Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / Paragraph.cs / 1 / Paragraph.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Paragraph element. // // History: // 05/05/2003 : [....] - created. // 10/28/2004 : [....] - ContentElements refactoring. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter, DesignerSerializationVisibility using System.Windows.Markup; // ContentProperty using MS.Internal; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Paragraph element /// [ContentProperty("Inlines")] public class Paragraph : Block { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Static ctor. Initializes property metadata. /// static Paragraph() { DefaultStyleKeyProperty.OverrideMetadata(typeof(Paragraph), new FrameworkPropertyMetadata(typeof(Paragraph))); } ////// Public constructor. /// public Paragraph() : base() { } ////// Paragraph constructor. /// public Paragraph(Inline inline) : base() { if (inline == null) { throw new ArgumentNullException("inline"); } this.Inlines.Add(inline); } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Inline items contained in this Paragraph. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public InlineCollection Inlines { get { return new InlineCollection(this, /*isOwnerParent*/true); } } ////// DependencyProperty for public static readonly DependencyProperty TextDecorationsProperty = Inline.TextDecorationsProperty.AddOwner( typeof(Paragraph), new FrameworkPropertyMetadata( new FreezableDefaultValueFactory(TextDecorationCollection.Empty), FrameworkPropertyMetadataOptions.AffectsRender )); ///property. /// /// The TextDecorations property specifies decorations that are added to the text of an element. /// public TextDecorationCollection TextDecorations { get { return (TextDecorationCollection) GetValue(TextDecorationsProperty); } set { SetValue(TextDecorationsProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextIndentProperty = DependencyProperty.Register( "TextIndent", typeof(double), typeof(Paragraph), new FrameworkPropertyMetadata( 0.0, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(IsValidTextIndent)); ///property. /// /// The TextIndent property specifies the indentation of the first line of a paragraph. /// [TypeConverter(typeof(LengthConverter))] public double TextIndent { get { return (double)GetValue(TextIndentProperty); } set { SetValue(TextIndentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty MinOrphanLinesProperty = DependencyProperty.Register( "MinOrphanLines", typeof(int), typeof(Paragraph), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidMinOrphanLines)); ///property. /// /// The MinOrphanLines is the minimum number of lines that /// can be left behind when a paragraph is broken on a page break /// or column break. /// public int MinOrphanLines { get { return (int)GetValue(MinOrphanLinesProperty); } set { SetValue(MinOrphanLinesProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty MinWidowLinesProperty = DependencyProperty.Register( "MinWidowLines", typeof(int), typeof(Paragraph), new FrameworkPropertyMetadata( 0, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidMinWidowLines)); ///property. /// /// The MinWidowLines is the minimum number of lines after a break /// to be put on the next page or column. /// public int MinWidowLines { get { return (int)GetValue(MinWidowLinesProperty); } set { SetValue(MinWidowLinesProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty KeepWithNextProperty = DependencyProperty.Register( "KeepWithNext", typeof(bool), typeof(Paragraph), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// The KeepWithNext property indicates that this paragraph should be kept with /// the next paragraph in the track. (This also implies that the paragraph itself /// will not be broken.) /// public bool KeepWithNext { get { return (bool)GetValue(KeepWithNextProperty); } set { SetValue(KeepWithNextProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty KeepTogetherProperty = DependencyProperty.Register( "KeepTogether", typeof(bool), typeof(Paragraph), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ///property. /// /// The KeepTogether property indicates that all the text in the paragraph /// should be kept together. /// public bool KeepTogether { get { return (bool)GetValue(KeepTogetherProperty); } set { SetValue(KeepTogetherProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- #region Internal Methods internal void GetDefaultMarginValue(ref Thickness