Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / DiagnosticTraceSchemas.cs / 1 / DiagnosticTraceSchemas.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file implements the schemas for the trace records published by WS-AT using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.Runtime.Serialization; using System.ServiceModel; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.Messaging; using System.Globalization; using System.ServiceModel.Diagnostics; namespace Microsoft.Transactions.Wsat.Protocol { [DataContract (Name = "UnhandledStateMachineException")] class UnhandledStateMachineExceptionRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "UnhandledStateMachineException" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.UnhandledStateMachineExceptionRecordSchema, this.transactionId, this.stateMachine, this.currentState, this.history != null ? this.history.ToString() : string.Empty); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "StateMachine", IsRequired=true)] string stateMachine; [DataMember (Name = "CurrentState", IsRequired=true)] string currentState; [DataMember (Name = "TransitionHistory")] StateMachineHistory history; public UnhandledStateMachineExceptionRecordSchema ( string transactionId, string stateMachine, string currentState, StateMachineHistory history) { this.transactionId = transactionId; this.stateMachine = stateMachine; this.currentState = currentState; this.history = history; } } [DataContract (Name = "UnexpectedStateMachineEvent")] class UnexpectedStateMachineEventRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "UnexpectedStateMachineEvent" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.UnexpectedStateMachineEventRecordSchema, this.transactionId, this.stateMachine, this.currentState, this.history != null ? this.history.ToString() : string.Empty, this.unexpectedEvent, this.unexpectedEventDetails); } [DataMember(Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "StateMachine", IsRequired=true)] string stateMachine; [DataMember (Name = "CurrentState", IsRequired=true)] string currentState; [DataMember (Name = "TransitionHistory")] StateMachineHistory history; [DataMember (Name = "UnexpectedEvent", IsRequired=true)] string unexpectedEvent; [DataMember (Name = "UnexpectedEventDetails")] string unexpectedEventDetails; public UnexpectedStateMachineEventRecordSchema ( string transactionId, string stateMachine, string currentState, StateMachineHistory history, string unexpectedEvent, string unexpectedEventDetails ) { this.transactionId = transactionId; this.stateMachine = stateMachine; this.currentState = currentState; this.history = history; this.unexpectedEvent = unexpectedEvent; this.unexpectedEventDetails = unexpectedEventDetails; } } [DataContract (Name = "RecoveryLogEntry")] class RecoveryLogEntryRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "RecoveryLogEntry" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.RecoveryLogEntryRecordSchema, this.transactionId, this.RecoveryDataLength.ToString(CultureInfo.CurrentCulture), this.RecoveryDataBase64); } [DataMember(Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "RecoveryDataLength", IsRequired=true)] long RecoveryDataLength { get { return this.recoveryData.Length; } set { } } [DataMember (Name = "RecoveryDataBase64", IsRequired=true)] string RecoveryDataBase64 { get { return Convert.ToBase64String (this.recoveryData); } set { } } byte[] recoveryData; public RecoveryLogEntryRecordSchema (string transactionId, byte[] recoveryData) { this.transactionId = transactionId; this.recoveryData = recoveryData; if (this.recoveryData == null) { this.recoveryData = new byte[0]; } } } [DataContract (Name = "Transaction")] class TransactionRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "Transaction" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; public TransactionRecordSchema (string transactionId) { this.transactionId = transactionId; } } [DataContract (Name = "Enlistment")] class EnlistmentRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "Enlistment" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; public EnlistmentRecordSchema(string transactionId, Guid enlistmentId) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; } } [DataContract (Name = "Reason")] class ReasonRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "Reason" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Reason", IsRequired=true)] string reason; public ReasonRecordSchema (string reason) { this.reason = reason; } } [DataContract (Name = "ReasonWithTransactionId")] class ReasonWithTransactionIdRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "ReasonWithTransactionId" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.ReasonWithTransactionIdRecordSchema, this.transactionId, this.reason); } [DataMember(Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "Reason", IsRequired=true)] string reason; public ReasonWithTransactionIdRecordSchema (string transactionId, string reason) { this.transactionId = transactionId; this.reason = reason; } } [DataContract (Name = "ReasonWithEnlistment")] class ReasonWithEnlistmentRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "ReasonWithEnlistment" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.ReasonWithEnlistmentRecordSchema, this.transactionId, this.enlistmentId, this.reason); } [DataMember(Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; [DataMember (Name = "Reason", IsRequired=true)] string reason; public ReasonWithEnlistmentRecordSchema(string transactionId, Guid enlistmentId, string reason) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; this.reason = reason; } } [DataContract (Name = "CoordinationContext")] class CoordinationContextRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "CoordinationContext" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Context", IsRequired=true)] CoordinationContext context; public CoordinationContextRecordSchema (CoordinationContext context) { this.context = context; } } //=================================================================================== // RegisterFailureRecordSchema classes //=================================================================================== [DataContract (Name = "RegisterFailure")] abstract class RegisterFailureRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString(); } set { } } ControlProtocol protocol; [DataMember (Name = "Reason", IsRequired=true)] string reason; protected RegisterFailureRecordSchema ( string transactionId, ControlProtocol protocol, string reason ) { this.transactionId = transactionId; this.protocol = protocol; this.reason = reason; } public static RegisterFailureRecordSchema Instance(string transactionId, ControlProtocol protocol, EndpointAddress protocolService, string reason, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RegisterFailureRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RegisterFailureRecordSchema10(transactionId, protocol, protocolService, reason); case ProtocolVersion.Version11 : return new RegisterFailureRecordSchema11(transactionId, protocol, protocolService, reason); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RegisterFailure10")] class RegisterFailureRecordSchema10 : RegisterFailureRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterFailure" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ProtocolService", IsRequired=true)] EndpointAddressAugust2004 protocolService; public RegisterFailureRecordSchema10 ( string transactionId, ControlProtocol protocol, EndpointAddress protocolService, string reason) : base(transactionId, protocol, reason) { this.schemaId = id; if (protocolService != null) { this.protocolService = EndpointAddressAugust2004.FromEndpointAddress(protocolService); } } } [DataContract (Name = "RegisterFailure11")] class RegisterFailureRecordSchema11 : RegisterFailureRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterFailure11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ProtocolService", IsRequired=true)] EndpointAddress10 protocolService; public RegisterFailureRecordSchema11 ( string transactionId, ControlProtocol protocol, EndpointAddress protocolService, string reason) : base(transactionId, protocol, reason) { this.schemaId = id; if (protocolService != null) { this.protocolService = EndpointAddress10.FromEndpointAddress(protocolService); } } } //==================================================================================== [DataContract (Name = "EnlistmentTimeout")] class EnlistmentTimeoutRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "EnlistmentTimeout" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; [DataMember (Name = "Outcome", IsRequired=true)] string Outcome { get { return this.outcome.ToString(); } set { } } TransactionOutcome outcome; [DataMember (Name = "Timeout", IsRequired=true)] string Timeout { get { return this.timeout.ToString(); } set { } } TimeSpan timeout; public EnlistmentTimeoutRecordSchema ( string transactionId, Guid enlistmentId, TransactionOutcome outcome, TimeSpan timeout ) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; this.outcome = outcome; this.timeout = timeout; } } //=================================================================================== // VolatileEnlistmentInDoubtRecordSchema classes //==================================================================================== [DataContract (Name = "VolatileEnlistmentInDoubt")] abstract class VolatileEnlistmentInDoubtRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; protected VolatileEnlistmentInDoubtRecordSchema ( Guid enlistmentId ) { this.enlistmentId = enlistmentId; } public static VolatileEnlistmentInDoubtRecordSchema Instance(Guid enlistmentId, EndpointAddress replyTo, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(VolatileEnlistmentInDoubtRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new VolatileEnlistmentInDoubtRecordSchema10(enlistmentId, replyTo); case ProtocolVersion.Version11 : return new VolatileEnlistmentInDoubtRecordSchema11(enlistmentId, replyTo); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "VolatileEnlistmentInDoubt10")] class