Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / AssemblyCache.cs / 1305376 / AssemblyCache.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Concurrent; using System.Diagnostics; using System.Reflection; using System.Web.Configuration; using System.Web.Script; // Caches Assembly APIs to improve performance internal static class AssemblyCache { // PERF: Cache reference to System.Web.Extensions assembly. Use ScriptManager since it's guaranteed to be in S.W.E public static readonly Assembly SystemWebExtensions = typeof(ScriptManager).Assembly; public static readonly Assembly SystemWeb = typeof(Page).Assembly; private static CompilationSection _compilationSection; internal static bool _useCompilationSection = true; // Maps string (assembly name) to Assembly private static readonly Hashtable _assemblyCache = Hashtable.Synchronized(new Hashtable()); // Maps assembly to Version // internal so it can be manipulated by the unit test suite internal static readonly Hashtable _versionCache = Hashtable.Synchronized(new Hashtable()); // Maps an assembly to its ajax framework assembly attribute. If it doesn't have one, it maps it to a null value private static readonly ConcurrentDictionary_ajaxAssemblyAttributeCache = new ConcurrentDictionary (); private static CompilationSection CompilationSection { get { if (_compilationSection == null) { _compilationSection = RuntimeConfig.GetAppConfig().Compilation; } return _compilationSection; } } public static Version GetVersion(Assembly assembly) { Debug.Assert(assembly != null); Version version = (Version)_versionCache[assembly]; if (version == null) { // use new AssemblyName() instead of assembly.GetName() so it works in medium trust version = new AssemblyName(assembly.FullName).Version; _versionCache[assembly] = version; } return version; } public static Assembly Load(string assemblyName) { Debug.Assert(!String.IsNullOrEmpty(assemblyName)); Assembly assembly = (Assembly)_assemblyCache[assemblyName]; if (assembly == null) { // _useCompilationSection must be set to false in a unit test environment since there // is no http runtime, and therefore no trust level is set. if (_useCompilationSection) { assembly = CompilationSection.LoadAssembly(assemblyName, true); } else { assembly = Assembly.Load(assemblyName); } _assemblyCache[assemblyName] = assembly; } return assembly; } public static bool IsAjaxFrameworkAssembly(Assembly assembly) { return (GetAjaxFrameworkAssemblyAttribute(assembly) != null); } public static AjaxFrameworkAssemblyAttribute GetAjaxFrameworkAssemblyAttribute(Assembly assembly) { Debug.Assert(assembly != null); AjaxFrameworkAssemblyAttribute ajaxFrameworkAssemblyAttribute = null; if (!_ajaxAssemblyAttributeCache.TryGetValue(assembly, out ajaxFrameworkAssemblyAttribute)) { foreach (Attribute attribute in assembly.GetCustomAttributes(false)) { if (attribute is AjaxFrameworkAssemblyAttribute) { ajaxFrameworkAssemblyAttribute = (AjaxFrameworkAssemblyAttribute)attribute; break; } } _ajaxAssemblyAttributeCache.TryAdd(assembly, ajaxFrameworkAssemblyAttribute); } return ajaxFrameworkAssemblyAttribute; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.Collections.Concurrent; using System.Diagnostics; using System.Reflection; using System.Web.Configuration; using System.Web.Script; // Caches Assembly APIs to improve performance internal static class AssemblyCache { // PERF: Cache reference to System.Web.Extensions assembly. Use ScriptManager since it's guaranteed to be in S.W.E public static readonly Assembly SystemWebExtensions = typeof(ScriptManager).Assembly; public static readonly Assembly SystemWeb = typeof(Page).Assembly; private static CompilationSection _compilationSection; internal static bool _useCompilationSection = true; // Maps string (assembly name) to Assembly private static readonly Hashtable _assemblyCache = Hashtable.Synchronized(new Hashtable()); // Maps assembly to Version // internal so it can be manipulated by the unit test suite internal static readonly Hashtable _versionCache = Hashtable.Synchronized(new Hashtable()); // Maps an assembly to its ajax framework assembly attribute. If it doesn't have one, it maps it to a null value private static readonly ConcurrentDictionary_ajaxAssemblyAttributeCache = new ConcurrentDictionary (); private static CompilationSection