Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Text / TextDpi.cs / 1305600 / TextDpi.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TextDpi.cs // // Description: Helpers to handle text dpi conversions and limitations. // // History: // 04/25/2003 : [....] - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; // Double, ... using System.Windows; // DependencyObject using System.Windows.Documents; // TextElement, ... using System.Windows.Media; // FontFamily using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS namespace MS.Internal.Text { // --------------------------------------------------------------------- // Helpers to handle text dpi conversions and limitations. // --------------------------------------------------------------------- internal static class TextDpi { // ------------------------------------------------------------------ // Minimum width for text measurement. // ----------------------------------------------------------------- internal static double MinWidth { get { return _minSize; } } // ------------------------------------------------------------------ // Maximum width for text measurement. // ------------------------------------------------------------------ internal static double MaxWidth { get { return _maxSize; } } // ----------------------------------------------------------------- // Convert from logical measurement unit to text measurement unit. // // d - value in logical measurement unit // // Returns: value in text measurement unit. // ------------------------------------------------------------------ internal static int ToTextDpi(double d) { if (DoubleUtil.IsZero(d)) { return 0; } else if (d > 0) { if (d > _maxSize) { d = _maxSize; } else if (d < _minSize) { d = _minSize; } } else { if (d < -_maxSize) { d = -_maxSize; } else if (d > -_minSize) { d = -_minSize; } } return (int)Math.Round(d * _scale); } // ----------------------------------------------------------------- // Convert from text measurement unit to logical measurement unit. // // i - value in text measurement unit // // Returns: value in logical measurement unit. // ----------------------------------------------------------------- internal static double FromTextDpi(int i) { return ((double)i) / _scale; } // ----------------------------------------------------------------- // Convert point from logical measurement unit to text measurement unit. // ------------------------------------------------------------------ internal static PTS.FSPOINT ToTextPoint(Point point) { PTS.FSPOINT fspoint = new PTS.FSPOINT(); fspoint.u = ToTextDpi(point.X); fspoint.v = ToTextDpi(point.Y); return fspoint; } // ----------------------------------------------------------------- // Convert size from logical measurement unit to text measurement unit. // ------------------------------------------------------------------ internal static PTS.FSVECTOR ToTextSize(Size size) { PTS.FSVECTOR fsvector = new PTS.FSVECTOR(); fsvector.du = ToTextDpi(size.Width); fsvector.dv = ToTextDpi(size.Height); return fsvector; } // ------------------------------------------------------------------ // Convert rect from text measurement unit to logical measurement unit. // ----------------------------------------------------------------- internal static Rect FromTextRect(PTS.FSRECT fsrect) { return new Rect( FromTextDpi(fsrect.u), FromTextDpi(fsrect.v), FromTextDpi(fsrect.du), FromTextDpi(fsrect.dv)); } // ------------------------------------------------------------------ // Make sure that LS/PTS limitations are not exceeded for offset // within a line. // ----------------------------------------------------------------- internal static void EnsureValidLineOffset(ref double offset) { // Offset has to be > min allowed size && < max allowed size. if (offset > _maxSize) { offset = _maxSize; } else if (offset < -_maxSize) { offset = -_maxSize; } } // ----------------------------------------------------------------- // Snaps the value to TextDPi, makeing sure that convertion to // TextDpi and the to double produces the same value. // ----------------------------------------------------------------- internal static void