Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / CodeDOM / CodeTypeDeclaration.cs / 1 / CodeTypeDeclaration.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using Microsoft.Win32; using System.Collections; using System.Reflection; using System.Runtime.Serialization; using System.Runtime.InteropServices; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeTypeDeclaration : CodeTypeMember { private TypeAttributes attributes = Reflection.TypeAttributes.Public | Reflection.TypeAttributes.Class; private CodeTypeReferenceCollection baseTypes = new CodeTypeReferenceCollection(); private CodeTypeMemberCollection members = new CodeTypeMemberCollection(); private bool isEnum; private bool isStruct; private int populated = 0x0; private const int BaseTypesCollection = 0x1; private const int MembersCollection = 0x2; // Need to be made optionally serializable [OptionalField] private CodeTypeParameterCollection typeParameters; [OptionalField] private bool isPartial = false; ////// Represents a /// class or nested class. /// ////// public event EventHandler PopulateBaseTypes; ////// An event that will be fired the first time the BaseTypes Collection is accessed. /// ////// public event EventHandler PopulateMembers; ////// An event that will be fired the first time the Members Collection is accessed. /// ////// public CodeTypeDeclaration() { } ////// Initializes a new instance of ///. /// /// public CodeTypeDeclaration(string name) { Name = name; } ////// Initializes a new instance of ///with the specified name. /// /// public TypeAttributes TypeAttributes { get { return attributes; } set { attributes = value; } } ////// Gets or sets the attributes of the class. /// ////// public CodeTypeReferenceCollection BaseTypes { get { if (0 == (populated & BaseTypesCollection)) { populated |= BaseTypesCollection; if (PopulateBaseTypes != null) PopulateBaseTypes(this, EventArgs.Empty); } return baseTypes; } } ////// Gets or sets /// the base types of the class. /// ////// public bool IsClass { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Class && !isEnum && !isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Class; isStruct = false; isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is a class. /// ////// public bool IsStruct { get { return isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = true; isEnum = false; } else { isStruct = false; } } } ////// Gets or sets a value /// indicating whether the class is a struct. /// ////// public bool IsEnum { get { return isEnum; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = false; isEnum = true; } else { isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is an enumeration. /// ////// public bool IsInterface { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Interface; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Interface; isStruct = false; isEnum = false; } else { attributes &= ~TypeAttributes.Interface; } } } public bool IsPartial { get { return isPartial; } set { isPartial = value; } } ////// Gets or sets a value /// indicating whether the class is an interface. /// ////// public CodeTypeMemberCollection Members { get { if (0 == (populated & MembersCollection)) { populated |= MembersCollection; if (PopulateMembers != null) PopulateMembers(this, EventArgs.Empty); } return members; } } [System.Runtime.InteropServices.ComVisible(false)] public CodeTypeParameterCollection TypeParameters { get { if( typeParameters == null) { typeParameters = new CodeTypeParameterCollection(); } return typeParameters; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets or sets the class member collection members. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using Microsoft.Win32; using System.Collections; using System.Reflection; using System.Runtime.Serialization; using System.Runtime.InteropServices; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeTypeDeclaration : CodeTypeMember { private TypeAttributes attributes = Reflection.TypeAttributes.Public | Reflection.TypeAttributes.Class; private CodeTypeReferenceCollection baseTypes = new CodeTypeReferenceCollection(); private CodeTypeMemberCollection members = new CodeTypeMemberCollection(); private bool isEnum; private bool isStruct; private int populated = 0x0; private const int BaseTypesCollection = 0x1; private const int MembersCollection = 0x2; // Need to be made optionally serializable [OptionalField] private CodeTypeParameterCollection typeParameters; [OptionalField] private bool isPartial = false; ////// Represents a /// class or nested class. /// ////// public event EventHandler PopulateBaseTypes; ////// An event that will be fired the first time the BaseTypes Collection is accessed. /// ////// public event EventHandler PopulateMembers; ////// An event that will be fired the first time the