Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / IO / Packaging / CompoundFile / VersionPair.cs / 1 / VersionPair.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A major, minor version number pair. // // History: // 06/20/2002: YoungGK: Created // 05/30/2003: LGolding: Ported to WCP tree. Split out from FormatVersion.cs. // 02/16/2005: YoungGK: Changed Class name to VersionPair from VersionTuple // //----------------------------------------------------------------------------- // Allow use of presharp warning numbers [6506] unknown to the compiler #pragma warning disable 1634, 1691 using System; using System.Globalization; #if PBTCOMPILER using MS.Utility; // For SR.cs #else using System.Windows; using System.Text; using MS.Internal.WindowsBase; // FriendAccessAllowed #endif namespace MS.Internal.IO.Packaging.CompoundFile { ///Class for a version pair which consists of major and minor numbers #if !PBTCOMPILER [FriendAccessAllowed] internal class VersionPair : IComparable #else internal class VersionPair #endif { //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Constructors ////// Constructor for VersionPair with given major and minor numbers. /// /// major part of version /// minor part of version internal VersionPair(Int16 major, Int16 minor) { if (major < 0) { throw new ArgumentOutOfRangeException("major", SR.Get(SRID.VersionNumberComponentNegative)); } if (minor < 0) { throw new ArgumentOutOfRangeException("minor", SR.Get(SRID.VersionNumberComponentNegative)); } _major = major; _minor = minor; } #endregion Constructors //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Public Properties ////// Major number of version /// public Int16 Major { get { return _major; } } ////// Minor number of version /// public Int16 Minor { get { return _minor; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public methods #if !PBTCOMPILER ////// Returns a string that represents the current VersionPair object. /// The string is of the form (major,minor). /// public override string ToString() { StringBuilder stringFormBuilder = new StringBuilder("("); stringFormBuilder.Append(_major); stringFormBuilder.Append(","); stringFormBuilder.Append(_minor); stringFormBuilder.Append(")"); return stringFormBuilder.ToString(); } #endif #endregion #region Operators ////// == comparison operator /// /// version to be compared /// version to be compared public static bool operator ==(VersionPair v1, VersionPair v2) { bool result = false; // If both v1 & v2 are null they are same if ((Object) v1 == null && (Object) v2 == null) { result = true; } // Do comparison only if both v1 and v2 are not null else if ((Object) v1 != null && (Object) v2 != null) { if (v1.Major == v2.Major && v1.Minor == v2.Minor) { result = true; } } return result; } ////// != comparison operator /// /// version to be compared /// version to be compared public static bool operator !=(VersionPair v1, VersionPair v2) { // == is previously define so it can be used return !(v1 == v2); } #if !PBTCOMPILER ////// "less than" comparison operator /// /// version to be compared /// version to be compared public static bool operator <(VersionPair v1, VersionPair v2) { bool result = false; if ((Object) v1 == null && (Object) v2 != null) { result = true; } else if ((Object) v1 != null && (object) v2 != null) { // == is previously define so it can be used if (v1.Major < v2.Major || ((v1.Major == v2.Major) && (v1.Minor < v2.Minor))) { result = true; } } return result; } ////// "greater than" comparison operator /// /// version to be compared /// version to be compared public static bool operator >(VersionPair v1, VersionPair v2) { bool result = false; if ((Object) v1 != null && (Object) v2 == null) { result = true; } // Comare only if neither v1 nor v2 are not null else if ((Object) v1 != null && (object) v2 != null) { // < and == are previously define so it can be used if (!(v1 < v2) && v1 != v2) { return true; } } return result; } ////// "less than or equal" comparison operator /// /// version to be compared /// version to be compared public static bool operator <=(VersionPair v1, VersionPair v2) { if (!(v1 > v2)) { return true; } return false; } ////// "greater than or equal" comparison operator /// /// version to be compared /// version to be compared public static bool operator >=(VersionPair v1, VersionPair v2) { if (!