Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Threading / DispatcherExceptionEventArgs.cs / 1305600 / DispatcherExceptionEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Arguments for the events that convey an exception that was // raised while executing code via the Dispatcher. // // History: // 07/22/2003 : KenLai - Created // 05/25/2005 : WeibZ - Rename it // //--------------------------------------------------------------------------- using System.Diagnostics; using System; namespace System.Windows.Threading { ////// Arguments for the events that convey an exception that was raised /// while executing code via the dispatcher. /// public sealed class DispatcherUnhandledExceptionEventArgs : DispatcherEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- // Initialize a new event argument. internal DispatcherUnhandledExceptionEventArgs(Dispatcher dispatcher) : base(dispatcher) { } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The exception that was raised while executing code via the /// dispatcher. /// public Exception Exception { get { return _exception; } } ////// Whether or not the exception event has been handled. /// Other handlers should respect this field, and not display any /// UI in response to being notified. Passive responses (such as /// logging) can still be done. /// If no handler sets this value to true, default UI will be shown. /// public bool Handled { get { return _handled; } set { // Only allow to be set true. if (value == true) { _handled = value; } } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Initialize the preallocated args class for use. /// ////// This method MUST NOT FAIL because it is called from an exception /// handler: do not do any heavy lifting or allocate more memory. /// This initialization step is separated from the constructor /// precisely because we wanted to preallocate the memory and avoid /// hitting a secondary exception in the out-of-memory case. /// /// /// The exception that was raised while executing code via the /// dispatcher /// /// /// Whether or not the exception has been handled /// internal void Initialize(Exception exception, bool handled) { Debug.Assert(exception != null); _exception = exception; _handled = handled; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Exception _exception; private bool _handled; } ////// Delegate for the events that convey the state of the UiConext /// in response to various actions that involve items. /// public delegate void DispatcherUnhandledExceptionEventHandler(object sender, DispatcherUnhandledExceptionEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Arguments for the events that convey an exception that was // raised while executing code via the Dispatcher. // // History: // 07/22/2003 : KenLai - Created // 05/25/2005 : WeibZ - Rename it // //--------------------------------------------------------------------------- using System.Diagnostics; using System; namespace System.Windows.Threading { ////// Arguments for the events that convey an exception that was raised /// while executing code via the dispatcher. /// public sealed class DispatcherUnhandledExceptionEventArgs : DispatcherEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- // Initialize a new event argument. internal DispatcherUnhandledExceptionEventArgs(Dispatcher dispatcher) : base(dispatcher) { } //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// The exception that was raised while executing code via the /// dispatcher. /// public Exception Exception { get { return _exception; } } ////// Whether or not the exception event has been handled. /// Other handlers should respect this field, and not display any /// UI in response to being notified. Passive responses (such as /// logging) can still be