Members Collection is accessed. /// ////// public CodeTypeDeclaration() { } ////// Initializes a new instance of ///. /// /// public CodeTypeDeclaration(string name) { Name = name; } ////// Initializes a new instance of ///with the specified name. /// /// public TypeAttributes TypeAttributes { get { return attributes; } set { attributes = value; } } ////// Gets or sets the attributes of the class. /// ////// public CodeTypeReferenceCollection BaseTypes { get { if (0 == (populated & BaseTypesCollection)) { populated |= BaseTypesCollection; if (PopulateBaseTypes != null) PopulateBaseTypes(this, EventArgs.Empty); } return baseTypes; } } ////// Gets or sets /// the base types of the class. /// ////// public bool IsClass { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Class && !isEnum && !isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Class; isStruct = false; isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is a class. /// ////// public bool IsStruct { get { return isStruct; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = true; isEnum = false; } else { isStruct = false; } } } ////// Gets or sets a value /// indicating whether the class is a struct. /// ////// public bool IsEnum { get { return isEnum; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; isStruct = false; isEnum = true; } else { isEnum = false; } } } ////// Gets or sets a value /// indicating whether the class is an enumeration. /// ////// public bool IsInterface { get { return(attributes & TypeAttributes.ClassSemanticsMask) == TypeAttributes.Interface; } set { if (value) { attributes &= ~TypeAttributes.ClassSemanticsMask; attributes |= TypeAttributes.Interface; isStruct = false; isEnum = false; } else { attributes &= ~TypeAttributes.Interface; } } } public bool IsPartial { get { return isPartial; } set { isPartial = value; } } ////// Gets or sets a value /// indicating whether the class is an interface. /// ////// public CodeTypeMemberCollection Members { get { if (0 == (populated & MembersCollection)) { populated |= MembersCollection; if (PopulateMembers != null) PopulateMembers(this, EventArgs.Empty); } return members; } } [System.Runtime.InteropServices.ComVisible(false)] public CodeTypeParameterCollection TypeParameters { get { if( typeParameters == null) { typeParameters = new CodeTypeParameterCollection(); } return typeParameters; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets or sets the class member collection members. /// ///
Link Menu
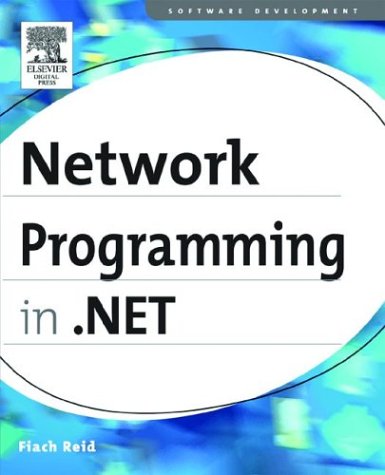
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChangesetResponse.cs
- SoapTypeAttribute.cs
- WindowsFont.cs
- EventLogTraceListener.cs
- SqlNamer.cs
- TagMapInfo.cs
- ColumnResizeAdorner.cs
- ResolveMatchesCD1.cs
- Substitution.cs
- TypefaceMap.cs
- ListItemParagraph.cs
- InstanceOwnerQueryResult.cs
- HtmlInputText.cs
- ByteStreamBufferedMessageData.cs
- input.cs
- SchemaCollectionCompiler.cs
- CompositeScriptReference.cs
- WebPartChrome.cs
- XmlHierarchicalDataSourceView.cs
- BamlBinaryReader.cs
- TokenBasedSet.cs
- ControlBuilderAttribute.cs
- _ListenerAsyncResult.cs
- TimerElapsedEvenArgs.cs
- CancelEventArgs.cs
- ProjectionPruner.cs
- RightsController.cs
- OptimizedTemplateContentHelper.cs
- ConstraintConverter.cs
- SessionParameter.cs
- ContourSegment.cs
- AttachedPropertiesService.cs
- Underline.cs
- XPathAncestorQuery.cs
- PrimitiveList.cs
- CorruptingExceptionCommon.cs
- BindingsCollection.cs
- HtmlDocument.cs
- EnumerableCollectionView.cs
- mediaeventshelper.cs
- RegexMatchCollection.cs
- ValidateNames.cs
- HtmlInputFile.cs
- BitmapFrameDecode.cs
- ArrayWithOffset.cs
- rsa.cs
- Cursors.cs
- Normalizer.cs
- ServiceReference.cs
- Menu.cs
- DateTimeFormatInfoScanner.cs
- CodeSubDirectoriesCollection.cs
- BitmapImage.cs
- Transform.cs
- FixedFindEngine.cs
- ToolStripPanelRow.cs
- DataGridViewRowStateChangedEventArgs.cs
- Brushes.cs
- ObjectCloneHelper.cs
- CustomTokenProvider.cs
- TileBrush.cs
- RIPEMD160.cs
- ArrayConverter.cs
- TrustSection.cs
- Rss20FeedFormatter.cs
- LightweightCodeGenerator.cs
- GlobalizationAssembly.cs
- InternalTypeHelper.cs
- pingexception.cs
- ParentQuery.cs
- TopClause.cs
- StackBuilderSink.cs
- SqlDataReader.cs
- JpegBitmapEncoder.cs
- SecurityHelper.cs
- Material.cs
- MultipartContentParser.cs
- ProgressPage.cs
- DeleteMemberBinder.cs
- WebPartCollection.cs
- LogSwitch.cs
- GlobalAllocSafeHandle.cs
- EmptyCollection.cs
- ipaddressinformationcollection.cs
- SqlUdtInfo.cs
- RectangleConverter.cs
- ChildChangedEventArgs.cs
- PreviewPageInfo.cs
- ExtensionQuery.cs
- BinaryObjectInfo.cs
- ScriptingSectionGroup.cs
- DataViewListener.cs
- XmlEnumAttribute.cs
- PathGeometry.cs
- OleTxTransaction.cs
- ColumnResult.cs
- LineGeometry.cs
- DesignerUtils.cs
- GeneralTransformGroup.cs
- MemberAccessException.cs