VolatileEnlistmentInDoubtRecordSchema10 : VolatileEnlistmentInDoubtRecordSchema { const string id = TraceRecord.EventIdBase + "VolatileEnlistmentInDoubt" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ReplyTo")] EndpointAddressAugust2004 replyTo; public VolatileEnlistmentInDoubtRecordSchema10 ( Guid enlistmentId, EndpointAddress replyTo ) : base(enlistmentId) { this.schemaId = id; if (replyTo != null) { this.replyTo = EndpointAddressAugust2004.FromEndpointAddress(replyTo); } } } [DataContract (Name = "VolatileEnlistmentInDoubt11")] class VolatileEnlistmentInDoubtRecordSchema11 : VolatileEnlistmentInDoubtRecordSchema { const string id = TraceRecord.EventIdBase + "VolatileEnlistmentInDoubt11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ReplyTo")] EndpointAddress10 replyTo; public VolatileEnlistmentInDoubtRecordSchema11 ( Guid enlistmentId, EndpointAddress replyTo ) : base(enlistmentId) { this.schemaId = id; if (replyTo != null) { this.replyTo = EndpointAddress10.FromEndpointAddress(replyTo); } } } //==================================================================================== // RegisterCoordinatorRecordSchema classes //=================================================================================== [DataContract (Name = "RegisterCoordinator")] abstract class RegisterCoordinatorRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Context", IsRequired=true)] CoordinationContext context; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString(); } set { } } ControlProtocol protocol; protected RegisterCoordinatorRecordSchema ( CoordinationContext context, ControlProtocol protocol ) { this.context = context; this.protocol = protocol; } public static RegisterCoordinatorRecordSchema Instance(CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RegisterCoordinatorRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RegisterCoordinatorRecordSchema10(context, protocol, coordinatorService); case ProtocolVersion.Version11 : return new RegisterCoordinatorRecordSchema11(context, protocol, coordinatorService); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RegisterCoordinator10")] class RegisterCoordinatorRecordSchema10 : RegisterCoordinatorRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterCoordinator" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService", IsRequired=true)] EndpointAddressAugust2004 coordinatorService; public RegisterCoordinatorRecordSchema10 ( CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService ) : base(context, protocol) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddressAugust2004.FromEndpointAddress(coordinatorService); } } } [DataContract (Name = "RegisterCoordinator11")] class RegisterCoordinatorRecordSchema11 : RegisterCoordinatorRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterCoordinator11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService", IsRequired=true)] EndpointAddress10 coordinatorService; public RegisterCoordinatorRecordSchema11 ( CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService ) : base(context, protocol) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddress10.FromEndpointAddress(coordinatorService); } } } //==================================================================================== // RegisterParticipantRecordSchema classes //=================================================================================== [DataContract (Name = "RegisterParticipant")] abstract class RegisterParticipantRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString(); } set { } } ControlProtocol protocol; protected RegisterParticipantRecordSchema ( string transactionId, Guid enlistmentId, ControlProtocol protocol ) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; this.protocol = protocol; } public static RegisterParticipantRecordSchema Instance(string transactionId, Guid enlistmentId, ControlProtocol protocol, EndpointAddress participantService, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RegisterParticipantRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RegisterParticipantRecordSchema10(transactionId, enlistmentId, protocol, participantService); case ProtocolVersion.Version11 : return new RegisterParticipantRecordSchema11(transactionId, enlistmentId, protocol, participantService); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RegisterParticipant10")] class RegisterParticipantRecordSchema10 : RegisterParticipantRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterParticipant" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ParticipantService", IsRequired=true)] EndpointAddressAugust2004 participantService; public RegisterParticipantRecordSchema10 ( string transactionId, Guid enlistmentId, ControlProtocol protocol, EndpointAddress participantService ) : base(transactionId, enlistmentId, protocol) { this.schemaId = id; if (participantService != null) { this.participantService = EndpointAddressAugust2004.FromEndpointAddress(participantService); } } } [DataContract (Name = "RegisterParticipant11")] class RegisterParticipantRecordSchema11 : RegisterParticipantRecordSchema { const string id = TraceRecord.EventIdBase + "RegisterParticipant11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "ParticipantService", IsRequired=true)] EndpointAddress10 participantService; public RegisterParticipantRecordSchema11 ( string transactionId, Guid enlistmentId, ControlProtocol protocol, EndpointAddress participantService ) : base(transactionId, enlistmentId, protocol) { this.schemaId = id; if (participantService != null) { this.participantService = EndpointAddress10.FromEndpointAddress(participantService); } } } //=================================================================================== [DataContract (Name = "ProtocolService")] class ProtocolServiceRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "ProtocolService" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.ProtocolServiceRecordSchema, this.protocolName, this.protocolId.ToString()); } [DataMember (Name = "ProtocolName", IsRequired=true)] string protocolName; [DataMember (Name = "ProtocolIdentifier", IsRequired=true)] Guid protocolId; public ProtocolServiceRecordSchema (string protocolName, Guid protocolId) { this.protocolName = protocolName; this.protocolId = protocolId; } } [DataContract (Name = "ParticipantRetryMessage")] class ParticipantRetryMessageRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "ParticipantRetryMessage" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; [DataMember (Name = "RetryCount", IsRequired=true)] int count; public ParticipantRetryMessageRecordSchema(string transactionId, Guid enlistmentId, int count) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; this.count = count; } } [DataContract (Name = "CoordinatorRetryMessage")] class CoordinatorRetryMessageRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "CoordinatorRetryMessage" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "RetryCount", IsRequired=true)] int count; public CoordinatorRetryMessageRecordSchema (string transactionId, int count) { this.transactionId = transactionId; this.count = count; } } //=================================================================================== // RecoverParticipantRecordSchema classes //==================================================================================== [DataContract (Name = "RecoverParticipant")] abstract class RecoverParticipantRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember(Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember(Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; protected RecoverParticipantRecordSchema ( string transactionId, Guid enlistmentId ) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; } public static RecoverParticipantRecordSchema Instance(string transactionId, Guid enlistmentId, EndpointAddress participantService, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RecoverParticipantRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RecoverParticipantRecordSchema10(transactionId, enlistmentId, participantService); case ProtocolVersion.Version11 : return new RecoverParticipantRecordSchema11(transactionId, enlistmentId, participantService); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RecoverParticipant10")] class RecoverParticipantRecordSchema10 : RecoverParticipantRecordSchema { const string id = TraceRecord.EventIdBase + "RecoverParticipant" + TraceRecord.NamespaceSuffix; [DataMember(Name = "ParticipantService")] EndpointAddressAugust2004 participantService; public RecoverParticipantRecordSchema10 ( string transactionId, Guid enlistmentId, EndpointAddress participantService ) : base(transactionId, enlistmentId) { this.schemaId = id; if (participantService != null) { this.participantService = EndpointAddressAugust2004.FromEndpointAddress(participantService); } } } [DataContract (Name = "RecoverParticipant11")] class RecoverParticipantRecordSchema11 : RecoverParticipantRecordSchema { const string id = TraceRecord.EventIdBase + "RecoverParticipant11" + TraceRecord.NamespaceSuffix; [DataMember(Name = "ParticipantService")] EndpointAddress10 participantService; public RecoverParticipantRecordSchema11 ( string transactionId, Guid enlistmentId, EndpointAddress participantService ) : base(transactionId, enlistmentId) { this.schemaId = id; if (participantService != null) { this.participantService = EndpointAddress10.FromEndpointAddress(participantService); } } } //=================================================================================== // RecoverCoordinatorRecordSchema classes //==================================================================================== [DataContract (Name = "RecoverCoordinator")] abstract class RecoverCoordinatorRecordSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; protected RecoverCoordinatorRecordSchema ( string transactionId ) { this.transactionId = transactionId; } public static RecoverCoordinatorRecordSchema Instance(string transactionId, EndpointAddress