Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ImageListImageEditor.cs / 1 / ImageListImageEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.IO; using System.Collections; using System.ComponentModel; using System.Windows.Forms; using System.Drawing; using System.Design; using System.Reflection; using System.Drawing.Design; using System.Windows.Forms.ComponentModel; ////// /// public class ImageListImageEditor : ImageEditor { // VSWhidbey 95227: Metafile types are not supported in the ImageListImageEditor and should // not be displayed as an option. internal static Type[] imageExtenders = new Type[] { typeof(BitmapEditor)/*, gpr typeof(Icon), typeof(MetafileEditor)*/}; private OpenFileDialog fileDialog = null; // VSWhidbey 95227: accessor needed into the static field so that derived classes // can implement a different list of supported image types. [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. protected override Type[] GetImageExtenders() { return imageExtenders; } ///Provides an editor that can perform default file searching for bitmap (.bmp) /// files. ////// /// Edits the given object value using the editor style provided by /// GetEditorStyle. A service provider is provided so that any /// required editing services can be obtained. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { ArrayList images = new ArrayList(); if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { if (fileDialog == null) { fileDialog = new OpenFileDialog(); fileDialog.Multiselect = true; string filter = CreateFilterEntry(this); for (int i = 0; i < GetImageExtenders().Length; i++) { ImageEditor e = (ImageEditor) Activator.CreateInstance(GetImageExtenders()[i], BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.CreateInstance, null, null, null); //.CreateInstance(); Type myClass = this.GetType(); Type editorClass = e.GetType(); if (!myClass.Equals(editorClass) && e != null && myClass.IsInstanceOfType(e)) filter += "|" + CreateFilterEntry(e); } fileDialog.Filter = filter; } IntPtr hwndFocus = UnsafeNativeMethods.GetFocus(); try { if (fileDialog.ShowDialog() == DialogResult.OK) { foreach (string name in fileDialog.FileNames) { ImageListImage image; FileStream file = new FileStream(name, FileMode.Open, FileAccess.Read, FileShare.Read); image = LoadImageFromStream(file, name.EndsWith(".ico")); image.Name = System.IO.Path.GetFileName(name); images.Add(image); } } } finally { if (hwndFocus != IntPtr.Zero) { UnsafeNativeMethods.SetFocus(new HandleRef(null, hwndFocus)); } } } return images; } return value; } ////// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. protected override string GetFileDialogDescription() { return SR.GetString(SR.imageFileDescription); } ///[To be supplied.] ////// /// Determines if this editor supports the painting of a representation /// of an object's value. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override bool GetPaintValueSupported(ITypeDescriptorContext context) { return true; } ////// /// private ImageListImage LoadImageFromStream(Stream stream, bool imageIsIcon) { //Copy the original stream to a buffer, then wrap a //memory stream around it. This way we can avoid //locking the file byte[] buffer = new byte[stream.Length]; stream.Read(buffer, 0, (int)stream.Length); MemoryStream ms = new MemoryStream(buffer); return (ImageListImage)ImageListImage.ImageListImageFromStream(ms, imageIsIcon); } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override void PaintValue(PaintValueEventArgs e) { if (e.Value is ImageListImage) { e= new PaintValueEventArgs(e.Context, ((ImageListImage)e.Value).Image, e.Graphics, e.Bounds); } base.PaintValue(e); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Paints a representative value of the given object to the provided /// canvas. Painting should be done within the boundaries of the /// provided rectangle. /// ///
Link Menu
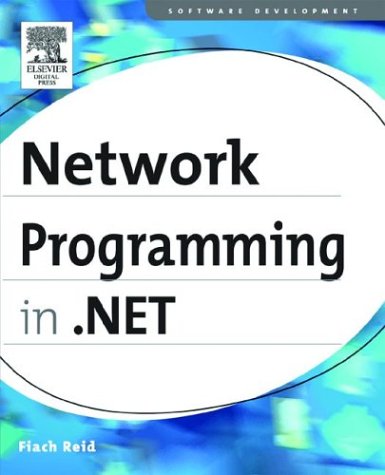
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlIlTypeHelper.cs
- MeasureItemEvent.cs
- OletxResourceManager.cs
- DataControlReference.cs
- Trace.cs
- ChtmlCalendarAdapter.cs
- Label.cs
- TransactionContextManager.cs
- DataGridViewCellStyleChangedEventArgs.cs
- EncodingStreamWrapper.cs
- SettingsPropertyValue.cs
- GuidConverter.cs
- HtmlShim.cs
- JavaScriptSerializer.cs
- UnsettableComboBox.cs
- WinCategoryAttribute.cs
- DesignTimeTemplateParser.cs
- ConnectionStringsExpressionBuilder.cs
- Tuple.cs
- DateTimePicker.cs
- CompilerInfo.cs
- AncillaryOps.cs
- ViewBase.cs
- PrintingPermissionAttribute.cs
- TextStore.cs
- DurationConverter.cs
- Polygon.cs
- BoundColumn.cs
- FileDialog.cs
- DataGridItemEventArgs.cs
- MissingSatelliteAssemblyException.cs
- PaintEvent.cs
- Rights.cs
- DesignRelationCollection.cs
- TraceUtils.cs
- EnvelopedSignatureTransform.cs
- SoapRpcServiceAttribute.cs
- ButtonField.cs
- CheckedListBox.cs
- CapabilitiesState.cs
- EpmSourceTree.cs
- WebBrowser.cs
- FigureHelper.cs
- XmlObjectSerializerWriteContext.cs
- Helpers.cs
- AdPostCacheSubstitution.cs
- KeyValueSerializer.cs
- ImmutablePropertyDescriptorGridEntry.cs
- ConfigurationStrings.cs
- BrushMappingModeValidation.cs
- LiteralControl.cs
- DynamicResourceExtensionConverter.cs
- NameValuePermission.cs
- ReferentialConstraint.cs
- SafeReadContext.cs
- DataServiceExpressionVisitor.cs
- BaseAsyncResult.cs
- DoubleAnimationUsingPath.cs
- ManifestBasedResourceGroveler.cs
- ObjectSecurity.cs
- ToolStripItem.cs
- LinkArea.cs
- TransactionManager.cs
- ProxySimple.cs
- TrustSection.cs
- FontInfo.cs
- VisualTarget.cs
- Attributes.cs
- AssemblyAttributes.cs
- HtmlMeta.cs
- AppDomainInfo.cs
- CroppedBitmap.cs
- ProtocolsConfigurationHandler.cs
- GenericAuthenticationEventArgs.cs
- SoapSchemaImporter.cs
- _LocalDataStoreMgr.cs
- ColorMap.cs
- UTF8Encoding.cs
- TextServicesCompartmentContext.cs
- SessionStateModule.cs
- DocComment.cs
- DataRecordInternal.cs
- OracleConnectionString.cs
- Ipv6Element.cs
- RenderingBiasValidation.cs
- AtomServiceDocumentSerializer.cs
- Win32Exception.cs
- CodeRegionDirective.cs
- DefaultTraceListener.cs
- DataGridViewRow.cs
- ThousandthOfEmRealPoints.cs
- Mutex.cs
- DataGridRelationshipRow.cs
- FixedStringLookup.cs
- DataTable.cs
- InnerItemCollectionView.cs
- EventLogTraceListener.cs
- ObjectToken.cs
- DesignerActionMethodItem.cs
- SafeViewOfFileHandle.cs