Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputFile.cs / 1 / HtmlInputFile.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputFile.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ValidationProperty("Value") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputFile : HtmlInputControl, IPostBackDataHandler { /* * Creates an intrinsic Html INPUT type=file control. */ ////// The ///class defines the /// methods, properties, and events for the control. This class allows /// programmatic access to the HTML <input type= file> element on the server. /// It provides access to the stream, as well as a useful SaveAs functionality /// provided by the /// property. /// /// This class only works if the /// HtmlForm.Enctype property is set to "multipart/form-data". /// Also, it does not maintain its /// state across multiple round trips between browser and server. If the user sets /// this value after a round trip, the value is lost. /// ////// public HtmlInputFile() : base("file") { } /* * Accept type property. */ ///Initializes a new instance of the ///class. /// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Accept { get { string s = Attributes["accept"]; return((s != null) ? s : String.Empty); } set { Attributes["accept"] = MapStringAttributeToString(value); } } /* * The property for the maximum characters allowed. */ ////// Gets /// or sets a comma-separated list of MIME encodings that /// can be used to constrain the file types that the browser lets the user /// select. For example, to restrict the /// selection to images, the accept value image/* should be specified. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int MaxLength { get { string s = Attributes["maxlength"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["maxlength"] = MapIntegerAttributeToString(value); } } /* * PostedFile property. */ ////// Gets or sets the /// maximum length of the file path of the file to upload /// from the client machine. /// ////// [ WebCategory("Default"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public HttpPostedFile PostedFile { get { return Context.Request.Files[RenderedNameAttribute];} } /* * The property for the width in characters. */ ///Gets access to the uploaded file specified by a client. ////// [ WebCategory("Appearance"), DefaultValue(-1), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Size { get { string s = Attributes["size"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["size"] = MapIntegerAttributeToString(value); } } // ASURT 122262 : The value property isn't submitted back to us when the a page // containing this control postsback, so required field validators are broken // (value would contain the empty string). To fix this, we return the filename. [ Browsable(false) ] public override string Value { get { HttpPostedFile postedFile = PostedFile; if (postedFile != null) { return postedFile.FileName; } return String.Empty; } set { // Throw here because setting the value on this tag has no effect on the // rendering behavior and since we're always returning the posted file's // filename, we don't want to get into a situation where the user // sets a value and does not get back that value. throw new NotSupportedException(SR.GetString(SR.Value_Set_Not_Supported, this.GetType().Name)); } } /* * Method of IPostBackDataHandler interface to process posted data. */ ///Gets or sets the width of the file-path text box that the /// browser displays when the ////// control is used on a page. bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { return false; } /* * Method of IPostBackDataHandler interface which is invoked whenever * posted data for a control has changed. RadioButton fires an * OnServerChange event. */ /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } protected virtual void RaisePostDataChangedEvent() { } /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); // ASURT 35328: use multipart encoding if no encoding is currently specified HtmlForm form = Page.Form; if (form != null && form.Enctype.Length == 0) { form.Enctype = "multipart/form-data"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Raises the ///event. This method uses event arguments /// to pass the event data to the control. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputFile.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ValidationProperty("Value") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputFile : HtmlInputControl, IPostBackDataHandler { /* * Creates an intrinsic Html INPUT type=file control. */ ////// The ///class defines the /// methods, properties, and events for the control. This class allows /// programmatic access to the HTML <input type= file> element on the server. /// It provides access to the stream, as well as a useful SaveAs functionality /// provided by the /// property. /// /// This class only works if the /// HtmlForm.Enctype property is set to "multipart/form-data". /// Also, it does not maintain its /// state across multiple round trips between browser and server. If the user sets /// this value after a round trip, the value is lost. /// ////// public HtmlInputFile() : base("file") { } /* * Accept type property. */ ///Initializes a new instance of the ///class. /// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Accept { get { string s = Attributes["accept"]; return((s != null) ? s : String.Empty); } set { Attributes["accept"] = MapStringAttributeToString(value); } } /* * The property for the maximum characters allowed. */ ////// Gets /// or sets a comma-separated list of MIME encodings that /// can be used to constrain the file types that the browser lets the user /// select. For example, to restrict the /// selection to images, the accept value image/* should be specified. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int MaxLength { get { string s = Attributes["maxlength"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["maxlength"] = MapIntegerAttributeToString(value); } } /* * PostedFile property. */ ////// Gets or sets the /// maximum length of the file path of the file to upload /// from the client machine. /// ////// [ WebCategory("Default"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public HttpPostedFile PostedFile { get { return Context.Request.Files[RenderedNameAttribute];} } /* * The property for the width in characters. */ ///Gets access to the uploaded file specified by a client. ////// [ WebCategory("Appearance"), DefaultValue(-1), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Size { get { string s = Attributes["size"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["size"] = MapIntegerAttributeToString(value); } } // ASURT 122262 : The value property isn't submitted back to us when the a page // containing this control postsback, so required field validators are broken // (value would contain the empty string). To fix this, we return the filename. [ Browsable(false) ] public override string Value { get { HttpPostedFile postedFile = PostedFile; if (postedFile != null) { return postedFile.FileName; } return String.Empty; } set { // Throw here because setting the value on this tag has no effect on the // rendering behavior and since we're always returning the posted file's // filename, we don't want to get into a situation where the user // sets a value and does not get back that value. throw new NotSupportedException(SR.GetString(SR.Value_Set_Not_Supported, this.GetType().Name)); } } /* * Method of IPostBackDataHandler interface to process posted data. */ ///Gets or sets the width of the file-path text box that the /// browser displays when the ////// control is used on a page. bool IPostBackDataHandler.LoadPostData(string postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } protected virtual bool LoadPostData(string postDataKey, NameValueCollection postCollection) { return false; } /* * Method of IPostBackDataHandler interface which is invoked whenever * posted data for a control has changed. RadioButton fires an * OnServerChange event. */ /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } protected virtual void RaisePostDataChangedEvent() { } /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); // ASURT 35328: use multipart encoding if no encoding is currently specified HtmlForm form = Page.Form; if (form != null && form.Enctype.Length == 0) { form.Enctype = "multipart/form-data"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Raises the ///event. This method uses event arguments /// to pass the event data to the control.
