Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / HitTestDrawingContextWalker.cs / 1 / HitTestDrawingContextWalker.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: HitTestDrawingContextWalker.cs // // Description: This file contains the implementation of HitTestDrawingContextWalker. // This DrawingContextWalker is used to perform hit tests on renderdata. // // History: // 04/10/2004 : adsmith - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; namespace System.Windows.Media { ////// HitTestDrawingContextWalker - a DrawingContextWalker which will perform a point or /// geometry based hit test on the contents of a render data. /// internal abstract class HitTestDrawingContextWalker: DrawingContextWalker { ////// Constructor /// internal HitTestDrawingContextWalker() { } ////// IsHit Property - Returns whether the point or geometry intersected the drawing instructions. /// internal abstract bool IsHit { get; } abstract internal IntersectionDetail IntersectionDetail { get; } #region Static Drawing Context Methods ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// The end Point for the line. public override void DrawLine( Pen pen, Point point0, Point point1) { // DrawGeometry(null /* brush */, pen, new LineGeometry(point0, point1)); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle) { // DrawGeometry(brush, pen, new RectangleGeometry(rectangle)); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, Double radiusX, Double radiusY) { // DrawGeometry(brush, pen, new RectangleGeometry(rectangle, radiusX, radiusY)); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// public override void DrawEllipse( Brush brush, Pen pen, Point center, Double radiusX, Double radiusY) { // DrawGeometry(brush, pen, new EllipseGeometry(center, radiusX, radiusY)); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// public override void DrawImage( ImageSource imageSource, Rect rectangle) { // ImageBrush imageBrush = new ImageBrush(); // The ImageSource provided will be shared between the original location and the new ImageBrush // we're creating - this will by default break property inheritance, dynamic resource references // and databinding. To prevent this, we mark the new ImageBrush.CanBeInheritanceContext == false. imageBrush.CanBeInheritanceContext = false; imageBrush.ImageSource = imageSource; DrawGeometry(imageBrush, null /* pen */, new RectangleGeometry(rectangle)); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with a VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// /// The Rect into which the MediaPlayer will be fit. /// public override void DrawVideo( MediaPlayer video, Rect rectangle) { // Hit test a rect with a VideoBrush once it exists. // DrawGeometry(new VideoBrush(video), null /* pen */, new RectangleGeometry(rectangle)); // DrawGeometry(Brushes.Black, null /* pen */, new RectangleGeometry(rectangle)); } #endregion Static Drawing Context Methods #region Protected Fields // Indicates if the Visual fully contains the hit-test point or geometry protected bool _contains; #endregion Protected Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: HitTestDrawingContextWalker.cs // // Description: This file contains the implementation of HitTestDrawingContextWalker. // This DrawingContextWalker is used to perform hit tests on renderdata. // // History: // 04/10/2004 : adsmith - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; namespace System.Windows.Media { ////// HitTestDrawingContextWalker - a DrawingContextWalker which will perform a point or /// geometry based hit test on the contents of a render data. /// internal abstract class HitTestDrawingContextWalker: DrawingContextWalker { ////// Constructor /// internal HitTestDrawingContextWalker() { } ////// IsHit Property - Returns whether the point or geometry intersected the drawing instructions. /// internal abstract bool IsHit { get; } abstract internal IntersectionDetail IntersectionDetail { get; } #region Static Drawing Context Methods ////// DrawLine - /// Draws a line with the specified pen. /// Note that this API does not accept a Brush, as there is no area to fill. /// /// The Pen with which to stroke the line. /// The start Point for the line. /// The end Point for the line. public override void DrawLine( Pen pen, Point point0, Point point1) { // DrawGeometry(null /* brush */, pen, new LineGeometry(point0, point1)); } ////// DrawRectangle - /// Draw a rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. public override void DrawRectangle( Brush brush, Pen pen, Rect rectangle) { // DrawGeometry(brush, pen, new RectangleGeometry(rectangle)); } ////// DrawRoundedRectangle - /// Draw a rounded rectangle with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the rectangle. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the rectangle. /// This is optional, and can be null, in which case no stroke is performed. /// /// The Rect to fill and/or stroke. /// /// The radius in the X dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Width/2] /// /// /// The radius in the Y dimension of the rounded corners of this /// rounded Rect. This value will be clamped to the range [0..rectangle.Height/2]. /// public override void DrawRoundedRectangle( Brush brush, Pen pen, Rect rectangle, Double radiusX, Double radiusY) { // DrawGeometry(brush, pen, new RectangleGeometry(rectangle, radiusX, radiusY)); } ////// DrawEllipse - /// Draw an ellipse with the provided Brush and/or Pen. /// If both the Brush and Pen are null this call is a no-op. /// /// /// The Brush with which to fill the ellipse. /// This is optional, and can be null, in which case no fill is performed. /// /// /// The Pen with which to stroke the ellipse. /// This is optional, and can be null, in which case no stroke is performed. /// /// /// The center of the ellipse to fill and/or stroke. /// /// /// The radius in the X dimension of the ellipse. /// The absolute value of the radius provided will be used. /// /// /// The radius in the Y dimension of the ellipse. /// The absolute value of the radius provided will be used. /// public override void DrawEllipse( Brush brush, Pen pen, Point center, Double radiusX, Double radiusY) { // DrawGeometry(brush, pen, new EllipseGeometry(center, radiusX, radiusY)); } ////// DrawImage - /// Draw an Image into the region specified by the Rect. /// The Image will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with an ImageBrush via /// DrawRectangle. /// /// The ImageSource to draw. /// /// The Rect into which the ImageSource will be fit. /// public override void DrawImage( ImageSource imageSource, Rect rectangle) { // ImageBrush imageBrush = new ImageBrush(); // The ImageSource provided will be shared between the original location and the new ImageBrush // we're creating - this will by default break property inheritance, dynamic resource references // and databinding. To prevent this, we mark the new ImageBrush.CanBeInheritanceContext == false. imageBrush.CanBeInheritanceContext = false; imageBrush.ImageSource = imageSource; DrawGeometry(imageBrush, null /* pen */, new RectangleGeometry(rectangle)); } ////// DrawVideo - /// Draw a Video into the region specified by the Rect. /// The Video will potentially be stretched and distorted to fit the Rect. /// For more fine grained control, consider filling a Rect with a VideoBrush via /// DrawRectangle. /// /// The MediaPlayer to draw. /// /// The Rect into which the MediaPlayer will be fit. /// public override void DrawVideo( MediaPlayer video, Rect rectangle) { // Hit test a rect with a VideoBrush once it exists. // DrawGeometry(new VideoBrush(video), null /* pen */, new RectangleGeometry(rectangle)); // DrawGeometry(Brushes.Black, null /* pen */, new RectangleGeometry(rectangle)); } #endregion Static Drawing Context Methods #region Protected Fields // Indicates if the Visual fully contains the hit-test point or geometry protected bool _contains; #endregion Protected Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
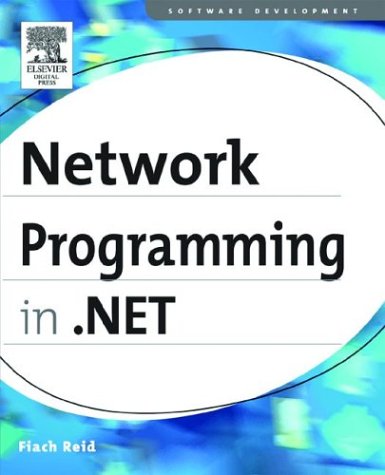
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlWrappingReader.cs
- CmsInterop.cs
- MetadataItem.cs
- TimeZone.cs
- HostedTransportConfigurationBase.cs
- DrawingBrush.cs
- Help.cs
- RuntimeConfigurationRecord.cs
- ErrorProvider.cs
- TempFiles.cs
- FixedSOMContainer.cs
- Item.cs
- X509CertificateRecipientServiceCredential.cs
- ResourceWriter.cs
- DefaultObjectMappingItemCollection.cs
- TrackBarRenderer.cs
- SafeRightsManagementPubHandle.cs
- PersonalizationStateInfo.cs
- UnlockInstanceCommand.cs
- PrtCap_Reader.cs
- ApplySecurityAndSendAsyncResult.cs
- ReadingWritingEntityEventArgs.cs
- ListViewUpdateEventArgs.cs
- TreeNodeMouseHoverEvent.cs
- PackWebRequestFactory.cs
- PropertyPathWorker.cs
- TextRangeEditLists.cs
- CompiledQuery.cs
- CommandHelper.cs
- UserMapPath.cs
- TextDecoration.cs
- TextRangeSerialization.cs
- SmiXetterAccessMap.cs
- SetterBaseCollection.cs
- FixedSchema.cs
- PermissionSetEnumerator.cs
- MultiDataTrigger.cs
- TextTreeUndo.cs
- Camera.cs
- Triplet.cs
- DrawingContextDrawingContextWalker.cs
- InvokeBinder.cs
- PartialCachingControl.cs
- MenuItem.cs
- MinMaxParagraphWidth.cs
- ImageListUtils.cs
- formatter.cs
- XpsSerializerFactory.cs
- CallbackException.cs
- NamespaceQuery.cs
- ExpressionPrefixAttribute.cs
- NumberSubstitution.cs
- AtlasWeb.Designer.cs
- QueuePathDialog.cs
- ExtensibleSyndicationObject.cs
- AnnotationComponentChooser.cs
- Vector3DCollectionConverter.cs
- OpCopier.cs
- ExtendedPropertyDescriptor.cs
- ZipIOExtraFieldElement.cs
- HtmlHistory.cs
- WebPartDisplayMode.cs
- Update.cs
- XmlNavigatorStack.cs
- DataTablePropertyDescriptor.cs
- ProtocolsConfiguration.cs
- DelayDesigner.cs
- DataServiceStreamProviderWrapper.cs
- ListViewItemEventArgs.cs
- VisualStyleInformation.cs
- WebMessageEncoderFactory.cs
- ObjectDataSourceMethodEditor.cs
- RotateTransform3D.cs
- RuntimeHelpers.cs
- ScriptBehaviorDescriptor.cs
- Point.cs
- TextRunCache.cs
- TrustLevelCollection.cs
- DecoderReplacementFallback.cs
- StructuralType.cs
- StringToken.cs
- URIFormatException.cs
- RegexCharClass.cs
- HostElement.cs
- BmpBitmapEncoder.cs
- FormsAuthentication.cs
- DiagnosticTraceSchemas.cs
- _SslSessionsCache.cs
- TreeBuilder.cs
- MaxSessionCountExceededException.cs
- ObjectKeyFrameCollection.cs
- UnsignedPublishLicense.cs
- RewritingSimplifier.cs
- SiteMapNodeCollection.cs
- AccessViolationException.cs
- thaishape.cs
- AssemblyFilter.cs
- X509Chain.cs
- EmissiveMaterial.cs
- Help.cs