SnapToTextDpi(ref Size size) { size = new Size(FromTextDpi(ToTextDpi(size.Width)), FromTextDpi(ToTextDpi(size.Height))); } // ------------------------------------------------------------------ // Make sure that LS/PTS limitations are not exceeded for line width. // ----------------------------------------------------------------- internal static void EnsureValidLineWidth(ref double width) { // Line width has to be > 0 && < max allowed size. if (width > _maxSize) { width = _maxSize; } else if (width < _minSize) { width = _minSize; } } internal static void EnsureValidLineWidth(ref Size size) { // Line width has to be > 0 && < max allowed size. if (size.Width > _maxSize) { size.Width = _maxSize; } else if (size.Width < _minSize) { size.Width = _minSize; } } internal static void EnsureValidLineWidth(ref int width) { // Line width has to be > 0 && < max allowed size. if (width > _maxSizeInt) { width = _maxSizeInt; } else if (width < _minSizeInt) { width = _minSizeInt; } } // ------------------------------------------------------------------ // Make sure that PTS limitations are not exceeded for page size. // ------------------------------------------------------------------ internal static void EnsureValidPageSize(ref Size size) { // Page size has to be > 0 && < max allowed size. if (size.Width > _maxSize) { size.Width = _maxSize; } else if (size.Width < _minSize) { size.Width = _minSize; } if (size.Height > _maxSize) { size.Height = _maxSize; } else if (size.Height < _minSize) { size.Height = _minSize; } } internal static void EnsureValidPageWidth(ref double width) { // Page size has to be > 0 && < max allowed size. if (width > _maxSize) { width = _maxSize; } else if (width < _minSize) { width = _minSize; } } internal static void EnsureValidPageMargin(ref Thickness pageMargin, Size pageSize) { if (pageMargin.Left >= pageSize.Width) { pageMargin.Right = 0.0; } if (pageMargin.Left + pageMargin.Right >= pageSize.Width) { pageMargin.Right = Math.Max(0.0, pageSize.Width - pageMargin.Left - _minSize); if (pageMargin.Left + pageMargin.Right >= pageSize.Width) { pageMargin.Left = pageSize.Width - _minSize; } } if (pageMargin.Top >= pageSize.Height) { pageMargin.Bottom = 0.0; } if (pageMargin.Top + pageMargin.Bottom >= pageSize.Height) { pageMargin.Bottom = Math.Max(0.0, pageSize.Height - pageMargin.Top - _minSize);; if (pageMargin.Top + pageMargin.Bottom >= pageSize.Height) { pageMargin.Top = pageSize.Height - _minSize; } } } // ----------------------------------------------------------------- // Make sure that LS/PTS limitations are not exceeded for object's size. // ------------------------------------------------------------------ internal static void EnsureValidObjSize(ref Size size) { // Embedded object can have size == 0, but its width and height // have to be less than max allowed size. if (size.Width > _maxObjSize) { size.Width = _maxObjSize; } if (size.Height > _maxObjSize) { size.Height = _maxObjSize; } } // ----------------------------------------------------------------- // Make sure that LS/PTS limitations are not exceeded. // ----------------------------------------------------------------- static TextDpi() { _scale = 300.0; _maxSizeInt = 0x3ffffffe; _minSizeInt = 0x00000001; _maxSize = ((double)_maxSizeInt) / _scale; // = 3,579,139.40 pixels _minSize = ((double)_minSizeInt) / _scale; // > 0 _maxObjSize = _maxSize / 3; // = 1,193,046.46 pixels } // ----------------------------------------------------------------- // Measuring unit for PTS presenters is int, but logical measuring // for the rest of the system is is double. // Logical measuring dpi = 96 // PTS presenters measuring dpi = 28800 // ------------------------------------------------------------------ private static double _scale; // ----------------------------------------------------------------- // PTS/LS limitation for max size. // ------------------------------------------------------------------ private static int _maxSizeInt; private static double _maxSize; // ------------------------------------------------------------------ // PTS/LS limitation for min size. // ----------------------------------------------------------------- private