Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Discovery / Configuration / EndpointDiscoveryElement.cs / 1305376 / EndpointDiscoveryElement.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Discovery.Configuration { using System.Configuration; using System.Diagnostics.CodeAnalysis; using System.Runtime; using System.ServiceModel.Configuration; using System.Xml.Linq; using System.Xml; public sealed class EndpointDiscoveryElement : BehaviorExtensionElement { ConfigurationPropertyCollection properties; public EndpointDiscoveryElement() { } [SuppressMessage(FxCop.Category.Configuration, FxCop.Rule.ConfigurationPropertyAttributeRule, Justification = "This property is defined by the base class to determine the type of the behavior.")] public override Type BehaviorType { get { return typeof(EndpointDiscoveryBehavior); } } [ConfigurationProperty(ConfigurationStrings.Enabled, DefaultValue = true)] public bool Enabled { get { return (bool)base[ConfigurationStrings.Enabled]; } set { base[ConfigurationStrings.Enabled] = value; } } [ConfigurationProperty(ConfigurationStrings.Types)] [SuppressMessage( FxCop.Category.Configuration, FxCop.Rule.ConfigurationPropertyNameRule, Justification = "The configuration name for this element is 'types'.")] public ContractTypeNameElementCollection ContractTypeNames { get { return (ContractTypeNameElementCollection)base[ConfigurationStrings.Types]; } } [ConfigurationProperty(ConfigurationStrings.Scopes)] public ScopeElementCollection Scopes { get { return (ScopeElementCollection)base[ConfigurationStrings.Scopes]; } } [ConfigurationProperty(ConfigurationStrings.Extensions)] public XmlElementElementCollection Extensions { get { return (XmlElementElementCollection)base[ConfigurationStrings.Extensions]; } } protected override ConfigurationPropertyCollection Properties { get { if (this.properties == null) { ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); properties.Add( new ConfigurationProperty( ConfigurationStrings.Enabled, typeof(Boolean), true, null, null, ConfigurationPropertyOptions.None)); properties.Add( new ConfigurationProperty( ConfigurationStrings.Types, typeof(ContractTypeNameElementCollection), null, null, null, ConfigurationPropertyOptions.None)); properties.Add( new ConfigurationProperty( ConfigurationStrings.Scopes, typeof(ScopeElementCollection), null, null, null, ConfigurationPropertyOptions.None)); properties.Add( new ConfigurationProperty( ConfigurationStrings.Extensions, typeof(XmlElementElementCollection), null, null, null, ConfigurationPropertyOptions.None)); this.properties = properties; } return this.properties; } } protected internal override object CreateBehavior() { EndpointDiscoveryBehavior behavior = new EndpointDiscoveryBehavior(); behavior.Enabled = Enabled; if ((Scopes != null) && (Scopes.Count > 0)) { foreach (ScopeElement scopeElement in Scopes) { behavior.Scopes.Add(scopeElement.Scope); } } if (ContractTypeNames != null) { foreach (ContractTypeNameElement contractTypeNameElement in ContractTypeNames) { behavior.ContractTypeNames.Add( new XmlQualifiedName(contractTypeNameElement.Name, contractTypeNameElement.Namespace)); } } if ((Extensions != null) && (Extensions.Count > 0)) { foreach (XmlElementElement xmlElement in Extensions) { behavior.Extensions.Add(XElement.Parse(xmlElement.XmlElement.OuterXml)); } } return behavior; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
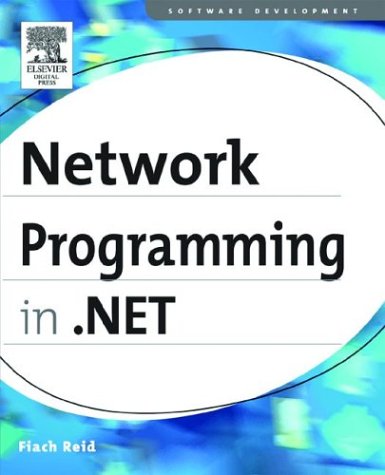
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionProviderAttribute.cs
- ControlCachePolicy.cs
- SmiMetaDataProperty.cs
- connectionpool.cs
- DefinitionBase.cs
- Quaternion.cs
- RijndaelManaged.cs
- PageCodeDomTreeGenerator.cs
- ControlAdapter.cs
- InkCanvas.cs
- RowUpdatedEventArgs.cs
- EmptyStringExpandableObjectConverter.cs
- VectorAnimationUsingKeyFrames.cs
- CalendarData.cs
- DragCompletedEventArgs.cs
- DataGridViewCellStyle.cs
- CommittableTransaction.cs
- Function.cs
- SmiEventStream.cs
- DescendentsWalkerBase.cs
- Floater.cs
- HostingEnvironment.cs
- CellTreeSimplifier.cs
- AutomationIdentifierGuids.cs
- ResourceSet.cs
- ProtocolsConfigurationEntry.cs
- SuspendDesigner.cs
- PeerUnsafeNativeCryptMethods.cs
- SafeLocalMemHandle.cs
- ParserHooks.cs
- ArraySegment.cs
- Connector.xaml.cs
- HandoffBehavior.cs
- TdsParser.cs
- IdentifierService.cs
- PagedDataSource.cs
- TdsEnums.cs
- WinFormsUtils.cs
- Point3DCollectionConverter.cs
- DataBoundControlHelper.cs
- SortKey.cs
- Documentation.cs
- SmtpDigestAuthenticationModule.cs
- FactoryMaker.cs
- PreProcessor.cs
- TextWriterTraceListener.cs
- MonitoringDescriptionAttribute.cs
- BaseParaClient.cs
- WebPartExportVerb.cs
- TypeElementCollection.cs
- Size.cs
- NetworkAddressChange.cs
- SQLInt32Storage.cs
- HttpRuntime.cs
- XmlCodeExporter.cs
- MediaScriptCommandRoutedEventArgs.cs
- NativeMethods.cs
- XmlValueConverter.cs
- ContentElementCollection.cs
- WindowsSpinner.cs
- TrackBar.cs
- TextHintingModeValidation.cs
- HiddenField.cs
- SelectionChangedEventArgs.cs
- MinMaxParagraphWidth.cs
- UITypeEditors.cs
- QilReference.cs
- OracleException.cs
- _SSPIWrapper.cs
- HashMembershipCondition.cs
- QilTargetType.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- CellTreeNode.cs
- TemplatePropertyEntry.cs
- SiteMapSection.cs
- HebrewCalendar.cs
- RegexNode.cs
- GridSplitterAutomationPeer.cs
- DesignerTransaction.cs
- InputBinding.cs
- WrappedIUnknown.cs
- ArgumentException.cs
- WrapperEqualityComparer.cs
- WebPartTransformerAttribute.cs
- DataGridViewLayoutData.cs
- Membership.cs
- HelpKeywordAttribute.cs
- TextClipboardData.cs
- XmlWrappingReader.cs
- XmlLanguage.cs
- relpropertyhelper.cs
- FieldMetadata.cs
- CapabilitiesSection.cs
- TcpClientSocketManager.cs
- FixedTextPointer.cs
- TemplateNameScope.cs
- ObjectQuery_EntitySqlExtensions.cs
- PackageDigitalSignature.cs
- InkCanvas.cs
- StaticFileHandler.cs