Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / SystemThemeKey.cs / 1 / SystemThemeKey.cs
//---------------------------------------------------------------------------- // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.Text; using System.Windows; using System.Windows.Markup; using System.ComponentModel; using System.Diagnostics; namespace System.Windows { ////// Implements ResourceKey to create unique keys for our system resources. /// Keys will be exposed publicly only with the ResourceKey API. /// [TypeConverter(typeof(System.Windows.Markup.SystemKeyConverter))] internal class SystemThemeKey : ResourceKey { ////// Constructs a new instance of the key with the given ID. /// /// The internal, unique ID of the system resource. internal SystemThemeKey(SystemResourceKeyID id) { _id = id; Debug.Assert(id > SystemResourceKeyID.InternalSystemThemeStylesStart && id < SystemResourceKeyID.InternalSystemThemeStylesEnd); } ////// Used to determine where to look for the resource dictionary that holds this resource. /// public override Assembly Assembly { get { if (_presentationFrameworkAssembly == null) { _presentationFrameworkAssembly = typeof(FrameworkElement).Assembly; } return _presentationFrameworkAssembly; } } ////// Determines if the passed in object is equal to this object. /// Two keys will be equal if they both have the same ID. /// /// The object to compare with. ///True if the objects are equal. False otherwise. public override bool Equals(object o) { SystemThemeKey key = o as SystemThemeKey; if (key != null) { return key._id == this._id; } return false; } ////// Serves as a hash function for a particular type. /// public override int GetHashCode() { return (int)_id; } ////// get string representation of this key /// ///the string representation of the key public override string ToString() { return _id.ToString(); } internal SystemResourceKeyID InternalKey { get { return _id; } } private SystemResourceKeyID _id; private static Assembly _presentationFrameworkAssembly; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
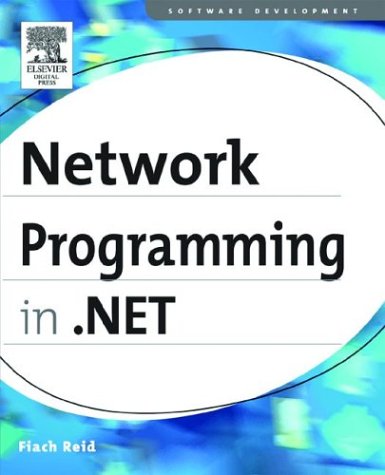
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StrokeSerializer.cs
- FilePrompt.cs
- ControllableStoryboardAction.cs
- RbTree.cs
- SBCSCodePageEncoding.cs
- DependentList.cs
- GeneralTransform.cs
- DataGridViewToolTip.cs
- StrongBox.cs
- ContextStack.cs
- ClientTargetCollection.cs
- IndentTextWriter.cs
- BamlTreeMap.cs
- AdRotator.cs
- HiddenField.cs
- ComponentCollection.cs
- PackWebResponse.cs
- ResourceAssociationType.cs
- TaskDesigner.cs
- AmbiguousMatchException.cs
- MarginCollapsingState.cs
- ApplicationSecurityInfo.cs
- SystemDropShadowChrome.cs
- unsafenativemethodstextservices.cs
- TableLayoutPanelCodeDomSerializer.cs
- EdmComplexTypeAttribute.cs
- Schema.cs
- LicFileLicenseProvider.cs
- ScriptingWebServicesSectionGroup.cs
- SqlDataSourceFilteringEventArgs.cs
- WindowsGraphicsWrapper.cs
- PointCollectionValueSerializer.cs
- XmlChildNodes.cs
- SelectionWordBreaker.cs
- SoapElementAttribute.cs
- Literal.cs
- RequestedSignatureDialog.cs
- ContentWrapperAttribute.cs
- MouseWheelEventArgs.cs
- DefinitionUpdate.cs
- CodeTypeDeclarationCollection.cs
- COM2ComponentEditor.cs
- InstanceDataCollectionCollection.cs
- unitconverter.cs
- KeysConverter.cs
- ListViewGroupConverter.cs
- TagNameToTypeMapper.cs
- recordstate.cs
- xmlglyphRunInfo.cs
- StandardCommands.cs
- AnnotationObservableCollection.cs
- ReferentialConstraint.cs
- RuntimeWrappedException.cs
- TableAutomationPeer.cs
- QueryOutputWriter.cs
- ConfigurationFileMap.cs
- Peer.cs
- WebPartDescription.cs
- DialogResultConverter.cs
- SqlResolver.cs
- TextShapeableCharacters.cs
- Menu.cs
- GridErrorDlg.cs
- RuntimeEnvironment.cs
- ApplicationGesture.cs
- ArraySet.cs
- ServicePoint.cs
- XmlException.cs
- StatusBarPanel.cs
- BaseComponentEditor.cs
- DataObjectSettingDataEventArgs.cs
- IntPtr.cs
- ArgumentOutOfRangeException.cs
- SizeConverter.cs
- BaseCodeDomTreeGenerator.cs
- CodeDomConfigurationHandler.cs
- StatusBar.cs
- EditingCoordinator.cs
- XpsPartBase.cs
- FontFamilyValueSerializer.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- PipeStream.cs
- SQLResource.cs
- QualificationDataItem.cs
- TranslateTransform.cs
- UserControlParser.cs
- SpoolingTaskBase.cs
- FixedSOMElement.cs
- RefreshEventArgs.cs
- SctClaimDictionary.cs
- WsdlInspector.cs
- RewritingPass.cs
- ScrollableControl.cs
- WebControlAdapter.cs
- FileSystemInfo.cs
- SspiNegotiationTokenProviderState.cs
- Renderer.cs
- TemplateBindingExpression.cs
- MultiBindingExpression.cs
- ToolBarOverflowPanel.cs