588888: Serialization produces non-compliant scRGB color string. // // Avalon Color.ToString() will use "R"oundtrip formatting specifier for scRGB colors, // which may produce numbers in scientific notation, which is invalid XPS. // // Fix: We detect such colors and format with "F"ixed specifier. We don't always use F // in order to properly handle RGB colors. // IFormattable formattableColor = (IFormattable)color; colorString = formattableColor.ToString("F", culture); } } else { string uriString = SerializeColorContext(context, color.ColorContext); StringBuilder sb = new StringBuilder(); IFormatProvider provider = culture; char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); sb.AppendFormat(provider, "ContextColor {0} ", uriString); sb.AppendFormat(provider, "{1:R}{0}", separator, color.ScA); for (int i = 0; i < color.GetNativeColorValues().GetLength(0); ++i) { sb.AppendFormat(provider, "{0:R}", color.GetNativeColorValues()[i]); if (i < color.GetNativeColorValues().GetLength(0) - 1) { sb.AppendFormat(provider, "{0}", separator); } } colorString = sb.ToString(); } return colorString; } ////// Gets a collection of properties for the type of object /// specified by the value parameter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// An Object that specifies the type of object to get the /// properties for. /// /// /// An array of type Attribute that will be used as a filter. /// ////// A PropertyDescriptorCollection with the properties that are /// exposed for the component, or null if there are no properties. /// public override PropertyDescriptorCollection GetProperties( ITypeDescriptorContext context, object value, Attribute[] attributes ) { throw new NotImplementedException(); } #endregion Public overrides for ExpandableObjectConverted #region Private static helper methods ////// Looks up the type in a table to determine /// whether this type is supported by this /// class. /// /// /// Type to lookup in table. /// ////// True is supported; otherwise false. /// private static bool IsSupportedType( Type type ) { bool isSupported = false; foreach (Type t in SupportedTargetTypes) { if (t.Equals(type)) { isSupported = true; break; } } return isSupported; } #endregion Private static helper methods #region Public static helper methods ////// Serializes a ColorContext to the package and returns it Uri /// /// /// Type descriptor context /// /// /// ColorContext /// ////// string containing the profileUri /// public static string SerializeColorContext( IServiceProvider context, ColorContext colorContext ) { Uri profileUri = null; if( colorContext == null ) { throw new ArgumentNullException("colorContext"); } if ( context!= null ) { PackageSerializationManager manager = (PackageSerializationManager)context.GetService(typeof(XpsSerializationManager)); DictionarycolorContextTable = manager.ResourcePolicy.ColorContextTable; Dictionary currentPageColorContextTable = manager.ResourcePolicy.CurrentPageColorContextTable; if(currentPageColorContextTable==null) { // // throw the appropriae exception // } if(colorContextTable==null) { // // throw the appropriae exception // } if (colorContextTable.ContainsKey(colorContext.GetHashCode())) { // // The colorContext has already been cached (and therefore serialized). // No need to serialize it again so we just use the Uri in the // package where the original was serialized. For that Uri used // a relationship is only created if this has not been included on // the current page before. // profileUri = colorContextTable[colorContext.GetHashCode()]; if (!currentPageColorContextTable.ContainsKey(colorContext.GetHashCode())) { // // Also, add a relationship for the current page to this Color Context // resource. This is needed to conform with Xps specification. // manager.AddRelationshipToCurrentPage(profileUri, XpsS0Markup.ResourceRelationshipName); currentPageColorContextTable.Add(colorContext.GetHashCode(), profileUri); } } else { MS.Internal.ContentType colorContextMimeType = XpsS0Markup.ColorContextContentType; XpsResourceStream