static int _minSizeInt; private static double _minSize; // ------------------------------------------------------------------ // Embedded object size is limited to 1/3 of max size accepted by LS/PTS. // ----------------------------------------------------------------- private static double _maxObjSize; } #if TEXTLAYOUTDEBUG // --------------------------------------------------------------------- // Pefrormance debugging helpers. // --------------------------------------------------------------------- internal static class TextDebug { // ------------------------------------------------------------------ // Enter the scope and log the message. // ----------------------------------------------------------------- internal static void BeginScope(string name) { Log(name); ++_indent; } // ------------------------------------------------------------------ // Exit the current scope. // ------------------------------------------------------------------ internal static void EndScope() { --_indent; } // ----------------------------------------------------------------- // Log message. // ------------------------------------------------------------------ internal static void Log(string msg) { Console.WriteLine("> " + CurrentIndent + msg); } // ----------------------------------------------------------------- // String representing current indent. // ----------------------------------------------------------------- private static string CurrentIndent { get { return new string(' ', _indent * 2); } } // ----------------------------------------------------------------- // Current indent. // ------------------------------------------------------------------ private static int _indent; } #endif // TEXTLAYOUTDEBUG } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
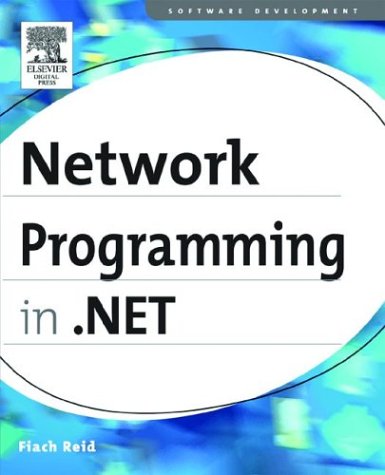
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNodeMouseHoverEvent.cs
- HtmlControlDesigner.cs
- TreeWalker.cs
- XmlConverter.cs
- ListViewGroupItemCollection.cs
- ThumbAutomationPeer.cs
- TemplateBindingExpression.cs
- MenuItem.cs
- columnmapkeybuilder.cs
- DeviceContext.cs
- ClientCredentials.cs
- FileUtil.cs
- WebPartMenuStyle.cs
- HtmlHistory.cs
- httpapplicationstate.cs
- TextRangeEditTables.cs
- RelationalExpressions.cs
- StringAttributeCollection.cs
- SchemaAttDef.cs
- UInt16Storage.cs
- DebugHandleTracker.cs
- Utils.cs
- SafeRightsManagementSessionHandle.cs
- ConsumerConnectionPointCollection.cs
- Point.cs
- ThrowHelper.cs
- TypeNameConverter.cs
- TextLineBreak.cs
- MenuDesigner.cs
- DialogResultConverter.cs
- RuntimeWrappedException.cs
- DataGridLength.cs
- SystemPens.cs
- LayoutEngine.cs
- ChildrenQuery.cs
- ArrangedElementCollection.cs
- GAC.cs
- HMACSHA384.cs
- SqlProvider.cs
- DetailsViewDeletedEventArgs.cs
- PolicyException.cs
- MethodInfo.cs
- LazyTextWriterCreator.cs
- Selection.cs
- Baml2006ReaderSettings.cs
- SqlGenericUtil.cs
- WindowsIPAddress.cs
- ImageConverter.cs
- GcSettings.cs
- KeyTimeConverter.cs
- DataPagerCommandEventArgs.cs
- RuntimeConfigLKG.cs
- WebContext.cs
- NativeMethods.cs
- SyndicationContent.cs
- MailHeaderInfo.cs
- FontNamesConverter.cs
- EventArgs.cs
- SplashScreen.cs
- TextUtf8RawTextWriter.cs
- RegexInterpreter.cs
- CertificateElement.cs
- ListSortDescriptionCollection.cs
- InputBindingCollection.cs
- EventHandlersDesigner.cs
- StrokeIntersection.cs
- ScaleTransform3D.cs
- WebPartEditorApplyVerb.cs
- CorePropertiesFilter.cs
- ListViewDeleteEventArgs.cs
- SqlAggregateChecker.cs
- Crypto.cs
- ImageListImageEditor.cs
- BackgroundWorker.cs
- ServiceParser.cs
- Point3DConverter.cs
- TranslateTransform.cs
- DependencyPropertyDescriptor.cs
- TypeInitializationException.cs
- IIS7UserPrincipal.cs
- ErasingStroke.cs
- SystemResources.cs
- AnnotationAdorner.cs
- CheckPair.cs
- WSDualHttpSecurity.cs
- Material.cs
- TextElementCollectionHelper.cs
- RectAnimationClockResource.cs
- DataGridViewTextBoxEditingControl.cs
- RoleService.cs
- ConstraintStruct.cs
- TextParaClient.cs
- _NegoState.cs
- AsyncContentLoadedEventArgs.cs
- PathTooLongException.cs
- DataListItem.cs
- MarkupCompiler.cs
- UrlMappingsModule.cs
- SecurityToken.cs
- OSFeature.cs