Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / WrapPanel.cs / 1 / WrapPanel.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: WrapPanel.cs // // Description: Contains the WrapPanel class. // Spec at [....]/layout/Specs/WrapPanel.xml // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using System.Windows.Media; using System; namespace System.Windows.Controls { ////// WrapPanel is used to place child UIElements at sequential positions from left to the right /// and then "wrap" the lines of children from top to the bottom. /// /// All children get the layout partition of size ItemWidth x ItemHeight. /// /// public class WrapPanel : Panel { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default constructor /// public WrapPanel() : base() { _orientation = (Orientation) OrientationProperty.GetDefaultValue(DependencyObjectType); } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods #endregion //-------------------------------------------------------------------- // // Public Properties + Dependency Properties's // //-------------------------------------------------------------------- #region Public Properties private static bool IsWidthHeightValid(object value) { double v = (double)value; return (DoubleUtil.IsNaN(v)) || (v >= 0.0d && !Double.IsPositiveInfinity(v)); } ////// DependencyProperty for public static readonly DependencyProperty ItemWidthProperty = DependencyProperty.Register( "ItemWidth", typeof(double), typeof(WrapPanel), new FrameworkPropertyMetadata( Double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsWidthHeightValid)); ///property. /// /// The ItemWidth and ItemHeight properties specify the size of all items in the WrapPanel. /// Note that children of /// WrapPanel may have their own Width/Height properties set - the ItemWidth/ItemHeight /// specifies the size of "layout partition" reserved by WrapPanel for the child. /// If this property is not set (or set to "Auto" in markup or Double.NaN in code) - the size of layout /// partition is equal to DesiredSize of the child element. /// [TypeConverter(typeof(LengthConverter))] public double ItemWidth { get { return (double) GetValue(ItemWidthProperty); } set { SetValue(ItemWidthProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty ItemHeightProperty = DependencyProperty.Register( "ItemHeight", typeof(double), typeof(WrapPanel), new FrameworkPropertyMetadata( Double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsWidthHeightValid)); ///property. /// /// The ItemWidth and ItemHeight properties specify the size of all items in the WrapPanel. /// Note that children of /// WrapPanel may have their own Width/Height properties set - the ItemWidth/ItemHeight /// specifies the size of "layout partition" reserved by WrapPanel for the child. /// If this property is not set (or set to "Auto" in markup or Double.NaN in code) - the size of layout /// partition is equal to DesiredSize of the child element. /// [TypeConverter(typeof(LengthConverter))] public double ItemHeight { get { return (double) GetValue(ItemHeightProperty); } set { SetValue(ItemHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty OrientationProperty = StackPanel.OrientationProperty.AddOwner( typeof(WrapPanel), new FrameworkPropertyMetadata( Orientation.Horizontal, FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnOrientationChanged))); ///property. /// /// Specifies dimension of children positioning in absence of wrapping. /// Wrapping occurs in orthogonal direction. For example, if Orientation is Horizontal, /// the items try to form horizontal rows first and if needed are wrapped and form vertical stack of rows. /// If Orientation is Vertical, items first positioned in a vertical column, and if there is /// not enough space - wrapping creates additional columns in horizontal dimension. /// public Orientation Orientation { get { return _orientation; } set { SetValue(OrientationProperty, value); } } ////// private static void OnOrientationChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { WrapPanel p = (WrapPanel)d; p._orientation = (Orientation) e.NewValue; } private Orientation _orientation; #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods private struct UVSize { internal UVSize (Orientation orientation, double width, double height) { U = V = 0d; _orientation = orientation; Width = width; Height = height; } internal UVSize (Orientation orientation) { U = V = 0d; _orientation = orientation; } internal double U; internal double V; private Orientation _orientation; internal double Width { get { return (_orientation == Orientation.Horizontal ? U : V); } set { if(_orientation == Orientation.Horizontal) U = value; else V = value; } } internal double Height { get { return (_orientation == Orientation.Horizontal ? V : U); } set { if(_orientation == Orientation.Horizontal) V = value; else U = value; } } } ////// /// protected override Size MeasureOverride(Size constraint) { UVSize curLineSize = new UVSize(Orientation); UVSize panelSize = new UVSize(Orientation); UVSize uvConstraint = new UVSize(Orientation, constraint.Width, constraint.Height); double itemWidth = ItemWidth; double itemHeight = ItemHeight; bool itemWidthSet = !DoubleUtil.IsNaN(itemWidth); bool itemHeightSet = !DoubleUtil.IsNaN(itemHeight); Size childConstraint = new Size( (itemWidthSet ? itemWidth : constraint.Width), (itemHeightSet ? itemHeight : constraint.Height)); UIElementCollection children = InternalChildren; for(int i=0, count = children.Count; i/// /// /// protected override Size ArrangeOverride(Size finalSize) { int firstInLine = 0; double itemWidth = ItemWidth; double itemHeight = ItemHeight; double accumulatedV = 0; double itemU = (Orientation == Orientation.Horizontal ? itemWidth : itemHeight); UVSize curLineSize = new UVSize(Orientation); UVSize uvFinalSize = new UVSize(Orientation, finalSize.Width, finalSize.Height); bool itemWidthSet = !DoubleUtil.IsNaN(itemWidth); bool itemHeightSet = !DoubleUtil.IsNaN(itemHeight); bool useItemU = (Orientation == Orientation.Horizontal ? itemWidthSet : itemHeightSet); UIElementCollection children = InternalChildren; for(int i=0, count = children.Count; i
Link Menu
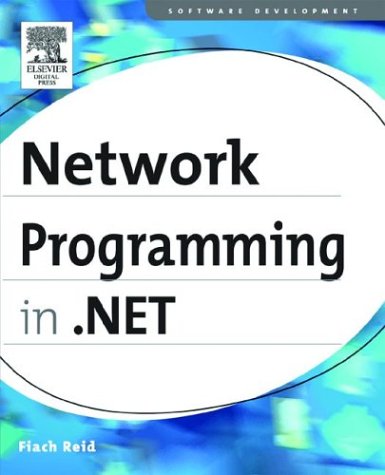
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandlerBase.cs
- DataSourceDesigner.cs
- ConstructorExpr.cs
- LinqDataSourceDisposeEventArgs.cs
- Canonicalizers.cs
- SingleAnimation.cs
- ContentOnlyMessage.cs
- Error.cs
- AutoResetEvent.cs
- CfgArc.cs
- ScriptingJsonSerializationSection.cs
- RedistVersionInfo.cs
- AsymmetricKeyExchangeDeformatter.cs
- RuntimeArgumentHandle.cs
- XsdBuildProvider.cs
- DataGridRow.cs
- GPRECTF.cs
- WebPartRestoreVerb.cs
- CodeGenerator.cs
- EncodingNLS.cs
- StrongBox.cs
- LocalizableAttribute.cs
- XPathPatternParser.cs
- IsolatedStorageFile.cs
- FrameSecurityDescriptor.cs
- Persist.cs
- Paragraph.cs
- XmlReflectionImporter.cs
- PowerStatus.cs
- EntitySetBaseCollection.cs
- Viewport3DVisual.cs
- ResponseStream.cs
- XmlSerializer.cs
- DesignerGenericWebPart.cs
- DataGridPageChangedEventArgs.cs
- precedingquery.cs
- WarningException.cs
- DocumentScope.cs
- TypeConverter.cs
- InstanceKeyCompleteException.cs
- BaseConfigurationRecord.cs
- SingleConverter.cs
- ContextBase.cs
- AutomationPattern.cs
- Expander.cs
- _CacheStreams.cs
- ButtonRenderer.cs
- KeyTime.cs
- UrlAuthFailureHandler.cs
- XPathParser.cs
- DataGridViewIntLinkedList.cs
- SafeFileMappingHandle.cs
- CommonXSendMessage.cs
- KeyProperty.cs
- MetadataArtifactLoaderFile.cs
- Camera.cs
- SimpleType.cs
- PermissionListSet.cs
- DataGridViewSelectedCellCollection.cs
- NameValueFileSectionHandler.cs
- SamlAuthorityBinding.cs
- GridViewUpdateEventArgs.cs
- ToolStripRendererSwitcher.cs
- TransactionOptions.cs
- FtpRequestCacheValidator.cs
- Timer.cs
- PersonalizationProviderCollection.cs
- DependencyPropertyChangedEventArgs.cs
- Pair.cs
- ToolStripItemDesigner.cs
- AttachedAnnotation.cs
- PickBranch.cs
- AmbientProperties.cs
- TextAnchor.cs
- DataBinding.cs
- MenuTracker.cs
- HttpListenerRequest.cs
- ExecutionEngineException.cs
- ChannelTerminatedException.cs
- CommandLibraryHelper.cs
- AppDomainProtocolHandler.cs
- AdapterUtil.cs
- GeneralTransform2DTo3DTo2D.cs
- SchemaObjectWriter.cs
- HtmlTextArea.cs
- ColorKeyFrameCollection.cs
- SqlRemoveConstantOrderBy.cs
- XmlSchemaAppInfo.cs
- AtlasWeb.Designer.cs
- FileDialogPermission.cs
- BinaryKeyIdentifierClause.cs
- XslCompiledTransform.cs
- ClientRuntimeConfig.cs
- GeometryGroup.cs
- GeneralTransform3DTo2D.cs
- NamedElement.cs
- XmlValidatingReader.cs
- TemplatePropertyEntry.cs
- WriteTimeStream.cs
- Gdiplus.cs