(v1 < v2)) { return true; } return false; } #endif ////// Eaual comparison operator /// /// Object to compare ///true if the object is equal to this instance public override bool Equals(Object obj) { if (obj == null) { return false; } if (obj.GetType() != GetType()) { return false; } VersionPair v = (VersionPair) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (this != v) { return false; } #pragma warning restore 6506 return true; } ////// Hash code /// public override int GetHashCode() { return (_major << 16 + _minor); } #if !PBTCOMPILER ////// Compare this instance to the object /// /// Object to compare ///Less than 0 - This instance is less than obj /// Zero - This instance is equal to obj /// Greater than 0 - This instance is greater than obj public int CompareTo(Object obj) { if (obj == null) { return 1; } if (obj.GetType() != GetType()) { throw new ArgumentException(SR.Get(SRID.ExpectedVersionPairObject)); } VersionPair v = (VersionPair) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (this.Equals(obj)) // equal { return 0; } // less than else if (this < v) { return -1; } #pragma warning restore 6506 // greater than return 1; } #endif #endregion Operators //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Member Variables private Int16 _major; // Major number private Int16 _minor; // Minor number #endregion Member Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // A major, minor version number pair. // // History: // 06/20/2002: YoungGK: Created // 05/30/2003: LGolding: Ported to WCP tree. Split out from FormatVersion.cs. // 02/16/2005: YoungGK: Changed Class name to VersionPair from VersionTuple // //----------------------------------------------------------------------------- // Allow use of presharp warning numbers [6506] unknown to the compiler #pragma warning disable 1634, 1691 using System; using System.Globalization; #if PBTCOMPILER using MS.Utility; // For SR.cs #else using System.Windows; using System.Text; using MS.Internal.WindowsBase; // FriendAccessAllowed #endif namespace MS.Internal.IO.Packaging.CompoundFile { ///Class for a version pair which consists of major and minor numbers #if !PBTCOMPILER [FriendAccessAllowed] internal class VersionPair : IComparable #else internal class VersionPair #endif { //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- #region Constructors ////// Constructor for VersionPair with given major and minor numbers. /// /// major part of version /// minor part of version internal VersionPair(Int16 major, Int16 minor) { if (major < 0) { throw new ArgumentOutOfRangeException("major", SR.Get(SRID.VersionNumberComponentNegative)); } if (minor < 0) { throw new ArgumentOutOfRangeException("minor", SR.Get(SRID.VersionNumberComponentNegative)); } _major = major; _minor = minor; } #endregion Constructors //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Public Properties ////// Major number of version /// public Int16 Major { get { return _major; } } ////// Minor number of version /// public Int16 Minor { get { return _minor; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public methods #if !PBTCOMPILER ////// Returns a string that represents the current VersionPair object. /// The string is of the form (major,minor). /// public override string ToString() { StringBuilder stringFormBuilder = new StringBuilder("("); stringFormBuilder.Append(_major); stringFormBuilder.Append(","); stringFormBuilder.Append(_minor); stringFormBuilder.Append(")"); return stringFormBuilder.ToString(); } #endif #endregion #region Operators ////// == comparison operator /// /// version to be compared /// version to be compared public static bool operator ==(VersionPair v1, VersionPair v2) { bool result = false; // If both v1 & v2 are null they are same if ((Object) v1 == null && (Object) v2 == null) { result = true; } // Do comparison only if both v1 and v2 are not null else if ((Object) v1 != null && (Object) v2 != null) { if (v1.Major == v2.Major && v1.Minor == v2.Minor) { result = true; } } return result; } ////// != comparison operator /// /// version to be compared /// version to be compared public static bool operator !=(VersionPair v1, VersionPair v2) { // == is previously define so it can be used return !(v1 == v2); } #if !PBTCOMPILER ////// "less than" comparison operator /// /// version to be compared /// version to be compared public static bool operator <(VersionPair v1, VersionPair v2) { bool result = false; if ((Object) v1 == null && (Object) v2 != null) { result = true; } else if ((Object) v1 != null && (object) v2 != null) { // == is previously define so it can be used if (v1.Major < v2.Major || ((v1.Major == v2.Major) && (v1.Minor < v2.Minor))) { result = true; } } return result; } ////// "greater than" comparison operator /// /// version to be compared /// version to be compared public static bool operator >(VersionPair v1, VersionPair v2) { bool result = false; if ((Object) v1 != null && (Object) v2 == null) { result = true; } // Comare only if neither v1 nor v2 are not null else if ((Object) v1 != null && (object) v2 != null) { // < and == are previously define so it can be used if (!