Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / HttpCookieCollection.cs / 1305376 / HttpCookieCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Collection of Http cookies for request and response intrinsics * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Web.Util; ////// public sealed class HttpCookieCollection : NameObjectCollectionBase { // Response object to notify about changes in collection private HttpResponse _response; // cached All[] arrays private HttpCookie[] _all; private String[] _allKeys; private bool _changed; internal HttpCookieCollection(HttpResponse response, bool readOnly) : base(StringComparer.OrdinalIgnoreCase) { _response = response; IsReadOnly = readOnly; } ////// Provides a type-safe /// way to manipulate HTTP cookies. /// ////// public HttpCookieCollection(): base(StringComparer.OrdinalIgnoreCase) { } internal bool Changed { get { return _changed; } set { _changed = value; } } internal void AddCookie(HttpCookie cookie, bool append) { _all = null; _allKeys = null; if (append) { // mark cookie as new cookie.Added = true; BaseAdd(cookie.Name, cookie); } else { if (BaseGet(cookie.Name) != null) { // mark the cookie as changed because we are overriding the existing one cookie.Changed = true; } BaseSet(cookie.Name, cookie); } } internal void RemoveCookie(String name) { _all = null; _allKeys = null; BaseRemove(name); _changed = true; } internal void Reset() { _all = null; _allKeys = null; BaseClear(); _changed = true; } // // Public APIs to add / remove // ////// Initializes a new instance of the HttpCookieCollection /// class. /// ////// public void Add(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, true); if (_response != null) _response.OnCookieAdd(cookie); } ////// Adds a cookie to the collection. /// ////// public void CopyTo(Array dest, int index) { if (_all == null) { int n = Count; _all = new HttpCookie[n]; for (int i = 0; i < n; i++) _all[i] = Get(i); } _all.CopyTo(dest, index); } ///[To be supplied.] ////// public void Set(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, false); if (_response != null) _response.OnCookieCollectionChange(); } ///Updates the value of a cookie. ////// public void Remove(String name) { if (_response != null) _response.BeforeCookieCollectionChange(); RemoveCookie(name); if (_response != null) _response.OnCookieCollectionChange(); } ////// Removes a cookie from the collection. /// ////// public void Clear() { Reset(); } // // Access by name // ////// Clears all cookies from the collection. /// ////// public HttpCookie Get(String name) { HttpCookie cookie = (HttpCookie)BaseGet(name); if (cookie == null && _response != null) { // response cookies are created on demand cookie = new HttpCookie(name); AddCookie(cookie, true); _response.OnCookieAdd(cookie); } return cookie; } ///Returns an ///item from the collection. /// public HttpCookie this[String name] { get { return Get(name);} } // // Indexed access // ///Indexed value that enables access to a cookie in the collection. ////// public HttpCookie Get(int index) { return(HttpCookie)BaseGet(index); } ////// Returns an ////// item from the collection. /// /// public String GetKey(int index) { return BaseGetKey(index); } ////// Returns key name from collection. /// ////// public HttpCookie this[int index] { get { return Get(index);} } // // Access to keys and values as arrays // /* * All keys */ ////// Default property. /// Indexed property that enables access to a cookie in the collection. /// ////// public String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns /// an array of all cookie keys in the cookie collection. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Collection of Http cookies for request and response intrinsics * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Web.Util; ////// public sealed class HttpCookieCollection : NameObjectCollectionBase { // Response object to notify about changes in collection private HttpResponse _response; // cached All[] arrays private HttpCookie[] _all; private String[] _allKeys; private bool _changed; internal HttpCookieCollection(HttpResponse response, bool readOnly) : base(StringComparer.OrdinalIgnoreCase) { _response = response; IsReadOnly = readOnly; } ////// Provides a type-safe /// way to manipulate HTTP cookies. /// ////// public HttpCookieCollection(): base(StringComparer.OrdinalIgnoreCase) { } internal bool Changed { get { return _changed; } set { _changed = value; } } internal void AddCookie(HttpCookie cookie, bool append) { _all = null; _allKeys = null; if (append) { // mark cookie as new cookie.Added = true; BaseAdd(cookie.Name, cookie); } else { if (BaseGet(cookie.Name) != null) { // mark the cookie as changed because we are overriding the existing one cookie.Changed = true; } BaseSet(cookie.Name, cookie); } } internal void RemoveCookie(String