margin) { double lineHeight = this.LineHeight; if (IsLineHeightAuto(lineHeight)) { lineHeight = this.FontFamily.LineSpacing * this.FontSize; } margin = new Thickness(0, lineHeight, 0, lineHeight); } internal static bool IsMarginAuto(Thickness margin) { return (Double.IsNaN(margin.Left) && Double.IsNaN(margin.Right) && Double.IsNaN(margin.Top) && Double.IsNaN(margin.Bottom)); } internal static bool IsLineHeightAuto(double lineHeight) { return (Double.IsNaN(lineHeight)); } // Returns true if there is no text/embedded element content in passed paragraph, // it may have zero or more inline formatting tags with no text. internal static bool HasNoTextContent(Paragraph paragraph) { ITextPointer navigator = paragraph.ContentStart.CreatePointer(); ITextPointer end = paragraph.ContentEnd; while (navigator.CompareTo(end) < 0) { TextPointerContext symbolType = navigator.GetPointerContext(LogicalDirection.Forward); if (symbolType == TextPointerContext.Text || symbolType == TextPointerContext.EmbeddedElement || typeof(LineBreak).IsAssignableFrom(navigator.ParentType) || typeof(AnchoredBlock).IsAssignableFrom(navigator.ParentType)) { return false; } navigator.MoveToNextContextPosition(LogicalDirection.Forward); } return true; } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeInlines(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidMinOrphanLines(object o) { int value = (int)o; const int maxLines = PTS.Restrictions.tscLineInParaRestriction; return (value >= 0 && value <= maxLines); } private static bool IsValidMinWidowLines(object o) { int value = (int)o; const int maxLines = PTS.Restrictions.tscLineInParaRestriction; return (value >= 0 && value <= maxLines); } private static bool IsValidTextIndent(object o) { double indent = (double)o; double maxIndent = Math.Min(1000000, PTS.MaxPageSize); double minIndent = -maxIndent; if (Double.IsNaN(indent)) { return false; } if (indent < minIndent || indent > maxIndent) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
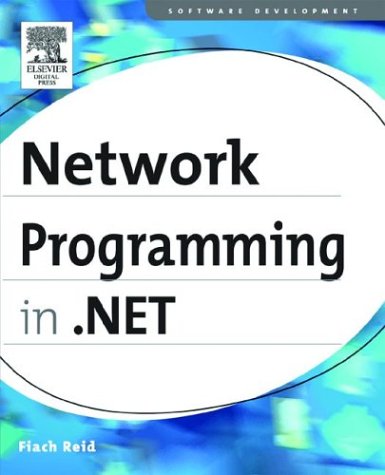
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyElement.cs
- Literal.cs
- SmiEventSink_DeferedProcessing.cs
- StorageEndPropertyMapping.cs
- PenThreadPool.cs
- TimelineCollection.cs
- MetadataResolver.cs
- UpdateCommand.cs
- Geometry.cs
- XmlSchemaObjectTable.cs
- SpecialFolderEnumConverter.cs
- DtdParser.cs
- Int32Storage.cs
- PatternMatcher.cs
- PointConverter.cs
- BmpBitmapDecoder.cs
- CodeSnippetStatement.cs
- WizardStepBase.cs
- DataGridViewComboBoxColumn.cs
- EFAssociationProvider.cs
- QuerySetOp.cs
- lengthconverter.cs
- ApplicationDirectory.cs
- BitmapSizeOptions.cs
- xmlfixedPageInfo.cs
- DataGridViewRowPostPaintEventArgs.cs
- HeaderLabel.cs
- JavaScriptObjectDeserializer.cs
- SerTrace.cs
- WindowsSysHeader.cs
- PackageRelationship.cs
- HttpListenerRequest.cs
- _NegotiateClient.cs
- BaseDataList.cs
- CompressedStack.cs
- DataBinding.cs
- UnsafeNativeMethods.cs
- BitmapSource.cs
- XPathNodeIterator.cs
- DataGridViewRowCollection.cs
- MembershipPasswordException.cs
- SqlCacheDependencyDatabase.cs
- EncoderNLS.cs
- BrushValueSerializer.cs
- ViewStateModeByIdAttribute.cs
- TransportSecurityHelpers.cs
- AspNetSynchronizationContext.cs
- Inflater.cs
- QueryRewriter.cs
- AuthenticationService.cs
- WMIGenerator.cs
- VisualCollection.cs
- ActivationArguments.cs
- XmlSchemaDocumentation.cs
- AuthenticationService.cs
- IPipelineRuntime.cs
- JoinCqlBlock.cs
- MenuStrip.cs
- StoreUtilities.cs
- TabletDeviceInfo.cs
- PropertyPathWorker.cs
- MethodExpr.cs
- StdValidatorsAndConverters.cs
- LicenseProviderAttribute.cs
- ParsedAttributeCollection.cs
- TranslateTransform3D.cs
- cryptoapiTransform.cs
- connectionpool.cs
- Vector3DIndependentAnimationStorage.cs
- DashStyle.cs
- RSAOAEPKeyExchangeFormatter.cs
- _FtpDataStream.cs
- NotImplementedException.cs
- UpdateCommand.cs
- WebPartConnectVerb.cs
- XamlToRtfWriter.cs
- ImageMap.cs
- WCFBuildProvider.cs
- ImpersonateTokenRef.cs
- AutomationPeer.cs
- HttpResponseHeader.cs
- StylusButtonCollection.cs
- AddressingVersion.cs
- lengthconverter.cs
- HttpCookieCollection.cs
- ObservableCollectionDefaultValueFactory.cs
- QuaternionAnimation.cs
- XPathChildIterator.cs
- PlainXmlSerializer.cs
- DetailsViewAutoFormat.cs
- InteropExecutor.cs
- HttpApplicationFactory.cs
- Triplet.cs
- NumberFormatter.cs
- ClientScriptManager.cs
- ExcCanonicalXml.cs
- TextMessageEncodingBindingElement.cs
- RegisteredArrayDeclaration.cs
- MessagePartDescription.cs
- __Error.cs