Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / NumericUpDownAccelerationCollection.cs / 1 / NumericUpDownAccelerationCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using System.ComponentModel; ////// Represents a SORTED collection of NumericUpDownAcceleration objects in the NumericUpDown Control. /// The elements in the collection are sorted by the NumericUpDownAcceleration.Seconds property. /// [ListBindable(false)] public class NumericUpDownAccelerationCollection : MarshalByRefObject, ICollection, IEnumerable { List items; /// ICollection implementation. /// /// Adds an item (NumericUpDownAcceleration object) to the ICollection. /// The item is added preserving the collection sorted. /// public void Add(NumericUpDownAcceleration acceleration) { if( acceleration == null ) { throw new ArgumentNullException("acceleration"); } // Keep the array sorted, insert in the right spot. int index = 0; while( index < this.items.Count ) { if( acceleration.Seconds < this.items[index].Seconds ) { break; } index++; } this.items.Insert(index, acceleration); } ////// Removes all items from the ICollection. /// public void Clear() { this.items.Clear(); } ////// Determines whether the IList contains a specific value. /// public bool Contains(NumericUpDownAcceleration acceleration) { return this.items.Contains(acceleration); } ////// Copies the elements of the ICollection to an Array, starting at a particular Array index. /// public void CopyTo(NumericUpDownAcceleration[] array, int index) { this.items.CopyTo(array, index); } ////// Gets the number of elements contained in the ICollection. /// public int Count { get {return this.items.Count;} } ////// Gets a value indicating whether the ICollection is read-only. /// This collection property returns false always. /// public bool IsReadOnly { get {return false;} } ////// Removes the specified item from the ICollection. /// public bool Remove(NumericUpDownAcceleration acceleration) { return this.items.Remove(acceleration); } /// IEnumerableimplementation. /// /// Returns an enumerator that can iterate through the collection. /// IEnumeratorIEnumerable .GetEnumerator() { return this.items.GetEnumerator(); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable)items).GetEnumerator(); } /// NumericUpDownAccelerationCollection methods. /// /// Class constructor. /// public NumericUpDownAccelerationCollection() { this.items = new List(); } /// /// Adds the elements of specified array to the collection, keeping the collection sorted. /// public void AddRange(params NumericUpDownAcceleration[] accelerations) { if (accelerations == null) { throw new ArgumentNullException("accelerations"); } // Accept the range only if ALL elements in the array are not null. foreach (NumericUpDownAcceleration acceleration in accelerations) { if (acceleration == null) { throw new ArgumentNullException(SR.GetString(SR.NumericUpDownAccelerationCollectionAtLeastOneEntryIsNull)); } } // The expected array size is typically small (5 items?), so we don't need to try to be smarter about the // way we add the elements to the collection, just call Add. foreach (NumericUpDownAcceleration acceleration in accelerations) { this.Add(acceleration); } } ////// Gets (ReadOnly) the element at the specified index. In C#, this property is the indexer for /// the IList class. /// public NumericUpDownAcceleration this[int index] { get { return this.items[index]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
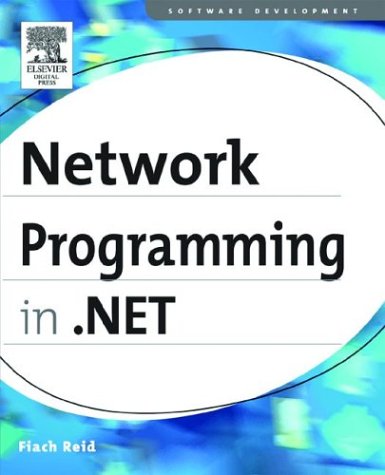
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HMACRIPEMD160.cs
- CollectionMarkupSerializer.cs
- ListSortDescriptionCollection.cs
- RecordManager.cs
- SettingsAttributeDictionary.cs
- ModelPropertyCollectionImpl.cs
- MenuItemStyle.cs
- GradientStop.cs
- UserCancellationException.cs
- CreateUserErrorEventArgs.cs
- GcHandle.cs
- WebReferencesBuildProvider.cs
- Or.cs
- RootBrowserWindowAutomationPeer.cs
- IpcClientManager.cs
- AppDomainShutdownMonitor.cs
- Variant.cs
- CompleteWizardStep.cs
- PageContentAsyncResult.cs
- StreamResourceInfo.cs
- InputGestureCollection.cs
- Util.cs
- SQlBooleanStorage.cs
- WebDescriptionAttribute.cs
- IOThreadScheduler.cs
- ValidatingPropertiesEventArgs.cs
- _SafeNetHandles.cs
- UTF7Encoding.cs
- SQLSingleStorage.cs
- MetadataItemEmitter.cs
- SchemaCollectionPreprocessor.cs
- DataRecordInternal.cs
- SmtpTransport.cs
- ElementsClipboardData.cs
- HwndStylusInputProvider.cs
- UserControl.cs
- PropertyTabAttribute.cs
- ObjectHandle.cs
- SequenceDesigner.cs
- XmlUnspecifiedAttribute.cs
- XmlTextAttribute.cs
- TextPatternIdentifiers.cs
- InputLanguage.cs
- ComponentChangingEvent.cs
- EdmValidator.cs
- ReferentialConstraintRoleElement.cs
- RecognitionResult.cs
- CaseExpr.cs
- AdCreatedEventArgs.cs
- XmlSchemaAnnotation.cs
- CallbackValidator.cs
- WindowPatternIdentifiers.cs
- TextRunTypographyProperties.cs
- CompilationUnit.cs
- CatalogPartCollection.cs
- SyntaxCheck.cs
- versioninfo.cs
- ConfigurationValue.cs
- GraphicsState.cs
- DataDesignUtil.cs
- documentsequencetextpointer.cs
- XmlQueryCardinality.cs
- PropertyItem.cs
- OleDbTransaction.cs
- Logging.cs
- XmlBinaryReader.cs
- StaticTextPointer.cs
- Win32SafeHandles.cs
- Bezier.cs
- AmbientProperties.cs
- webbrowsersite.cs
- ToolboxItemFilterAttribute.cs
- EntityDataSourceValidationException.cs
- MutexSecurity.cs
- AbstractDataSvcMapFileLoader.cs
- FixedPageProcessor.cs
- ReadContentAsBinaryHelper.cs
- AppDomainProtocolHandler.cs
- ControlEvent.cs
- XmlIlTypeHelper.cs
- StaticContext.cs
- DuplexClientBase.cs
- SynchronousReceiveElement.cs
- TimeZone.cs
- ILGenerator.cs
- WebServiceFaultDesigner.cs
- FlowLayoutPanel.cs
- SpeechDetectedEventArgs.cs
- NotificationContext.cs
- SystemException.cs
- WebPartTransformerAttribute.cs
- FormsAuthenticationModule.cs
- DetailsViewUpdatedEventArgs.cs
- DataServiceQuery.cs
- ReflectionTypeLoadException.cs
- XmlDataSourceView.cs
- AnnotationAuthorChangedEventArgs.cs
- DataGridViewLinkCell.cs
- AgileSafeNativeMemoryHandle.cs
- SettingsAttributes.cs