name) { _all = null; _allKeys = null; BaseRemove(name); _changed = true; } internal void Reset() { _all = null; _allKeys = null; BaseClear(); _changed = true; } // // Public APIs to add / remove // ////// Initializes a new instance of the HttpCookieCollection /// class. /// ////// public void Add(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, true); if (_response != null) _response.OnCookieAdd(cookie); } ////// Adds a cookie to the collection. /// ////// public void CopyTo(Array dest, int index) { if (_all == null) { int n = Count; _all = new HttpCookie[n]; for (int i = 0; i < n; i++) _all[i] = Get(i); } _all.CopyTo(dest, index); } ///[To be supplied.] ////// public void Set(HttpCookie cookie) { if (_response != null) _response.BeforeCookieCollectionChange(); AddCookie(cookie, false); if (_response != null) _response.OnCookieCollectionChange(); } ///Updates the value of a cookie. ////// public void Remove(String name) { if (_response != null) _response.BeforeCookieCollectionChange(); RemoveCookie(name); if (_response != null) _response.OnCookieCollectionChange(); } ////// Removes a cookie from the collection. /// ////// public void Clear() { Reset(); } // // Access by name // ////// Clears all cookies from the collection. /// ////// public HttpCookie Get(String name) { HttpCookie cookie = (HttpCookie)BaseGet(name); if (cookie == null && _response != null) { // response cookies are created on demand cookie = new HttpCookie(name); AddCookie(cookie, true); _response.OnCookieAdd(cookie); } return cookie; } ///Returns an ///item from the collection. /// public HttpCookie this[String name] { get { return Get(name);} } // // Indexed access // ///Indexed value that enables access to a cookie in the collection. ////// public HttpCookie Get(int index) { return(HttpCookie)BaseGet(index); } ////// Returns an ////// item from the collection. /// /// public String GetKey(int index) { return BaseGetKey(index); } ////// Returns key name from collection. /// ////// public HttpCookie this[int index] { get { return Get(index);} } // // Access to keys and values as arrays // /* * All keys */ ////// Default property. /// Indexed property that enables access to a cookie in the collection. /// ////// public String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns /// an array of all cookie keys in the cookie collection. /// ///
Link Menu
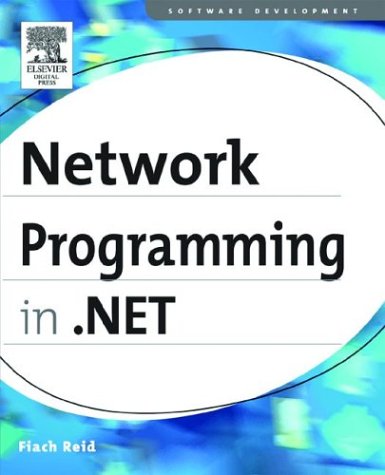
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- listitem.cs
- MetadataReference.cs
- SrgsSemanticInterpretationTag.cs
- QuaternionIndependentAnimationStorage.cs
- ScrollViewer.cs
- XmlElementAttribute.cs
- AttributeCollection.cs
- StringHandle.cs
- HtmlElement.cs
- SqlNode.cs
- QueryStringParameter.cs
- RequestContext.cs
- SerializationObjectManager.cs
- XamlTypeWithExplicitNamespace.cs
- FrameworkContentElement.cs
- FixedDocumentSequencePaginator.cs
- XmlSchemaAny.cs
- RtfControls.cs
- Gdiplus.cs
- RC2.cs
- SelectedGridItemChangedEvent.cs
- VerticalConnector.xaml.cs
- __Filters.cs
- TextClipboardData.cs
- Symbol.cs
- UriWriter.cs
- PhonemeEventArgs.cs
- TimeStampChecker.cs
- TimeSpanValidator.cs
- DrawingAttributes.cs
- XmlTextEncoder.cs
- ToolStripDesigner.cs
- XmlIgnoreAttribute.cs
- MeasureItemEvent.cs
- SizeF.cs
- CodeGeneratorOptions.cs
- StandardCommandToolStripMenuItem.cs
- PeerName.cs
- BrushValueSerializer.cs
- Point3DCollection.cs
- PageContent.cs
- CompressionTransform.cs
- CollectionConverter.cs
- ResourceContainer.cs
- SemaphoreSecurity.cs
- FacetValues.cs
- PageParser.cs
- StylusPointDescription.cs
- ScaleTransform.cs
- SessionState.cs
- _TLSstream.cs
- ResourceBinder.cs
- ControlUtil.cs
- UserUseLicenseDictionaryLoader.cs
- SessionStateUtil.cs
- TimelineClockCollection.cs
- GeneralTransformGroup.cs
- ScanQueryOperator.cs
- XmlSchemaValidationException.cs
- HebrewCalendar.cs
- XmlReaderDelegator.cs
- InheritanceContextChangedEventManager.cs
- ServiceReference.cs
- OdbcConnectionStringbuilder.cs
- QuaternionConverter.cs
- NullableFloatSumAggregationOperator.cs
- StylusPointProperty.cs
- XmlSerializationGeneratedCode.cs
- Utils.cs
- DataGridViewCellFormattingEventArgs.cs
- ArraySubsetEnumerator.cs
- TypeUsageBuilder.cs
- HtmlForm.cs
- HttpWebResponse.cs
- RegionInfo.cs
- ParentUndoUnit.cs
- Serializer.cs
- EditBehavior.cs
- CodeGroup.cs
- LicenseException.cs
- BitmapEffectGroup.cs
- DataReaderContainer.cs
- MaskedTextBox.cs
- securitycriticaldataClass.cs
- Comparer.cs
- ValidatedControlConverter.cs
- CapabilitiesState.cs
- StructureChangedEventArgs.cs
- DataGridViewCell.cs
- ObjectPersistData.cs
- GridProviderWrapper.cs
- OpenTypeLayoutCache.cs
- UrlMappingsSection.cs
- DataRelationCollection.cs
- InheritanceAttribute.cs
- RelationalExpressions.cs
- FixedTextView.cs
- DrawListViewColumnHeaderEventArgs.cs
- DataSourceHelper.cs
- SmtpReplyReaderFactory.cs