Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / Documentation.cs / 2 / Documentation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; using System.Xml; using System.Data; using System.Data.Common.Utils; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for Documentation. /// internal sealed class DocumentationElement: SchemaElement { #region Instance Fields Documentation _metdataDocumentation = new Documentation(); #endregion #region Public Methods ////// /// /// public DocumentationElement(SchemaElement parentElement) : base(parentElement) { } ////// replace troublesome xml characters with equivalent entities /// /// text that make have characters troublesome in xml ///text with troublesome characters replaced with equivalent entities [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll public static string Entityize(string text) { if ( string.IsNullOrEmpty(text) ) return ""; text = text.Replace("&","&"); text = text.Replace("<","<").Replace(">",">"); return text.Replace("\'","'").Replace("\"","""); } #endregion #region Public Properties ////// Returns the wrapped metaDocumentation instance /// public Documentation MetadataDocumentation { get { _metdataDocumentation.SetReadOnly(); return _metdataDocumentation; } } #endregion #region Protected Properties protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.Summary)) { HandleSummaryElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.LongDescription)) { HandleLongDescriptionElement(reader); return true; } return false; } #endregion #region Private Methods protected override bool HandleText(XmlReader reader) { string text = reader.Value; if (!StringUtil.IsNullOrEmptyOrWhiteSpace(text)) { AddError(ErrorCode.UnexpectedXmlElement, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidDocumentationBothTextAndStructure); } return true; } ////// /// /// private void HandleSummaryElement(XmlReader reader) { TextElement text = new TextElement(this); text.Parse(reader); _metdataDocumentation.Summary = text.Value; } ////// /// /// private void HandleLongDescriptionElement(XmlReader reader) { TextElement text = new TextElement(this); text.Parse(reader); _metdataDocumentation.LongDescription = text.Value; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; using System.Xml; using System.Data; using System.Data.Common.Utils; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for Documentation. /// internal sealed class DocumentationElement: SchemaElement { #region Instance Fields Documentation _metdataDocumentation = new Documentation(); #endregion #region Public Methods ////// /// /// public DocumentationElement(SchemaElement parentElement) : base(parentElement) { } ////// replace troublesome xml characters with equivalent entities /// /// text that make have characters troublesome in xml ///text with troublesome characters replaced with equivalent entities [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] // referenced by System.Data.Entity.Design.dll public static string Entityize(string text) { if ( string.IsNullOrEmpty(text) ) return ""; text = text.Replace("&","&"); text = text.Replace("<","<").Replace(">",">"); return text.Replace("\'","'").Replace("\"","""); } #endregion #region Public Properties ////// Returns the wrapped metaDocumentation instance /// public Documentation MetadataDocumentation { get { _metdataDocumentation.SetReadOnly(); return _metdataDocumentation; } } #endregion #region Protected Properties protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.Summary)) { HandleSummaryElement(reader); return true; } else if (CanHandleElement(reader, XmlConstants.LongDescription)) { HandleLongDescriptionElement(reader); return true; } return false; } #endregion #region Private Methods protected override bool HandleText(XmlReader reader) { string text = reader.Value; if (!StringUtil.IsNullOrEmptyOrWhiteSpace(text)) { AddError(ErrorCode.UnexpectedXmlElement, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidDocumentationBothTextAndStructure); } return true; } ////// /// /// private void HandleSummaryElement(XmlReader reader) { TextElement text = new TextElement(this); text.Parse(reader); _metdataDocumentation.Summary = text.Value; } ////// /// /// private void HandleLongDescriptionElement(XmlReader reader) { TextElement text = new TextElement(this); text.Parse(reader); _metdataDocumentation.LongDescription = text.Value; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
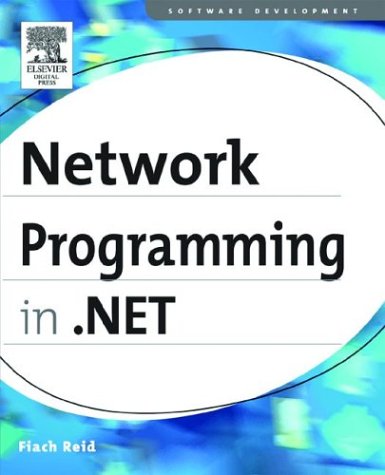
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Fonts.cs
- DocumentOrderQuery.cs
- DataTable.cs
- DecoratedNameAttribute.cs
- CodeCompiler.cs
- XPathLexer.cs
- EventData.cs
- XmlObjectSerializerReadContextComplexJson.cs
- FontNamesConverter.cs
- DataRelation.cs
- SecurityCriticalDataForSet.cs
- ReadOnlyNameValueCollection.cs
- ObjectResult.cs
- ECDsa.cs
- PriorityChain.cs
- ProfileSettingsCollection.cs
- FontStyle.cs
- ScrollBarAutomationPeer.cs
- MessageQueueInstaller.cs
- InboundActivityHelper.cs
- PageStatePersister.cs
- SqlIdentifier.cs
- BindingExpression.cs
- SafeReadContext.cs
- ToolboxComponentsCreatingEventArgs.cs
- MobileContainerDesigner.cs
- FormViewDeleteEventArgs.cs
- UndoEngine.cs
- WaitHandleCannotBeOpenedException.cs
- ProgressPage.cs
- Win32NamedPipes.cs
- FixedStringLookup.cs
- X500Name.cs
- TextTabProperties.cs
- FrameAutomationPeer.cs
- _AutoWebProxyScriptWrapper.cs
- WebUtility.cs
- mediaeventshelper.cs
- EntityDataSourceQueryBuilder.cs
- TemplateParser.cs
- PcmConverter.cs
- PerfCounters.cs
- ZipIOExtraFieldElement.cs
- PoisonMessageException.cs
- AtomEntry.cs
- Listen.cs
- RewritingSimplifier.cs
- PropertyValueUIItem.cs
- ContextMenu.cs
- Hex.cs
- ObjectItemAssemblyLoader.cs
- Perspective.cs
- DelegateBodyWriter.cs
- LinqExpressionNormalizer.cs
- FacetChecker.cs
- ExpressionBinding.cs
- WorkBatch.cs
- RenderDataDrawingContext.cs
- HMACMD5.cs
- BitmapEffectDrawingContextState.cs
- BinaryReader.cs
- InlineObject.cs
- XmlEntityReference.cs
- Utils.cs
- Types.cs
- Binding.cs
- BaseCodePageEncoding.cs
- WebPartZoneCollection.cs
- SqlInternalConnection.cs
- ClassicBorderDecorator.cs
- DiagnosticTraceSchemas.cs
- GenericUriParser.cs
- ItemContainerGenerator.cs
- DataGridAddNewRow.cs
- ToolStripSeparator.cs
- NativeMethods.cs
- Parameter.cs
- GridProviderWrapper.cs
- TokenBasedSet.cs
- GeneralTransform3DTo2DTo3D.cs
- ServiceChannelProxy.cs
- Evidence.cs
- PngBitmapDecoder.cs
- GlobalizationSection.cs
- OleDbParameterCollection.cs
- ToolStripContainerDesigner.cs
- HtmlContainerControl.cs
- XmlAnyElementAttributes.cs
- controlskin.cs
- AutoResizedEvent.cs
- NamespaceList.cs
- IsolatedStorageException.cs
- Line.cs
- XmlSchemaRedefine.cs
- Vector3DCollectionValueSerializer.cs
- XmlElementAttribute.cs
- Grid.cs
- PropertyTabAttribute.cs
- ObjectResult.cs
- XmlSchemaSet.cs