Link Menu
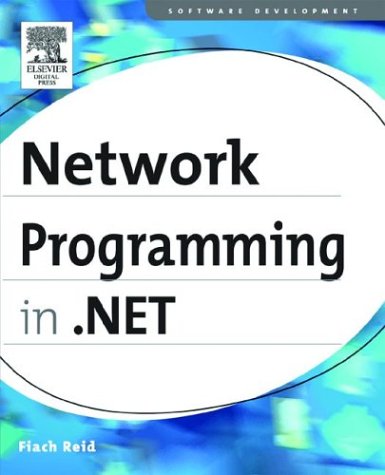
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataProtection.cs
- TableLayoutColumnStyleCollection.cs
- DataTemplateKey.cs
- BufferedGraphicsManager.cs
- Floater.cs
- TemplateContainer.cs
- XPathPatternParser.cs
- TypedRowGenerator.cs
- WebServiceClientProxyGenerator.cs
- IntegerValidatorAttribute.cs
- SignatureConfirmationElement.cs
- DescendantBaseQuery.cs
- webeventbuffer.cs
- BroadcastEventHelper.cs
- InputScopeAttribute.cs
- HttpModuleCollection.cs
- BrowserCapabilitiesCodeGenerator.cs
- UrlPath.cs
- SocketPermission.cs
- PostBackOptions.cs
- HttpPostedFile.cs
- TextFindEngine.cs
- FillErrorEventArgs.cs
- QilPatternFactory.cs
- ProxyWebPartConnectionCollection.cs
- XmlAttributeCollection.cs
- CompressedStack.cs
- RuntimeCompatibilityAttribute.cs
- InfoCardCryptoHelper.cs
- InstanceNameConverter.cs
- RuntimeWrappedException.cs
- CategoryValueConverter.cs
- FormatterConverter.cs
- SecurityResources.cs
- DBConnectionString.cs
- BuildTopDownAttribute.cs
- CustomSignedXml.cs
- ToolStripMenuItem.cs
- ButtonColumn.cs
- SubMenuStyle.cs
- RenameRuleObjectDialog.Designer.cs
- AudioBase.cs
- RenderData.cs
- XPathCompiler.cs
- SqlBuffer.cs
- LogicalTreeHelper.cs
- WinCategoryAttribute.cs
- __Error.cs
- Listbox.cs
- FreezableCollection.cs
- TableCellCollection.cs
- OdbcConnectionHandle.cs
- SettingsAttributeDictionary.cs
- WindowsListViewItem.cs
- CheckBoxField.cs
- TraceSection.cs
- SecurityTokenParametersEnumerable.cs
- SqlDataSourceCustomCommandEditor.cs
- Rect3DValueSerializer.cs
- TypographyProperties.cs
- Transform3DGroup.cs
- TextEditorCopyPaste.cs
- tooltip.cs
- EventMap.cs
- List.cs
- ToolStripStatusLabel.cs
- Positioning.cs
- HwndHost.cs
- DataRowChangeEvent.cs
- TargetFrameworkUtil.cs
- OleStrCAMarshaler.cs
- GiveFeedbackEventArgs.cs
- Style.cs
- SafePEFileHandle.cs
- SqlTypeSystemProvider.cs
- RectConverter.cs
- DispatchProxy.cs
- WebPartPersonalization.cs
- ChangePassword.cs
- DefaultWorkflowTransactionService.cs
- DoWorkEventArgs.cs
- MetadataArtifactLoaderCompositeResource.cs
- XmlSchemaProviderAttribute.cs
- TriState.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- XmlDataProvider.cs
- ByteStorage.cs
- HtmlPageAdapter.cs
- PerfService.cs
- TextElementEditingBehaviorAttribute.cs
- ResourceReferenceKeyNotFoundException.cs
- HostingPreferredMapPath.cs
- EncoderNLS.cs
- TextBox.cs
- HtmlInputFile.cs
- DocumentViewerBase.cs
- ToolStripItemTextRenderEventArgs.cs
- DivideByZeroException.cs
- RequestTimeoutManager.cs
- ValueTable.cs