CompilationSection { get { if (_compilationSection == null) { _compilationSection = RuntimeConfig.GetAppConfig().Compilation; } return _compilationSection; } } public static Version GetVersion(Assembly assembly) { Debug.Assert(assembly != null); Version version = (Version)_versionCache[assembly]; if (version == null) { // use new AssemblyName() instead of assembly.GetName() so it works in medium trust version = new AssemblyName(assembly.FullName).Version; _versionCache[assembly] = version; } return version; } public static Assembly Load(string assemblyName) { Debug.Assert(!String.IsNullOrEmpty(assemblyName)); Assembly assembly = (Assembly)_assemblyCache[assemblyName]; if (assembly == null) { // _useCompilationSection must be set to false in a unit test environment since there // is no http runtime, and therefore no trust level is set. if (_useCompilationSection) { assembly = CompilationSection.LoadAssembly(assemblyName, true); } else { assembly = Assembly.Load(assemblyName); } _assemblyCache[assemblyName] = assembly; } return assembly; } public static bool IsAjaxFrameworkAssembly(Assembly assembly) { return (GetAjaxFrameworkAssemblyAttribute(assembly) != null); } public static AjaxFrameworkAssemblyAttribute GetAjaxFrameworkAssemblyAttribute(Assembly assembly) { Debug.Assert(assembly != null); AjaxFrameworkAssemblyAttribute ajaxFrameworkAssemblyAttribute = null; if (!_ajaxAssemblyAttributeCache.TryGetValue(assembly, out ajaxFrameworkAssemblyAttribute)) { foreach (Attribute attribute in assembly.GetCustomAttributes(false)) { if (attribute is AjaxFrameworkAssemblyAttribute) { ajaxFrameworkAssemblyAttribute = (AjaxFrameworkAssemblyAttribute)attribute; break; } } _ajaxAssemblyAttributeCache.TryAdd(assembly, ajaxFrameworkAssemblyAttribute); } return ajaxFrameworkAssemblyAttribute; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
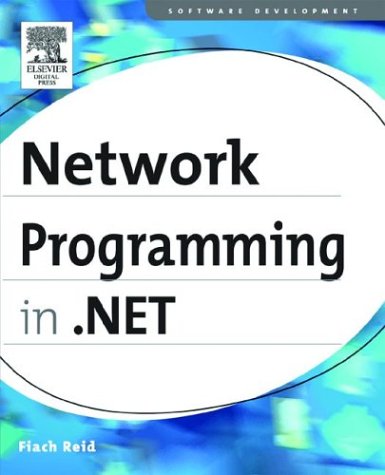
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VoiceObjectToken.cs
- FontStretches.cs
- DynamicObject.cs
- GridEntry.cs
- FrameworkName.cs
- SqlInternalConnectionSmi.cs
- IEnumerable.cs
- SamlConditions.cs
- Query.cs
- connectionpool.cs
- ErrorLog.cs
- LinqDataSourceSelectEventArgs.cs
- XamlFigureLengthSerializer.cs
- ConnectionStringsSection.cs
- AmbientLight.cs
- BufferedReadStream.cs
- PersonalizationStateInfoCollection.cs
- DocumentViewerBaseAutomationPeer.cs
- ProgressBar.cs
- TimeoutException.cs
- FilterElement.cs
- ResourceProviderFactory.cs
- PrimitiveSchema.cs
- BamlLocalizationDictionary.cs
- ImpersonateTokenRef.cs
- TraceSwitch.cs
- Vector3DCollectionValueSerializer.cs
- WindowPatternIdentifiers.cs
- CalendarModeChangedEventArgs.cs
- ReflectionTypeLoadException.cs
- EventRoute.cs
- Container.cs
- Size.cs
- CodeSnippetStatement.cs
- CodeCommentStatementCollection.cs
- TrailingSpaceComparer.cs
- EventHandlerList.cs
- CodeArrayCreateExpression.cs
- DesignerCategoryAttribute.cs
- PathNode.cs
- RuleSettings.cs
- MimeFormReflector.cs
- RealizationContext.cs
- FormsAuthenticationEventArgs.cs
- MobileUserControlDesigner.cs
- RegexRunnerFactory.cs
- GridViewAutomationPeer.cs
- Control.cs
- OdbcConnectionPoolProviderInfo.cs
- TextSpanModifier.cs
- DecimalAnimation.cs
- BindingContext.cs
- QueryConverter.cs
- RangeValuePatternIdentifiers.cs
- CookielessHelper.cs
- PeerNearMe.cs
- ClientOperation.cs
- CodeThrowExceptionStatement.cs
- XmlChoiceIdentifierAttribute.cs
- wmiprovider.cs
- DocumentSequence.cs
- DataGridViewAdvancedBorderStyle.cs
- ThreadStateException.cs
- documentsequencetextpointer.cs
- WindowsIdentity.cs
- CompilerParameters.cs
- WebPartVerbsEventArgs.cs
- _HTTPDateParse.cs
- Stack.cs
- CollectionViewGroup.cs
- DeliveryStrategy.cs
- NotSupportedException.cs
- LabelDesigner.cs
- WCFBuildProvider.cs
- ItemsControlAutomationPeer.cs
- TopClause.cs
- Container.cs
- RoutedEvent.cs
- SqlException.cs
- DrawingCollection.cs
- Container.cs
- DataObjectCopyingEventArgs.cs
- GridViewSelectEventArgs.cs
- ToolboxItemAttribute.cs
- OracleBoolean.cs
- XmlSignatureManifest.cs
- CompilerCollection.cs
- DateTimeConstantAttribute.cs
- Executor.cs
- EntitySetBase.cs
- WebBrowser.cs
- ColorMap.cs
- X500Name.cs
- InvalidFilterCriteriaException.cs
- Stream.cs
- SyncMethodInvoker.cs
- TrustLevelCollection.cs
- RegistryKey.cs
- LinqDataSourceDisposeEventArgs.cs
- ExpressionValueEditor.cs