resourceStream = manager.AcquireResourceStream(typeof(ColorContext), colorContextMimeType.ToString()); byte [] buffer = new byte[512]; Stream profileStream = colorContext.OpenProfileStream(); int count; while ( (count = profileStream.Read( buffer, 0, buffer.GetLength(0)) ) > 0 ) { resourceStream.Stream.Write(buffer,0,count); } profileStream.Close(); // // Make sure to commit the resource stream by releasing it. // profileUri = resourceStream.Uri; manager.ReleaseResourceStream(typeof(ColorContext)); colorContextTable.Add(colorContext.GetHashCode(), profileUri); currentPageColorContextTable.Add(colorContext.GetHashCode(), profileUri); } } else // Testing only { profileUri = colorContext.ProfileUri; } //First Step make sure that nothing that should not be escaped is escaped Uri safeUnescapedUri = new Uri(profileUri.GetComponents(UriComponents.SerializationInfoString, UriFormat.SafeUnescaped), profileUri.IsAbsoluteUri ? UriKind.Absolute : UriKind.Relative); //Second Step make sure that everything that should escaped is escaped String uriString = safeUnescapedUri.GetComponents(UriComponents.SerializationInfoString, UriFormat.UriEscaped); return uriString; } #endregion Public static helper methods #region Private static data /// /// A table of supported types for this type converter /// private static Type[] SupportedTargetTypes = { typeof(string) }; #endregion Private static data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
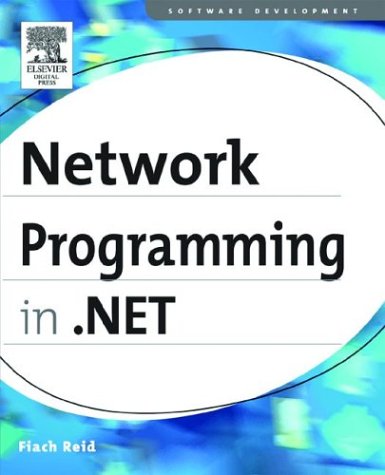
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PopOutPanel.cs
- ValueUtilsSmi.cs
- SafeRightsManagementQueryHandle.cs
- COM2PropertyDescriptor.cs
- WebPartZone.cs
- TemplatingOptionsDialog.cs
- __Filters.cs
- ReaderOutput.cs
- SchemaImporterExtensionElement.cs
- HandlerBase.cs
- ColumnMapCopier.cs
- LoginName.cs
- EdmSchemaError.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- AmbientValueAttribute.cs
- MemberMemberBinding.cs
- RectAnimationClockResource.cs
- DesignTimeVisibleAttribute.cs
- Automation.cs
- BrushValueSerializer.cs
- FieldToken.cs
- DbConnectionStringBuilder.cs
- JsonFormatGeneratorStatics.cs
- XmlSerializerNamespaces.cs
- SlotInfo.cs
- CurrentChangingEventArgs.cs
- BrowserTree.cs
- RadioButton.cs
- UdpDuplexChannel.cs
- ByteRangeDownloader.cs
- LocationUpdates.cs
- ConfigXmlWhitespace.cs
- ProtocolsConfiguration.cs
- MeshGeometry3D.cs
- ToolStripItemImageRenderEventArgs.cs
- TextBlockAutomationPeer.cs
- AdapterUtil.cs
- DbRetry.cs
- SiteMapNodeItemEventArgs.cs
- PolyLineSegment.cs
- Pts.cs
- XmlQueryRuntime.cs
- CompositeDataBoundControl.cs
- LOSFormatter.cs
- ResponseStream.cs
- DataViewManager.cs
- FrameworkElementAutomationPeer.cs
- ToolStripDropDownButton.cs
- ObjectDataSourceDisposingEventArgs.cs
- MessageBox.cs
- ProfileService.cs
- OrderByQueryOptionExpression.cs
- Point3D.cs
- Visitors.cs
- RequestStatusBarUpdateEventArgs.cs
- ObjectDataSourceMethodEditor.cs
- Size3DValueSerializer.cs
- ExpressionTextBoxAutomationPeer.cs
- StateWorkerRequest.cs
- SingleStorage.cs
- KeyInfo.cs
- _BufferOffsetSize.cs
- XamlInt32CollectionSerializer.cs
- DataServiceQuery.cs
- SafeFindHandle.cs
- CompositeScriptReferenceEventArgs.cs
- BrowserDefinitionCollection.cs
- LocalizationComments.cs
- DataReceivedEventArgs.cs
- DesignerSerializerAttribute.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- GridViewCellAutomationPeer.cs
- InvokeGenerator.cs
- VisualStateChangedEventArgs.cs
- AliasGenerator.cs
- Internal.cs
- UniqueEventHelper.cs
- ScriptBehaviorDescriptor.cs
- SQLBoolean.cs
- SatelliteContractVersionAttribute.cs
- DragEventArgs.cs
- RtfToXamlReader.cs
- SqlMethods.cs
- RootBuilder.cs
- AuthorizationRule.cs
- SID.cs
- EnumerableCollectionView.cs
- SoapMessage.cs
- RequestQueryParser.cs
- UpDownEvent.cs
- UIAgentMonitor.cs
- GridViewColumnHeaderAutomationPeer.cs
- ControlCollection.cs
- DecimalConverter.cs
- DNS.cs
- ErrorInfoXmlDocument.cs
- BulletChrome.cs
- embossbitmapeffect.cs
- CompositeTypefaceMetrics.cs
- FillRuleValidation.cs