(v1 < v2) && v1 != v2) { return true; } } return result; } ////// "less than or equal" comparison operator /// /// version to be compared /// version to be compared public static bool operator <=(VersionPair v1, VersionPair v2) { if (!(v1 > v2)) { return true; } return false; } ////// "greater than or equal" comparison operator /// /// version to be compared /// version to be compared public static bool operator >=(VersionPair v1, VersionPair v2) { if (!(v1 < v2)) { return true; } return false; } #endif ////// Eaual comparison operator /// /// Object to compare ///true if the object is equal to this instance public override bool Equals(Object obj) { if (obj == null) { return false; } if (obj.GetType() != GetType()) { return false; } VersionPair v = (VersionPair) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (this != v) { return false; } #pragma warning restore 6506 return true; } ////// Hash code /// public override int GetHashCode() { return (_major << 16 + _minor); } #if !PBTCOMPILER ////// Compare this instance to the object /// /// Object to compare ///Less than 0 - This instance is less than obj /// Zero - This instance is equal to obj /// Greater than 0 - This instance is greater than obj public int CompareTo(Object obj) { if (obj == null) { return 1; } if (obj.GetType() != GetType()) { throw new ArgumentException(SR.Get(SRID.ExpectedVersionPairObject)); } VersionPair v = (VersionPair) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (this.Equals(obj)) // equal { return 0; } // less than else if (this < v) { return -1; } #pragma warning restore 6506 // greater than return 1; } #endif #endregion Operators //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ // None //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Member Variables private Int16 _major; // Major number private Int16 _minor; // Minor number #endregion Member Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
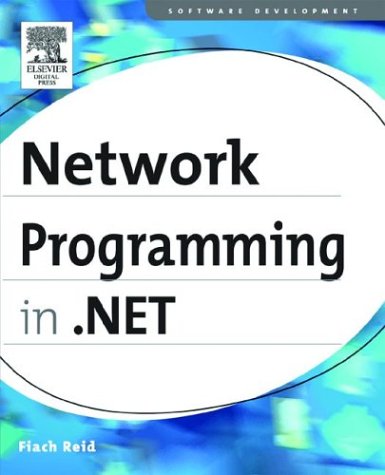
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LocationSectionRecord.cs
- KeySplineConverter.cs
- CookieHandler.cs
- RemotingServices.cs
- CacheForPrimitiveTypes.cs
- UnionCqlBlock.cs
- WebConfigurationManager.cs
- BinaryMethodMessage.cs
- BufferedWebEventProvider.cs
- Mappings.cs
- AxWrapperGen.cs
- HttpApplication.cs
- VisualCollection.cs
- WorkflowApplicationAbortedException.cs
- RichTextBoxContextMenu.cs
- TextElementAutomationPeer.cs
- ConstructorArgumentAttribute.cs
- SqlProfileProvider.cs
- RoutedUICommand.cs
- FormatConvertedBitmap.cs
- ModelItemKeyValuePair.cs
- SoapServerMethod.cs
- SafeHandles.cs
- DateTimeSerializationSection.cs
- ButtonFieldBase.cs
- FormView.cs
- LinqDataSourceHelper.cs
- QilGenerator.cs
- Int64AnimationBase.cs
- Effect.cs
- BaseTemplateBuildProvider.cs
- HttpListenerContext.cs
- HttpRuntime.cs
- WebRequest.cs
- EntityDataSourceEntityTypeFilterItem.cs
- StyleSelector.cs
- PrimitiveDataContract.cs
- FormViewInsertedEventArgs.cs
- XdrBuilder.cs
- NetworkStream.cs
- HeaderCollection.cs
- ControlPropertyNameConverter.cs
- ServiceDiscoveryElement.cs
- TrackingDataItemValue.cs
- dtdvalidator.cs
- ValuePatternIdentifiers.cs
- MemberInfoSerializationHolder.cs
- Missing.cs
- Assert.cs
- DrawingImage.cs
- AttributeQuery.cs
- DataViewManagerListItemTypeDescriptor.cs
- FileChangesMonitor.cs
- TdsValueSetter.cs
- UIElementHelper.cs
- RecordsAffectedEventArgs.cs
- StdValidatorsAndConverters.cs
- GridViewColumnHeader.cs
- AutomationElementIdentifiers.cs
- ListView.cs
- EnumUnknown.cs
- AspNetCompatibilityRequirementsMode.cs
- SqlCommandBuilder.cs
- MD5.cs
- ProxyWebPart.cs
- FixedTextContainer.cs
- NetStream.cs
- InvalidProgramException.cs
- RegisteredArrayDeclaration.cs
- Bind.cs
- PathGeometry.cs
- ComponentChangedEvent.cs
- Exception.cs
- ServiceOperationHelpers.cs
- BindingMAnagerBase.cs
- OracleRowUpdatedEventArgs.cs
- SqlProcedureAttribute.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- FeedUtils.cs
- DataSourceCache.cs
- Publisher.cs
- ConstraintConverter.cs
- TileBrush.cs
- DataStreams.cs
- ForeignKeyFactory.cs
- SelectorItemAutomationPeer.cs
- MatrixCamera.cs
- DataTableReader.cs
- OleDbSchemaGuid.cs
- Trace.cs
- TemplateBuilder.cs
- coordinator.cs
- GrowingArray.cs
- ObjectComplexPropertyMapping.cs
- StringUtil.cs
- EntityTypeEmitter.cs
- BufferedWebEventProvider.cs
- DataGridViewColumn.cs
- MatrixTransform3D.cs
- DoubleUtil.cs