Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Collections / Generic / Comparer.cs / 1305376 / Comparer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics.Contracts; //using System.Globalization; using System.Runtime.CompilerServices; namespace System.Collections.Generic { [Serializable] [TypeDependencyAttribute("System.Collections.Generic.GenericComparer`1")] public abstract class Comparer: IComparer, IComparer { static Comparer defaultComparer; public static Comparer Default { [System.Security.SecuritySafeCritical] // auto-generated get { Contract.Ensures(Contract.Result >() != null); Comparer comparer = defaultComparer; if (comparer == null) { comparer = CreateComparer(); defaultComparer = comparer; } return comparer; } } [System.Security.SecuritySafeCritical] // auto-generated private static Comparer CreateComparer() { RuntimeType t = (RuntimeType)typeof(T); // If T implements IComparable return a GenericComparer if (typeof(IComparable ).IsAssignableFrom(t)) { //return (Comparer )Activator.CreateInstance(typeof(GenericComparer<>).MakeGenericType(t)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(GenericComparer ), t); } // If T is a Nullable where U implements IComparable return a NullableComparer if (t.IsGenericType && t.GetGenericTypeDefinition() == typeof(Nullable<>)) { RuntimeType u = (RuntimeType)t.GetGenericArguments()[0]; if (typeof(IComparable<>).MakeGenericType(u).IsAssignableFrom(u)) { //return (Comparer )Activator.CreateInstance(typeof(NullableComparer<>).MakeGenericType(u)); return (Comparer )RuntimeTypeHandle.CreateInstanceForAnotherGenericParameter((RuntimeType)typeof(NullableComparer ), u); } } // Otherwise return an ObjectComparer return new ObjectComparer (); } public abstract int Compare(T x, T y); int IComparer.Compare(object x, object y) { if (x == null) return y == null ? 0 : -1; if (y == null) return 1; if (x is T && y is T) return Compare((T)x, (T)y); ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_InvalidArgumentForComparison); return 0; } } [Serializable] internal class GenericComparer : Comparer where T: IComparable { public override int Compare(T x, T y) { if (x != null) { if (y != null) return x.CompareTo(y); return 1; } if (y != null) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ GenericComparer comparer = obj as GenericComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class NullableComparer : Comparer > where T : struct, IComparable { public override int Compare(Nullable x, Nullable y) { if (x.HasValue) { if (y.HasValue) return x.value.CompareTo(y.value); return 1; } if (y.HasValue) return -1; return 0; } // Equals method for the comparer itself. public override bool Equals(Object obj){ NullableComparer comparer = obj as NullableComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } [Serializable] internal class ObjectComparer : Comparer { public override int Compare(T x, T y) { return System.Collections.Comparer.Default.Compare(x, y); } // Equals method for the comparer itself. public override bool Equals(Object obj){ ObjectComparer comparer = obj as ObjectComparer ; return comparer != null; } public override int GetHashCode() { return this.GetType().Name.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
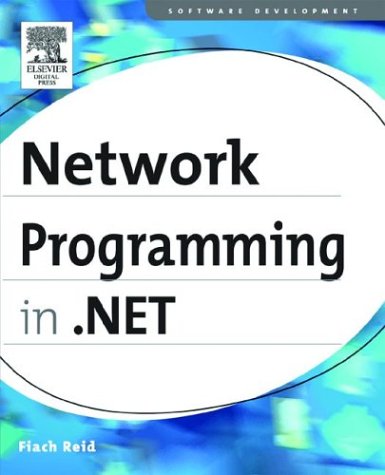
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityValidator.cs
- ControlLocalizer.cs
- MethodBuilder.cs
- InternalCache.cs
- ExpressionEditorAttribute.cs
- EventSetter.cs
- _NestedSingleAsyncResult.cs
- XPathParser.cs
- altserialization.cs
- WebPartEventArgs.cs
- ThrowOnMultipleAssignment.cs
- Wow64ConfigurationLoader.cs
- NativeBuffer.cs
- WeakEventManager.cs
- PasswordPropertyTextAttribute.cs
- ValueType.cs
- GlyphShapingProperties.cs
- Journal.cs
- ReadWriteObjectLock.cs
- ConfigurationSettings.cs
- Rect.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- CompilerState.cs
- UpdateTranslator.cs
- DetailsViewRowCollection.cs
- ReadOnlyDictionary.cs
- WorkflowControlEndpoint.cs
- PageContentAsyncResult.cs
- Geometry3D.cs
- StrokeFIndices.cs
- ScriptHandlerFactory.cs
- TextTreeUndo.cs
- DelegatingTypeDescriptionProvider.cs
- ObjectAssociationEndMapping.cs
- PointCollection.cs
- SecurityPolicySection.cs
- safex509handles.cs
- MiniAssembly.cs
- RegistrySecurity.cs
- CompiledELinqQueryState.cs
- BooleanExpr.cs
- UserNamePasswordClientCredential.cs
- PartialCachingControl.cs
- InvariantComparer.cs
- DbDataSourceEnumerator.cs
- TextTreeText.cs
- HeaderUtility.cs
- DeadCharTextComposition.cs
- RuntimeConfig.cs
- MonitorWrapper.cs
- XmlAutoDetectWriter.cs
- TextRangeProviderWrapper.cs
- ProjectionPlan.cs
- hresults.cs
- GeometryModel3D.cs
- HtmlValidatorAdapter.cs
- Pair.cs
- EdmTypeAttribute.cs
- GenericIdentity.cs
- SqlDataSource.cs
- WebPartAddingEventArgs.cs
- SHA256.cs
- ColorAnimationUsingKeyFrames.cs
- EntitySetDataBindingList.cs
- AbsoluteQuery.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- WebPartConnectionCollection.cs
- VerificationAttribute.cs
- ScrollItemPattern.cs
- VariableQuery.cs
- Debug.cs
- PropertyTabAttribute.cs
- ProfilePropertySettingsCollection.cs
- DataGridViewRow.cs
- TreeNodeStyle.cs
- CheckBoxList.cs
- EmptyImpersonationContext.cs
- RenderOptions.cs
- X509ChainPolicy.cs
- FileDialogCustomPlacesCollection.cs
- PeerResolverElement.cs
- JsonGlobals.cs
- SamlAuthorizationDecisionStatement.cs
- ProfileParameter.cs
- WSSecureConversationDec2005.cs
- X509Extension.cs
- ViewKeyConstraint.cs
- WebBrowsableAttribute.cs
- ContentHostHelper.cs
- FrugalMap.cs
- TabControlCancelEvent.cs
- HttpCapabilitiesSectionHandler.cs
- UIElementParaClient.cs
- MultiView.cs
- SpecialNameAttribute.cs
- RealProxy.cs
- ListControl.cs
- dbdatarecord.cs
- Pkcs9Attribute.cs
- TemplateBamlRecordReader.cs