Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / System / Windows / Generated / SizeConverter.cs / 1 / SizeConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// SizeConverter - Converter class for converting instances of other types to and from Size instances /// public sealed class SizeConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Size from the given object. /// ////// The Size which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Size. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Size. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Size.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Size to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Size, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Size instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Size) { Size instance = (Size)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows { ////// SizeConverter - Converter class for converting instances of other types to and from Size instances /// public sealed class SizeConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Size from the given object. /// ////// The Size which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Size. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Size. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Size.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Size to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Size, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Size instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Size) { Size instance = (Size)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
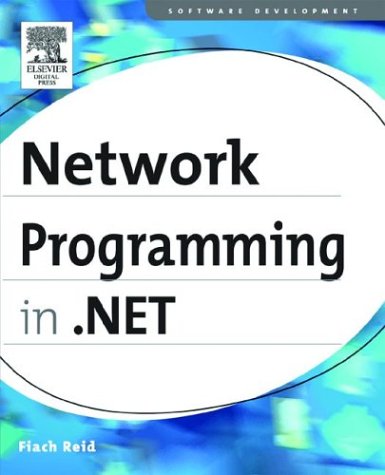
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataControlLinkButton.cs
- IdentifierCreationService.cs
- CodeTypeParameter.cs
- EntityDataSourceColumn.cs
- CloseSequenceResponse.cs
- ExtensionElement.cs
- XmlSchemaAttributeGroup.cs
- Image.cs
- TrustManagerPromptUI.cs
- BlobPersonalizationState.cs
- RsaKeyIdentifierClause.cs
- TraceHandler.cs
- StringUtil.cs
- PatternMatcher.cs
- ObjectDataSourceView.cs
- WebDisplayNameAttribute.cs
- WebRequestModulesSection.cs
- Util.cs
- ExpressionVisitor.cs
- ParserOptions.cs
- ImageMapEventArgs.cs
- StylusPointPropertyInfo.cs
- BooleanFunctions.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- MimeTypePropertyAttribute.cs
- NonVisualControlAttribute.cs
- InternalSafeNativeMethods.cs
- BindingList.cs
- EpmSyndicationContentDeSerializer.cs
- GcSettings.cs
- TraceListener.cs
- DataGridViewCellCollection.cs
- PublisherMembershipCondition.cs
- Help.cs
- sitestring.cs
- Parser.cs
- XamlToRtfParser.cs
- SolidColorBrush.cs
- DataIdProcessor.cs
- SessionPageStatePersister.cs
- TableLayoutPanelCellPosition.cs
- XhtmlBasicFormAdapter.cs
- BitmapEffectDrawingContextWalker.cs
- CookielessHelper.cs
- Separator.cs
- XsltOutput.cs
- MessageQueueKey.cs
- DefaultBindingPropertyAttribute.cs
- EntryIndex.cs
- GroupBoxAutomationPeer.cs
- TrustManagerMoreInformation.cs
- DiagnosticTraceSource.cs
- updatecommandorderer.cs
- BulletChrome.cs
- Exceptions.cs
- XmlWriter.cs
- MimeTypeMapper.cs
- safemediahandle.cs
- _SslState.cs
- CultureSpecificCharacterBufferRange.cs
- DialogResultConverter.cs
- TransportSecurityProtocol.cs
- AsyncStreamReader.cs
- ErrorTableItemStyle.cs
- DataGridParentRows.cs
- PaperSource.cs
- FontStyleConverter.cs
- XmlEncodedRawTextWriter.cs
- TagPrefixAttribute.cs
- XmlDictionaryString.cs
- PropertyFilterAttribute.cs
- DrawingContextDrawingContextWalker.cs
- IQueryable.cs
- __Error.cs
- InputProcessorProfilesLoader.cs
- HtmlLink.cs
- WebPartCatalogAddVerb.cs
- DataRow.cs
- LicenseException.cs
- InputScopeAttribute.cs
- ResizeBehavior.cs
- Pens.cs
- ApplicationException.cs
- InkCanvasSelection.cs
- PartitionedStreamMerger.cs
- XmlSchemaImporter.cs
- EndpointIdentityExtension.cs
- NavigationService.cs
- SchemaLookupTable.cs
- MsmqProcessProtocolHandler.cs
- Compress.cs
- IdentifierCollection.cs
- CodeObjectCreateExpression.cs
- RtfNavigator.cs
- TimerElapsedEvenArgs.cs
- StringFormat.cs
- Point3DAnimationUsingKeyFrames.cs
- StringComparer.cs
- TouchDevice.cs
- TableCell.cs