done. /// If no handler sets this value to true, default UI will be shown. /// public bool Handled { get { return _handled; } set { // Only allow to be set true. if (value == true) { _handled = value; } } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Initialize the preallocated args class for use. /// ////// This method MUST NOT FAIL because it is called from an exception /// handler: do not do any heavy lifting or allocate more memory. /// This initialization step is separated from the constructor /// precisely because we wanted to preallocate the memory and avoid /// hitting a secondary exception in the out-of-memory case. /// /// /// The exception that was raised while executing code via the /// dispatcher /// /// /// Whether or not the exception has been handled /// internal void Initialize(Exception exception, bool handled) { Debug.Assert(exception != null); _exception = exception; _handled = handled; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Exception _exception; private bool _handled; } ////// Delegate for the events that convey the state of the UiConext /// in response to various actions that involve items. /// public delegate void DispatcherUnhandledExceptionEventHandler(object sender, DispatcherUnhandledExceptionEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
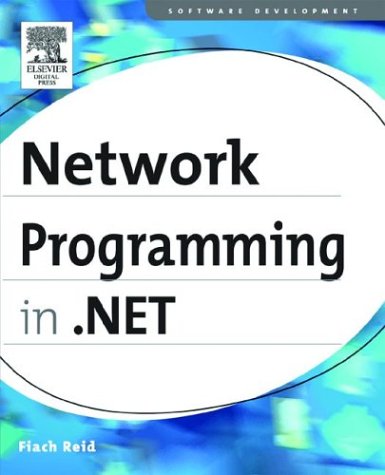
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Facet.cs
- PageCatalogPart.cs
- DesignerRegion.cs
- RewritingSimplifier.cs
- VisualTreeUtils.cs
- QilGeneratorEnv.cs
- ButtonFieldBase.cs
- HttpCacheParams.cs
- AngleUtil.cs
- UserControlCodeDomTreeGenerator.cs
- BaseTreeIterator.cs
- UnsafeNativeMethods.cs
- connectionpool.cs
- _LocalDataStoreMgr.cs
- TableProviderWrapper.cs
- DrawingContextDrawingContextWalker.cs
- XmlCharacterData.cs
- DATA_BLOB.cs
- UInt64.cs
- DataGridAutomationPeer.cs
- CodeStatement.cs
- TrackingStringDictionary.cs
- PropertyValueUIItem.cs
- ValuePattern.cs
- SmtpNetworkElement.cs
- CollectionBuilder.cs
- XmlWriter.cs
- DesignerCategoryAttribute.cs
- CapabilitiesRule.cs
- RoutedCommand.cs
- PrintingPermissionAttribute.cs
- MutexSecurity.cs
- Events.cs
- ConditionalAttribute.cs
- RSAPKCS1KeyExchangeFormatter.cs
- MexBindingElement.cs
- Expr.cs
- DataGrid.cs
- XPSSignatureDefinition.cs
- Converter.cs
- ThreadSafeList.cs
- CompilerGeneratedAttribute.cs
- PlainXmlDeserializer.cs
- ListSortDescription.cs
- PreloadedPackages.cs
- AddDataControlFieldDialog.cs
- StaticContext.cs
- CodeMethodInvokeExpression.cs
- SmtpException.cs
- DataPager.cs
- PrintDialog.cs
- _DynamicWinsockMethods.cs
- XmlQueryRuntime.cs
- ToolStripDropTargetManager.cs
- TextRenderingModeValidation.cs
- TextBlockAutomationPeer.cs
- TrustManagerMoreInformation.cs
- DiscoveryMessageSequenceGenerator.cs
- AnnotationResourceChangedEventArgs.cs
- CopyNamespacesAction.cs
- EntityTypeEmitter.cs
- MergeExecutor.cs
- _SSPISessionCache.cs
- SystemColors.cs
- SQLChars.cs
- EncryptedPackage.cs
- SafeNativeHandle.cs
- Rotation3DAnimationUsingKeyFrames.cs
- Line.cs
- OutputCacheProfileCollection.cs
- QueryAccessibilityHelpEvent.cs
- XmlAnyAttributeAttribute.cs
- SHA256Cng.cs
- PropertyGridCommands.cs
- TextServicesLoader.cs
- PenThreadWorker.cs
- AVElementHelper.cs
- StatusBarAutomationPeer.cs
- FixedSOMFixedBlock.cs
- Grid.cs
- HttpHandlerActionCollection.cs
- XPathMultyIterator.cs
- WebRequestModulesSection.cs
- FixedBufferAttribute.cs
- AdornerDecorator.cs
- ObjectReaderCompiler.cs
- StorageFunctionMapping.cs
- TypeUtil.cs
- Utility.cs
- IntPtr.cs
- FindCriteria11.cs
- MediaPlayer.cs
- BamlBinaryWriter.cs
- MatrixTransform.cs
- CalculatedColumn.cs
- ToggleButtonAutomationPeer.cs
- SizeValueSerializer.cs
- MergablePropertyAttribute.cs
- ExpressionBindingCollection.cs
- DeploymentExceptionMapper.cs