coordinatorService, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RecoverCoordinatorRecordSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RecoverCoordinatorRecordSchema10(transactionId, coordinatorService); case ProtocolVersion.Version11 : return new RecoverCoordinatorRecordSchema11(transactionId, coordinatorService); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RecoverCoordinator10")] class RecoverCoordinatorRecordSchema10 : RecoverCoordinatorRecordSchema { const string id = TraceRecord.EventIdBase + "RecoverCoordinator" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService")] EndpointAddressAugust2004 coordinatorService; public RecoverCoordinatorRecordSchema10 ( string transactionId, EndpointAddress coordinatorService ) : base(transactionId) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddressAugust2004.FromEndpointAddress(coordinatorService); } } } [DataContract (Name = "RecoverCoordinator11")] class RecoverCoordinatorRecordSchema11 : RecoverCoordinatorRecordSchema { const string id = TraceRecord.EventIdBase + "RecoverCoordinator11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService")] EndpointAddress10 coordinatorService; public RecoverCoordinatorRecordSchema11 ( string transactionId, EndpointAddress coordinatorService ) : base(transactionId) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddress10.FromEndpointAddress(coordinatorService); } } } //==================================================================================== [DataContract (Name = "ParticipantOutcome")] class ParticipantOutcomeRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "ParticipantOutcome" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "EnlistmentId", IsRequired=true)] Guid enlistmentId; [DataMember (Name = "Outcome", IsRequired=true)] string Outcome { get { return this.outcome.ToString(); } set { } } TransactionOutcome outcome; public ParticipantOutcomeRecordSchema ( string transactionId, Guid enlistmentId, TransactionOutcome outcome ) { this.transactionId = transactionId; this.enlistmentId = enlistmentId; this.outcome = outcome; } } [DataContract (Name = "CoordinatorOutcome")] class CoordinatorOutcomeRecordSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "CoordinatorOutcome" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "TransactionId", IsRequired=true)] string transactionId; [DataMember (Name = "Outcome", IsRequired=true)] string Outcome { get { return this.outcome.ToString(); } set { } } TransactionOutcome outcome; public CoordinatorOutcomeRecordSchema ( string transactionId, TransactionOutcome outcome ) { this.transactionId = transactionId; this.outcome = outcome; } } [DataContract (Name = "RegistrationCoordinatorFaulted")] class RegistrationCoordinatorFaultedSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "RegistrationCoordinatorFaulted" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Context", IsRequired=true)] CoordinationContext context; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString (); } set { } } ControlProtocol protocol; [DataMember (Name = "Fault")] string Fault { get { return Library.GetFaultCodeName(this.fault); } set { } } MessageFault fault; public RegistrationCoordinatorFaultedSchema ( CoordinationContext context, ControlProtocol protocol, MessageFault fault) { this.context = context; this.protocol = protocol; this.fault = fault; } } [DataContract (Name = "RegistrationCoordinatorFailed")] class RegistrationCoordinatorFailedSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "RegistrationCoordinatorFailed" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Context", IsRequired=true)] CoordinationContext context; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString(); } set { } } ControlProtocol protocol; public RegistrationCoordinatorFailedSchema ( CoordinationContext context, ControlProtocol protocol) { this.context = context; this.protocol = protocol; } } //=================================================================================== // RegistrationCoordinatorResponseInvalidMetadataSchema classes //==================================================================================== [DataContract (Name = "RegistrationCoordinatorResponseInvalidMetadata")] abstract class RegistrationCoordinatorResponseInvalidMetadataSchema : TraceRecord { protected string schemaId; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } [DataMember (Name = "Context", IsRequired=true)] CoordinationContext context; [DataMember (Name = "Protocol", IsRequired=true)] string Protocol { get { return this.protocol.ToString (); } set { } } ControlProtocol protocol; protected RegistrationCoordinatorResponseInvalidMetadataSchema ( CoordinationContext context, ControlProtocol protocol ) { this.context = context; this.protocol = protocol; } public static RegistrationCoordinatorResponseInvalidMetadataSchema Instance(CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService, ProtocolVersion protocolVersion) { ProtocolVersionHelper.AssertProtocolVersion(protocolVersion, typeof(RegistrationCoordinatorResponseInvalidMetadataSchema), "Instance"); switch (protocolVersion) { case ProtocolVersion.Version10 : return new RegistrationCoordinatorResponseInvalidMetadataSchema10(context, protocol, coordinatorService); case ProtocolVersion.Version11 : return new RegistrationCoordinatorResponseInvalidMetadataSchema11(context, protocol, coordinatorService); default: return null; // inaccessible path because we have asserted the protocol version } } } [DataContract (Name = "RegistrationCoordinatorResponseInvalidMetadata10")] class RegistrationCoordinatorResponseInvalidMetadataSchema10 : RegistrationCoordinatorResponseInvalidMetadataSchema { const string id = TraceRecord.EventIdBase + "RegistrationCoordinatorResponseInvalidMetadata" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService", IsRequired=true)] EndpointAddressAugust2004 coordinatorService; public RegistrationCoordinatorResponseInvalidMetadataSchema10 ( CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService) : base(context, protocol) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddressAugust2004.FromEndpointAddress(coordinatorService); } } } [DataContract (Name = "RegistrationCoordinatorResponseInvalidMetadata11")] class RegistrationCoordinatorResponseInvalidMetadataSchema11 : RegistrationCoordinatorResponseInvalidMetadataSchema { const string id = TraceRecord.EventIdBase + "RegistrationCoordinatorResponseInvalidMetadata11" + TraceRecord.NamespaceSuffix; [DataMember (Name = "CoordinatorService", IsRequired=true)] EndpointAddress10 coordinatorService; public RegistrationCoordinatorResponseInvalidMetadataSchema11 ( CoordinationContext context, ControlProtocol protocol, EndpointAddress coordinatorService) : base(context, protocol) { this.schemaId = id; if (coordinatorService != null) { this.coordinatorService = EndpointAddress10.FromEndpointAddress(coordinatorService); } } } //=================================================================================== [DataContract (Name = "PerformanceCounter")] class PerformanceCounterSchema : TraceRecord { const string schemaId = TraceRecord.EventIdBase + "PerformanceCounter" + TraceRecord.NamespaceSuffix; internal override string EventId { get { return schemaId; } } internal override void WriteTo (XmlWriter xmlWriter) { TransactionTraceRecord.SerializeRecord (xmlWriter, this); } public override string ToString() { return SR.GetString(SR.PerformanceCounterSchema, this.counterName); } [DataMember(Name = "Name", IsRequired=true)] string counterName; public PerformanceCounterSchema (string counterName) { this.counterName = counterName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
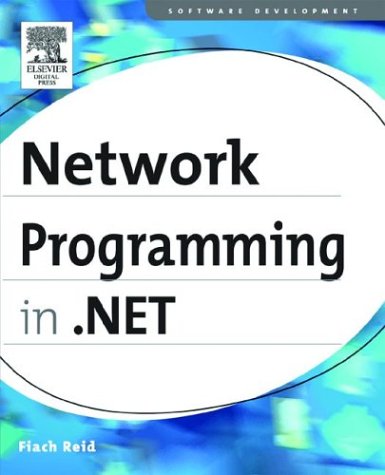
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultTypeArgumentAttribute.cs
- MethodBody.cs
- FileDataSourceCache.cs
- VersionPair.cs
- Root.cs
- SqlRecordBuffer.cs
- ButtonPopupAdapter.cs
- CannotUnloadAppDomainException.cs
- ResizeBehavior.cs
- ItemMap.cs
- HttpWriter.cs
- GradientBrush.cs
- Inline.cs
- HtmlPanelAdapter.cs
- ConstructorNeedsTagAttribute.cs
- ZipIOExtraFieldPaddingElement.cs
- TreeView.cs
- MetadataWorkspace.cs
- PropertyDescriptor.cs
- CAGDesigner.cs
- TypeResolver.cs
- SharedPersonalizationStateInfo.cs
- CharUnicodeInfo.cs
- Label.cs
- securestring.cs
- SizeIndependentAnimationStorage.cs
- WhitespaceRuleReader.cs
- WebPartTransformerCollection.cs
- SplayTreeNode.cs
- CroppedBitmap.cs
- SmtpTransport.cs
- SystemIcmpV4Statistics.cs
- ItemPager.cs
- ECDiffieHellmanCng.cs
- FileBasedResourceGroveler.cs
- SamlSecurityToken.cs
- CrossSiteScriptingValidation.cs
- SqlFactory.cs
- OdbcTransaction.cs
- BufferAllocator.cs
- xsdvalidator.cs
- CustomValidator.cs
- prompt.cs
- ToolTipService.cs
- BufferModesCollection.cs
- EarlyBoundInfo.cs
- ApplicationProxyInternal.cs
- DynamicPropertyHolder.cs
- ExceptionRoutedEventArgs.cs
- ReflectEventDescriptor.cs
- MailDefinitionBodyFileNameEditor.cs
- RequestCache.cs
- GlobalDataBindingHandler.cs
- WrapPanel.cs
- SqlFacetAttribute.cs
- ChannelServices.cs
- LeftCellWrapper.cs
- TargetPerspective.cs
- ComponentRenameEvent.cs
- AutoScrollExpandMessageFilter.cs
- ValueProviderWrapper.cs
- XmlnsDefinitionAttribute.cs
- Input.cs
- ColumnMapCopier.cs
- StrongNameMembershipCondition.cs
- ImmutableObjectAttribute.cs
- TextElementEnumerator.cs
- IdnElement.cs
- PageAsyncTask.cs
- ProfileGroupSettingsCollection.cs
- ContainerVisual.cs
- StateWorkerRequest.cs
- TextOnlyOutput.cs
- ScrollPattern.cs
- PartialToken.cs
- EastAsianLunisolarCalendar.cs
- DataColumnMapping.cs
- Stylus.cs
- TypeDescriptor.cs
- ResourceKey.cs
- StickyNoteHelper.cs
- ComplexLine.cs
- ExplicitDiscriminatorMap.cs
- StatusBar.cs
- DataGridViewComboBoxCell.cs
- GridViewColumnCollection.cs
- Metafile.cs
- ConsoleKeyInfo.cs
- Stack.cs
- DirectionalLight.cs
- Camera.cs
- DataSourceHelper.cs
- SqlGenerator.cs
- ArrayElementGridEntry.cs
- XmlSchemaSimpleTypeList.cs
- sqlcontext.cs
- XmlWellformedWriter.cs
- HtmlContainerControl.cs
- MinMaxParagraphWidth.cs
- DataServiceHostFactory.cs